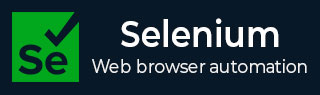
- Selenium Tutorial
- Selenium - Home
- Selenium - Overview
- Selenium - Components
- Selenium - Automation Testing
- Selenium - Environment Setup
- Selenium - Remote Control
- Selenium IDE Tutorial
- Selenium - IDE Introduction
- Selenium - Features
- Selenium - Limitations
- Selenium - Installation
- Selenium - Creating Tests
- Selenium - Creating Script
- Selenium - Store Variables
- Selenium - Alerts & Popups
- Selenium - Selenese Commands
- Selenium - Actions Commands
- Selenium - Accessors Commands
- Selenium - Assertions Commands
- Selenium - Assert/Verify Methods
- Selenium - Locating Strategies
- Selenium - Script Debugging
- Selenium - Verification Points
- Selenium - Pattern Matching
- Selenium - JSON Data File
- Selenium - Browser Execution
- Selenium - User Extensions
- Selenium - Code Export
- Selenium - JavaScript Functions
- Selenium - Plugins
- Selenium WebDriver Tutorial
- Selenium - Introduction
- Selenium WebDriver vs RC
- Selenium - Installation
- Selenium - First Test Script
- Selenium - Driver Sessions
- Selenium - Browser Options
- Selenium - Chrome Options
- Selenium - Edge Options
- Selenium - Firefox Options
- Selenium - Safari Options
- Selenium Web Elements
- Selenium - File Upload
- Selenium - Locator Strategies
- Selenium - Relative Locators
- Selenium - Finders
- Selenium - Find All Links
- Selenium - User Interactions
- Selenium - WebElement Commands
- Selenium - Browser Interactions
- Selenium - Browser Commands
- Selenium - Browser Navigation
- Selenium - Alerts & Popups
- Selenium - Handling Forms
- Selenium - Windows and Tabs
- Selenium - Handling Links
- Selenium - Input Boxes
- Selenium - Radio Button
- Selenium - Checkboxes
- Selenium - Dropdown Box
- Selenium - Handling IFrames
- Selenium - Handling Cookies
- Selenium - Date Time Picker
- Selenium - Dynamic Web Tables
- Selenium - Actions Class
- Selenium - Action Class
- Selenium - Keyboard Events
- Selenium - Key Up/Down
- Selenium - Copy and Paste
- Selenium - Handle Special Keys
- Selenium - Mouse Events
- Selenium - Drag and Drop
- Selenium - Scroll Operations
- Selenium - Waiting Strategies
- Selenium - Explicit/Implicit Wait
- Selenium - Support Features
- Selenium - Multi Select
- Selenium - Wait Support
- Selenium - Select Support
- Selenium - Color Support
- Selenium - Errors & Logging
- Selenium - Logging
- Selenium - Exception Handling
- Selenium - Miscellaneous
- Selenium - Handling Ajax Calls
- Selenium - JSON Data File
- Selenium - CSV Data File
- Selenium - Excel Data File
- Selenium - Cross Browser Testing
- Selenium - Multi Browser Testing
- Selenium - Multi Windows Testing
- Selenium - JavaScript Executor
- Selenium - Headless Execution
- Selenium - Capture Screenshots
- Selenium - Capture Videos
- Selenium - Page Object Model
- Selenium - Page Factory
- Selenium - Record & Playback
- Selenium - Frameworks
- Selenium - Browsing Context
- Selenium Grid Tutorial
- Selenium - Overview
- Selenium - Architecture
- Selenium - Components
- Selenium - Configuration
- Selenium - Create Test Script
- Selenium - Test Execution
- Selenium - Endpoints
- Selenium - Customizing a Node
- Selenium Reporting Tools
- Selenium - Reporting Tools
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & other Technologies
- Selenium - Java Tutorial
- Selenium - Python Tutorial
- Selenium - C# Tutorial
- Selenium - Javascript Tutorial
- Selenium - Kotlin Tutorial
- Selenium - Ruby Tutorial
- Selenium - Maven & Jenkins
- Selenium - Database Testing
- Selenium - LogExpert Logging
- Selenium - Log4j Logging
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash Testing
- Selenium - Apache Ant
- Selenium - Github
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium Miscellaneous Concepts
- Selenium - Automation Frameworks
- Selenium - Keyword Driven Framework
- Selenium - Data Driven Framework
- Selenium - Hybrid Driven Framework
- Selenium - SSL Certificate Error
- Selenium - Alternatives
- Selenium Useful Resources
- Selenium - Quick Guide
- Selenium - Useful Resources
- Selenium - Automation Practice
- Selenium - Discussion
Selenium Webdriver - Find All Links
Selenium Webdriver can be used to count the total number of links on a web application. Often, while performing scrapping of a webpage, we would normally need to count the links.
In HTML terminology, every link (referred to as hyperlinks) are identified by the tagname called anchor. Also, each link on a webpage has an attribute called href.
Let us now discuss the identification of anchor tags for hyperlink - Home on a webpage shown in the below image. First, we would need to right click on the webpage, and then click on the Inspect button in the Chrome browser. Then, the corresponding HTML code for the whole page would be visible. For investigating a single element on a page, we would need to click on the left upward arrow, available to the top of the visible HTML code as highlighted below.
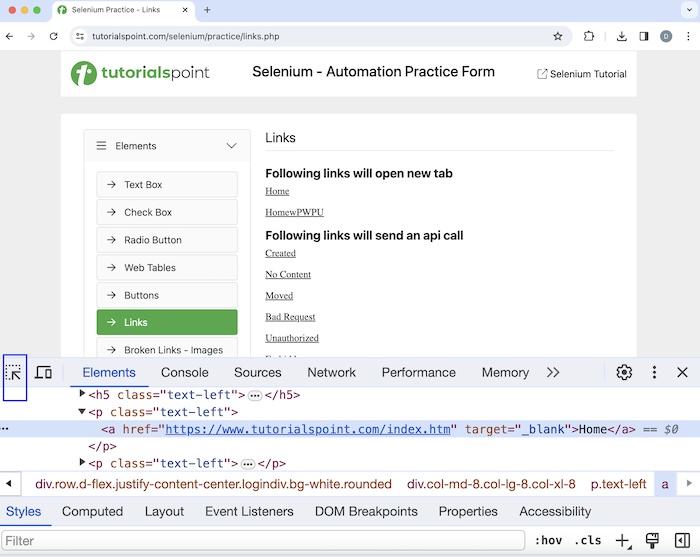
Once, we had clicked and pointed the arrow to the Home hyperlink, its HTML code would be visible, both reflecting the anchor tagname (referred to as ‘a’ and enclosed in <>), and href attribute containing the link to a page.
<a href="https://www.tutorialspoint.com/index.htm" target="_blank">Home</a>
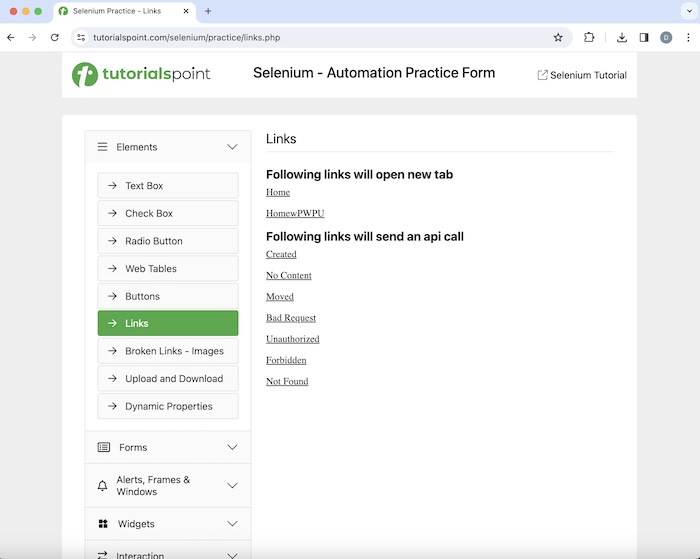
Let us take an example of the above page, where we would count the total number of links. Here on this page, the total number of links should be 42.
Syntax
Webdriver driver = new ChromeDriver(); // Retrieve all links using locator By.tagName a and storing in List List<WebElement> totalLnks = driver.findElements(By.tagName("a")); System.out.println( "Total number of links on a page: " + totalLnks.size() ) ;
Example
Code Implementation on TotalLinks.java class file.
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import java.util.List; import java.util.concurrent.TimeUnit; import org.openqa.selenium.By; import org.openqa.selenium.WebElement; public class TotalLink { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver() ; // adding implicit wait of 10 secs driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // Opening the webpage where we will count the links driver.get( "https://www.tutorialspoint.com/selenium/practice/links.php" ); // Retrieve all links using locator By.tagName a and storing in List List<WebElement> totalLnks = driver.findElements(By.tagName("a")); System.out.println( "Total number of links on a page: " + totalLnks.size() ); // Closing browser driver.quit(); } }
Maven dependencies added to pom.xml
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>SeleniumJava</artifactId> <version>1.0-SNAPSHOT</version> <properties> <maven.compiler.source>16</maven.compiler.source> <maven.compiler.target>16</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java --> <dependencies> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.11.0</version> </dependency> </dependencies> </project>
Output
Total number of links on a page: 42 Process finished with exit code 0
In the above example, we had counted the total number of links on a web page, and received the messages in the console - Total number of links on a page: 42.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Now, let us take a look at an example of getting the names of all the hyperlinks on the web page discussed above.
Syntax
Webdriver driver = new ChromeDriver(); // Retrieve all links using locator By.tagName a and storing in List List<WebElement> totalLnks = driver.findElements(By.tagName("a")); System.out.println( "Total number of links on a page: " + totalLnks.size()) ; // Running loop through list of web elements for ( int j = 0; j < totalLnks.size() ; j++) { // get the all hyperlink texts System.out.println( "Link text is: " + totalLnks.get(j).getText() ) ; }
Example
Code Implementation on TotalLinksWithNm.java class file −
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.List; import java.util.concurrent.TimeUnit; public class TotalLinksWithNm { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver() ; // adding implicit wait of 10 secs driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // Opening the webpage where we will count the links driver.get( "https://www.tutorialspoint.com/selenium/practice/links.php") ; // Retrieve all links using locator By.tagName and storing in List List<WebElement> totalLnks = driver.findElements(By.tagName("a")); System.out.println( "Total number of links: " + totalLnks.size() ); // Running loop through list of web elements for ( int j = 0; j < totalLnks.size() ; j++) { // get the all hyperlink texts System.out.println( "Link text is: " + totalLnks.get(j).getText() ) ; } // Closing browser driver.quit(); } }
Output
Total number of links: 42 Link text is: Link text is: Selenium Tutorial Link text is: Text Box Link text is: Check Box Link text is: Radio Button Link text is: Web Tables Link text is: Buttons Link text is: Links Link text is: Broken Links - Images Link text is: Upload and Download Link text is: Dynamic Properties Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Home Link text is: HomewPWPU Link text is: Created Link text is: No Content Link text is: Moved Link text is: Bad Request Link text is: Unauthorized Link text is: Forbidden Link text is: Not Found Process finished with exit code 0
In the above example, we had counted the total number of links on a web page, and received the message in the console - Total number of links: 42 and all the link texts.
At last, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Thus we were able to count the total number of links on a webpage. Along with that we had implemented how to get the text associated with a link.