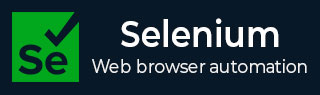
- Selenium Tutorial
- Selenium - Home
- Selenium - Overview
- Selenium - Components
- Selenium - Automation Testing
- Selenium - Environment Setup
- Selenium - Remote Control
- Selenium IDE Tutorial
- Selenium - IDE Introduction
- Selenium - Features
- Selenium - Limitations
- Selenium - Installation
- Selenium - Creating Tests
- Selenium - Creating Script
- Selenium - Store Variables
- Selenium - Alerts & Popups
- Selenium - Selenese Commands
- Selenium - Actions Commands
- Selenium - Accessors Commands
- Selenium - Assertions Commands
- Selenium - Assert/Verify Methods
- Selenium - Locating Strategies
- Selenium - Script Debugging
- Selenium - Verification Points
- Selenium - Pattern Matching
- Selenium - JSON Data File
- Selenium - Browser Execution
- Selenium - User Extensions
- Selenium - Code Export
- Selenium - JavaScript Functions
- Selenium - Plugins
- Selenium WebDriver Tutorial
- Selenium - Introduction
- Selenium WebDriver vs RC
- Selenium - Installation
- Selenium - First Test Script
- Selenium - Driver Sessions
- Selenium - Browser Options
- Selenium - Chrome Options
- Selenium - Edge Options
- Selenium - Firefox Options
- Selenium - Safari Options
- Selenium Web Elements
- Selenium - File Upload
- Selenium - Locator Strategies
- Selenium - Relative Locators
- Selenium - Finders
- Selenium - Find All Links
- Selenium - User Interactions
- Selenium - WebElement Commands
- Selenium - Browser Interactions
- Selenium - Browser Commands
- Selenium - Browser Navigation
- Selenium - Alerts & Popups
- Selenium - Handling Forms
- Selenium - Windows and Tabs
- Selenium - Handling Links
- Selenium - Input Boxes
- Selenium - Radio Button
- Selenium - Checkboxes
- Selenium - Dropdown Box
- Selenium - Handling IFrames
- Selenium - Handling Cookies
- Selenium - Date Time Picker
- Selenium - Dynamic Web Tables
- Selenium - Actions Class
- Selenium - Action Class
- Selenium - Keyboard Events
- Selenium - Key Up/Down
- Selenium - Copy and Paste
- Selenium - Handle Special Keys
- Selenium - Mouse Events
- Selenium - Drag and Drop
- Selenium - Scroll Operations
- Selenium - Waiting Strategies
- Selenium - Explicit/Implicit Wait
- Selenium - Support Features
- Selenium - Multi Select
- Selenium - Wait Support
- Selenium - Select Support
- Selenium - Color Support
- Selenium - Errors & Logging
- Selenium - Logging
- Selenium - Exception Handling
- Selenium - Miscellaneous
- Selenium - Handling Ajax Calls
- Selenium - JSON Data File
- Selenium - CSV Data File
- Selenium - Excel Data File
- Selenium - Cross Browser Testing
- Selenium - Multi Browser Testing
- Selenium - Multi Windows Testing
- Selenium - JavaScript Executor
- Selenium - Headless Execution
- Selenium - Capture Screenshots
- Selenium - Capture Videos
- Selenium - Page Object Model
- Selenium - Page Factory
- Selenium - Record & Playback
- Selenium - Frameworks
- Selenium - Browsing Context
- Selenium Grid Tutorial
- Selenium - Overview
- Selenium - Architecture
- Selenium - Components
- Selenium - Configuration
- Selenium - Create Test Script
- Selenium - Test Execution
- Selenium - Endpoints
- Selenium - Customizing a Node
- Selenium Reporting Tools
- Selenium - Reporting Tools
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & other Technologies
- Selenium - Java Tutorial
- Selenium - Python Tutorial
- Selenium - C# Tutorial
- Selenium - Javascript Tutorial
- Selenium - Kotlin Tutorial
- Selenium - Ruby  Tutorial
- Selenium - Maven & Jenkins
- Selenium - Database Testing
- Selenium - LogExpert Logging
- Selenium - Log4j Logging
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash Testing
- Selenium - Apache Ant
- Selenium - Github
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium Miscellaneous Concepts
- Selenium - Automation Frameworks
- Selenium - Keyword Driven Framework
- Selenium - Data Driven Framework
- Selenium - Hybrid Driven Framework
- Selenium - SSL Certificate Error
- Selenium - Alternatives
- Selenium Useful Resources
- Selenium - Quick Guide
- Selenium - Useful Resources
- Selenium - Automation Practice
- Selenium - Discussion
Selenium Webdriver - Handling Cookies
Selenium Webdriver can be used to handle cookies. We can add a cookie, obtain a cookie with a particular name, and delete a cookie with the help of various methods in Selenium Webdriver.
Some of the methods on cookies are discussed below −
addCookie() − This method is used to add a cookie to the current session.
getCookieNamed() − This method is used to get a cookie with a particular name among all the created cookies. It yields none, if there is no cookie available with the name, passed as parameter.
getCookies() − This method is used to get all the cookies for the current session. If the current browser session had terminated, this method would return an error.
deleteCookieNamed() − This method is used to delete a cookie with a particular name, passed as a parameter to the method.
deleteCookie() − This method is used to delete a cookie with the cookie object, passed as a parameter to the method.
deleteAllCookies() − This method is used to delete all cookies from the current browser session.
Let us take an example where we would add and get the details of the cookie added for an existing browsing context.
Syntax
WebDriver driver = new ChromeDriver(); // Add cookie in key-value pairs driver.manage().addCookie(new Cookie("C1", "VAL1")); // Get added cookie with name Cookie c1 = driver.manage().getCookieNamed("C1"); System.out.println("Cookie details: " + c1);
Example
Code Implementation on AndGetCookie.java class file.
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class AndGetCookie { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // open browser session driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Add cookie in key-value pairs driver.manage().addCookie(new Cookie("C1", "VAL1")); // Get added cookie with name Cookie c1 = driver.manage().getCookieNamed("C1"); System.out.println("Cookie details: " + c1); // Closing browser driver.quit(); } }
Output
Cookie details: C1=VAL1; path=/; domain=www.tutorialspoint.com;secure;; sameSite=Lax Process finished with exit code 0
In the above example, we had first added a cookie with name C1 and then obtained its details as a message in the console - Cookie details: C1=VAL1; path=/; domain=www.tutorialspoint.com;secure;; sameSite=Lax.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Let us take an example where we would add multiple cookies and then get all the details of the cookie added for an existing browsing context.
Syntax
WebDriver driver = new ChromeDriver(); // Add two cookies in key-value pairs driver.manage().addCookie(new Cookie("C1", "VAL1")); driver.manage().addCookie(new Cookie("C2", "VAL2")); // get every cookie details Set<Cookie> c = driver.manage().getCookies(); System.out.println("Cookie details are: " + c);
Example
Code Implementation on AndGetCookies.java class file.
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; import java.util.Set; import java.util.concurrent.TimeUnit; public class AndGetCookies { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // open browser session driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Add two cookies in key-value pairs driver.manage().addCookie(new Cookie("C1", "VAL1")); driver.manage().addCookie(new Cookie("C2", "VAL2")); // get every cookie details Set<Cookie> c = driver.manage().getCookies(); System.out.println("Cookie details are: " + c); // Closing browser driver.quit(); } }
Output
Cookie details are: [C1=VAL1; path=/; domain=www.tutorialspoint.com;secure;; sameSite=Lax, C2=VAL2; path=/; domain=www.tutorialspoint.com;secure;; sameSite=Lax] Process finished with exit code 0
In the above example, we had first added multiple cookies with names C1 and C2 and then obtained its details as a message in the console - Cookie details are: [C1=VAL1; path=/; domain=www.tutorialspoint.com;secure;; sameSite=Lax, C2=VAL2; path=/; domain=www.tutorialspoint.com;secure;; sameSite=Lax].
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Let us take an example where we would add multiple cookies and delete a cookie(with cookie name and object) and then get all the details of the cookie added for an existing browsing context.
Syntax
WebDriver driver = new ChromeDriver(); // Add three cookies in key-value pairs driver.manage().addCookie(new Cookie("C1", "VAL1")); Cookie m = new Cookie("C2", "VAL2"); driver.manage().addCookie(m); driver.manage().addCookie(new Cookie("C3", "VAL3")); // get every cookie details Set<Cookie> c = driver.manage().getCookies(); System.out.println("Cookie details are: " + c); // delete a cookie with its name driver.manage().deleteCookieNamed("C3"); // get every cookie details after deleting cookie C3 Set<Cookie> c1 = driver.manage().getCookies(); System.out.println("Cookie details after deleting C3 cookie are: " + c1); // delete a cookie with cookie object driver.manage().deleteCookie(m); // get every cookie details after deleting cookie C2 Set<Cookie> c2 = driver.manage().getCookies(); System.out.println("Cookie details after deleting C2 cookie are: " + c2);
Example
Code Implementation on AndDeleteCookies.java class file.
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; import java.util.Set; import java.util.concurrent.TimeUnit; public class AndDeleteCookies { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // open browser session driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Add three cookies in key-value pairs driver.manage().addCookie(new Cookie("C1", "VAL1")); Cookie m = new Cookie("C2", "VAL2"); driver.manage().addCookie(m); driver.manage().addCookie(new Cookie("C3", "VAL3")); // get every cookie details Set<Cookie> c = driver.manage().getCookies(); System.out.println("Cookie details are: " + c); // delete a cookie with its name driver.manage().deleteCookieNamed("C3"); // get every cookie details after deleting cookie C3 Set<Cookie> c1 = driver.manage().getCookies(); System.out.println("Cookie details after deleting C3 cookie are: " + c1); // delete a cookie with cookie object driver.manage().deleteCookie(m); // get every cookie details after deleting cookie C2 Set<Cookie> c2 = driver.manage().getCookies(); System.out.println("Cookie details after deleting C2 cookie are: " + c2); // Closing browser driver.quit(); } }
Output
Cookie details are: [C3=VAL3; path=/; domain=www.tutorialspoint.com;secure;; sameSite=Lax, C1=VAL1; path=/; domain=www.tutorialspoint.com;secure;; sameSite=Lax, C2=VAL2; path=/; domain=www.tutorialspoint.com;secure;; sameSite=Lax] Cookie details after deleting C3 cookie are: [C1=VAL1; path=/; domain=www.tutorialspoint.com;secure;; sameSite=Lax, C2=VAL2; path=/; domain=www.tutorialspoint.com;secure;; sameSite=Lax] Cookie details after deleting C2 cookie are: [C1=VAL1; path=/; domain=www.tutorialspoint.com;secure;; sameSite=Lax] Process finished with exit code 0
In the above example, we had first added three cookies with names C1, C2, and C3. Then, deleted the cookies C3, and C2 with cookie name and object and retrieved the cookie details after deletion.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Let us take an example where we would add multiple cookies and then delete all the cookies added for an existing browsing context.
Syntax
WebDriver driver = new ChromeDriver(); // Add two cookies in key-value pairs driver.manage().addCookie(new Cookie("C1", "VAL1")); driver.manage().addCookie(new Cookie("C3", "VAL3")); // get every cookie details Set<Cookie> c = driver.manage().getCookies(); System.out.println("Cookie details are: " + c); // delete a cookie with its name driver.manage().deleteAllCookies(); // get every cookie details after deleting cookie C2 Set<Cookie> c2 = driver.manage().getCookies(); System.out.println("Cookie details after deleting all cookies: " + c2);
Example
Code Implementation on AndDeleteAllCookies.java class file.
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; import java.util.Set; import java.util.concurrent.TimeUnit; public class AndDeleteAllCookies { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // open browser session driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Add two cookies in key-value pairs driver.manage().addCookie(new Cookie("C1", "VAL1")); driver.manage().addCookie(new Cookie("C3", "VAL3")); // get every cookie details Set<Cookie> c = driver.manage().getCookies(); System.out.println("Cookie details are: " + c); // delete a cookie with its name driver.manage().deleteAllCookies(); // get every cookie details after deleting cookie C2 Set<Cookie> c2 = driver.manage().getCookies(); System.out.println("Cookie details after deleting all cookies: " + c2); // Closing browser driver.quit(); } }
Output
Cookie details are: [C3=VAL3; path=/; domain=www.tutorialspoint.com;secure;; sameSite=Lax, C1=VAL1; path=/; domain=www.tutorialspoint.com;secure;; sameSite=Lax] Cookie details after deleting all cookies: [] Process finished with exit code 0
In the above example, we had first added two cookies with names C1, and C2. Then, we had deleted all the cookies.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
In this article, we discussed how to handle cookies using Selenium Webdriver.