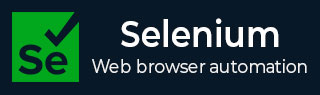
- Selenium Tutorial
- Selenium - Home
- Selenium - Overview
- Selenium - Components
- Selenium - Automation Testing
- Selenium - Environment Setup
- Selenium - Remote Control
- Selenium IDE Tutorial
- Selenium - IDE Introduction
- Selenium - Features
- Selenium - Limitations
- Selenium - Installation
- Selenium - Creating Tests
- Selenium - Creating Script
- Selenium - Store Variables
- Selenium - Alerts & Popups
- Selenium - Selenese Commands
- Selenium - Actions Commands
- Selenium - Accessors Commands
- Selenium - Assertions Commands
- Selenium - Assert/Verify Methods
- Selenium - Locating Strategies
- Selenium - Script Debugging
- Selenium - Verification Points
- Selenium - Pattern Matching
- Selenium - JSON Data File
- Selenium - Browser Execution
- Selenium - User Extensions
- Selenium - Code Export
- Selenium - JavaScript Functions
- Selenium - Plugins
- Selenium WebDriver Tutorial
- Selenium - Introduction
- Selenium WebDriver vs RC
- Selenium - Installation
- Selenium - First Test Script
- Selenium - Driver Sessions
- Selenium - Browser Options
- Selenium - Chrome Options
- Selenium - Edge Options
- Selenium - Firefox Options
- Selenium - Safari Options
- Selenium Web Elements
- Selenium - File Upload
- Selenium - Locator Strategies
- Selenium - Relative Locators
- Selenium - Finders
- Selenium - Find All Links
- Selenium - User Interactions
- Selenium - WebElement Commands
- Selenium - Browser Interactions
- Selenium - Browser Commands
- Selenium - Browser Navigation
- Selenium - Alerts & Popups
- Selenium - Handling Forms
- Selenium - Windows and Tabs
- Selenium - Handling Links
- Selenium - Input Boxes
- Selenium - Radio Button
- Selenium - Checkboxes
- Selenium - Dropdown Box
- Selenium - Handling IFrames
- Selenium - Handling Cookies
- Selenium - Date Time Picker
- Selenium - Dynamic Web Tables
- Selenium - Actions Class
- Selenium - Action Class
- Selenium - Keyboard Events
- Selenium - Key Up/Down
- Selenium - Copy and Paste
- Selenium - Handle Special Keys
- Selenium - Mouse Events
- Selenium - Drag and Drop
- Selenium - Scroll Operations
- Selenium - Waiting Strategies
- Selenium - Explicit/Implicit Wait
- Selenium - Support Features
- Selenium - Multi Select
- Selenium - Wait Support
- Selenium - Select Support
- Selenium - Color Support
- Selenium - Errors & Logging
- Selenium - Logging
- Selenium - Exception Handling
- Selenium - Miscellaneous
- Selenium - Handling Ajax Calls
- Selenium - JSON Data File
- Selenium - CSV Data File
- Selenium - Excel Data File
- Selenium - Cross Browser Testing
- Selenium - Multi Browser Testing
- Selenium - Multi Windows Testing
- Selenium - JavaScript Executor
- Selenium - Headless Execution
- Selenium - Capture Screenshots
- Selenium - Capture Videos
- Selenium - Page Object Model
- Selenium - Page Factory
- Selenium - Record & Playback
- Selenium - Frameworks
- Selenium - Browsing Context
- Selenium Grid Tutorial
- Selenium - Overview
- Selenium - Architecture
- Selenium - Components
- Selenium - Configuration
- Selenium - Create Test Script
- Selenium - Test Execution
- Selenium - Endpoints
- Selenium - Customizing a Node
- Selenium Reporting Tools
- Selenium - Reporting Tools
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & other Technologies
- Selenium - Java Tutorial
- Selenium - Python Tutorial
- Selenium - C# Tutorial
- Selenium - Javascript Tutorial
- Selenium - Kotlin Tutorial
- Selenium - Ruby Tutorial
- Selenium - Maven & Jenkins
- Selenium - Database Testing
- Selenium - LogExpert Logging
- Selenium - Log4j Logging
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash Testing
- Selenium - Apache Ant
- Selenium - Github
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium Miscellaneous Concepts
- Selenium - Automation Frameworks
- Selenium - Keyword Driven Framework
- Selenium - Data Driven Framework
- Selenium - Hybrid Driven Framework
- Selenium - SSL Certificate Error
- Selenium - Alternatives
- Selenium Useful Resources
- Selenium - Quick Guide
- Selenium - Useful Resources
- Selenium - Automation Practice
- Selenium - Discussion
Selenium Webdriver - Date Time Picker
Selenium Webdriver can be used to select date and time from a calendar. A Date Time Picker in a calendar can be designed in numerous ways on the web UI. Based on the UI, the test case needs to be designed.
What is a Date Time Picker?
The Date Time Picker is basically a user interface to create a calendar in the application under test. The functionality of a Date time picker is to select a particular date and/or time from a range.
How to Inspect a Date Time Picker?
To identify a calendar shown in the below image first we would need to click on the first input box, and then right click on the web page, and finally click on the Inspect button in the Chrome browser. Then, the corresponding HTML code for the whole page would be visible. For investigating a single element on a page, we would need to click on the left upward arrow, available to the top of the visible HTML code as highlighted below.
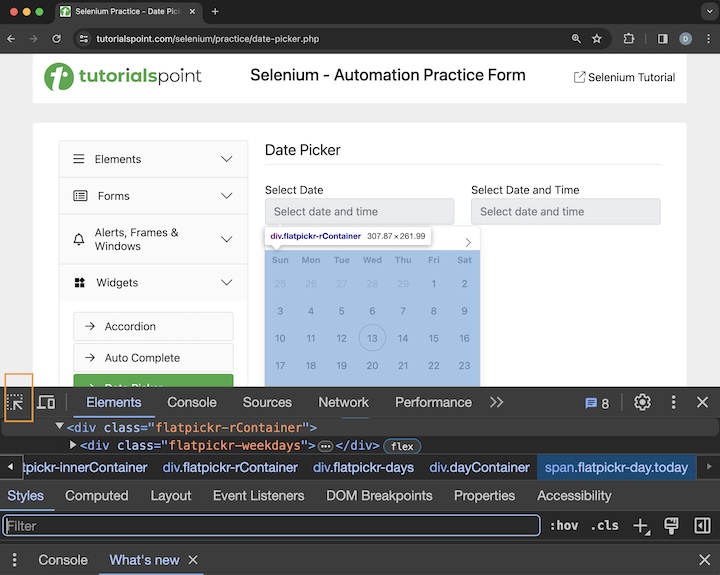
Example 1 - Past Date Selection
Let us take the example of the Date Time Picker in the page below having the option to select year, month, day, hour, minutes, and so on. It also contains the option to select backdated or future date and time as well. Let's select a back dated date and time - 2023-06-04 09:05 in the morning, and then verify them.
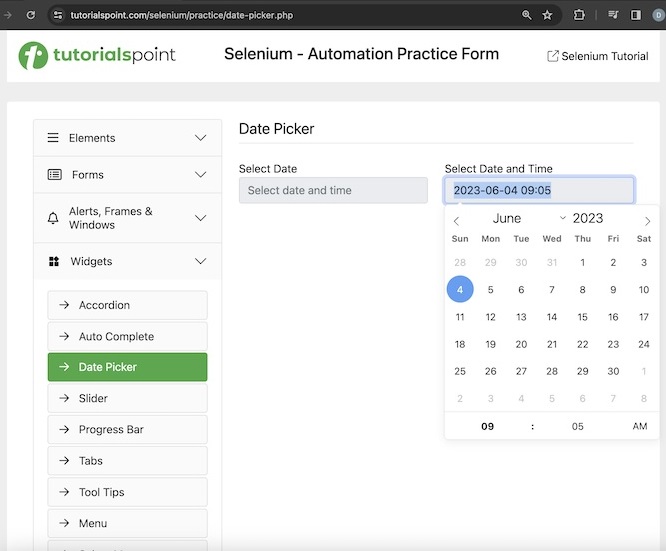
Code Implementation
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.Select; import java.util.concurrent.TimeUnit; public class DatTimePickers { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 20 secs driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS); // URL launch for accessing calendar driver.get("https://www.tutorialspoint.com/selenium/practice/date-picker.php"); // identify element to get calendar WebElement f = driver.findElement (By.xpath("//*[@id='datetimepicker2']")); f.click(); // selecting month June WebElement month = driver.findElement (By.xpath("/html/body/div[3]/div[1]/div/div/select")); Select select = new Select(month); select.selectByVisibleText("June"); // getting selected month String selectedMonth = select.getFirstSelectedOption().getText(); // selecting year 2023 WebElement year = driver.findElement (By.xpath("/html/body/div[3]/div[1]/div/div/div/input")); // removing existing year then entering year.clear(); year.sendKeys("2023"); // selecting day 4 WebElement day = driver.findElement (By.xpath("//span[contains(@aria-label,'"+selectedMonth+" 4')]")); day.click(); // selecting AM time WebElement time = driver.findElement (By.xpath("/html/body/div[3]/div[3]/span[2]")); if (time.getText().equalsIgnoreCase("PM")){ time.click(); } // selecting hour WebElement hour = driver.findElement (By.xpath("/html/body/div[3]/div[3]/div[1]/input")); // removing existing hour then entering hour.clear(); hour.sendKeys("9"); // selecting minutes WebElement minutes = driver.findElement (By.xpath("/html/body/div[3]/div[3]/div[2]/input")); // removing existing minutes then entering minutes.clear(); minutes.sendKeys("5"); // reflecting both date and time f.click(); // get date and time selected String v = f.getAttribute("value"); System.out.println("Date and time selected by Date Time Picker: " + v); // check date and time selected if (v.equalsIgnoreCase("2023-06-04 09:05")){ System.out.print("Date and Time selected successfully"); } else { System.out.print("Date and Time selected unsuccessfully"); } // close browser driver.quit(); } }
Output
Date and time selected by Date Time Picker: 2023-06-04 09:05 Date and Time selected successfully Process finished with exit code 0
In the above example, we had selected the back dated date and time from the calendar using a Date Time Picker, and then verified if the proper date is selected in the console with the messages - Date and time selected by Date Time Picker: 2023-06-04 09:05 and Date and Time selected successfully.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Example 2 - Future Date Selection
Let us take another example of the Date Time Picker in the below page we would select a future dated date and time - 2025-06-04 21:05 in the evening, and then verify them.
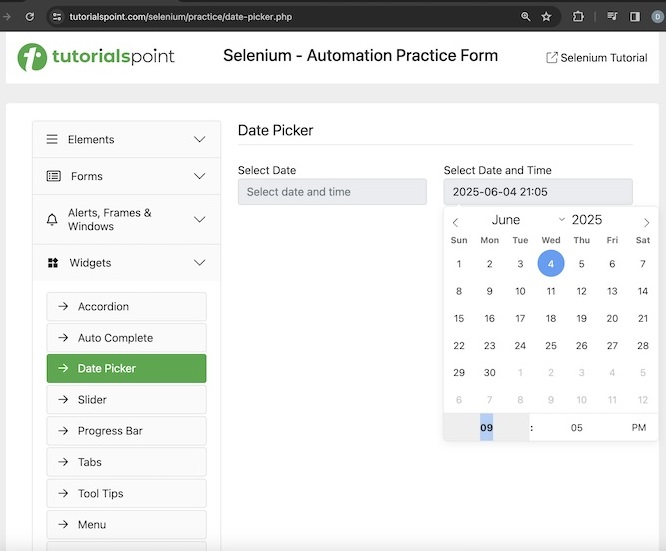
Code Implementation
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.Select; import java.util.concurrent.TimeUnit; public class DatTimePickersForward { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 20 secs driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS); // URL launch for accessing calendar driver.get("https://www.tutorialspoint.com/selenium/practice/date-picker.php"); // identify element to get calendar WebElement f = driver.findElement (By.xpath("//*[@id='datetimepicker2']")); f.click(); // selecting month June WebElement month = driver.findElement (By.xpath("/html/body/div[3]/div[1]/div/div/select")); Select select = new Select(month); select.selectByVisibleText("June"); // getting selected month String selectedMonth = select.getFirstSelectedOption().getText(); // selecting year 2023 WebElement year = driver.findElement (By.xpath("/html/body/div[3]/div[1]/div/div/div/input")); // removing existing year then entering year.clear(); year.sendKeys("2025"); // selecting day 4 WebElement day = driver.findElement (By.xpath("//span[contains(@aria-label,'"+selectedMonth+" 4')]")); day.click(); // selecting PM time WebElement time = driver.findElement (By.xpath("/html/body/div[3]/div[3]/span[2]")); if (time.getText().equalsIgnoreCase("AM")){ time.click(); } // selecting hour WebElement hour = driver.findElement (By.xpath("/html/body/div[3]/div[3]/div[1]/input")); // removing existing hour then entering hour.clear(); hour.sendKeys("9"); // selecting minutes WebElement minutes = driver.findElement (By.xpath("/html/body/div[3]/div[3]/div[2]/input")); // removing existing minutes then entering minutes.clear(); minutes.sendKeys("5"); // reflecting both date and time f.click(); // get date and time selected String v = f.getAttribute("value"); System.out.println("Date and time selected by Date Time Picker: " + v); // check date and time selected if (v.equalsIgnoreCase("2025-06-04 21:05")){ System.out.print("Date and Time selected successfully"); } else { System.out.print("Date and Time selected unsuccessfully"); } // close browser driver.quit(); } }
Output
Date and time selected by Date Time Picker: 2025-06-04 21:05 Date and Time selected successfully Process finished with exit code 0
In the above example, we had selected the future dated date and time from the calendar using a Date Time Picker, and then verified if the proper date is selected in the console with the messages - Date and time selected by Date Time Picker: 2025-06-04 21:05 and Date and Time selected successfully.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Example 3 - Current Date Selection
Let us take another example of the Date Time Picker in the below page we would select a current dated date and time and then verify them. Please note, in this Date Time Picker, the current date is selected by default, we would need to only select the current time.
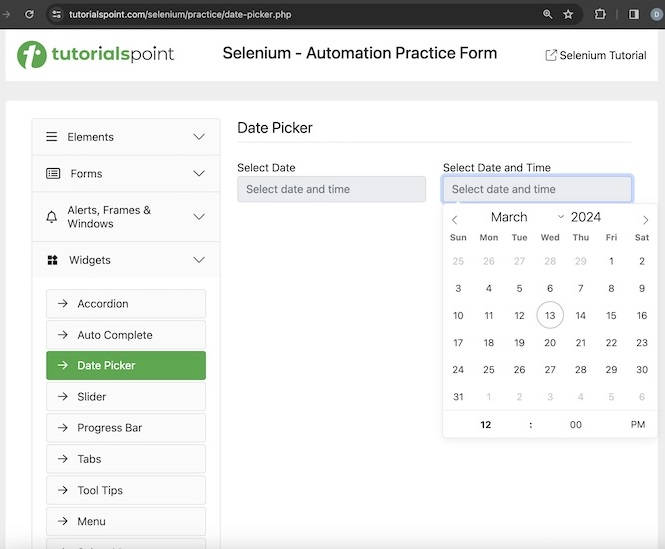
Code Implementation
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.time.LocalDateTime; import java.time.format.DateTimeFormatter; import java.util.concurrent.TimeUnit; public class DateTimePickersCurrent { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 20 secs driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS); // URL launch for accessing calendar driver.get("https://www.tutorialspoint.com/selenium/practice/date-picker.php"); // get current time LocalDateTime l = LocalDateTime.now(); DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm"); String dateTime = l.format(formatter); String[] dtTime = dateTime.split(" "); String time = dtTime[1]; String[] t = time.split(":"); String hour = t[0]; String minute = t[1]; // identify element to get calendar WebElement f = driver.findElement (By.xpath("//*[@id='datetimepicker2']")); f.click(); // selecting hour based on current WebElement hours = driver.findElement (By.xpath("/html/body/div[3]/div[3]/div[1]/input")); // removing existing hour then entering hours.clear(); hours.sendKeys(hour); // selecting minutes based on current minutes WebElement minutes = driver.findElement (By.xpath("/html/body/div[3]/div[3]/div[2]/input")); // removing existing minutes then entering minutes.clear(); minutes.sendKeys(minute); // reflecting both date and time f.click(); // get date and time selected String v = f.getAttribute("value"); System.out.println("Date and time selected by Date Time Picker: " + v); // check date and time selected if (v.equalsIgnoreCase(dateTime)){ System.out.print("Date and Time selected successfully"); } else { System.out.print("Date and Time selected unsuccessfully"); } // close browser driver.quit(); } }
Output
Date and time selected by Date Time Picker: 2024-03-13 13:29 Date and Time selected successfully Process finished with exit code 0
In the above example, we had selected the current date and time from the calendar using a Date Time Picker, and then verified if the proper date is selected in the console with the messages - Date and time selected by Date Time Picker: 2024-03-13 13:29 and Date and Time selected successfully.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
This concludes our comprehensive take on the tutorial on Selenium Webdriver - Date Time Picker. We’ve started with describing what is a Date Time Picker, how to inspect it and examples to walk through how to select past, current, and present date and time with Selenium. This equips you with in-depth knowledge of the Date Time Picker in Selenium. It is wise to keep practicing what you’ve learned and exploring others relevant to Selenium to deepen your understanding and expand your horizons.