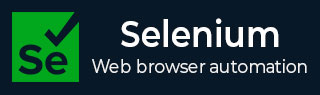
- Selenium Tutorial
- Selenium - Home
- Selenium - Overview
- Selenium - Components
- Selenium - Automation Testing
- Selenium - Environment Setup
- Selenium - Remote Control
- Selenium IDE Tutorial
- Selenium - IDE Introduction
- Selenium - Features
- Selenium - Limitations
- Selenium - Installation
- Selenium - Creating Tests
- Selenium - Creating Script
- Selenium - Store Variables
- Selenium - Alerts & Popups
- Selenium - Selenese Commands
- Selenium - Actions Commands
- Selenium - Accessors Commands
- Selenium - Assertions Commands
- Selenium - Assert/Verify Methods
- Selenium - Locating Strategies
- Selenium - Script Debugging
- Selenium - Verification Points
- Selenium - Pattern Matching
- Selenium - JSON Data File
- Selenium - Browser Execution
- Selenium - User Extensions
- Selenium - Code Export
- Selenium - JavaScript Functions
- Selenium - Plugins
- Selenium WebDriver Tutorial
- Selenium - Introduction
- Selenium WebDriver vs RC
- Selenium - Installation
- Selenium - First Test Script
- Selenium - Driver Sessions
- Selenium - Browser Options
- Selenium - Chrome Options
- Selenium - Edge Options
- Selenium - Firefox Options
- Selenium - Safari Options
- Selenium Web Elements
- Selenium - File Upload
- Selenium - Locator Strategies
- Selenium - Relative Locators
- Selenium - Finders
- Selenium - Find All Links
- Selenium - User Interactions
- Selenium - WebElement Commands
- Selenium - Browser Interactions
- Selenium - Browser Commands
- Selenium - Browser Navigation
- Selenium - Alerts & Popups
- Selenium - Handling Forms
- Selenium - Windows and Tabs
- Selenium - Handling Links
- Selenium - Input Boxes
- Selenium - Radio Button
- Selenium - Checkboxes
- Selenium - Dropdown Box
- Selenium - Handling IFrames
- Selenium - Handling Cookies
- Selenium - Date Time Picker
- Selenium - Dynamic Web Tables
- Selenium - Actions Class
- Selenium - Action Class
- Selenium - Keyboard Events
- Selenium - Key Up/Down
- Selenium - Copy and Paste
- Selenium - Handle Special Keys
- Selenium - Mouse Events
- Selenium - Drag and Drop
- Selenium - Scroll Operations
- Selenium - Waiting Strategies
- Selenium - Explicit/Implicit Wait
- Selenium - Support Features
- Selenium - Multi Select
- Selenium - Wait Support
- Selenium - Select Support
- Selenium - Color Support
- Selenium - Errors & Logging
- Selenium - Logging
- Selenium - Exception Handling
- Selenium - Miscellaneous
- Selenium - Handling Ajax Calls
- Selenium - JSON Data File
- Selenium - CSV Data File
- Selenium - Excel Data File
- Selenium - Cross Browser Testing
- Selenium - Multi Browser Testing
- Selenium - Multi Windows Testing
- Selenium - JavaScript Executor
- Selenium - Headless Execution
- Selenium - Capture Screenshots
- Selenium - Capture Videos
- Selenium - Page Object Model
- Selenium - Page Factory
- Selenium - Record & Playback
- Selenium - Frameworks
- Selenium - Browsing Context
- Selenium Grid Tutorial
- Selenium - Overview
- Selenium - Architecture
- Selenium - Components
- Selenium - Configuration
- Selenium - Create Test Script
- Selenium - Test Execution
- Selenium - Endpoints
- Selenium - Customizing a Node
- Selenium Reporting Tools
- Selenium - Reporting Tools
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & other Technologies
- Selenium - Java Tutorial
- Selenium - Python Tutorial
- Selenium - C# Tutorial
- Selenium - Javascript Tutorial
- Selenium - Kotlin Tutorial
- Selenium - Ruby  Tutorial
- Selenium - Maven & Jenkins
- Selenium - Database Testing
- Selenium - LogExpert Logging
- Selenium - Log4j Logging
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash Testing
- Selenium - Apache Ant
- Selenium - Github
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium Miscellaneous Concepts
- Selenium - Automation Frameworks
- Selenium - Keyword Driven Framework
- Selenium - Data Driven Framework
- Selenium - Hybrid Driven Framework
- Selenium - SSL Certificate Error
- Selenium - Alternatives
- Selenium Useful Resources
- Selenium - Quick Guide
- Selenium - Useful Resources
- Selenium - Automation Practice
- Selenium - Discussion
Selenium WebDriver - Logging
Selenium Webdriver can be used for logging information during test execution. The logging is mainly used to extract information about how the execution took place.
The first step towards logging comes with enabling logging with default settings with respect to each class. Let us first enable the default logging, which is applicable to all loggers using the root logger.
Logger logger = Logger.getLogger("");
Since logging is set with respect to a class, we can set the logging level applicable to a class.
((RemoteWebDriver) driver).setLogLevel(Level.INFO); Logger.getLogger(SeleniumManager.class.getName()) .setLevel(Level.SEVERE);
There are a total seven log levels - SEVERE, WARNING, INFO, CONFIG, FINE, FINER, and FINEST. The INFO log level is the default one which means there are no action items to be taken care of in our code and purely used for informational purposes.
Logger logger = Logger.getLogger(""); logger.setLevel(Level.INFO);
Always, all logging levels are not required, so we can filter out the logs based on the levels using the setLevel() method.
Logger logger = Logger.getLogger(""); logger.setLevel(Level.WARNING);
In the above example, log level of WARNING has been set, meaning that some actions need to be taken care, specifically for using deprecated versions in our code.
Example
Code Implementation with WARNING log levels.
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.remote.RemoteWebDriver; import java.util.concurrent.TimeUnit; import java.util.logging.Level; public class LoggingLvl { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // enabling log levels to Warning ((RemoteWebDriver) driver).setLogLevel(Level.WARNING); //adding implicit wait of 12 secs driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS); // Opening the webpage driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // getting current URL System.out.println("Getting the Current URL: " + driver.getCurrentUrl()); // quitting the browser driver.quit(); } }
Output
Feb 15, 2024 4:41:42 PM org.openqa.selenium.remote.RemoteWebDriver log WARNING: Executing: setTimeout [510e0fcbc35b47f7637445d1b69bedc2, setTimeout {implicit=12000}] Feb 15, 2024 4:41:42 PM org.openqa.selenium.remote.RemoteWebDriver log WARNING: Executed: setTimeout (Response: SessionID: 510e0fcbc35b47f7637445d1b69bedc2, Status: 0, Value: null) Feb 15, 2024 4:41:42 PM org.openqa.selenium.remote.RemoteWebDriver log WARNING: Executing: get [510e0fcbc35b47f7637445d1b69bedc2, get {url=https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php}] Feb 15, 2024 4:41:43 PM org.openqa.selenium.remote.RemoteWebDriver log WARNING: Executed: get (Response: SessionID: 510e0fcbc35b47f7637445d1b69bedc2, Status: 0, Value: null) Feb 15, 2024 4:41:43 PM org.openqa.selenium.remote.RemoteWebDriver log WARNING: Executing: getCurrentUrl [510e0fcbc35b47f7637445d1b69bedc2, getCurrentUrl {}] Feb 15, 2024 4:41:43 PM org.openqa.selenium.remote.RemoteWebDriver log WARNING: Executed: getCurrentUrl (Response: SessionID: 510e0fcbc35b47f7637445d1b69bedc2, Status: 0, Value: https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php) Feb 15, 2024 4:41:43 PM org.openqa.selenium.remote.RemoteWebDriver log WARNING: Executing: quit [510e0fcbc35b47f7637445d1b69bedc2, quit {}] Getting the Current URL: https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php Feb 15, 2024 4:41:43 PM org.openqa.selenium.remote.RemoteWebDriver log WARNING: Executed: quit (Response: SessionID: 510e0fcbc35b47f7637445d1b69bedc2, Status: 0, Value: null) Process finished with exit code 0
In the above example, we have obtained the WARNING log levels along with the browser title with the message as Getting the Current URL: https://www.tutorialspoint.com/selenium/.
The logging information also contains debug information for looking into a specific issue and correcting it. This can be done by setting the log level to FINE.
Logger logger = Logger.getLogger(""); logger.setLevel(Level.FINE);
Let us take another example, where we would set the log level to SEVERE.
Example
Code Implementation with SEVERE log levels.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.remote.RemoteWebDriver; import java.util.concurrent.TimeUnit; import java.util.logging.Level; public class LoggingLvls { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // enabling log levels to SEVERE ((RemoteWebDriver) driver).setLogLevel(Level.SEVERE); //adding implicit wait of 12 secs driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS); // Opening the webpage driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Identify the input box with xpath locator WebElement e = driver.findElement(By.xpath("//*[@id='name']")); // enter text in input box e.sendKeys("Selenium"); // getting current URL System.out.println("Getting the Current URL: " + driver.getCurrentUrl()); //quitting the browser driver.quit(); } }
Output
Feb 15, 2024 4:48:27 PM org.openqa.selenium.remote.RemoteWebDriver log SEVERE: Executing: setTimeout [ca1196b6a589eeac011ff53fbc3312aa, setTimeout {implicit=12000}] Feb 15, 2024 4:48:27 PM org.openqa.selenium.remote.RemoteWebDriver log SEVERE: Executed: setTimeout (Response: SessionID: ca1196b6a589eeac011ff53fbc3312aa, Status: 0, Value: null) Feb 15, 2024 4:48:27 PM org.openqa.selenium.remote.RemoteWebDriver log SEVERE: Executing: get [ca1196b6a589eeac011ff53fbc3312aa, get {url=https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php}] Feb 15, 2024 4:48:28 PM org.openqa.selenium.remote.RemoteWebDriver log SEVERE: Executed: get (Response: SessionID: ca1196b6a589eeac011ff53fbc3312aa, Status: 0, Value: null) Feb 15, 2024 4:48:28 PM org.openqa.selenium.remote.RemoteWebDriver log SEVERE: Executing: findElement [ca1196b6a589eeac011ff53fbc3312aa, findElement {using=xpath, value=//*[@id='name']}] Feb 15, 2024 4:48:28 PM org.openqa.selenium.remote.RemoteWebDriver log SEVERE: Executed: findElement (Response: SessionID: ca1196b6a589eeac011ff53fbc3312aa, Status: 0, Value: {element-6066-11e4-a52e-4f735466cecf=B6679A9C1F8F9717E6BD42C9A8EC529E_element_15}) Feb 15, 2024 4:48:28 PM org.openqa.selenium.remote.RemoteWebDriver log SEVERE: Executing: sendKeysToElement [ca1196b6a589eeac011ff53fbc3312aa, sendKeysToElement {id=B6679A9C1F8F9717E6BD42C9A8EC529E_element_15, value=[Ljava.lang.CharSequence;@4c51bb7}] Feb 15, 2024 4:48:28 PM org.openqa.selenium.remote.RemoteWebDriver log SEVERE: Executed: sendKeysToElement (Response: SessionID: ca1196b6a589eeac011ff53fbc3312aa, Status: 0, Value: null) Feb 15, 2024 4:48:28 PM org.openqa.selenium.remote.RemoteWebDriver log SEVERE: Executing: getCurrentUrl [ca1196b6a589eeac011ff53fbc3312aa, getCurrentUrl {}] Feb 15, 2024 4:48:28 PM org.openqa.selenium.remote.RemoteWebDriver log SEVERE: Executed: getCurrentUrl (Response: SessionID: ca1196b6a589eeac011ff53fbc3312aa, Status: 0, Value: https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php) Feb 15, 2024 4:48:28 PM org.openqa.selenium.remote.RemoteWebDriver log SEVERE: Executing: quit [ca1196b6a589eeac011ff53fbc3312aa, quit {}] Getting the Current URL: https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php Feb 15, 2024 4:48:28 PM org.openqa.selenium.remote.RemoteWebDriver log SEVERE: Executed: quit (Response: SessionID: ca1196b6a589eeac011ff53fbc3312aa, Status: 0, Value: null) Process finished with exit code 0
In the above example, we have obtained the SEVERE log levels along with the browser title with the message as Getting the Current URL: https://www.tutorialspoint.com/selenium/.
The logs generated in the console can be written to another file by using a handler. All the logs are placed in System.err by default.
Syntax
// enabling log levels to WARNING Logger logger = Logger.getLogger(""); logger.setLevel(Level.WARNING); // output logging to another file Logs.xml Handler handler = new FileHandler("Logs1.xml"); logger.addHandler(handler);
Example
Code Implementation on LoggingLvelFile.java class file.
package org.example; import org.openqa.selenium.WebDriver; import java.io.IOException; import java.util.logging.*; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; import java.util.logging.Handler; public class LoggingLvelFile { public static void main(String[] args) throws InterruptedException, IOException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // enabling log levels to WARNING Logger logger = Logger.getLogger(""); logger.setLevel(Level.WARNING); // output logging to another file Logs1.xml Handler handler = new FileHandler("Logs1.xml"); logger.addHandler(handler); //adding implicit wait of 12 secs driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS); // Opening the webpage driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // getting current URL System.out.println("Getting the Current URL: " + driver.getCurrentUrl()); //quitting the browser driver.quit(); } }
Output
Getting the Current URL: https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php Process finished with exit code 0
In the above example, we retrieved the browser title with the message in the console - Getting the Current URL:
https://www.tutorialspoint.com/selenium/.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.