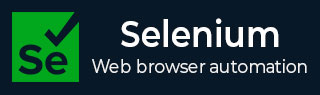
- Selenium Tutorial
- Selenium - Home
- Selenium - Overview
- Selenium - Components
- Selenium - Automation Testing
- Selenium - Environment Setup
- Selenium - Remote Control
- Selenium IDE Tutorial
- Selenium - IDE Introduction
- Selenium - Features
- Selenium - Limitations
- Selenium - Installation
- Selenium - Creating Tests
- Selenium - Creating Script
- Selenium - Store Variables
- Selenium - Alerts & Popups
- Selenium - Selenese Commands
- Selenium - Actions Commands
- Selenium - Accessors Commands
- Selenium - Assertions Commands
- Selenium - Assert/Verify Methods
- Selenium - Locating Strategies
- Selenium - Script Debugging
- Selenium - Verification Points
- Selenium - Pattern Matching
- Selenium - JSON Data File
- Selenium - Browser Execution
- Selenium - User Extensions
- Selenium - Code Export
- Selenium - JavaScript Functions
- Selenium - Plugins
- Selenium WebDriver Tutorial
- Selenium - Introduction
- Selenium WebDriver vs RC
- Selenium - Installation
- Selenium - First Test Script
- Selenium - Driver Sessions
- Selenium - Browser Options
- Selenium - Chrome Options
- Selenium - Edge Options
- Selenium - Firefox Options
- Selenium - Safari Options
- Selenium Web Elements
- Selenium - File Upload
- Selenium - Locator Strategies
- Selenium - Relative Locators
- Selenium - Finders
- Selenium - Find All Links
- Selenium - User Interactions
- Selenium - WebElement Commands
- Selenium - Browser Interactions
- Selenium - Browser Commands
- Selenium - Browser Navigation
- Selenium - Alerts & Popups
- Selenium - Handling Forms
- Selenium - Windows and Tabs
- Selenium - Handling Links
- Selenium - Input Boxes
- Selenium - Radio Button
- Selenium - Checkboxes
- Selenium - Dropdown Box
- Selenium - Handling IFrames
- Selenium - Handling Cookies
- Selenium - Date Time Picker
- Selenium - Dynamic Web Tables
- Selenium - Actions Class
- Selenium - Action Class
- Selenium - Keyboard Events
- Selenium - Key Up/Down
- Selenium - Copy and Paste
- Selenium - Handle Special Keys
- Selenium - Mouse Events
- Selenium - Drag and Drop
- Selenium - Scroll Operations
- Selenium - Waiting Strategies
- Selenium - Explicit/Implicit Wait
- Selenium - Support Features
- Selenium - Multi Select
- Selenium - Wait Support
- Selenium - Select Support
- Selenium - Color Support
- Selenium - Errors & Logging
- Selenium - Logging
- Selenium - Exception Handling
- Selenium - Miscellaneous
- Selenium - Handling Ajax Calls
- Selenium - JSON Data File
- Selenium - CSV Data File
- Selenium - Excel Data File
- Selenium - Cross Browser Testing
- Selenium - Multi Browser Testing
- Selenium - Multi Windows Testing
- Selenium - JavaScript Executor
- Selenium - Headless Execution
- Selenium - Capture Screenshots
- Selenium - Capture Videos
- Selenium - Page Object Model
- Selenium - Page Factory
- Selenium - Record & Playback
- Selenium - Frameworks
- Selenium - Browsing Context
- Selenium Grid Tutorial
- Selenium - Overview
- Selenium - Architecture
- Selenium - Components
- Selenium - Configuration
- Selenium - Create Test Script
- Selenium - Test Execution
- Selenium - Endpoints
- Selenium - Customizing a Node
- Selenium Reporting Tools
- Selenium - Reporting Tools
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & other Technologies
- Selenium - Java Tutorial
- Selenium - Python Tutorial
- Selenium - C# Tutorial
- Selenium - Javascript Tutorial
- Selenium - Kotlin Tutorial
- Selenium - Ruby Tutorial
- Selenium - Maven & Jenkins
- Selenium - Database Testing
- Selenium - LogExpert Logging
- Selenium - Log4j Logging
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash Testing
- Selenium - Apache Ant
- Selenium - Github
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium Miscellaneous Concepts
- Selenium - Automation Frameworks
- Selenium - Keyword Driven Framework
- Selenium - Data Driven Framework
- Selenium - Hybrid Driven Framework
- Selenium - SSL Certificate Error
- Selenium - Alternatives
- Selenium Useful Resources
- Selenium - Quick Guide
- Selenium - Useful Resources
- Selenium - Automation Practice
- Selenium - Discussion
Selenium with Python Tutorial
Selenium can be used with multiple languages like Java, Python, JavaScript, Ruby, and so on. Selenium is used extensively for web automation testing. Selenium is an open-source and a portable automated software testing tool for testing web applications. It has capabilities to operate across different browsers and operating systems. Selenium is not just a single tool but a set of tools that helps testers to automate web-based applications more efficiently.
Setup Selenium with Python and Launch Browser
Step 1 − Download and install Python from the link − https://www.python.org/downloads/.
To get a more detailed view on how set up Python, refer to the link − Python Environment.
Once we have successfully installed Python, we can confirm its installation by running the command: python –version, from the command prompt. The output of the command executed would point to the Python version installed in the machine.
Step 2 − Install the Python code editor called the PyCharm from the link − https://www.jetbrains.com/pycharm/.
Using this editor, we can start working on a Python project to start our test automation. To get a more detailed view on how to set up PyCharm, refer to the link − PyCharm.
Step 3 − Run the command: pip install selenium from the command prompt. This is done to install the Selenium. To confirm the version of Selenium installed in the machine, run the command −
pip show selenium
The output of this command gave the below result −
Name: selenium Version: 4.19.0 Summary: None Home-page: https://www.selenium.dev Author: None Author-email: None License: Apache 2.0.
Step 4 − Restart PyCharm once Step 3 has been completed.
Step 5 − Open PyCharm and create a New Project by navigating to the File menu.
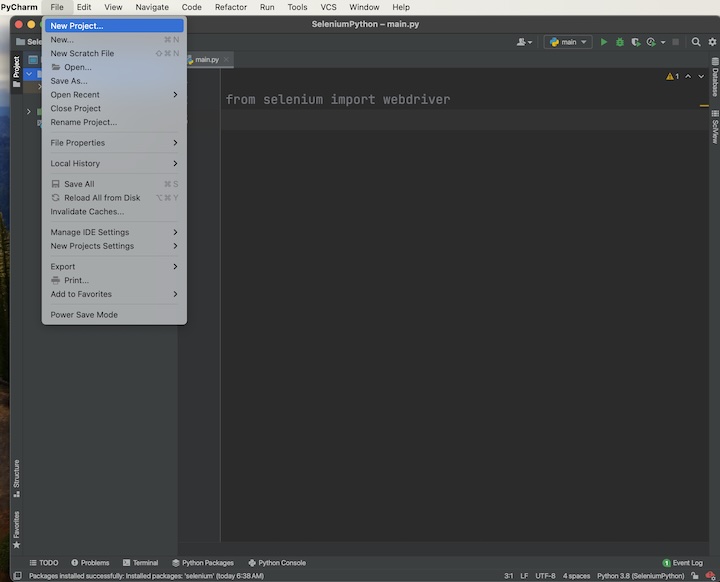
Enter the project name and location within the Location field, and then click on the Create button.
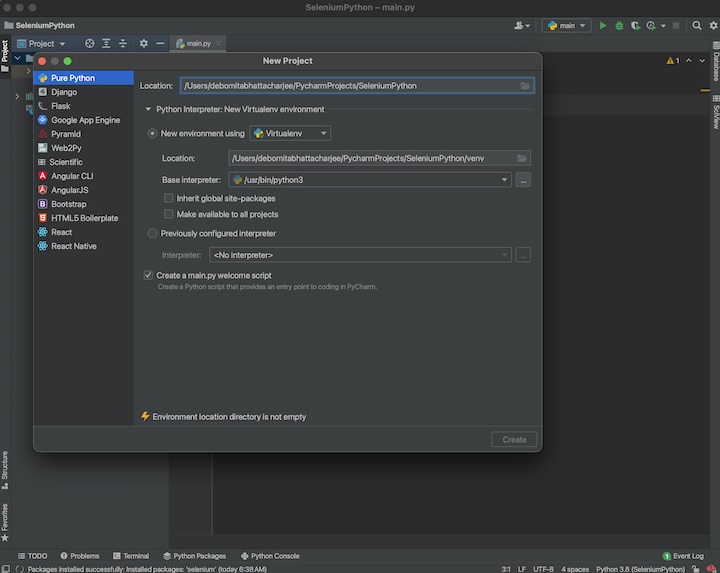
Step 6 − From the right bottom corner of the PyCharm editor, select the option Interpreter Settings.
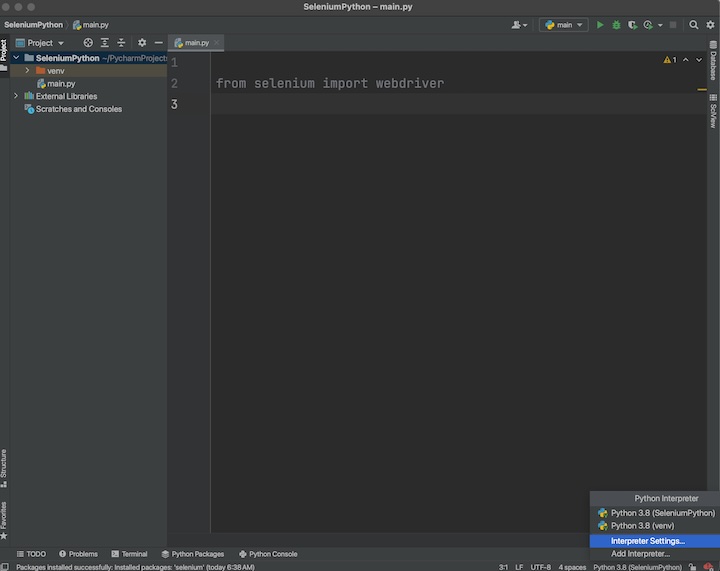
Select the option Python Interpreter from the left, then click on '+'.
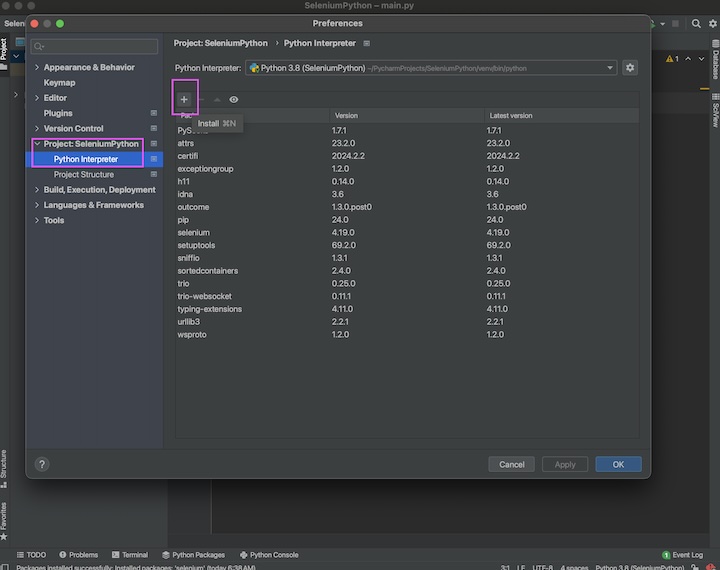
Step 7 − Enter selenium in the package search box inside the Available Packages popup, then the search results appear along with the Description to the right. The Description contains information about the version of the Selenium package that shall be installed.
There is also an option to install a specific version of the Selenium package beside the Specify version field. Then click on the Install Package button. After successful installation, the message Package 'selenium' installed successfully should display.
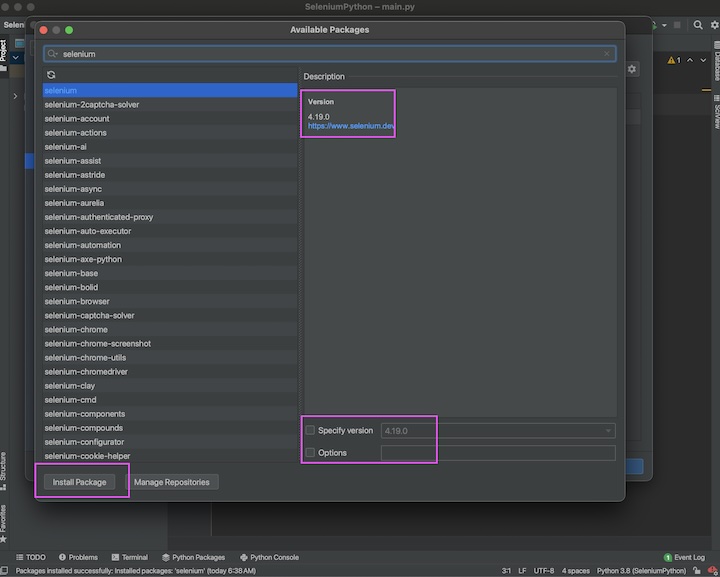
Step 8 − Enter webdriver-manager in the package search box inside the Available Packages popup, and install it in the same way.
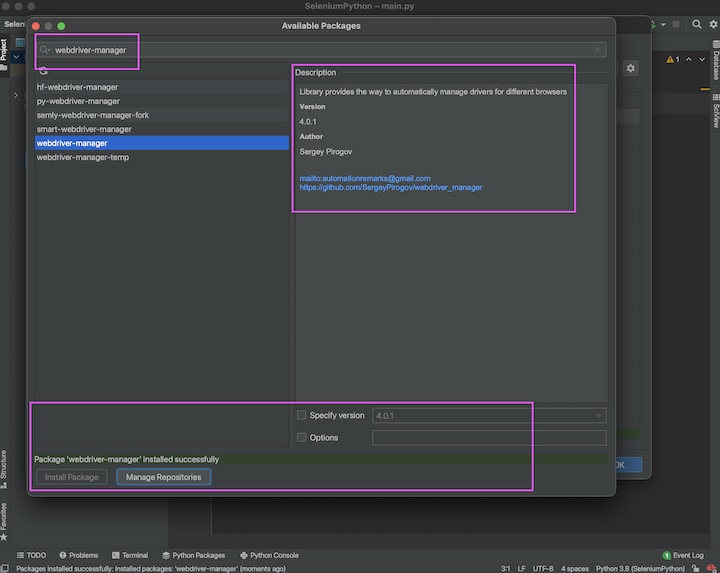
Exit out of the Available Packages popup.
Step 9 − Both the packages selenium, and webdriver-manager should reflect under the Package. Click on the OK button. Restart PyCharm.
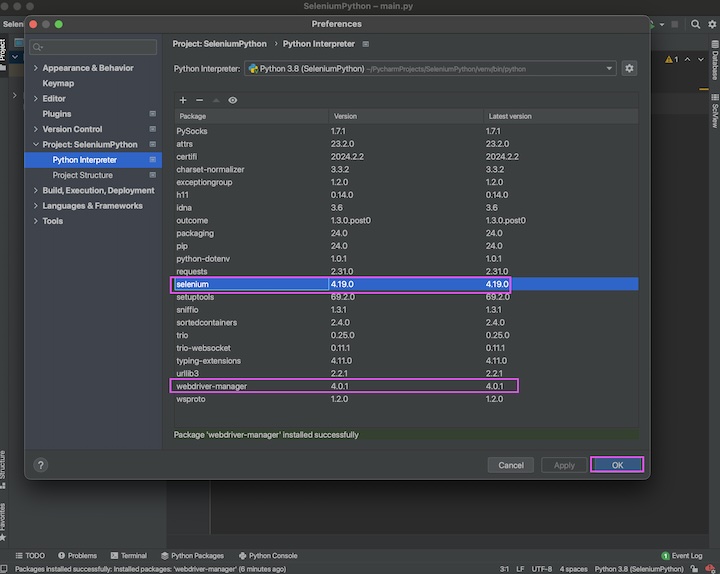
Step 10 − Create the first test case, by right clicking on the Project folder. Here, we have given the project name as SeleniumPython. Then click on New and finally click on the Python File option.
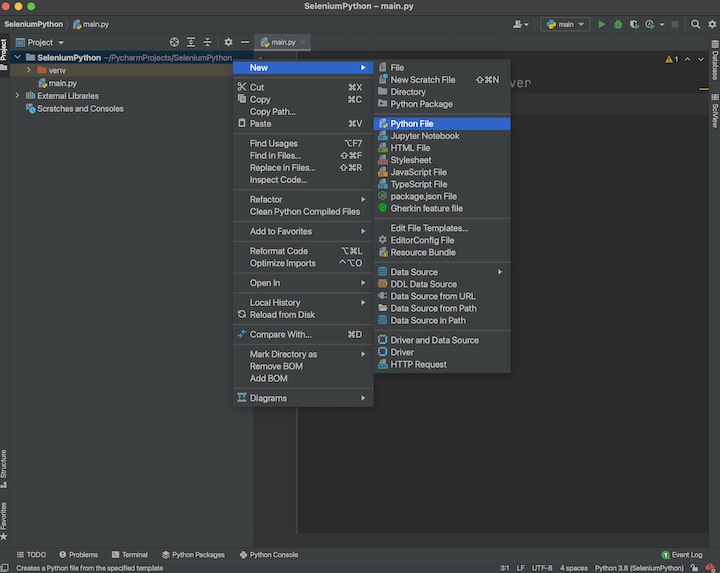
Step 11 − Enter a filename, say Test1.py and select the option Python File, finally click on Enter.
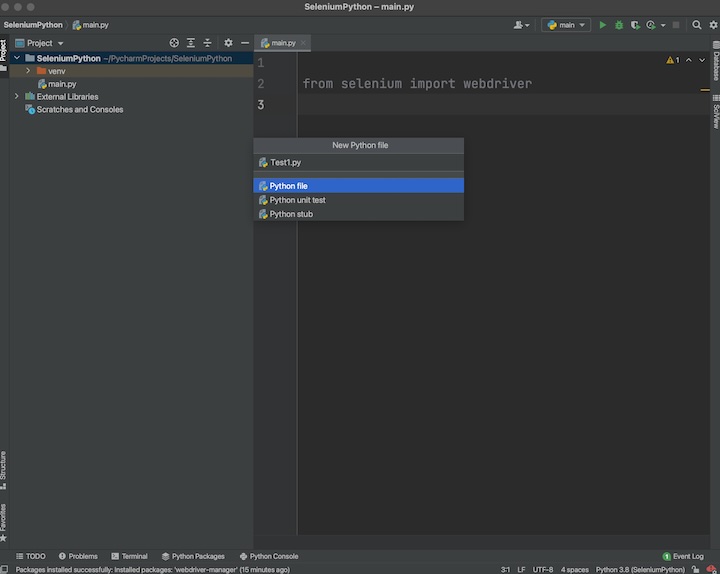
The Test1.py should appear in the left under the SeleniumPython project folder.
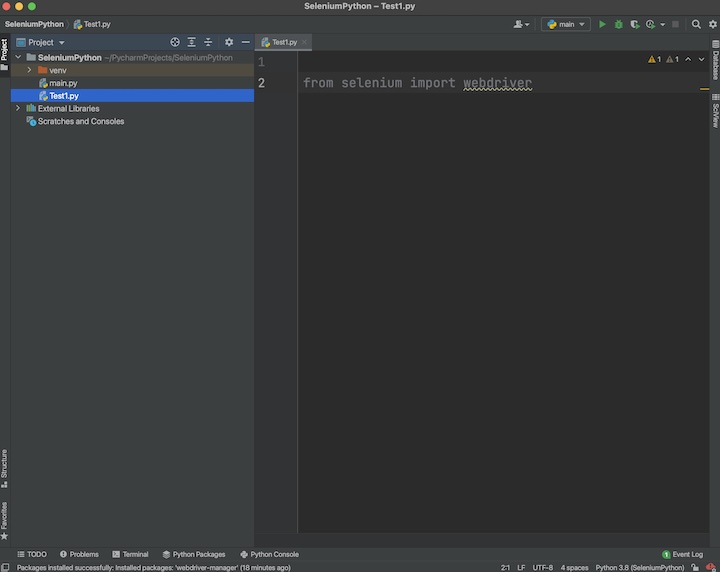
Step 12 − Open the newly created Python file with the name Test1.py, and obtain the page title - Selenium Practice - Alerts of the below page.
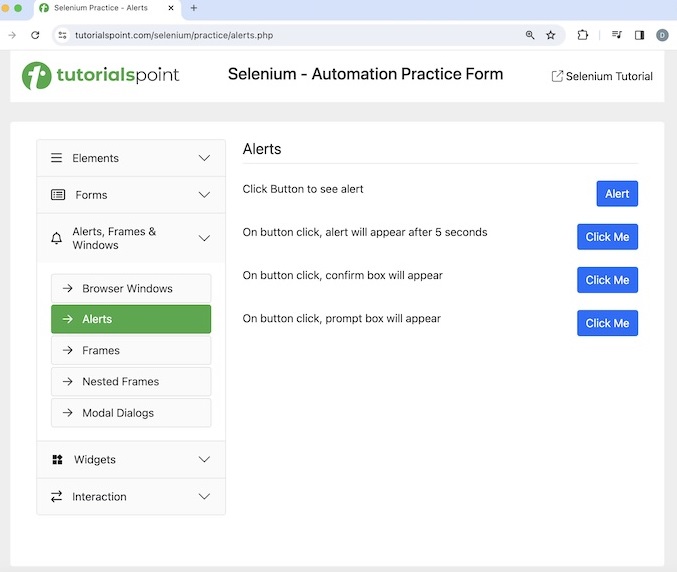
Example
from selenium import webdriver # create instance of webdriver driver = webdriver.Chrome() # launch application driver.get("https://www.tutorialspoint.com/selenium/practice/alerts.php") # get page title print("Page title is: " + driver.title) # quitting browser driver.quit
Output
Page title is: Selenium Practice - Alerts Process finished with exit code 0
In the above example, we had launched an application and obtained its page title in the console with the message - Page title is: Selenium Practice - Alerts.
The output shows the message - Process with exit code 0 meaning that the above code executed successfully.
Identify Element and Check Its Functionality using Selenium Python
Once we navigate to a webpage, we have to interact with the web elements available on the page like clicking a link/button, entering text within an edit box, and so on to complete our automation test case.
For this, our first job is to identify the element. There are some locators available in Selenium for this purpose, they are id, class, class name, name, link text, partial link text, tagname, css, and xpath. These locators need to be used with the find_element() method.
For example, driver.find_element("classname", 'btn-primary') will locate the first web element with the given class name attribute value. In case there is no element with the matching value of the class name attribute, NoSuchElementException should be thrown.
Let us see the html code of the Click Me button highlighted in the below image −
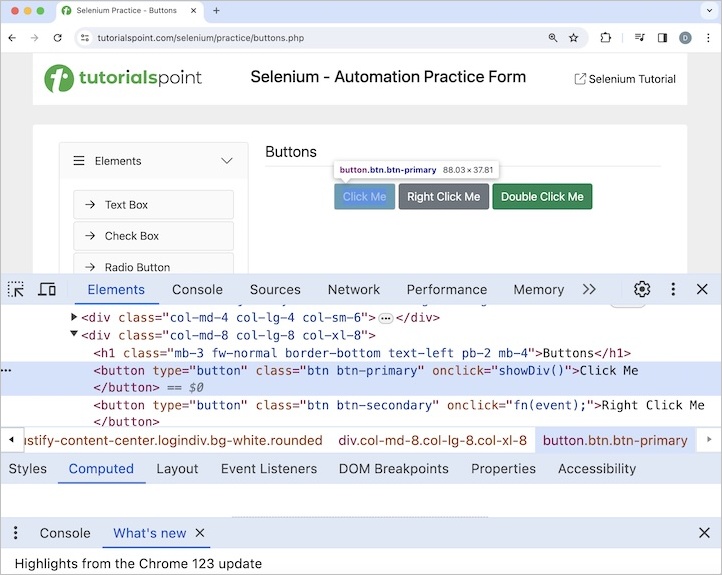
<button type="button" class="btn btn-primary" onclick="showDiv()">Click Me</button>
It has a class name attribute with value as btn-primary. Let us click on that Click Me button after which we would get the text You have done a dynamic click on the page.

Example
from selenium import webdriver from selenium.webdriver.common.by import By # create instance of webdriver driver = webdriver.Chrome() # implicit wait of 15 seconds driver.implicitly_wait(15) # launch application driver.get("https://www.tutorialspoint.com/selenium/practice/buttons.php") # identify element with class name button = driver.find_element(By.CLASS_NAME, 'btn-primary') # click on button button.click() # identify element with id txt = driver.find_element(By.ID, 'welcomeDiv') # get text print("Text obtained is: " + txt.text) # quitting browser driver.quit
Output
Text obtained is: You have done a dynamic click Process finished with exit code 0
The output shows the message - Process with exit code 0 meaning that the above code executed successfully. Also, we obtained the text after clicking the Click Me button with the message in the console- Text is: You have done a dynamic click.
This concludes our comprehensive take on the tutorial on Selenium - Python Tutorial. We’ve started with describing how to set up Selenium with Python and launch a browser, and how to identify an element and check its functionality using Selenium Python.
This equips you with in-depth knowledge of the Selenium - Python Tutorial. It is wise to keep practicing what you’ve learned and exploring others relevant to Selenium to deepen your understanding and expand your horizons.