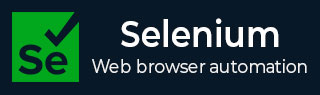
- Selenium Tutorial
- Selenium - Home
- Selenium - Overview
- Selenium - Components
- Selenium - Automation Testing
- Selenium - Environment Setup
- Selenium - Remote Control
- Selenium IDE Tutorial
- Selenium - IDE Introduction
- Selenium - Features
- Selenium - Limitations
- Selenium - Installation
- Selenium - Creating Tests
- Selenium - Creating Script
- Selenium - Store Variables
- Selenium - Alerts & Popups
- Selenium - Selenese Commands
- Selenium - Actions Commands
- Selenium - Accessors Commands
- Selenium - Assertions Commands
- Selenium - Assert/Verify Methods
- Selenium - Locating Strategies
- Selenium - Script Debugging
- Selenium - Verification Points
- Selenium - Pattern Matching
- Selenium - JSON Data File
- Selenium - Browser Execution
- Selenium - User Extensions
- Selenium - Code Export
- Selenium - JavaScript Functions
- Selenium - Plugins
- Selenium WebDriver Tutorial
- Selenium - Introduction
- Selenium WebDriver vs RC
- Selenium - Installation
- Selenium - First Test Script
- Selenium - Driver Sessions
- Selenium - Browser Options
- Selenium - Chrome Options
- Selenium - Edge Options
- Selenium - Firefox Options
- Selenium - Safari Options
- Selenium Web Elements
- Selenium - File Upload
- Selenium - Locator Strategies
- Selenium - Relative Locators
- Selenium - Finders
- Selenium - Find All Links
- Selenium - User Interactions
- Selenium - WebElement Commands
- Selenium - Browser Interactions
- Selenium - Browser Commands
- Selenium - Browser Navigation
- Selenium - Alerts & Popups
- Selenium - Handling Forms
- Selenium - Windows and Tabs
- Selenium - Handling Links
- Selenium - Input Boxes
- Selenium - Radio Button
- Selenium - Checkboxes
- Selenium - Dropdown Box
- Selenium - Handling IFrames
- Selenium - Handling Cookies
- Selenium - Date Time Picker
- Selenium - Dynamic Web Tables
- Selenium - Actions Class
- Selenium - Action Class
- Selenium - Keyboard Events
- Selenium - Key Up/Down
- Selenium - Copy and Paste
- Selenium - Handle Special Keys
- Selenium - Mouse Events
- Selenium - Drag and Drop
- Selenium - Scroll Operations
- Selenium - Waiting Strategies
- Selenium - Explicit/Implicit Wait
- Selenium - Support Features
- Selenium - Multi Select
- Selenium - Wait Support
- Selenium - Select Support
- Selenium - Color Support
- Selenium - Errors & Logging
- Selenium - Logging
- Selenium - Exception Handling
- Selenium - Miscellaneous
- Selenium - Handling Ajax Calls
- Selenium - JSON Data File
- Selenium - CSV Data File
- Selenium - Excel Data File
- Selenium - Cross Browser Testing
- Selenium - Multi Browser Testing
- Selenium - Multi Windows Testing
- Selenium - JavaScript Executor
- Selenium - Headless Execution
- Selenium - Capture Screenshots
- Selenium - Capture Videos
- Selenium - Page Object Model
- Selenium - Page Factory
- Selenium - Record & Playback
- Selenium - Frameworks
- Selenium - Browsing Context
- Selenium Grid Tutorial
- Selenium - Overview
- Selenium - Architecture
- Selenium - Components
- Selenium - Configuration
- Selenium - Create Test Script
- Selenium - Test Execution
- Selenium - Endpoints
- Selenium - Customizing a Node
- Selenium Reporting Tools
- Selenium - Reporting Tools
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & other Technologies
- Selenium - Java Tutorial
- Selenium - Python Tutorial
- Selenium - C# Tutorial
- Selenium - Javascript Tutorial
- Selenium - Kotlin Tutorial
- Selenium - Ruby  Tutorial
- Selenium - Maven & Jenkins
- Selenium - Database Testing
- Selenium - LogExpert Logging
- Selenium - Log4j Logging
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash Testing
- Selenium - Apache Ant
- Selenium - Github
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium Miscellaneous Concepts
- Selenium - Automation Frameworks
- Selenium - Keyword Driven Framework
- Selenium - Data Driven Framework
- Selenium - Hybrid Driven Framework
- Selenium - SSL Certificate Error
- Selenium - Alternatives
- Selenium Useful Resources
- Selenium - Quick Guide
- Selenium - Useful Resources
- Selenium - Automation Practice
- Selenium - Discussion
Selenium WebDriver - Safari Options
Selenium Webdriver can be used to run automated tests on the Safari browser. There are certain functionalities and characteristics which are applicable to only Safari for Apple users. Safari is a prominent browser and is provided by default by Apple devices. For Safari browser versions 10 and greater than 10, the safaridriver comes automatically and is not required to be installed separately.
The safari driver is installed on the operating system by default which is not the case for Firefox and Chromium browsers. We would need to do initial setups - enable Develop menu in the Safari browser before actually running the tests on it. By default, the Develop menu does not remain visible.
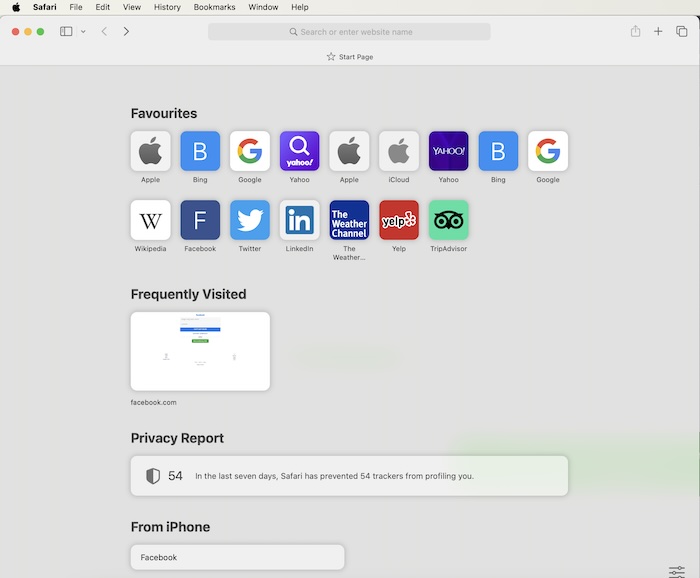
To do so, we have to open Safari, then click on Settings. Next, we would need to move to the Advanced tab, and check the option Show Features for web developers.
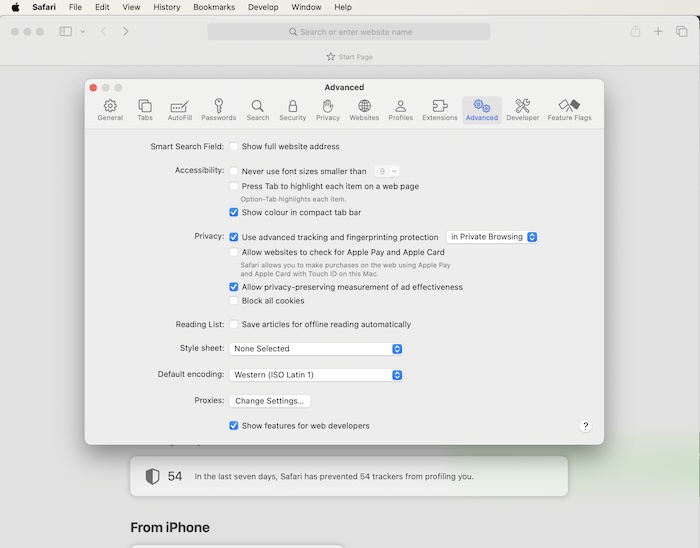
To start running automation tests on Safari browser, we would need to run the command from the terminal −
safaridriver --enable
Let us take an example of the page below, where we initiate a Safari driver session along the SafariOptions. Then we would obtain the browser title - Selenium Practice - Student Registration Form and current URL - https://www.tutorialspoint.com/selenium/.
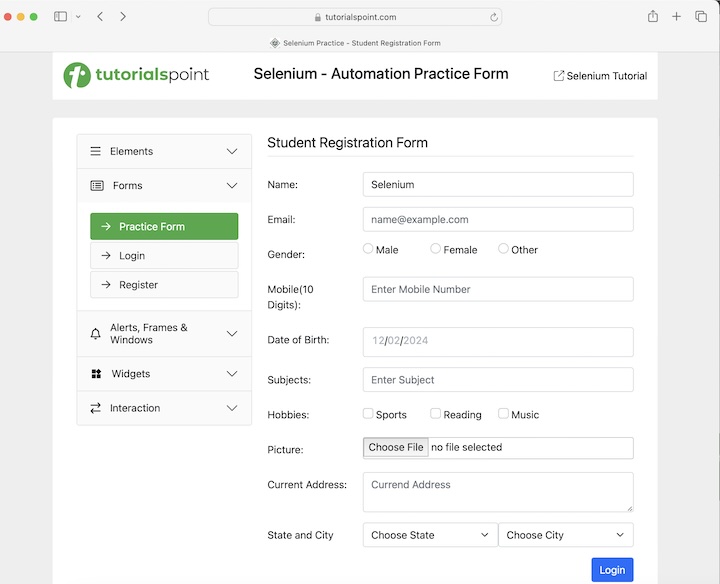
To use SafariOptions class along with SafariDriver, we would need to add the import statements: import org.openqa.selenium.safari.SafariOptions and import org.openqa.selenium.safari.SafariDriver.
Syntax
// Using the Safari options SafariOptions opts = new SafariOptions(); WebDriver driver = new SafariDriver(opts); // opening the Safari browser and launch a URL driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // get the page title System.out.println("Browser title is: " + driver.getTitle()); // get the current URL System.out.println("Current URL is: " + driver.getCurrentUrl());
Example
Code Implementation on SafariOpts.java class file.
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.safari.SafariDriver; import org.openqa.selenium.safari.SafariOptions; import java.util.concurrent.TimeUnit; public class SafariOpts { public static void main(String[] args) throws InterruptedException { // Using the Safari options SafariOptions opts = new SafariOptions(); WebDriver driver = new SafariDriver(opts); // adding an implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // opening the Safari browser and launch a URL driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // get the page title System.out.println("Browser title is: " + driver.getTitle()); // get the current URL System.out.println("Current URL is: " + driver.getCurrentUrl()); // closing the browser driver.quit(); } }
Output
Browser title is: Selenium Practice - Student Registration Form Current URL is: https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php Process finished with exit code 0
In the above example, we had launched the Safari browser with SafariOptions then obtained browser title and current URL with the message in the console - Browser title is: Selenium Practice − Student Registration Form and Current URL is: https://www.tutorialspoint.com/selenium/ respectively.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Safari browsers would always store the logs(if enabled) in the location − ~/Library/Logs/com.apple.WebDriver/. Unlike other browsers like Chrome, Firefox, and so on, we would not get the option to save the logs and its levels to other locations and values respectively.
Let us take an example, where we would enable the Safari Logs with the below implementation.
Syntax
Syntax to enable logs −
// Using the Safari options SafariOptions opts = new SafariOptions(); WebDriver driver = new SafariDriver(opts); // adding an implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // opening the Safari browser and launch a URL driver.get("https://www.tutorialspoint.com/index.htm"); // to the enable logs SafariDriverService service = new SafariDriverService.Builder() .withLogging(true) .build();
Example
Code Implementation on SafariOptsLogs.java class file.
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.safari.SafariDriver; import org.openqa.selenium.safari.SafariDriverService; import org.openqa.selenium.safari.SafariOptions; import java.util.concurrent.TimeUnit; public class SafariOptsLogs { public static void main(String[] args) throws InterruptedException { // Using the Safari options SafariOptions opts = new SafariOptions(); WebDriver driver = new SafariDriver(opts); // adding an implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // opening the Safari browser and launch a URL driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // enabling the Safari logs SafariDriverService service = new SafariDriverService.Builder().withLogging(true).build(); // get the page title System.out.println("Logging enabled: " + service); // close the browser driver.quit(); } }
Output
Logging enabled: org.openqa.selenium.safari.SafariDriverService@3eeb318f Process finished with exit code 0
Let us take an example, where we would disable the Safari Logs with the below implementation.
Syntax
Syntax to disable logs −
// Using the Safari options SafariOptions opts = new SafariOptions(); WebDriver driver = new SafariDriver(opts); // adding an implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // opening the Safari browser and launch a URL driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // to disable the logs to false SafariDriverService service = new SafariDriverService.Builder() .withLogging(false) .build();
Example
Code Implementation on SafariOptsLogsFalse.java class file.
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.safari.SafariDriver; import org.openqa.selenium.safari.SafariDriverService; import org.openqa.selenium.safari.SafariOptions; import java.util.concurrent.TimeUnit; public class SafariOptsLogsFalse { public static void main(String[] args) throws InterruptedException { // Using the Safari options SafariOptions opts = new SafariOptions(); WebDriver driver = new SafariDriver(opts); // adding an implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // opening the Safari browser and launch a URL driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // disabling the Safari Logs SafariDriverService service = new SafariDriverService.Builder() .withLogging(false) .build(); // get the page title System.out.println("Launched Browser title is: " + driver.getTitle()); // get the current URL System.out.println("Current URL is: " + driver.getCurrentUrl()); // close the browser driver.quit(); } }
Output
Browser title is: Selenium Practice - Student Registration Form Current URL is: https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php Process finished with exit code 0
In this tutorial, we had discussed how to handle Safari Options with the help of various examples along with the Selenium Webdriver.