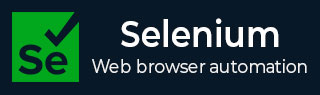
- Selenium Tutorial
- Selenium - Home
- Selenium - Overview
- Selenium - Components
- Selenium - Automation Testing
- Selenium - Environment Setup
- Selenium - Remote Control
- Selenium IDE Tutorial
- Selenium - IDE Introduction
- Selenium - Features
- Selenium - Limitations
- Selenium - Installation
- Selenium - Creating Tests
- Selenium - Creating Script
- Selenium - Store Variables
- Selenium - Alerts & Popups
- Selenium - Selenese Commands
- Selenium - Actions Commands
- Selenium - Accessors Commands
- Selenium - Assertions Commands
- Selenium - Assert/Verify Methods
- Selenium - Locating Strategies
- Selenium - Script Debugging
- Selenium - Verification Points
- Selenium - Pattern Matching
- Selenium - JSON Data File
- Selenium - Browser Execution
- Selenium - User Extensions
- Selenium - Code Export
- Selenium - JavaScript Functions
- Selenium - Plugins
- Selenium WebDriver Tutorial
- Selenium - Introduction
- Selenium WebDriver vs RC
- Selenium - Installation
- Selenium - First Test Script
- Selenium - Driver Sessions
- Selenium - Browser Options
- Selenium - Chrome Options
- Selenium - Edge Options
- Selenium - Firefox Options
- Selenium - Safari Options
- Selenium Web Elements
- Selenium - File Upload
- Selenium - Locator Strategies
- Selenium - Relative Locators
- Selenium - Finders
- Selenium - Find All Links
- Selenium - User Interactions
- Selenium - WebElement Commands
- Selenium - Browser Interactions
- Selenium - Browser Commands
- Selenium - Browser Navigation
- Selenium - Alerts & Popups
- Selenium - Handling Forms
- Selenium - Windows and Tabs
- Selenium - Handling Links
- Selenium - Input Boxes
- Selenium - Radio Button
- Selenium - Checkboxes
- Selenium - Dropdown Box
- Selenium - Handling IFrames
- Selenium - Handling Cookies
- Selenium - Date Time Picker
- Selenium - Dynamic Web Tables
- Selenium - Actions Class
- Selenium - Action Class
- Selenium - Keyboard Events
- Selenium - Key Up/Down
- Selenium - Copy and Paste
- Selenium - Handle Special Keys
- Selenium - Mouse Events
- Selenium - Drag and Drop
- Selenium - Scroll Operations
- Selenium - Waiting Strategies
- Selenium - Explicit/Implicit Wait
- Selenium - Support Features
- Selenium - Multi Select
- Selenium - Wait Support
- Selenium - Select Support
- Selenium - Color Support
- Selenium - Errors & Logging
- Selenium - Logging
- Selenium - Exception Handling
- Selenium - Miscellaneous
- Selenium - Handling Ajax Calls
- Selenium - JSON Data File
- Selenium - CSV Data File
- Selenium - Excel Data File
- Selenium - Cross Browser Testing
- Selenium - Multi Browser Testing
- Selenium - Multi Windows Testing
- Selenium - JavaScript Executor
- Selenium - Headless Execution
- Selenium - Capture Screenshots
- Selenium - Capture Videos
- Selenium - Page Object Model
- Selenium - Page Factory
- Selenium - Record & Playback
- Selenium - Frameworks
- Selenium - Browsing Context
- Selenium Grid Tutorial
- Selenium - Overview
- Selenium - Architecture
- Selenium - Components
- Selenium - Configuration
- Selenium - Create Test Script
- Selenium - Test Execution
- Selenium - Endpoints
- Selenium - Customizing a Node
- Selenium Reporting Tools
- Selenium - Reporting Tools
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & other Technologies
- Selenium - Java Tutorial
- Selenium - Python Tutorial
- Selenium - C# Tutorial
- Selenium - Javascript Tutorial
- Selenium - Kotlin Tutorial
- Selenium - Ruby Tutorial
- Selenium - Maven & Jenkins
- Selenium - Database Testing
- Selenium - LogExpert Logging
- Selenium - Log4j Logging
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash Testing
- Selenium - Apache Ant
- Selenium - Github
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium Miscellaneous Concepts
- Selenium - Automation Frameworks
- Selenium - Keyword Driven Framework
- Selenium - Data Driven Framework
- Selenium - Hybrid Driven Framework
- Selenium - SSL Certificate Error
- Selenium - Alternatives
- Selenium Useful Resources
- Selenium - Quick Guide
- Selenium - Useful Resources
- Selenium - Automation Practice
- Selenium - Discussion
Selenium Webdriver - CSV Data File
Selenium Webdriver can be used to interact with the csv data file. Often in an automation test, there remains a need to feed a large amount of data through a csv file for a test case to verify a specific scenario or to create a data driven framework. A csv file looks similar to an excel file and it has an extension of .csv.
Java provides few classes and methods to carry out read and write data operations on a csv file using the OpenCSV libraries. The OpenCSV has two most important classes - CSVReader, and CSVWriter to perform read, and write operations on a csv file.
How to Install the OpenCSV?
Step 1 − Add the OpenCSV dependencies from the below link −
https://mvnrepository.com/artifact/com.opencsv/opencsv.
Step 2 − Save the pom.xml with all the dependencies and update the maven project.
Example 1 - Read all Values From a CSV
Let us take an example of the below csv named the Details1.csv file, where we will read the whole csv file and retrieve all its values using the CSVReader class and its method readNext().
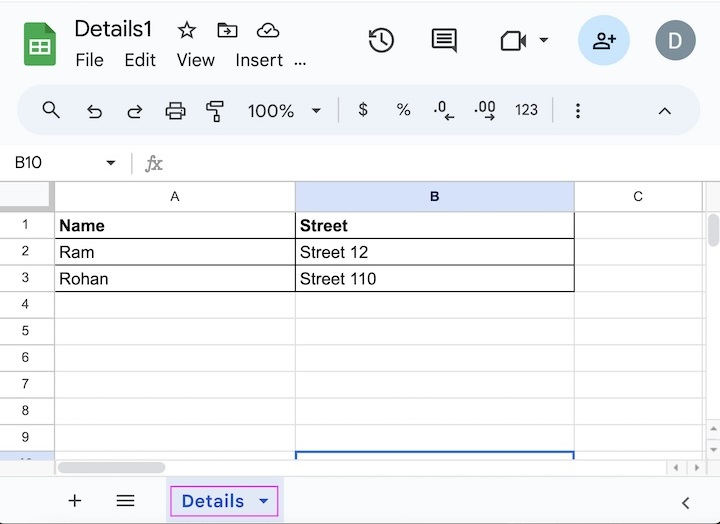
Please Note: The Details1.csv file was placed within the project under the Resources folder as shown in the below image.
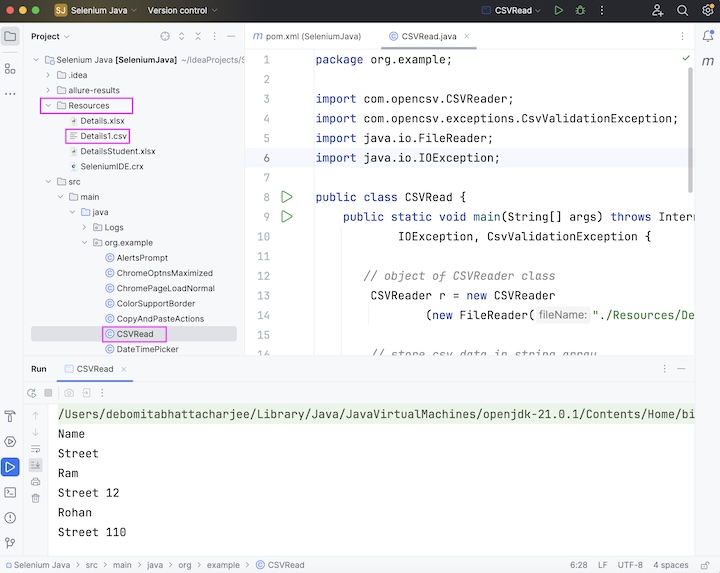
Code Implementation
package org.example; import com.opencsv.CSVReader; import com.opencsv.exceptions.CsvValidationException; import java.io.FileReader; import java.io.IOException; public class CSVRead { public static void main(String[] args) throws InterruptedException, IOException, CsvValidationException { // object of CSVReader class CSVReader r = new CSVReader(new FileReader("./Resources/Details1.CSV")); // store csv data in string array String [] csvValues; // iterate through csv till the end of the values while ((csvValues = r.readNext())!= null){ // iterate through rows for (String csvValue : csvValues){ System.out.println(csvValue); } } } }
Dependencies added to pom.xml.
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>SeleniumJava</artifactId> <version>1.0-SNAPSHOT</version> <properties> <maven.compiler.source>16</maven.compiler.source> <maven.compiler.target>16</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java --> <dependencies> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.11.0</version> </dependency> <!-- https://mvnrepository.com/artifact/com.opencsv/opencsv --> <dependency> <groupId>com.opencsv</groupId> <artifactId>opencsv</artifactId> <version>5.9</version> </dependency> </dependencies> </project>
Output
Name Street Ram Street 12 Rohan Street 110 Process finished with exit code 0
In the above example, we had read the whole csv file and obtained all its value in the console.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Example 2 - Write and read values in a csv
Let us take another example where we will create a csv file named Details2.csv under the Resources folder in the project and write some values using the CSVWriter class and its methods: writeNext() or writeAll(), and flush(). Finally, read those values using the CSVReader class and its method readNext().
package org.example; import com.opencsv.CSVReader; import com.opencsv.CSVWriter; import com.opencsv.exceptions.CsvValidationException; import java.io.FileReader; import java.io.FileWriter; import java.io.IOException; import java.util.ArrayList; import java.util.List; public class CSVReadWrite { public static void main(String[] args) throws InterruptedException, IOException, CsvValidationException { // object of CSVWriter class CSVWriter w = new CSVWriter (new FileWriter("./Resources/Details2.CSV")); // stores values in csv String [] rows1 = {"Name", "Street"}; String [] rows2 = {"Ram", "Street 12"}; String [] rows3 = {"Rohan", "Street 110"}; // add values to be written to list List<String[]> write = new ArrayList<>(); write.add(rows1); write.add(rows2); write.add(rows3); // write and flush all values w.writeAll(write); w.flush(); CSVReader r = new CSVReader (new FileReader("./Resources/Details2.CSV")); // store csv data in string array String [] csvValues; // iterate through csv till the end of the values while ((csvValues = r.readNext())!= null){ // iterate through rows for (String csvValue : csvValues){ System.out.println(csvValue); } } } }
Dependencies added to pom.xml.
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>SeleniumJava</artifactId> <version>1.0-SNAPSHOT</version> <properties> <maven.compiler.source>16</maven.compiler.source> <maven.compiler.target>16</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java --> <dependencies> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.11.0</version> </dependency> <!-- https://mvnrepository.com/artifact/com.opencsv/opencsv --> <dependency> <groupId>com.opencsv</groupId> <artifactId>opencsv</artifactId> <version>5.9</version> </dependency> </dependencies> </project>
Output
Name Street Ram Street 12 Rohan Street 110 Process finished with exit code 0
In the above example, we created a csv file Details2.csv under the Resources folder inside the project and wrote some values in it. Then we read all those values, and finally obtained them in the console.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Also, the csv with the filename Details2.csv got created in the project directory. On clicking it, we would get the values written through the above code.
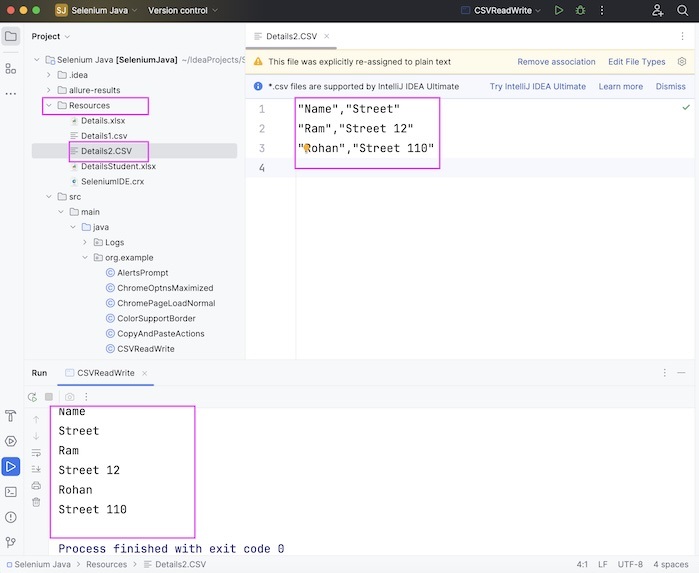
This concludes our comprehensive take on the tutorial on Selenium Webdriver - CSV Data File. We’ve started with describing what is an OpenCSV library, how to install OpenCSV, and walked through examples of how to read and write values in csv taking help of OpenCSV along with Selenium Webdriver. This equips you with in-depth knowledge of the CSV Data File in Selenium Webdriver. It is wise to keep practicing what you’ve learned and exploring others relevant to Selenium to deepen your understanding and expand your horizons.