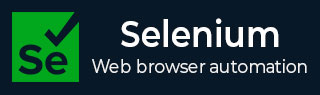
- Selenium Tutorial
- Selenium - Home
- Selenium - Overview
- Selenium - Components
- Selenium - Automation Testing
- Selenium - Environment Setup
- Selenium - Remote Control
- Selenium IDE Tutorial
- Selenium - IDE Introduction
- Selenium - Features
- Selenium - Limitations
- Selenium - Installation
- Selenium - Creating Tests
- Selenium - Creating Script
- Selenium - Store Variables
- Selenium - Alerts & Popups
- Selenium - Selenese Commands
- Selenium - Actions Commands
- Selenium - Accessors Commands
- Selenium - Assertions Commands
- Selenium - Assert/Verify Methods
- Selenium - Locating Strategies
- Selenium - Script Debugging
- Selenium - Verification Points
- Selenium - Pattern Matching
- Selenium - JSON Data File
- Selenium - Browser Execution
- Selenium - User Extensions
- Selenium - Code Export
- Selenium - JavaScript Functions
- Selenium - Plugins
- Selenium WebDriver Tutorial
- Selenium - Introduction
- Selenium WebDriver vs RC
- Selenium - Installation
- Selenium - First Test Script
- Selenium - Driver Sessions
- Selenium - Browser Options
- Selenium - Chrome Options
- Selenium - Edge Options
- Selenium - Firefox Options
- Selenium - Safari Options
- Selenium Web Elements
- Selenium - File Upload
- Selenium - Locator Strategies
- Selenium - Relative Locators
- Selenium - Finders
- Selenium - Find All Links
- Selenium - User Interactions
- Selenium - WebElement Commands
- Selenium - Browser Interactions
- Selenium - Browser Commands
- Selenium - Browser Navigation
- Selenium - Alerts & Popups
- Selenium - Handling Forms
- Selenium - Windows and Tabs
- Selenium - Handling Links
- Selenium - Input Boxes
- Selenium - Radio Button
- Selenium - Checkboxes
- Selenium - Dropdown Box
- Selenium - Handling IFrames
- Selenium - Handling Cookies
- Selenium - Date Time Picker
- Selenium - Dynamic Web Tables
- Selenium - Actions Class
- Selenium - Action Class
- Selenium - Keyboard Events
- Selenium - Key Up/Down
- Selenium - Copy and Paste
- Selenium - Handle Special Keys
- Selenium - Mouse Events
- Selenium - Drag and Drop
- Selenium - Scroll Operations
- Selenium - Waiting Strategies
- Selenium - Explicit/Implicit Wait
- Selenium - Support Features
- Selenium - Multi Select
- Selenium - Wait Support
- Selenium - Select Support
- Selenium - Color Support
- Selenium - Errors & Logging
- Selenium - Logging
- Selenium - Exception Handling
- Selenium - Miscellaneous
- Selenium - Handling Ajax Calls
- Selenium - JSON Data File
- Selenium - CSV Data File
- Selenium - Excel Data File
- Selenium - Cross Browser Testing
- Selenium - Multi Browser Testing
- Selenium - Multi Windows Testing
- Selenium - JavaScript Executor
- Selenium - Headless Execution
- Selenium - Capture Screenshots
- Selenium - Capture Videos
- Selenium - Page Object Model
- Selenium - Page Factory
- Selenium - Record & Playback
- Selenium - Frameworks
- Selenium - Browsing Context
- Selenium Grid Tutorial
- Selenium - Overview
- Selenium - Architecture
- Selenium - Components
- Selenium - Configuration
- Selenium - Create Test Script
- Selenium - Test Execution
- Selenium - Endpoints
- Selenium - Customizing a Node
- Selenium Reporting Tools
- Selenium - Reporting Tools
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & other Technologies
- Selenium - Java Tutorial
- Selenium - Python Tutorial
- Selenium - C# Tutorial
- Selenium - Javascript Tutorial
- Selenium - Kotlin Tutorial
- Selenium - Ruby Tutorial
- Selenium - Maven & Jenkins
- Selenium - Database Testing
- Selenium - LogExpert Logging
- Selenium - Log4j Logging
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash Testing
- Selenium - Apache Ant
- Selenium - Github
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium Miscellaneous Concepts
- Selenium - Automation Frameworks
- Selenium - Keyword Driven Framework
- Selenium - Data Driven Framework
- Selenium - Hybrid Driven Framework
- Selenium - SSL Certificate Error
- Selenium - Alternatives
- Selenium Useful Resources
- Selenium - Quick Guide
- Selenium - Useful Resources
- Selenium - Automation Practice
- Selenium - Discussion
Selenium Grid - Create Test Script
Selenium Grid consists of six components namely, the Router, Distributor, Nodes, Session Queue, Session Map, and Event Bus which enables the option to deploy it in various ways. Depending on the requirements, we can begin each of the components of the Selenium Grid individually in a Distributed mode, segregate them as Hub and Node in the Hub and Node mode, and all at once in a Standalone mode.
Standalone Mode in Selenium Grid
In a Standalone Mode, every component of Selenium Grid works as a single unit and can be triggered with a single command using a single process. It is the most convenient way to run the Selenium Grid. The server connects with the RemoteWebdriver requests on http://localhost:4444 and locates all the drivers from the System path automatically. Post triggering the Selenium Grid in Standalone mode, all the tests should use http://localhost:4444.
Benefits of Standalone Mode in Selenium Grid
The benefits of Standalone Mode in Selenium Grid are listed below −
Create and troubleshoot tests built using the RemoteWebdriver locally.
Executing and unit testing quickly prior to pushing the code.
Can be easily configured with CI/CD tools.
Hub and Node Mode in Selenium Grid
In Selenium Grid, the Hub comprises the components namely the Router, Session Map, Session Queue, and the Event Bus. The server connects with the RemoteWebdriver requests on http://localhost:4444 automatically.
The Node locates all the drivers from the System path during the starting point. Multiple Nodes can execute in the same machine. For example, if there are two Nodes, A and B running in the same machine, using the below commands −
For Node A,
java -jar selenium-server-<version>.jar node --port 5555
For Node B,
java -jar selenium-server-<version>.jar node --port 6666
The Hub and Node can be positioned on different machines where they communicate via the HTTP and the Event Bus. The Node directs messages to the Hub using the Event Bus for registration. Once the Hub gets the message, it extends to the Node using the HTTP to check its presence.
For registering a Node properly to the Hub, the Event Bus ports(default ports: 4442 and 4443) need to be available on the Hub machine and to the Nodes. This helps to establish the connection between the Hub and the Node. In case the Hub is utilizing the default ports, the --hub flag is used to register the Node as shown in the below command.
java -jar selenium-server-<version>.jar node --hub http://<hub-ip>:4444
If the Hub is not utilizing the default ports, the flags --publish-events and --subscribe-events are used as shown in the below command.
java -jar selenium-server-<version>.jar hub --publish-events tcp://<hub-ip>:8886 --subscribe-events tcp://<hub-ip>:8887 --port 8888
In the above example, the Hub is using the ports 8886, 8887, and 8888. After the Hub is registered, the Node uses the same ports for successful registration using the same Hub ports as shown in the below command.
java -jar selenium-server-<version>.jar node --publish-events tcp://<hub-ip>:8886 --subscribe-events tcp://<hub-ip>:8887
Benefits of Hub and Node mode in Selenium Grid
In Selenium Grid, it is observed that the Hub and Node mode is the most commonly used mode. The benefits of Hub and Node Mode in Selenium Grid are listed below −
The Hub and Node mode allows integration of various machines having different platforms, browsers, and their versions in the Selenium Grid.
The Hub and Node mode runs by pointing to one entry to execute the WebDriver tests in various environments.
The tests can be scaled up and down easily without impacting the complete Grid.
Distributed Mode in Selenium Grid
In a Distributed mode, each component of the Selenium Grid should be ideally positioned at different machines and they need to be kicked off separately. It is mandatory to utilize every port correctly to have correct interaction among all components.
To start with the Event Bus allows communication among Grid components. The default ports for the Event Bus are 4442, 4443, and 5557.
java -jar selenium-server-<version>.jar event-bus --publish-events tcp://<event-bus-ip>:4442 --subscribe-events tcp://<event-bus-ip>:4443 --port 5557
Then the New Session Queue appends a new session to the queue which will be obtained by the Distributor. The default ports for the New Session Queue is 5559.
java -jar selenium-server-<version>.jar sessionqueue --port 5559
The Session Map connects the session id to the Node where the session is executing and also communicates with the Event Bus. The default port for the New Session Queue is 5556.
java -jar selenium-server-<version>.jar sessions --publish-events tcp://<event-bus-ip>:4442 --subscribe-events tcp://<event-bus-ip>:4443 --port 5556
The Distributor obtains the New Session Queue for new session requests to the Node provided their capabilities are similar. The Nodes are registered to the Distributor in the similar way a Node is registered in a Hub and Node mode. The default port for the Distributor is 5553. The Distributor communicates with the New Session Queue, Session Map, Event Bus, and the Nodes.
java -jar selenium-server-<version>.jar distributor --publish-events tcp://<event-bus-ip>:4442 --subscribe-events tcp://<event-bus-ip>:4443 --sessions http://<sessions-ip>:5556 --sessionqueue http://<new-session-queue-ip>:5559 --port 5553 --bind-bus false
The Router changes the new session requests to the queue, and forwards the running session requests to the Node which is running the session. The default port for the Router is 4444. The Router communicates with the New Session Queue, Session Map, and the Distributor.
java -jar selenium-server-<version>.jar router --sessions http://<sessions-ip>:5556 --distributor http://<distributor-ip>:5553 --sessionqueue http://<new-session-queue-ip>:5559 --port 4444
The default port for Node is 5555.
java -jar selenium-server-<version>.jar node --publish-events tcp://<event-bus-ip>:4442 --subscribe-events tcp://<event-bus-ip>:4443
Benefits of Distributed Mode in Selenium Grid
The Distributed mode of Selenium Grid is used when there is a requirement to set up a large number of Nodes within a big size Grid(where there is only one Hub and so many Nodes across multiple machines). In such a situation, Hub and Node mode is not an ideal choice.
Prerequisites to Create a Test Script
Step 1 − Install Java(version above 8) in the system and check if it is present with the command: java -version. The java version installed will be visible if installation has been completed successfully.
Step 2 − Check Grid status by opening a browser and entering −
For the UI version, type http://localhost:4444.
For Non UI version, type http://localhost:4444/status.
For both the cases, we would get the error - This site can’t be reached. Since Selenium Grid has not been started yet.

Create a Test Script in Selenium Grid
The steps to create a test script in a Standalone mode are listed below −
Step 1 − Download Selenium Standalone Jar from the below link and save it in a folder −
https://github.com/SeleniumHQ/selenium/releases.
Step 2 − From the location of the folder where the Selenium Standalone Jar had been stored, run the below command from the terminal −
java -jar selenium-server-<version>.jar standalone.
Step 3 − Check Grid status again by opening a browser and entering: http://localhost:4444.
The Error − This site can’t be reached would no longer be there, and we would get Grid status showing different browsers. This would prove Selenium Grid had been triggered in the Standalone mode.

Step 4 −
Code Implementation in Base.java
package BaseClass; import java.net.MalformedURLException; import java.net.URL; import org.openqa.selenium.WebDriver; import org.openqa.selenium.remote.DesiredCapabilities; import org.openqa.selenium.remote.RemoteWebDriver; public class Base { public WebDriver setBrowser(String browserName) throws MalformedURLException { WebDriver driver = null; DesiredCapabilities dc = new DesiredCapabilities(); if(browserName.equalsIgnoreCase("chrome")) { dc.setBrowserName("chrome"); } else if(browserName.equalsIgnoreCase("edge")) { dc.setBrowserName("MicrosoftEdge"); } // Initiate RemoteWebDriver driver = new RemoteWebDriver(new URL("http://localhost:4444"),dc); return driver; } }
Code Implementation in TestOne.java
package Grid; import BaseClass.Base; import org.openqa.selenium.WebDriver; import org.testng.annotations.AfterMethod; import org.testng.annotations.BeforeMethod; import org.testng.annotations.Test; import java.net.MalformedURLException; public class TestOne extends Base { public WebDriver driver = null; @Test public void testOne() { // launch application driver.get("https://www.tutorialspoint.com/selenium/practice/links.php"); // get page title System.out.println("Page title is: " + driver.getTitle() + " obtained from testOne"); } @BeforeMethod public void setup() throws MalformedURLException { driver = setBrowser("chrome"); } @AfterMethod public void tearDown() { // quitting browser driver.quit(); } }
Code Implementation in TestTwo.java
package Grid; import BaseClass.Base; import org.openqa.selenium.WebDriver; import org.testng.annotations.AfterMethod; import org.testng.annotations.BeforeMethod; import org.testng.annotations.Test; import java.net.MalformedURLException; public class TestTwo extends Base { public WebDriver driver = null; @Test public void testTwo() { // launch application driver.get("https://www.tutorialspoint.com/selenium/practice/links.php"); // get page title System.out.println("Page title is: " + driver.getTitle() + " obtained from testTwo"); } @BeforeMethod public void setup() throws MalformedURLException { driver = setBrowser("edge"); } @AfterMethod public void tearDown() { // quitting browser driver.quit(); } }
Configurations in testng.xml file.
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd"> <suite name = "Grid Test"> <test thread-count = "5" name="Test"> <classes> <class name="Grid.TestOne" /> <class name="Grid.TestTwo"/> </classes> </test> </suite>
Step 5 − Run the test from the testng.xml file.
It will show the following output −
Page title is: Selenium Practice - Links obtained from testOne Page title is: Selenium Practice - Links obtained from testTwo =============================================== Grid Test Total tests run: 2, Passes: 2, Failures: 0, Skips: 0 =============================================== Process finished with exit code 0
In the above example, we had configured the Standalone mode of the Selenium Grid.
This concludes our comprehensive take on the tutorial on Selenium Grid - Create Test Script. We’ve started with describing Standalone, Hub and Node, and Distributed modes in Selenium Grid and their benefits, prerequisites to create a test script, and how to create a test script in Selenium Grid.
This equips you with in-depth knowledge of the Selenium Grid - Create Test Script. It is wise to keep practicing what you’ve learned and exploring others relevant to Selenium to deepen your understanding and expand your horizons.