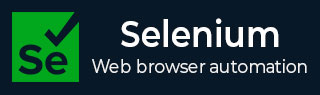
- Selenium Tutorial
- Selenium - Home
- Selenium - Overview
- Selenium - Components
- Selenium - Automation Testing
- Selenium - Environment Setup
- Selenium - Remote Control
- Selenium IDE Tutorial
- Selenium - IDE Introduction
- Selenium - Features
- Selenium - Limitations
- Selenium - Installation
- Selenium - Creating Tests
- Selenium - Creating Script
- Selenium - Store Variables
- Selenium - Alerts & Popups
- Selenium - Selenese Commands
- Selenium - Actions Commands
- Selenium - Accessors Commands
- Selenium - Assertions Commands
- Selenium - Assert/Verify Methods
- Selenium - Locating Strategies
- Selenium - Script Debugging
- Selenium - Verification Points
- Selenium - Pattern Matching
- Selenium - JSON Data File
- Selenium - Browser Execution
- Selenium - User Extensions
- Selenium - Code Export
- Selenium - JavaScript Functions
- Selenium - Plugins
- Selenium WebDriver Tutorial
- Selenium - Introduction
- Selenium WebDriver vs RC
- Selenium - Installation
- Selenium - First Test Script
- Selenium - Driver Sessions
- Selenium - Browser Options
- Selenium - Chrome Options
- Selenium - Edge Options
- Selenium - Firefox Options
- Selenium - Safari Options
- Selenium Web Elements
- Selenium - File Upload
- Selenium - Locator Strategies
- Selenium - Relative Locators
- Selenium - Finders
- Selenium - Find All Links
- Selenium - User Interactions
- Selenium - WebElement Commands
- Selenium - Browser Interactions
- Selenium - Browser Commands
- Selenium - Browser Navigation
- Selenium - Alerts & Popups
- Selenium - Handling Forms
- Selenium - Windows and Tabs
- Selenium - Handling Links
- Selenium - Input Boxes
- Selenium - Radio Button
- Selenium - Checkboxes
- Selenium - Dropdown Box
- Selenium - Handling IFrames
- Selenium - Handling Cookies
- Selenium - Date Time Picker
- Selenium - Dynamic Web Tables
- Selenium - Actions Class
- Selenium - Action Class
- Selenium - Keyboard Events
- Selenium - Key Up/Down
- Selenium - Copy and Paste
- Selenium - Handle Special Keys
- Selenium - Mouse Events
- Selenium - Drag and Drop
- Selenium - Scroll Operations
- Selenium - Waiting Strategies
- Selenium - Explicit/Implicit Wait
- Selenium - Support Features
- Selenium - Multi Select
- Selenium - Wait Support
- Selenium - Select Support
- Selenium - Color Support
- Selenium - Errors & Logging
- Selenium - Logging
- Selenium - Exception Handling
- Selenium - Miscellaneous
- Selenium - Handling Ajax Calls
- Selenium - JSON Data File
- Selenium - CSV Data File
- Selenium - Excel Data File
- Selenium - Cross Browser Testing
- Selenium - Multi Browser Testing
- Selenium - Multi Windows Testing
- Selenium - JavaScript Executor
- Selenium - Headless Execution
- Selenium - Capture Screenshots
- Selenium - Capture Videos
- Selenium - Page Object Model
- Selenium - Page Factory
- Selenium - Record & Playback
- Selenium - Frameworks
- Selenium - Browsing Context
- Selenium Grid Tutorial
- Selenium - Overview
- Selenium - Architecture
- Selenium - Components
- Selenium - Configuration
- Selenium - Create Test Script
- Selenium - Test Execution
- Selenium - Endpoints
- Selenium - Customizing a Node
- Selenium Reporting Tools
- Selenium - Reporting Tools
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & other Technologies
- Selenium - Java Tutorial
- Selenium - Python Tutorial
- Selenium - C# Tutorial
- Selenium - Javascript Tutorial
- Selenium - Kotlin Tutorial
- Selenium - Ruby Tutorial
- Selenium - Maven & Jenkins
- Selenium - Database Testing
- Selenium - LogExpert Logging
- Selenium - Log4j Logging
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash Testing
- Selenium - Apache Ant
- Selenium - Github
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium Miscellaneous Concepts
- Selenium - Automation Frameworks
- Selenium - Keyword Driven Framework
- Selenium - Data Driven Framework
- Selenium - Hybrid Driven Framework
- Selenium - SSL Certificate Error
- Selenium - Alternatives
- Selenium Useful Resources
- Selenium - Quick Guide
- Selenium - Useful Resources
- Selenium - Automation Practice
- Selenium - Discussion
Selenium Webdriver - Handle Special Keys
Selenium Webdriver can be used to handle special keys while creating the automation tests. This is done using the Actions class and sendKeys() method in Selenium. The operations like key up/down using the keyUp() and keyDown() methods are mostly used to work with special keys. In case we are using sendKeys() method we would need to pass Key.chord as a parameter to this method.
Please note that, while using the methods of Actions class, we would need to add the below import statement in our test −
import org.openqa.selenium.interactions.Actions.
Copy and Paste Example With Actions Class
Let us now discuss the identification of elements where the copy and paste operations are to be performed on a web page as shown in the below image. First, we would need to right click on the web page, and then click on the Inspect button in the Chrome browser. Then, the corresponding HTML code for the whole page would be visible. For investigating both the source and destination elements on that web page, we would need to click on the left upward arrow, available to the top of the visible HTML code as highlighted below.
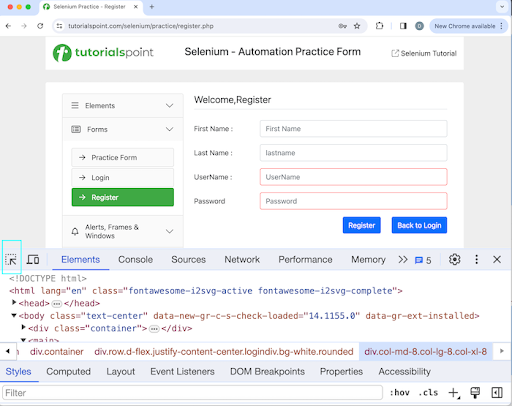
Let us take an example on the below page, where we would first enter the text - Selenium beside the Full Name: in the first input box(highlighted) then copy and paste the same text in another input box (highlighted) beside the Last Name:.
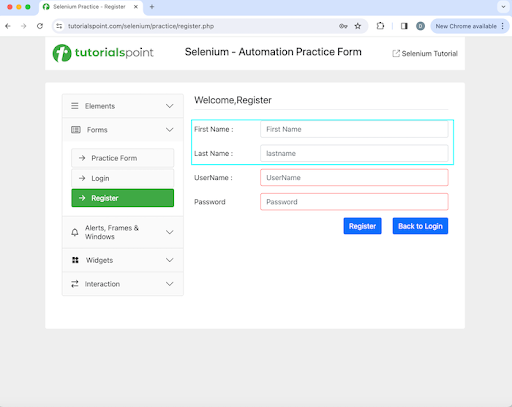
Example
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.interactions.Actions; import java.util.concurrent.TimeUnit; public class CopyPasteAction { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify an element driver.get("https://www.tutorialspoint.com/selenium/practice/register.php"); // Identify the first input box with xpath locator WebElement e = driver.findElement(By.xpath("//*[@id='firstname']")); // enter some text e.sendKeys("Selenium"); // chose the key as per platform Keys k = Platform.getCurrent().is(Platform.MAC) ? Keys.COMMAND : Keys.CONTROL; // object of Actions class to copy then paste Actions a = new Actions(driver); a.keyDown(k); a.sendKeys("a"); a.keyUp(k); a.build().perform(); // Actions class methods to copy text a.keyDown(k); a.sendKeys("c"); a.keyUp(k); a.build().perform(); // Action class methods to tab and reach to next input box a.sendKeys(Keys.TAB); a.build().perform(); // Actions class methods to paste text a.keyDown(k); a.sendKeys("v"); a.keyUp(k); a.build().perform(); // Identify the second input box with xpath locator WebElement s = driver.findElement(By.xpath("//*[@id='lastname']")); // Getting text in the second input box String text = s.getAttribute("value"); System.out.println("Value copied and pasted: " + text); // Closing browser driver.quit(); } }
Output
Value copied and pasted: Selenium Process finished with exit code 0
In the above example, we had first entered the text Selenium in the first input box and then copied and pasted the same text in the second input box using the methods of the Actions class. Finally, we had obtained the entered text in the second input box as a message in the console - Value copied and pasted: Selenium.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Copy and Paste Example Without Actions Class
Let us take the same above example, and implement the same without using the Actions class.
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class CopyPaste { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify an element driver.get("https://www.tutorialspoint.com/selenium/practice/register.php"); // Identify the first input box with xpath locator WebElement e = driver.findElement(By.xpath("//*[@id='firstname']")); // Identify the second input box with xpath locator WebElement s = driver.findElement(By.xpath("//*[@id='lastname']")); // chose the key as per platform Keys k = Platform.getCurrent().is(Platform.MAC) ? Keys.COMMAND : Keys.CONTROL; // enter some text e.sendKeys("Selenium"); // select the whole entered text e.sendKeys(Keys.chord(k, "a")); // copy the whole entered text e.sendKeys(Keys.chord(k, "c")); // tab and reach to next input box e.sendKeys(Keys.TAB); // paste the whole entered text s.sendKeys(Keys.chord(k, "v")); // Getting text in the second input box String text = s.getAttribute("value"); System.out.println("Value copied and pasted: " + text); // Closing browser driver.quit(); } }
Output
Value copied and pasted: Selenium Process finished with exit code 0
In the above example, we had first entered the text Selenium in the first input box and then copied and pasted the same text in the second input box using the sendKeys() and Key.chord() methods. Finally, we had obtained the entered text in the second input box as a message in the console - Value copied and pasted: Selenium.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Input Text in Upper Case
Let us take another example, where we would enter text SELENIUM in an input box in capital letters using the Key Up Key Down features of Actions class. Please note that, while sending the value to the sendKeys() method, we would pass selenium along with pressing SHIFT keys. Hence, we would get the output entered in the input box as SELENIUM.
Example
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.Keys; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.interactions.Actions; import java.util.concurrent.TimeUnit; public class KeyAction { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify an element driver.get("https://www.tutorialspoint.com/selenium/practice/text-box.php"); // Identify the first input box with xpath locator WebElement e = driver.findElement(By.xpath("//*[@id='fullname']")); // Actions class Actions a = new Actions(driver); // moving to an input box and clicking on it a.moveToElement(e).click(); // key UP and DOWN action for SHIFT a.keyDown(Keys.SHIFT); a.sendKeys("Selenium").keyUp(Keys.SHIFT).build().perform(); // get value entered System.out.println("Text entered: " + e.getAttribute("value")); // Closing browser driver.quit(); } }
Output
Text entered: SELENIUM Process finished with exit code 0
In the above example, we had entered the text selenium along with pressing SHIFT key in the input box and also obtained the entered text in upper case with the message in the console - Text entered: SELENIUM.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
This concludes our comprehensive take on the tutorial on Selenium Webdriver- Handle Special Keys. We’ve started with describing an example of copying and pasting text taking help of special keys like CONTROL, SHIFT, TAB, CONTROL + A, CONTROL + V, CONTROL + C, and so on along with and without the Actions class, and illustrating how to input text in upper case with Selenium. This equips you with in-depth knowledge of handling special keys in Selenium Webdriver. It is wise to keep practicing what you’ve learned and exploring others relevant to Selenium to deepen your understanding and expand your horizons.