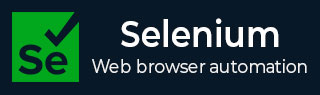
- Selenium Tutorial
- Selenium - Home
- Selenium - Overview
- Selenium - Components
- Selenium - Automation Testing
- Selenium - Environment Setup
- Selenium - Remote Control
- Selenium IDE Tutorial
- Selenium - IDE Introduction
- Selenium - Features
- Selenium - Limitations
- Selenium - Installation
- Selenium - Creating Tests
- Selenium - Creating Script
- Selenium - Store Variables
- Selenium - Alerts & Popups
- Selenium - Selenese Commands
- Selenium - Actions Commands
- Selenium - Accessors Commands
- Selenium - Assertions Commands
- Selenium - Assert/Verify Methods
- Selenium - Locating Strategies
- Selenium - Script Debugging
- Selenium - Verification Points
- Selenium - Pattern Matching
- Selenium - JSON Data File
- Selenium - Browser Execution
- Selenium - User Extensions
- Selenium - Code Export
- Selenium - JavaScript Functions
- Selenium - Plugins
- Selenium WebDriver Tutorial
- Selenium - Introduction
- Selenium WebDriver vs RC
- Selenium - Installation
- Selenium - First Test Script
- Selenium - Driver Sessions
- Selenium - Browser Options
- Selenium - Chrome Options
- Selenium - Edge Options
- Selenium - Firefox Options
- Selenium - Safari Options
- Selenium Web Elements
- Selenium - File Upload
- Selenium - Locator Strategies
- Selenium - Relative Locators
- Selenium - Finders
- Selenium - Find All Links
- Selenium - User Interactions
- Selenium - WebElement Commands
- Selenium - Browser Interactions
- Selenium - Browser Commands
- Selenium - Browser Navigation
- Selenium - Alerts & Popups
- Selenium - Handling Forms
- Selenium - Windows and Tabs
- Selenium - Handling Links
- Selenium - Input Boxes
- Selenium - Radio Button
- Selenium - Checkboxes
- Selenium - Dropdown Box
- Selenium - Handling IFrames
- Selenium - Handling Cookies
- Selenium - Date Time Picker
- Selenium - Dynamic Web Tables
- Selenium - Actions Class
- Selenium - Action Class
- Selenium - Keyboard Events
- Selenium - Key Up/Down
- Selenium - Copy and Paste
- Selenium - Handle Special Keys
- Selenium - Mouse Events
- Selenium - Drag and Drop
- Selenium - Scroll Operations
- Selenium - Waiting Strategies
- Selenium - Explicit/Implicit Wait
- Selenium - Support Features
- Selenium - Multi Select
- Selenium - Wait Support
- Selenium - Select Support
- Selenium - Color Support
- Selenium - Errors & Logging
- Selenium - Logging
- Selenium - Exception Handling
- Selenium - Miscellaneous
- Selenium - Handling Ajax Calls
- Selenium - JSON Data File
- Selenium - CSV Data File
- Selenium - Excel Data File
- Selenium - Cross Browser Testing
- Selenium - Multi Browser Testing
- Selenium - Multi Windows Testing
- Selenium - JavaScript Executor
- Selenium - Headless Execution
- Selenium - Capture Screenshots
- Selenium - Capture Videos
- Selenium - Page Object Model
- Selenium - Page Factory
- Selenium - Record & Playback
- Selenium - Frameworks
- Selenium - Browsing Context
- Selenium Grid Tutorial
- Selenium - Overview
- Selenium - Architecture
- Selenium - Components
- Selenium - Configuration
- Selenium - Create Test Script
- Selenium - Test Execution
- Selenium - Endpoints
- Selenium - Customizing a Node
- Selenium Reporting Tools
- Selenium - Reporting Tools
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & other Technologies
- Selenium - Java Tutorial
- Selenium - Python Tutorial
- Selenium - C# Tutorial
- Selenium - Javascript Tutorial
- Selenium - Kotlin Tutorial
- Selenium - Ruby Tutorial
- Selenium - Maven & Jenkins
- Selenium - Database Testing
- Selenium - LogExpert Logging
- Selenium - Log4j Logging
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash Testing
- Selenium - Apache Ant
- Selenium - Github
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium Miscellaneous Concepts
- Selenium - Automation Frameworks
- Selenium - Keyword Driven Framework
- Selenium - Data Driven Framework
- Selenium - Hybrid Driven Framework
- Selenium - SSL Certificate Error
- Selenium - Alternatives
- Selenium Useful Resources
- Selenium - Quick Guide
- Selenium - Useful Resources
- Selenium - Automation Practice
- Selenium - Discussion
Selenium WebDriver - Firefox Options
FirefoxOptions is a specific class in Selenium Webdriver which helps to handle options which are only applicable to the Firefox driver. It helps to modify the settings and capabilities of the browser while running an automated test on Firefox. The FirefoxOptions class extends another class known as the MutableCapabilities class.
The FirefoxOptions class is available in the latest version of Selenium. Selenium Webdriver begins with a fresh browser profile without any predefined settings on cookies, history, and so on by default.
Example 1 - Open in Headless Browser Using FirefoxOptions
In this example, we would open and launch an application in the headless browser using the FirefoxOptions class.
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.firefox.FirefoxDriver; import org.openqa.selenium.firefox.FirefoxOptions; import java.io.File; import java.util.List; import java.util.concurrent.TimeUnit; public class FirefoxOptnsHeadless { public static void main(String[] args) throws InterruptedException { // object of FirefoxOptions FirefoxOptions opt = new FirefoxOptions(); // open in headless mode opt.addArguments("-headless"); // Initiate the Webdriver WebDriver driver = new FirefoxDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage with headless mode driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // getting page title System.out.println("Getting the page title: " + driver.getTitle()); // Quitting browser driver.quit(); } }
Output
Getting the page title: Selenium Practice - Student Registration Form Process finished with exit code 0
In the above example, we observed that the Firefox browser was launched in a headless mode. We had also obtained the browser title with the message in the console - Getting the page title: Selenium Practice - Student Registration Form.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Example 2 - SSL Certificates Using FirefoxOptions
To handle SSL certificates in Firefox, we have to use the FirefoxOptions class along with the DesiredCapabilities class. SSL error shown in Firefox is Warning: Potential Security Risk Ahead.
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.firefox.FirefoxDriver; import org.openqa.selenium.firefox.FirefoxOptions; import org.openqa.selenium.remote.CapabilityType; import org.openqa.selenium.remote.DesiredCapabilities; import java.util.concurrent.TimeUnit; public class SSLErrorFirefox { public static void main(String[] args) throws InterruptedException { // Desired Capabilities class to profile Firefox DesiredCapabilities dc = new DesiredCapabilities(); dc.setCapability(CapabilityType.ACCEPT_INSECURE_CERTS, true); // Firefox Options class FirefoxOptions opt = new FirefoxOptions(); opt.merge(dc); // Initiate the Webdriver with options WebDriver driver = new FirefoxDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launch application with SSL error driver.get("https://expired.badssl.com"); // get browser title System.out.println("Browser title in Firefox: " + driver.getTitle()); // quitting the browser driver.quit(); } }
Output
Browser title in Firefox: Privacy error Process finished with exit code 0
In the above example, we had the SSL certificate error in Firefox and launched the application and then obtained the browser title with the message in the console - Browser title in Firefox: Privacy error.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Example 3 - Firefox Profile Using FirefoxOptions
Let us take an example, where we would launch the Firefox browser with our own Firefox profile using the FirefoxOptions class.
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.firefox.FirefoxDriver; import org.openqa.selenium.firefox.FirefoxOptions; import org.openqa.selenium.firefox.FirefoxProfile; import org.openqa.selenium.firefox.ProfilesIni; import java.util.concurrent.TimeUnit; public class FirefoxOptnsProfile { public static void main(String[] args) throws InterruptedException { // object of FirefoxOptions FirefoxOptions opt = new FirefoxOptions(); // object of ProfilesIni ProfilesIni prof = new ProfilesIni(); // object of ProfilesIni FirefoxProfile p = prof.getProfile("<profileName>"); opt.setProfile(p); // Initiate the Webdriver WebDriver driver = new FirefoxDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // getting page title System.out.println("Getting the page title: " + driver.getTitle()); // Quitting browser driver.quit(); } }
Output
Getting the page title: Selenium Practice - Student Registration Form Process finished with exit code 0
In the above example, we observed that the Firefox browser was launched with a created profile. We had also obtained the browser title with the message in the console - Getting the page title: Selenium Practice - Student Registration Form.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Example 4 - Open Maximized Browser Using FirefoxOptions
In this example, we would open and launch an application in the browser maximized size using the FirefoxOptions class.
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.firefox.FirefoxDriver; import org.openqa.selenium.firefox.FirefoxOptions import java.io.File; import java.util.Collections; import java.util.concurrent.TimeUnit; public class FirefoxOptnsMaximized { public static void main(String[] args) throws InterruptedException { // object of FirefoxOptions FirefoxOptions opt = new FirefoxOptions(); // open browser in maximized mode opt.addArguments("--kiosk") // Initiate the Webdriver WebDriver driver = new FirefoxDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage driver.get("https://www.tutorialspoint.com/selenium/practice/register.php"); // quitting browser driver.quit(); } }
Output
Process finished with exit code 0
In the above example, we observed that the Firefox browser was launched in a maximized browser.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
This concludes our comprehensive take on the tutorial on Selenium Webdriver - Firefox Options. We’ve started with describing a FirefoxOptions class, and walked through examples of how to add profiles to Firefox browser, how to launch the Firefox browser maximized, how to open the Firefox browser in headless mode, and handle SSL certificates errors taking help of FirefoxOptions along with Selenium Webdriver. This equips you with in-depth knowledge of the FirefoxOptions class in Selenium Webdriver. It is wise to keep practicing what you’ve learned and exploring others relevant to Selenium to deepen your understanding and expand your horizons.