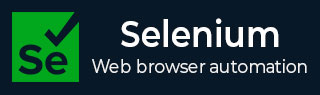
- Selenium Tutorial
- Selenium - Home
- Selenium - Overview
- Selenium - Components
- Selenium - Automation Testing
- Selenium - Environment Setup
- Selenium - Remote Control
- Selenium IDE Tutorial
- Selenium - IDE Introduction
- Selenium - Features
- Selenium - Limitations
- Selenium - Installation
- Selenium - Creating Tests
- Selenium - Creating Script
- Selenium - Store Variables
- Selenium - Alerts & Popups
- Selenium - Selenese Commands
- Selenium - Actions Commands
- Selenium - Accessors Commands
- Selenium - Assertions Commands
- Selenium - Assert/Verify Methods
- Selenium - Locating Strategies
- Selenium - Script Debugging
- Selenium - Verification Points
- Selenium - Pattern Matching
- Selenium - JSON Data File
- Selenium - Browser Execution
- Selenium - User Extensions
- Selenium - Code Export
- Selenium - JavaScript Functions
- Selenium - Plugins
- Selenium WebDriver Tutorial
- Selenium - Introduction
- Selenium WebDriver vs RC
- Selenium - Installation
- Selenium - First Test Script
- Selenium - Driver Sessions
- Selenium - Browser Options
- Selenium - Chrome Options
- Selenium - Edge Options
- Selenium - Firefox Options
- Selenium - Safari Options
- Selenium Web Elements
- Selenium - File Upload
- Selenium - Locator Strategies
- Selenium - Relative Locators
- Selenium - Finders
- Selenium - Find All Links
- Selenium - User Interactions
- Selenium - WebElement Commands
- Selenium - Browser Interactions
- Selenium - Browser Commands
- Selenium - Browser Navigation
- Selenium - Alerts & Popups
- Selenium - Handling Forms
- Selenium - Windows and Tabs
- Selenium - Handling Links
- Selenium - Input Boxes
- Selenium - Radio Button
- Selenium - Checkboxes
- Selenium - Dropdown Box
- Selenium - Handling IFrames
- Selenium - Handling Cookies
- Selenium - Date Time Picker
- Selenium - Dynamic Web Tables
- Selenium - Actions Class
- Selenium - Action Class
- Selenium - Keyboard Events
- Selenium - Key Up/Down
- Selenium - Copy and Paste
- Selenium - Handle Special Keys
- Selenium - Mouse Events
- Selenium - Drag and Drop
- Selenium - Scroll Operations
- Selenium - Waiting Strategies
- Selenium - Explicit/Implicit Wait
- Selenium - Support Features
- Selenium - Multi Select
- Selenium - Wait Support
- Selenium - Select Support
- Selenium - Color Support
- Selenium - Errors & Logging
- Selenium - Logging
- Selenium - Exception Handling
- Selenium - Miscellaneous
- Selenium - Handling Ajax Calls
- Selenium - JSON Data File
- Selenium - CSV Data File
- Selenium - Excel Data File
- Selenium - Cross Browser Testing
- Selenium - Multi Browser Testing
- Selenium - Multi Windows Testing
- Selenium - JavaScript Executor
- Selenium - Headless Execution
- Selenium - Capture Screenshots
- Selenium - Capture Videos
- Selenium - Page Object Model
- Selenium - Page Factory
- Selenium - Record & Playback
- Selenium - Frameworks
- Selenium - Browsing Context
- Selenium Grid Tutorial
- Selenium - Overview
- Selenium - Architecture
- Selenium - Components
- Selenium - Configuration
- Selenium - Create Test Script
- Selenium - Test Execution
- Selenium - Endpoints
- Selenium - Customizing a Node
- Selenium Reporting Tools
- Selenium - Reporting Tools
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & other Technologies
- Selenium - Java Tutorial
- Selenium - Python Tutorial
- Selenium - C# Tutorial
- Selenium - Javascript Tutorial
- Selenium - Kotlin Tutorial
- Selenium - Ruby Tutorial
- Selenium - Maven & Jenkins
- Selenium - Database Testing
- Selenium - LogExpert Logging
- Selenium - Log4j Logging
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash Testing
- Selenium - Apache Ant
- Selenium - Github
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium Miscellaneous Concepts
- Selenium - Automation Frameworks
- Selenium - Keyword Driven Framework
- Selenium - Data Driven Framework
- Selenium - Hybrid Driven Framework
- Selenium - SSL Certificate Error
- Selenium - Alternatives
- Selenium Useful Resources
- Selenium - Quick Guide
- Selenium - Useful Resources
- Selenium - Automation Practice
- Selenium - Discussion
Selenium WebDriver - Chrome Options
ChromeOptions is a specific class in Selenium Webdriver which helps to handle options which are only applicable to the Chrome driver. It helps to modify the settings and capabilities of the browser while running an automated test on Chrome. The ChromeOptions class extends another class known as the MutableCapabilities class.
The ChromeOptions class was added from the Selenium 3.6 version onwards. Selenium Webdriver begins with a fresh browser profile without any predefined settings on cookies, history, and so on by default.
Example 1 - Add Chrome Extensions Using ChromeOptions
Let us take an example, where we would launch the Chrome browser with a Selenium IDE extension using the ChromeOptions class. Every Chrome extension should have a .crx file.
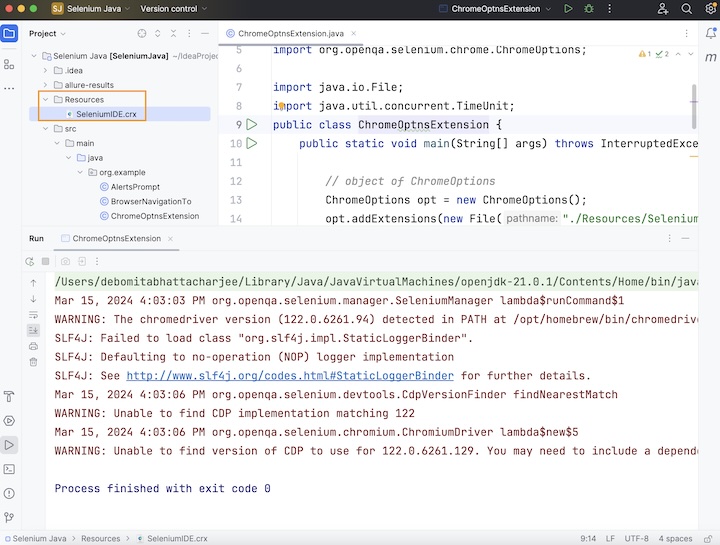
We would get the .crx file for Selenium IDE Chrome extension and place it under the Resources folder within our test project.
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.io.File; import java.util.concurrent.TimeUnit; public class ChromeOptnsExtension { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); // adding .crx extension opt.addExtensions(new File("./Resources/SeleniumIDE.crx")); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage with Selenium IDE extension driver.get("https://www.tutorialspoint.com/selenium/practice/register.php"); } }
Output
Process finished with exit code 0
In the above example, we observed that the Chrome browser got launched with the Selenium IDE extension along with the information bar Chrome is being controlled by automated test software.

Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Example 2 - Disable Infobar Using ChromeOptions
In the previous example, we obtained an information bar with the text Chrome is being controlled by automated test software, however we can disable this information bar using the ChromeOptions class.
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.io.File; import java.util.Collections; import java.util.concurrent.TimeUnit; public class ChromeOptns { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); // adding .crx extensions opt.addExtensions(new File("./Resources/SeleniumIDE.crx")); // disable information bar opt.setExperimentalOption("excludeSwitches", Collections.singletonList("enable-automation")); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage with disabling information bar driver.get("https://www.tutorialspoint.com/selenium/practice/register.php"); } }
Output
Process finished with exit code 0
In the above example, we observed that the Chrome browser was launched with the Selenium IDE extension without the information bar Chrome is being controlled by automated test software.

Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Example 3 - Open Maximized Browser Using ChromeOptions
In this example, we would open and launch an application in the browser maximized size using the ChromeOptions class.
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.io.File; import java.util.Collections; import java.util.concurrent.TimeUnit; public class ChromeOptnsMaximized { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); // adding .crx extensions opt.addExtensions(new File("./Resources/SeleniumIDE.crx")); // disable information bar opt.setExperimentalOption("excludeSwitches", Collections.singletonList("enable-automation")); // open browser in maximized opt.addArguments("--start-maximized"); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage with Selenium IDE extension driver.get("https://www.tutorialspoint.com/selenium/practice/register.php"); // quitting browser driver.quit(); } }
Output
Process finished with exit code 0
In the above example, we observed that the Chrome browser was launched with the Selenium IDE extension without the information bar Chrome is being controlled by automated test software and in a maximized browser.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Example 4 - SSL Certificates Using ChromeOptions
To handle SSL certificates in Chrome, we have to use the ChromeOptions class along with the DesiredCapabilities class. In order to have the capabilities defined with the DesiredCapabilities to be available with the ChromeOptions we would need the help of the merge method from the ChromeOptions class. The SSL error shown in Chrome is Your connection is not private.
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import org.openqa.selenium.remote.CapabilityType; import org.openqa.selenium.remote.DesiredCapabilities; import java.util.concurrent.TimeUnit; public class SSLErrorChrome { public static void main(String[] args) throws InterruptedException { // Desired Capabilities class to profile Chrome DesiredCapabilities dc = new DesiredCapabilities(); dc.setCapability(CapabilityType.ACCEPT_INSECURE_CERTS, true); // Chrome Options class ChromeOptions opt = new ChromeOptions(); // merging browser capabilities with options opt.merge(dc); // Initiate the Webdriver with options WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launch application with SSL error driver.get("https://expired.badssl.com"); // get browser title System.out.println("Browser title in Chrome: " + driver.getTitle()); // quiting the browser driver.quit(); } }
Output
Browser title in Chrome: Privacy error Process finished with exit code 0
In the above example, we had the SSL certificate error in Chrome and launched the application and then obtained the browser title with the message in the console - Browser title in Chrome: Privacy error.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
This concludes our comprehensive take on the tutorial on Selenium Webdriver - Chrome Options. We’ve started with describing a ChromeOptions class, and walked through examples of how to add extensions to Chrome browser, how to disable information bar, how to maximize the browser, and handle SSL certificates errors taking help of ChromeOptions along with Selenium Webdriver. This equips you with in-depth knowledge of the ChromeOptions class in Selenium Webdriver. It is wise to keep practicing what you’ve learned and exploring others relevant to Selenium to deepen your understanding and expand your horizons.