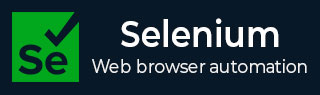
- Selenium Tutorial
- Selenium - Home
- Selenium - Overview
- Selenium - Components
- Selenium - Automation Testing
- Selenium - Environment Setup
- Selenium - Remote Control
- Selenium IDE Tutorial
- Selenium - IDE Introduction
- Selenium - Features
- Selenium - Limitations
- Selenium - Installation
- Selenium - Creating Tests
- Selenium - Creating Script
- Selenium - Store Variables
- Selenium - Alerts & Popups
- Selenium - Selenese Commands
- Selenium - Actions Commands
- Selenium - Accessors Commands
- Selenium - Assertions Commands
- Selenium - Assert/Verify Methods
- Selenium - Locating Strategies
- Selenium - Script Debugging
- Selenium - Verification Points
- Selenium - Pattern Matching
- Selenium - JSON Data File
- Selenium - Browser Execution
- Selenium - User Extensions
- Selenium - Code Export
- Selenium - JavaScript Functions
- Selenium - Plugins
- Selenium WebDriver Tutorial
- Selenium - Introduction
- Selenium WebDriver vs RC
- Selenium - Installation
- Selenium - First Test Script
- Selenium - Driver Sessions
- Selenium - Browser Options
- Selenium - Chrome Options
- Selenium - Edge Options
- Selenium - Firefox Options
- Selenium - Safari Options
- Selenium Web Elements
- Selenium - File Upload
- Selenium - Locator Strategies
- Selenium - Relative Locators
- Selenium - Finders
- Selenium - Find All Links
- Selenium - User Interactions
- Selenium - WebElement Commands
- Selenium - Browser Interactions
- Selenium - Browser Commands
- Selenium - Browser Navigation
- Selenium - Alerts & Popups
- Selenium - Handling Forms
- Selenium - Windows and Tabs
- Selenium - Handling Links
- Selenium - Input Boxes
- Selenium - Radio Button
- Selenium - Checkboxes
- Selenium - Dropdown Box
- Selenium - Handling IFrames
- Selenium - Handling Cookies
- Selenium - Date Time Picker
- Selenium - Dynamic Web Tables
- Selenium - Actions Class
- Selenium - Action Class
- Selenium - Keyboard Events
- Selenium - Key Up/Down
- Selenium - Copy and Paste
- Selenium - Handle Special Keys
- Selenium - Mouse Events
- Selenium - Drag and Drop
- Selenium - Scroll Operations
- Selenium - Waiting Strategies
- Selenium - Explicit/Implicit Wait
- Selenium - Support Features
- Selenium - Multi Select
- Selenium - Wait Support
- Selenium - Select Support
- Selenium - Color Support
- Selenium - Errors & Logging
- Selenium - Logging
- Selenium - Exception Handling
- Selenium - Miscellaneous
- Selenium - Handling Ajax Calls
- Selenium - JSON Data File
- Selenium - CSV Data File
- Selenium - Excel Data File
- Selenium - Cross Browser Testing
- Selenium - Multi Browser Testing
- Selenium - Multi Windows Testing
- Selenium - JavaScript Executor
- Selenium - Headless Execution
- Selenium - Capture Screenshots
- Selenium - Capture Videos
- Selenium - Page Object Model
- Selenium - Page Factory
- Selenium - Record & Playback
- Selenium - Frameworks
- Selenium - Browsing Context
- Selenium Grid Tutorial
- Selenium - Overview
- Selenium - Architecture
- Selenium - Components
- Selenium - Configuration
- Selenium - Create Test Script
- Selenium - Test Execution
- Selenium - Endpoints
- Selenium - Customizing a Node
- Selenium Reporting Tools
- Selenium - Reporting Tools
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & other Technologies
- Selenium - Java Tutorial
- Selenium - Python Tutorial
- Selenium - C# Tutorial
- Selenium - Javascript Tutorial
- Selenium - Kotlin Tutorial
- Selenium - Ruby  Tutorial
- Selenium - Maven & Jenkins
- Selenium - Database Testing
- Selenium - LogExpert Logging
- Selenium - Log4j Logging
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash Testing
- Selenium - Apache Ant
- Selenium - Github
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium Miscellaneous Concepts
- Selenium - Automation Frameworks
- Selenium - Keyword Driven Framework
- Selenium - Data Driven Framework
- Selenium - Hybrid Driven Framework
- Selenium - SSL Certificate Error
- Selenium - Alternatives
- Selenium Useful Resources
- Selenium - Quick Guide
- Selenium - Useful Resources
- Selenium - Automation Practice
- Selenium - Discussion
Selenium Webdriver - Checkboxes
Selenium Webdriver can be used to handle checkboxes on a web page. In HTML terminology, every checkbox is identified by the tagname called input. Also, each checkbox on a web page will always have an attribute called type with its value as checkbox.
Let us now discuss the identification of a checkbox on a web page shown in the below image. First, we would need to right click on the webpage, and then click on the Inspect button in the Chrome broswer. Then, the corresponding HTML code for the whole page would be visible. For investigating a single element on a page, we would need to click on the left upward arrow, available to the top of the visible HTML code as highlighted below.
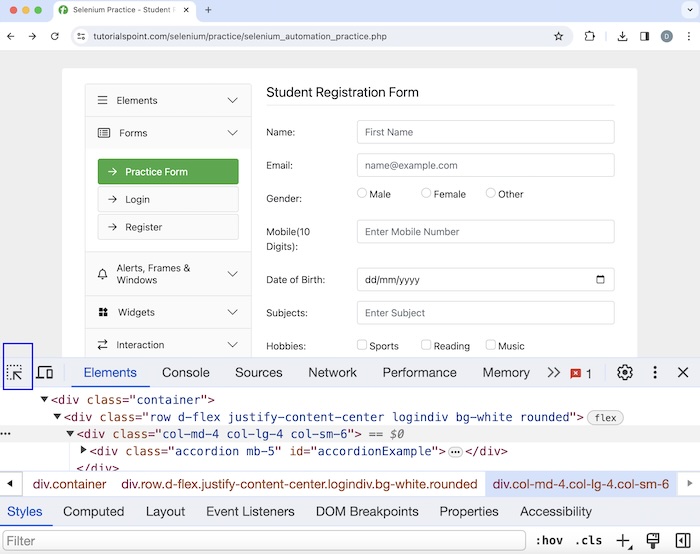
Once we had clicked and pointed the arrow to the checkbox (highlighted in the below image), its HTML code was visible, both reflecting the input tagname(enclosed in <>) and value of type attribute as checkbox.
<input id="hobbies" name="dob" type="checkbox" class="form-check-input mt-0">
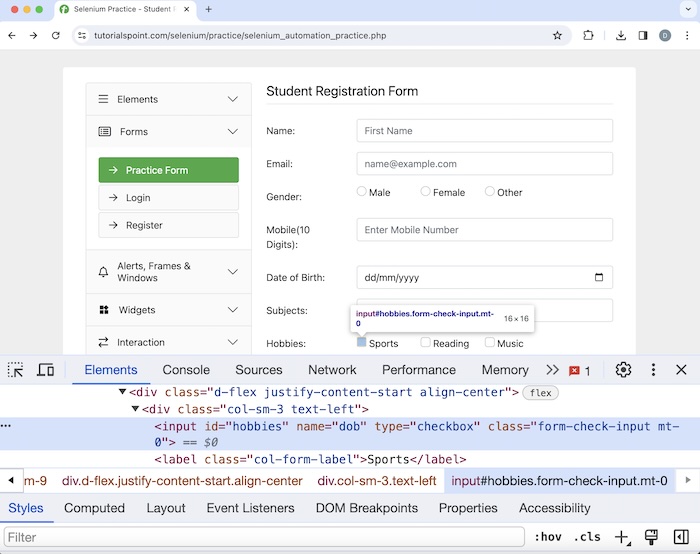
Let us take an example of the above page, where we would click the first checkbox with the help of the click() method. Then we would verify if the checkbox is checked using the isSelected() method. This method returns a boolean value(true or false). If a checkbox is selected, isSelected() would return true, else false.
Syntax
Webdriver driver = new ChromeDriver(); WebElement checkbox= driver.findElement(By.xpath("value of xpath")); checkbox.click(); boolean result = checkBox.isSelected();
Example
Code Implementation on HandlingCheckbox.java class file.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class HandlingCheckbox { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify checkbox driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // identify the first checkbox WebElement checkBox = driver.findElement(By.xpath("//*[@id='hobbies']")); // click the checkbox checkBox.click(); // check if a checkbox is selected boolean result = checkBox.isSelected(); System.out.println("Checking if a checkbox is selected: " + result); // Closing browser driver.quit(); } }
Output
Checking if a checkbox is selected: true Process finished with exit code 0
In the above example, we had first clicked on the first checkbox, and then verified if the checkbox was checked in the console with the message - Checking if a checkbox is selected: true.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Let us take another example of the below page, where we would count the total number of checkboxes. In this example, the total number of checkboxes should be 3.
Syntax
Webdriver driver = new ChromeDriver(); List<WebElement> totalChks = driver.findElements (By.xpath("<xpath value of all checkboxes>")); int count = totalChks.size();
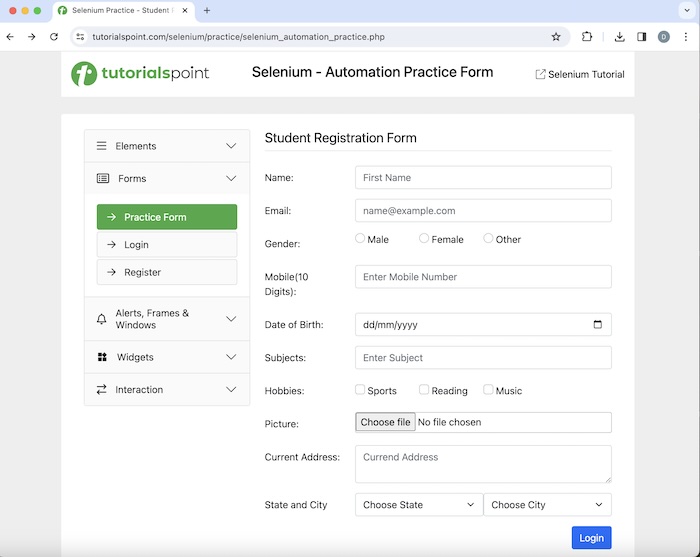
Example
Code Implementation on CountingCheckbox.java class file.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; import java.util.List; public class CountingCheckbox { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify checkbox driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Retrieve all checkboxes using locator and storing in List List<WebElement> totalChks = driver.findElements(By.xpath("//input[@type='checkbox']")); // count number of checkboxes int count = totalChks.size(); System.out.println("Count the checkboxes: " + count); //Closing browser driver.quit(); } }
Output
Count the checkboxes: 3 Process finished with exit code 0
In the above example, we had counted the total number of checkboxes on a webpage, and received the messages in the console - Count the checkboxes: 3.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Let us take another example on checkboxes, where we would perform some validation on the checkboxes. First, we would check if the checkbox is enabled/disabled using the isEnabled() method. Also, we would verify if it is displayed and checked/unchecked using the isDisplayed() and isSelected() methods respectively.
These methods return a boolean value(true or false). If a checkbox is selected, enabled and displayed it would return true, else false.
Syntax
Webdriver driver = new ChromeDriver(); // identify checkbox but not selected WebElement checkBox = driver.findElement(By.xpath("<xpath value of checkbox>")); // verify if checkbox is selected boolean result = checkBox.isSelected(); System.out.println("Checking if a checkbox is selected: " + result); // verify if checkbox is displayed boolean result1 = checkBox.isDisplayed(); System.out.println("Checking if a checkbox is displayed: " + result1); // verify if checkbox is enabled boolean result2 = checkBox.isEnabled(); System.out.println("Checking if a checkbox is enabled: " + result2);
Example
Code Implementation on CheckboxValidates.java class file.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class CheckboxValidates { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify checkbox driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // identify checkbox but not select WebElement checkBox = driver.findElement (By.xpath("//*[@id='practiceForm']/div[7]/div/div/div[2]/input")); // verify if checkbox is selected boolean result = checkBox.isSelected(); System.out.println("Checking if a checkbox is selected: " + result); // verify if checkbox is displayed boolean result1 = checkBox.isDisplayed(); System.out.println("Checking if a checkbox is displayed: " + result1); // verify if checkbox is enabled boolean result2 = checkBox.isEnabled(); System.out.println("Checking if a checkbox is enabled: " + result2); // Closing browser driver.quit(); } }
Output
Checking if a checkbox is selected: false Checking if a checkbox is displayed: true Checking if a checkbox is enabled: true Process finished with exit code 0
In the above example, we had validated if a checkbox was displayed, enabled, and selected, and received the following messages in the console - Checking if a checkbox is selected: false, Checking if a checkbox is displayed: true and Checking if a checkbox is enabled: true.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Thus, in this tutorial, we had discussed how to handle checkboxes using the Selenium Webdriver.