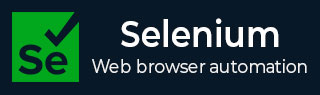
- Selenium Tutorial
- Selenium - Home
- Selenium - Overview
- Selenium - Components
- Selenium - Automation Testing
- Selenium - Environment Setup
- Selenium - Remote Control
- Selenium IDE Tutorial
- Selenium - IDE Introduction
- Selenium - Features
- Selenium - Limitations
- Selenium - Installation
- Selenium - Creating Tests
- Selenium - Creating Script
- Selenium - Store Variables
- Selenium - Alerts & Popups
- Selenium - Selenese Commands
- Selenium - Actions Commands
- Selenium - Accessors Commands
- Selenium - Assertions Commands
- Selenium - Assert/Verify Methods
- Selenium - Locating Strategies
- Selenium - Script Debugging
- Selenium - Verification Points
- Selenium - Pattern Matching
- Selenium - JSON Data File
- Selenium - Browser Execution
- Selenium - User Extensions
- Selenium - Code Export
- Selenium - JavaScript Functions
- Selenium - Plugins
- Selenium WebDriver Tutorial
- Selenium - Introduction
- Selenium WebDriver vs RC
- Selenium - Installation
- Selenium - First Test Script
- Selenium - Driver Sessions
- Selenium - Browser Options
- Selenium - Chrome Options
- Selenium - Edge Options
- Selenium - Firefox Options
- Selenium - Safari Options
- Selenium Web Elements
- Selenium - File Upload
- Selenium - Locator Strategies
- Selenium - Relative Locators
- Selenium - Finders
- Selenium - Find All Links
- Selenium - User Interactions
- Selenium - WebElement Commands
- Selenium - Browser Interactions
- Selenium - Browser Commands
- Selenium - Browser Navigation
- Selenium - Alerts & Popups
- Selenium - Handling Forms
- Selenium - Windows and Tabs
- Selenium - Handling Links
- Selenium - Input Boxes
- Selenium - Radio Button
- Selenium - Checkboxes
- Selenium - Dropdown Box
- Selenium - Handling IFrames
- Selenium - Handling Cookies
- Selenium - Date Time Picker
- Selenium - Dynamic Web Tables
- Selenium - Actions Class
- Selenium - Action Class
- Selenium - Keyboard Events
- Selenium - Key Up/Down
- Selenium - Copy and Paste
- Selenium - Handle Special Keys
- Selenium - Mouse Events
- Selenium - Drag and Drop
- Selenium - Scroll Operations
- Selenium - Waiting Strategies
- Selenium - Explicit/Implicit Wait
- Selenium - Support Features
- Selenium - Multi Select
- Selenium - Wait Support
- Selenium - Select Support
- Selenium - Color Support
- Selenium - Errors & Logging
- Selenium - Logging
- Selenium - Exception Handling
- Selenium - Miscellaneous
- Selenium - Handling Ajax Calls
- Selenium - JSON Data File
- Selenium - CSV Data File
- Selenium - Excel Data File
- Selenium - Cross Browser Testing
- Selenium - Multi Browser Testing
- Selenium - Multi Windows Testing
- Selenium - JavaScript Executor
- Selenium - Headless Execution
- Selenium - Capture Screenshots
- Selenium - Capture Videos
- Selenium - Page Object Model
- Selenium - Page Factory
- Selenium - Record & Playback
- Selenium - Frameworks
- Selenium - Browsing Context
- Selenium Grid Tutorial
- Selenium - Overview
- Selenium - Architecture
- Selenium - Components
- Selenium - Configuration
- Selenium - Create Test Script
- Selenium - Test Execution
- Selenium - Endpoints
- Selenium - Customizing a Node
- Selenium Reporting Tools
- Selenium - Reporting Tools
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & other Technologies
- Selenium - Java Tutorial
- Selenium - Python Tutorial
- Selenium - C# Tutorial
- Selenium - Javascript Tutorial
- Selenium - Kotlin Tutorial
- Selenium - Ruby Tutorial
- Selenium - Maven & Jenkins
- Selenium - Database Testing
- Selenium - LogExpert Logging
- Selenium - Log4j Logging
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash Testing
- Selenium - Apache Ant
- Selenium - Github
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium Miscellaneous Concepts
- Selenium - Automation Frameworks
- Selenium - Keyword Driven Framework
- Selenium - Data Driven Framework
- Selenium - Hybrid Driven Framework
- Selenium - SSL Certificate Error
- Selenium - Alternatives
- Selenium Useful Resources
- Selenium - Quick Guide
- Selenium - Useful Resources
- Selenium - Automation Practice
- Selenium - Discussion
Selenium WebDriver - Locator Strategies
Once we navigate to a webpage, we have to interact with the web elements available on the page like clicking a link/button, entering text within an edit box, and so on to complete our automation test case.
By Id
For this, our first job is to identify the element. We can use the id attribute for an element for its identification and utilize the method findElement(By.id(“<value of id attribute>")). With this, the first element with the matching value of the attribute id is returned.
In case there is no element with the matching value of the id attribute, NoSuchElementException shall be thrown.
Syntax
The syntax for identifying an element with id attribute is as follows −
Webdriver driver = new ChromeDriver(); driver.findElement(By.id("id value"));
Let us see the html code of the input box beside the Name label highlighted in the below image −
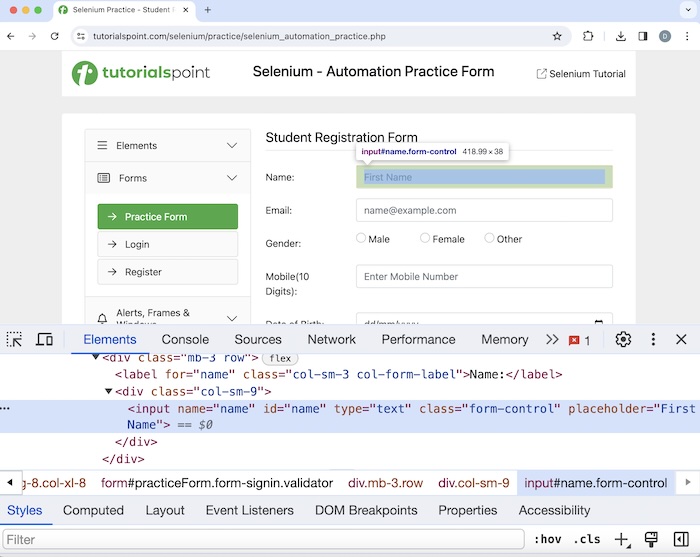
The edit box highlighted in the above image has an id attribute with value name. Let us input the text Selenium into this edit box after identifying it.
Example
Code Implementation on LocatorsID.java class file.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class LocatorsID { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 20 secs driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS); // Opening the webpage where we will identify edit box enter text driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Identify the search box with id locator then enter text Selenium WebElement i = driver.findElement(By.id("name")); i.sendKeys("Selenium"); // Get the value entered String text = i.getAttribute("value"); System.out.println("Entered text is: " + text); // Closing browser driver.quit(); } }
Output
Entered text is: Selenium Process finished with exit code 0
The output shows the message - Process with exit code 0 meaning that the above code executed successfully. Also, the value entered within the edit box (obtained from the getAttribute method) - Selenium got printed in the console.
By Name
Once we navigate to a webpage, we have to interact with the web elements available on the page like clicking a link/button, entering text within an edit box, and so on to complete our automation test case.
For this, our first job should be to identify the element. We can use the name attribute for an element for its identification and utilize the method findElement(By.name(“<value of name attribute>")). With this, the first element with the matching value of the attribute name should be returned.
In case there is no element with the matching value of the name attribute, NoSuchElementException should be thrown.
Syntax
The syntax for identifying an element with name attribute is as follows −
Webdriver driver = new ChromeDriver(); driver.findElement(By.name("name value"));
Let us see the html code of the same input box as discussed before in the below image −
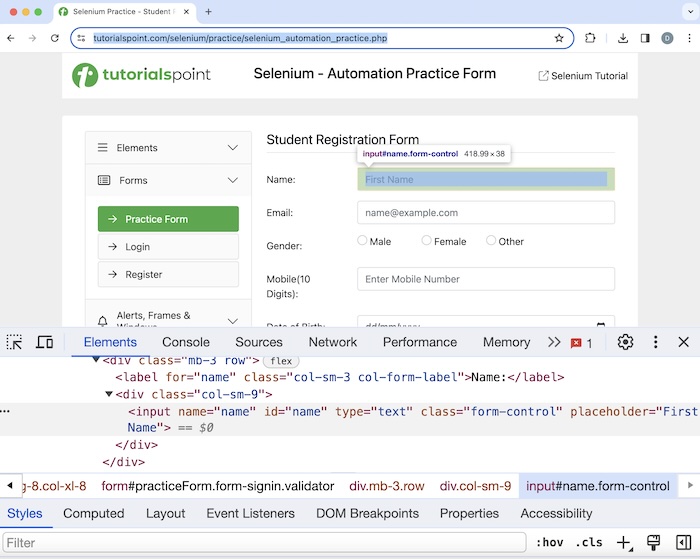
The edit box highlighted in the above image has a name attribute with a value as name. Let us input the text Selenium into this edit box after identifying it.
Example
Code Implementation on LocatorsName.java class file.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class LocatorsName { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 20 secs driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS); // Opening the webpage where we will identify edit box enter text driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Identify the search box with name locator to enter text WebElement i = driver.findElement(By.name("name")); i.sendKeys("Selenium"); // Get the value entered String text = i.getAttribute("value"); System.out.println("Entered text is: " + text); // Closing browser driver.quit(); } }
Output
Entered text is: Selenium Process finished with exit code 0
The output shows the message - Process with exit code 0 meaning that the above code executed successfully. Also, the value entered within the edit box (obtained from the getAttribute method) - Selenium got printed in the console.
By ClassName
Once we navigate to a webpage, we have to interact with the web elements available on the page like clicking a link/button, entering text within an edit box, and so on to complete our automation test case.
For this, our first job should be to identify the element. We can use the class name attribute for an element for its identification and utilize the method findElement(By.className(“<value of class name attribute>")). With this, the first element with the matching value of the attribute class name should be returned.
In case there is no element with the matching value of the class name attribute, NoSuchElementException should be thrown.
Syntax
The syntax for identifying an element with class name attribute is as follows −
Webdriver driver = new ChromeDriver(); driver.findElement(By.className("class name value"));
Let us see the html code of the Click Me button highlighted in the below image −
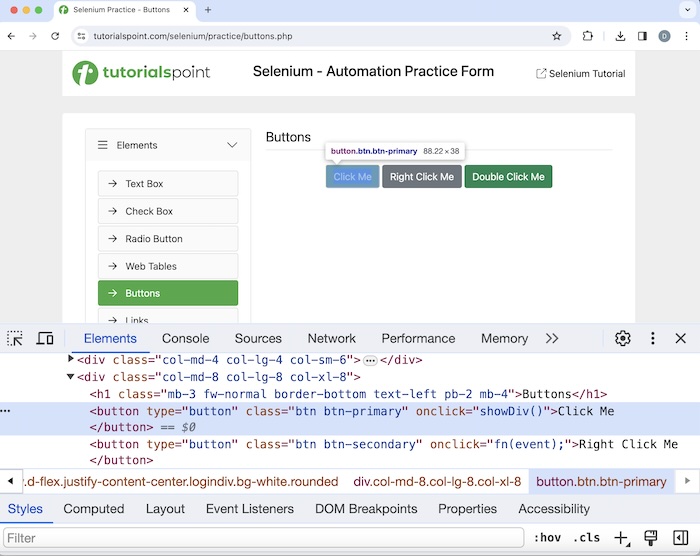
It has a class name attribute with value as btn-primary. Let us click on that element after which we would get the text You have done a dynamic click on the page.
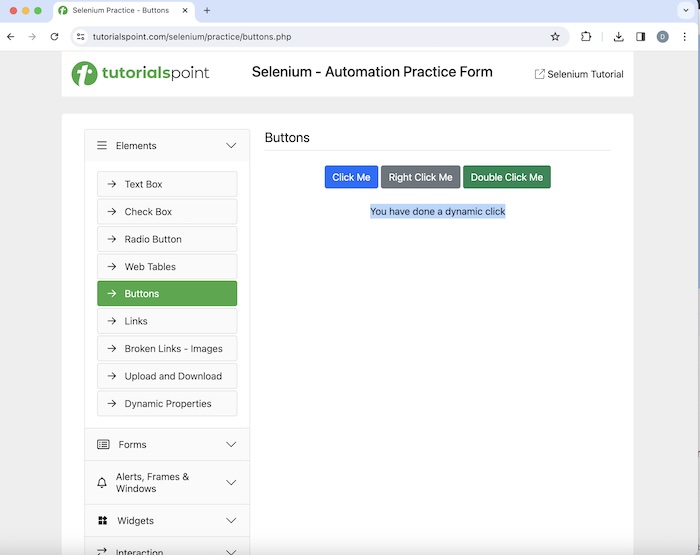
Example
Code Implementation on LocatorsClassName.java class file.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class LocatorsClassName { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 20 secs driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS); // Opening the webpage where we will identify button to click driver.get("https://www.tutorialspoint.com/selenium/practice/buttons.php"); // Identify button with class name to click WebElement i = driver.findElement(By.className("btn-primary")); i.click(); // Get text after click WebElement e = driver.findElement(By.xpath("//*[@id='welcomeDiv']")); String text = e.getText(); System.out.println("Text is: " + text); // Closing browser driver.quit(); } }
Output
Text is: You have done a dynamic click Process finished with exit code 0
The output shows the message - Process with exit code 0 meaning that the above code executed successfully. Also, we obtained the text after clicking the Click Me button with the message in the console- Text is: You have done a dynamic click.
By TagName
Once we navigate to a webpage, we have to interact with the web elements available on the page like clicking a link/button, entering text within an edit box, and so on to complete our automation test case.
For this, our first job should be to identify the element. We can use the tag name for an element for its identification and utilize the method findElement(By.tagName(“<value of tag name>")). With this, the first element with the matching value of the tag name should be returned.
In case there is no element with the matching value of tag name, NoSuchElementException should be thrown.
Syntax
The syntax for identifying an element with tag name is as follows −
Webdriver driver = new ChromeDriver(); driver.findElement(By.tagName("tag name value"));
Let us see the html code of the input box beside the text Name in the below image −
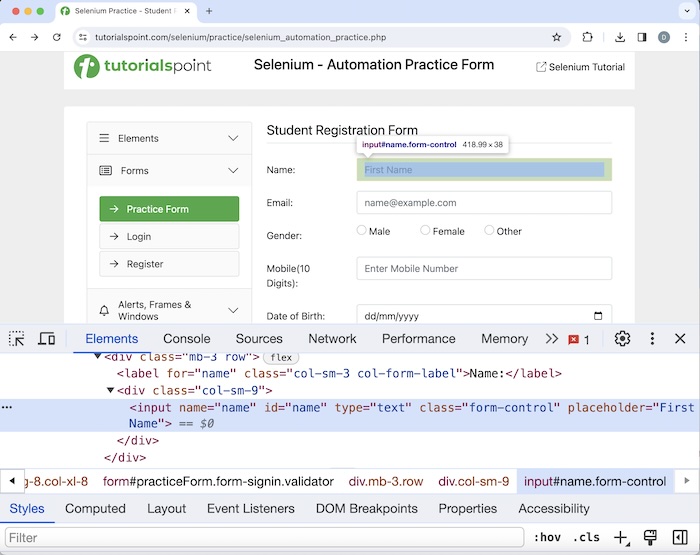
The input box highlighted in the above image has the tag name as input. Let us enter the text Selenium in that input box.
Example
Code Implementation on LocatorsTagName.java class file.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class LocatorsTagName { public static void main(String[] args) throws InterruptedException { //Initiate the Webdriver WebDriver driver = new ChromeDriver(); //adding implicit wait of 10 secs driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // launch a URL driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Identify input box with tagname locator WebElement t = driver.findElement(By.tagName("input")); // then enter text t.sendKeys("Selenium"); // Get the value entered String text = t.getAttribute("value"); System.out.println("Entered text is: " + text); // Closing browser driver.quit(); } }
Output
Entered text is: Selenium Process finished with exit code 0
The output shows the message - Process with exit code 0 meaning that the above code executed successfully. Also, the value entered within the edit box (obtained from the getAttribute method) - Selenium got printed in the console.
By LinkText
Once we navigate to a webpage, we have to interact with the web elements available on the page like clicking a link/button, entering text within an edit box, and so on to complete our automation test case.
For this, our first job should be to identify the element. We can use the link text for a link for its identification and utilize the method findElement(By.linkText(“<value of link text>")). With this, the first element with the matching value of the link text should be returned.
In case there is no element with the matching value of the link text, NoSuchElementException should be thrown.
Syntax
The syntax for identifying an element with tag name is as follows −
Webdriver driver = new ChromeDriver(); driver.findElement(By.linkText("value of link text"));
Let us see the html code of the link Created highlighted in the below image −
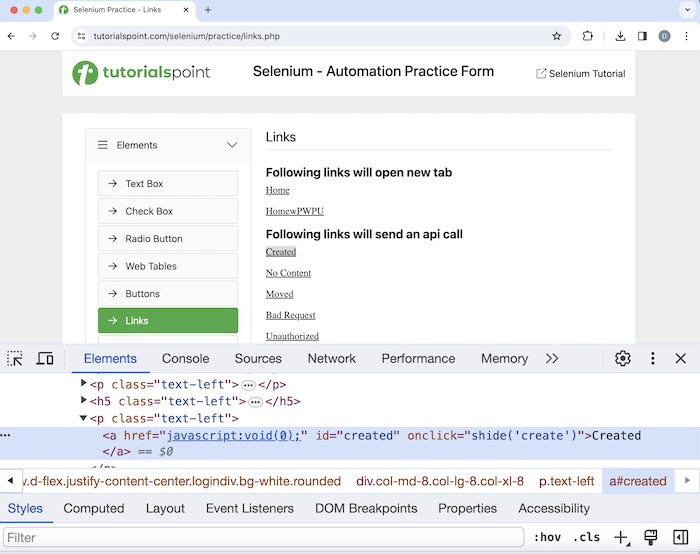
It has the link text value as Created. Let us click on that after which we would get the text Link has responded with status 201 and status text Created on the page.
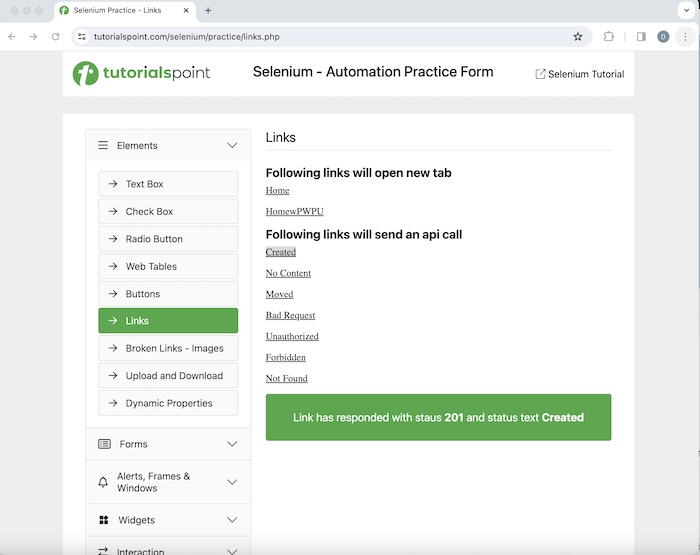
Example
Code Implementation on LocatorsLinkText.java class file.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class LocatorsLinkText { public static void main(String[] args) throws InterruptedException { //Initiate the Webdriver WebDriver driver = new ChromeDriver(); //adding implicit wait of 10 secs driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // launch a URL driver.get("https://www.tutorialspoint.com/selenium/practice/links.php"); // Identify link with tagname link text WebElement t = driver.findElement(By.linkText("Created")); // then click t.click(); // Get the text WebElement e = driver.findElement (By.xpath("/html/body/main/div/div/div[2]/div[1]")); String text = e.getText(); System.out.println("Text is: " + text); // Closing browser driver.quit(); } }
Output
Text is: Link has responded with status 201 and status text Created Process finished with exit code 0
The output shows the message - Process with exit code 0 meaning that the above code executed successfully. Also, the text obtained after clicking the Created link(obtained from the getText() method) - Link has responded with status 201 and status text Created got printed in the console.
By PartialLinkText
Once we navigate to a webpage, we have to interact with the web elements available on the page like clicking a link/button, entering text within an edit box, and so on to complete our automation test case.
For this, our first job should be to identify the element. We can use the partial link text for a link for its identification and utilize the method findElement(By.partialLinkText(“<value of partial link text>")). With this, the first element with the matching value of the partial link text should be returned.
In case there is no element with the matching value of the partial link text, NoSuchElementException should be thrown.
Syntax
The syntax for identifying an element with partial link text is as follows −
Webdriver driver = new ChromeDriver(); driver.findElement(By.partialLinkText("value of partial link text"));
Let us see the html code of the link Bad Request highlighted in the below image −
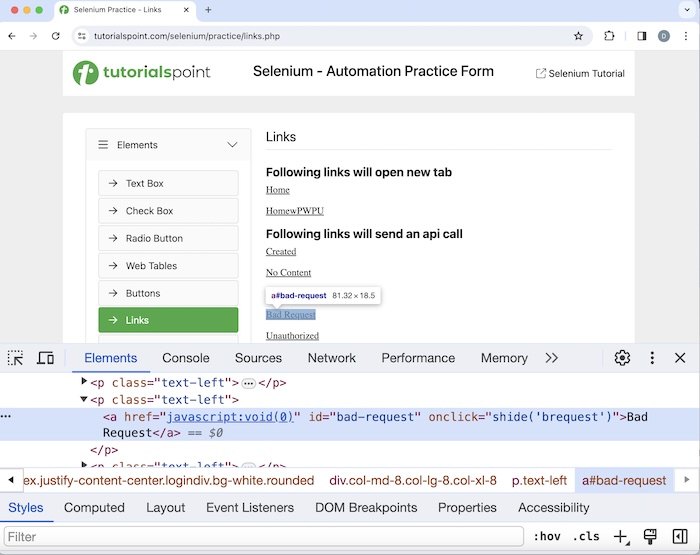
It has the link text value as Bad Request. Let us click on that after which we would get the text Link has responded with status 400 and status text Bad Request on the page.
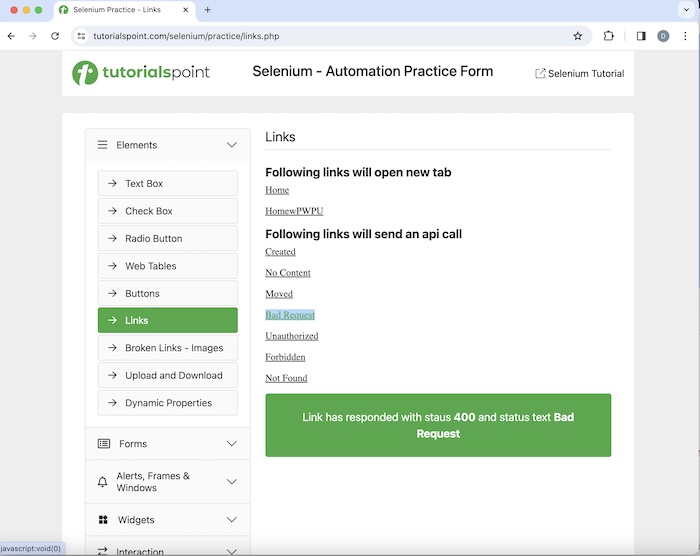
Example
Code Implementation on LocatorPartialsLinkText.java class file.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class LocatorPartialsLinkText { public static void main(String[] args) throws InterruptedException { //Initiate the Webdriver WebDriver driver = new ChromeDriver(); //adding implicit wait of 10 secs driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // launch a URL driver.get("https://www.tutorialspoint.com/selenium/practice/links.php"); // Identify link with tagname partial link text WebElement t = driver.findElement(By.partialLinkText("Bad")); // then click t.click(); // Get the text WebElement e = driver.findElement (By.xpath("/html/body/main/div/div/div[2]/div[4]")); String text = e.getText(); System.out.println("Text is: " + text); // Closing browser driver.quit(); } }
Output
Text is: Link has responded with status 400 and status text Bad Request Process finished with exit code 0
The output shows the message - Process with exit code 0 meaning that the above code executed successfully. Also, the text obtained after clicking the Bad Request link(obtained from the getText() method) - Link has responded with status 400 and status text Bad Request got printed in the console.
By CSS Selector
Once we navigate to a webpage, we have to interact with the web elements available on the page like clicking a link/button, entering text within an edit box, and so on to complete our automation test case.
For this, our first job should be to identify the element. We can create a css selector for an element for its identification and utilize the method findElement(By.cssSelector(“<value of css selector>")). With this, the first element with the matching value of the css selector should be returned.
In case there is no element with the matching value of the css selector, NoSuchElementException should be thrown.
Syntax
The syntax for identifying an element with css selector is as follows −
Webdriver driver = new ChromeDriver(); driver.findElement(By.cssSelector("value of css selector"));
Rules to Create CSS Expression
The rules to create a css expression are discussed below:
To identify the element with css, the expression should be tagname[attribute='value'].
We can also specifically use the id attribute to create a css expression. With id, the format of a css expression should be tagname#id. For example, input#txt [here input is the tagname and the txt is the value of the id attribute].
With class, the format of css expression should be tagname.class. For example, input.cls-txt [here input is the tagname and the cls-txt is the value of the class attribute].
If there are n children of a parent element, and we want to identify the nth child, the css expression should have nth-of –type(n). Similarly, to identify the last child, the css expression should be ul.reading li:last-child.
In the above code, if we want to identify the fourth li child of ul[Questions and Answers], the css expression should be ul.reading li:nth-of-type(4). Similarly, to identify the last child, the css expression should be ul.reading li:last-child.
For attributes whose values are dynamically changing, we can use ^= to locate an element whose attribute value starts with a particular text. For example, input[name^='qa'] Here, input is the tagname and the value of the name attribute starts with qa.
For attributes whose values are dynamically changing, we can use $ = to locate an element whose attribute value ends with a particular text. For example, input[class $ ='txt'] Here, input is the tagname and the value of the class attribute ends with txt.
For attributes whose values are dynamically changing, we can use *= to locate an element whose attribute value contains a specific sub-text. For example, input[name*='nam'] Here, input is the tagname and the value of the name attribute contains the sub-text nam.
Let us see the html code of the input box beside the Name label as given below −
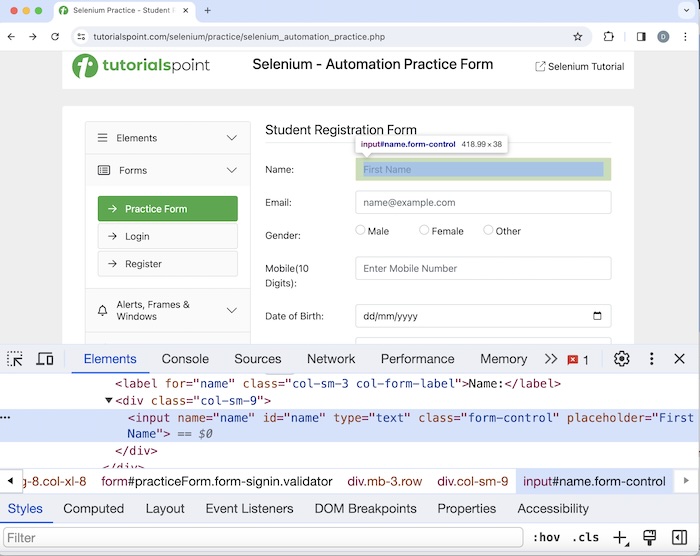
The edit box highlighted in the above image has a name attribute with value name, the css expression should be input[name='name'].
ByXpath
Once we navigate to a webpage, we have to interact with the web elements available on the page like clicking a link/button, entering text within an edit box, and so on to complete our automation test case.
For this, our first job should be to identify the element. We can create an xpath for an element for its identification and utilize the method findElement(By.xpath(“<value of xpath>")). With this, the first element with the matching value of the xpath should be returned.
In case there is no element with the matching value of the xpath, NoSuchElementException shall be thrown.
Syntax
The syntax for identifying an element with xpath is as follows −
Webdriver driver = new ChromeDriver(); driver.findElement(By.xpath("value of xpath"));
Rules to Create Xpath Expression
To identify the element with xpath, the expression should be //tagname[@attribute='value']. There can be two types of xpath – relative and absolute. The absolute xpath begins with / symbol and starts from the root node up to the element that we want to identify.
For example −
/html/body/div[1]/div/div[1]/a
The relative xpath begins with // symbol and does not start from the root node.
For example −
//img[@alt='tutorialspoint']
Let us see the html code of the highlighted link - Home starting from the root.
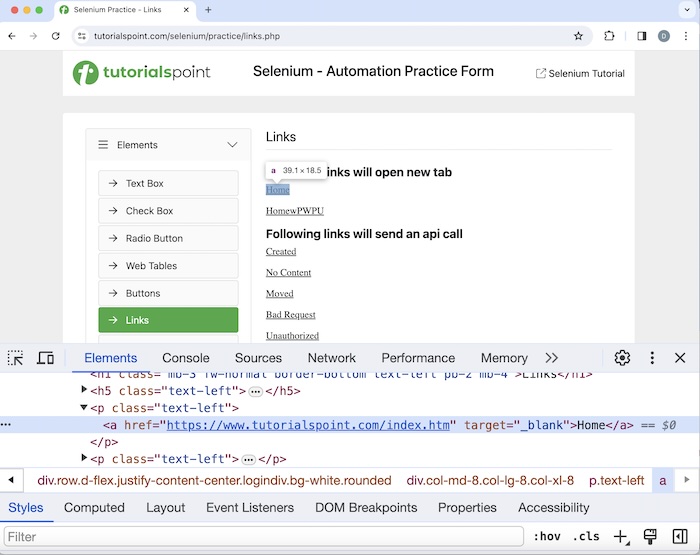
The absolute xpath for this element can be as follows −
/html/body/main/div/div/div[2]/p[1]/a
The relative xpath for element Home can be as follows −
//a[@href='https://www.tutorialspoint.com/index.htm']
Functions
There are also functions available which help to frame relative xpath expressions.
text()
It is used to identify an element with its visible text on the page. The xpath expression for the Home link is as follows −
//*[text()='Home']
starts-with
It is used to identify an element whose attribute value begins with a specific text. This function is normally used for attributes whose value changes on each page load.
Let us see the html of the link Moved −
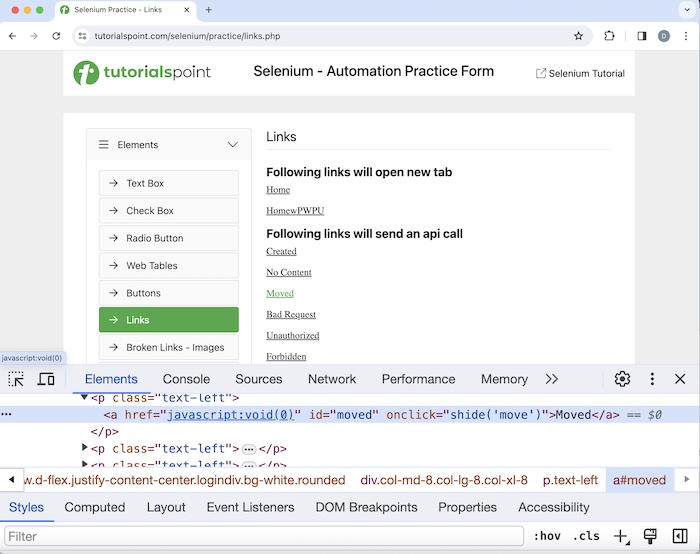
The xpath expression should be as follows −
//a[starts-with(@id, 'mov')].
In this tutorial, we had discussed the locator strategies used in Selenium Webdriver.