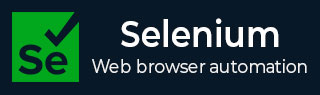
- Selenium Tutorial
- Selenium - Home
- Selenium - Overview
- Selenium - Components
- Selenium - Automation Testing
- Selenium - Environment Setup
- Selenium - Remote Control
- Selenium IDE Tutorial
- Selenium - IDE Introduction
- Selenium - Features
- Selenium - Limitations
- Selenium - Installation
- Selenium - Creating Tests
- Selenium - Creating Script
- Selenium - Store Variables
- Selenium - Alerts & Popups
- Selenium - Selenese Commands
- Selenium - Actions Commands
- Selenium - Accessors Commands
- Selenium - Assertions Commands
- Selenium - Assert/Verify Methods
- Selenium - Locating Strategies
- Selenium - Script Debugging
- Selenium - Verification Points
- Selenium - Pattern Matching
- Selenium - JSON Data File
- Selenium - Browser Execution
- Selenium - User Extensions
- Selenium - Code Export
- Selenium - JavaScript Functions
- Selenium - Plugins
- Selenium WebDriver Tutorial
- Selenium - Introduction
- Selenium WebDriver vs RC
- Selenium - Installation
- Selenium - First Test Script
- Selenium - Driver Sessions
- Selenium - Browser Options
- Selenium - Chrome Options
- Selenium - Edge Options
- Selenium - Firefox Options
- Selenium - Safari Options
- Selenium Web Elements
- Selenium - File Upload
- Selenium - Locator Strategies
- Selenium - Relative Locators
- Selenium - Finders
- Selenium - Find All Links
- Selenium - User Interactions
- Selenium - WebElement Commands
- Selenium - Browser Interactions
- Selenium - Browser Commands
- Selenium - Browser Navigation
- Selenium - Alerts & Popups
- Selenium - Handling Forms
- Selenium - Windows and Tabs
- Selenium - Handling Links
- Selenium - Input Boxes
- Selenium - Radio Button
- Selenium - Checkboxes
- Selenium - Dropdown Box
- Selenium - Handling IFrames
- Selenium - Handling Cookies
- Selenium - Date Time Picker
- Selenium - Dynamic Web Tables
- Selenium - Actions Class
- Selenium - Action Class
- Selenium - Keyboard Events
- Selenium - Key Up/Down
- Selenium - Copy and Paste
- Selenium - Handle Special Keys
- Selenium - Mouse Events
- Selenium - Drag and Drop
- Selenium - Scroll Operations
- Selenium - Waiting Strategies
- Selenium - Explicit/Implicit Wait
- Selenium - Support Features
- Selenium - Multi Select
- Selenium - Wait Support
- Selenium - Select Support
- Selenium - Color Support
- Selenium - Errors & Logging
- Selenium - Logging
- Selenium - Exception Handling
- Selenium - Miscellaneous
- Selenium - Handling Ajax Calls
- Selenium - JSON Data File
- Selenium - CSV Data File
- Selenium - Excel Data File
- Selenium - Cross Browser Testing
- Selenium - Multi Browser Testing
- Selenium - Multi Windows Testing
- Selenium - JavaScript Executor
- Selenium - Headless Execution
- Selenium - Capture Screenshots
- Selenium - Capture Videos
- Selenium - Page Object Model
- Selenium - Page Factory
- Selenium - Record & Playback
- Selenium - Frameworks
- Selenium - Browsing Context
- Selenium Grid Tutorial
- Selenium - Overview
- Selenium - Architecture
- Selenium - Components
- Selenium - Configuration
- Selenium - Create Test Script
- Selenium - Test Execution
- Selenium - Endpoints
- Selenium - Customizing a Node
- Selenium Reporting Tools
- Selenium - Reporting Tools
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & other Technologies
- Selenium - Java Tutorial
- Selenium - Python Tutorial
- Selenium - C# Tutorial
- Selenium - Javascript Tutorial
- Selenium - Kotlin Tutorial
- Selenium - Ruby  Tutorial
- Selenium - Maven & Jenkins
- Selenium - Database Testing
- Selenium - LogExpert Logging
- Selenium - Log4j Logging
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash Testing
- Selenium - Apache Ant
- Selenium - Github
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium Miscellaneous Concepts
- Selenium - Automation Frameworks
- Selenium - Keyword Driven Framework
- Selenium - Data Driven Framework
- Selenium - Hybrid Driven Framework
- Selenium - SSL Certificate Error
- Selenium - Alternatives
- Selenium Useful Resources
- Selenium - Quick Guide
- Selenium - Useful Resources
- Selenium - Automation Practice
- Selenium - Discussion
Selenium WebDriver - Windows and Tabs
Selenium Webdriver can be used to handle windows and tabs. There is no difference in handling windows and tabs by Selenium Webdriver. Each window opened has a unique identifier for that session.
Once a new window is opened, the driver context still remains on the parent window. In order to perform some tasks on the child windows, we would need to switch the driver context from the main window to the child windows.
There are multiple methods available in Selenium that can enable us to automate tests based on windows and tabs. For accessing the child windows, we have to first switch the driver context to the other window using the switchTo().window("window handle id of the child window") method. Let us discuss some other methods −
driver.getWindowHandle() − This is used to get the window handle id of the window in focus. Its return type is a String.
driver.getWindowHandles() − This is used to get all the window handle ids of the windows which are opened. Its return type is a Set of Strings.
Let us take an example of the below page, where we would click on the New Tab button.
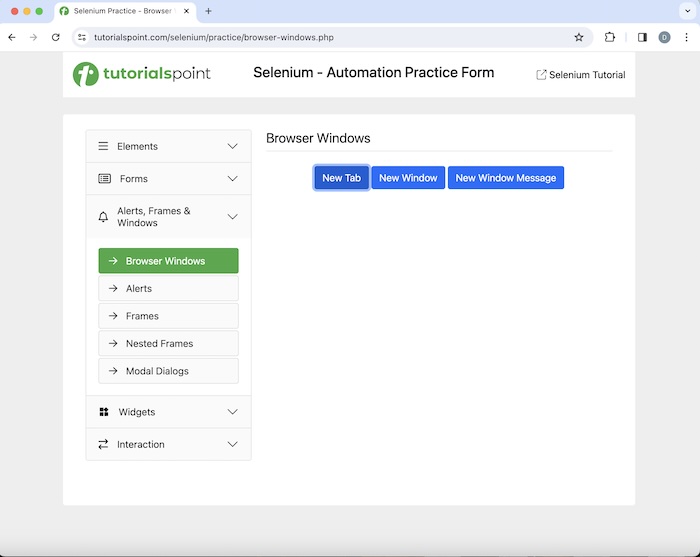
After clicking on the New Tab, we would navigate to another tab having the text New Tab.

Syntax
WebDriver driver = new ChromeDriver(); // Get the window handle of the original window String oW = driver.getWindowHandle(); // get all opened windows handle ids Set<String> windows = driver.getWindowHandles(); // Iterating through all window handles for (String w : windows) { if(!oW.equalsIgnoreCase(w)) { // switching to child window driver.switchTo().window(w); // accessing element in new tab WebElement e = driver.findElement(By.xpath("<value of xpath>")); System.out.println("Text in new tab is: " + e.getText()); break; } }
Example
Code Implementation on Tabs.java class file.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.Set; import java.util.concurrent.TimeUnit; public class Tabs { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 12 secs driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS); // Opening the webpage where we will open a new tab driver.get("https://www.tutorialspoint.com/selenium/practice/browser-windows.php"); // click link and navigate to next tab WebElement b = driver.findElement (By.xpath("/html/body/main/div/div/div[2]/button[1]")); b.click(); // Get the window handle of the original window String oW = driver.getWindowHandle(); // get all opened windows handle ids Set<String> windows = driver.getWindowHandles(); // Iterating through all window handles for (String w : windows) { if(!oW.equalsIgnoreCase(w)) { // switching to child tab driver.switchTo().window(w); // accessing element in new tab WebElement e = driver.findElement (By.xpath("/html/body/main/div/div/h1")); System.out.println("Text in new tab is: " + e.getText()); break; } } // quitting the browser driver.quit(); } }
Output
Text in new tab is: New Tab Process finished with exit code 0
In the above example, we captured the text on the new tab and received the message in the console - Text in new tab is: New Tab.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Let us take another example as shown in the below image where we would click on the New Window Message button.
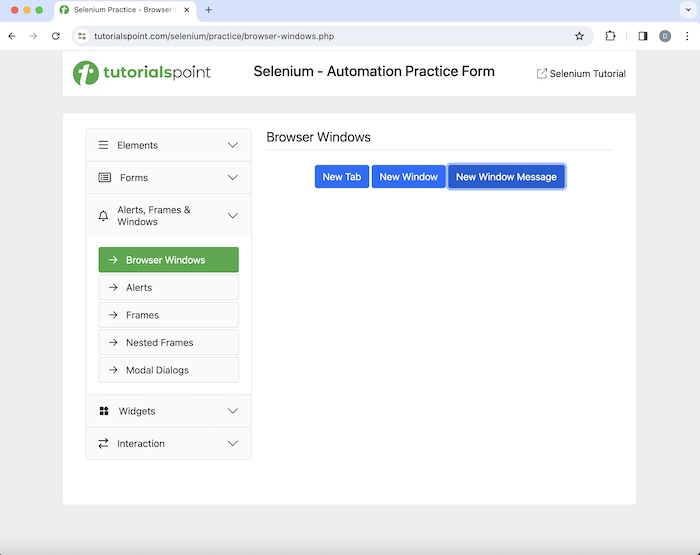
After clicking on the New Window Message, we would navigate to another window having the text New Window Message.
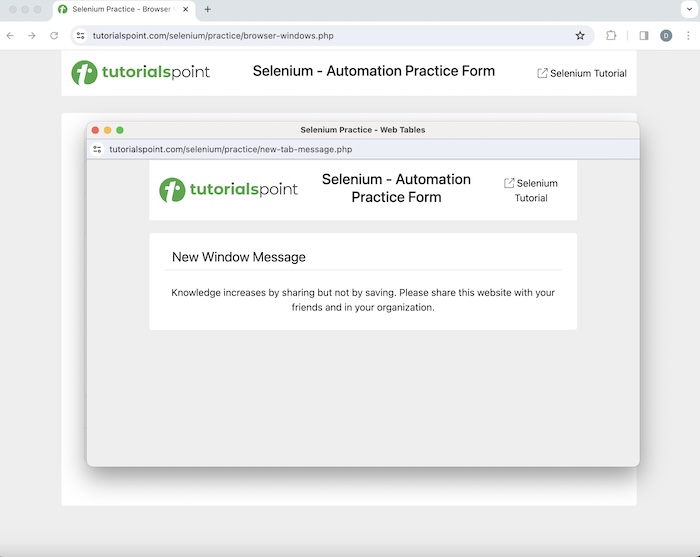
Then we would close the new window and switch back to the original window and get the text - Browser Windows there. Finally we would quit the session.
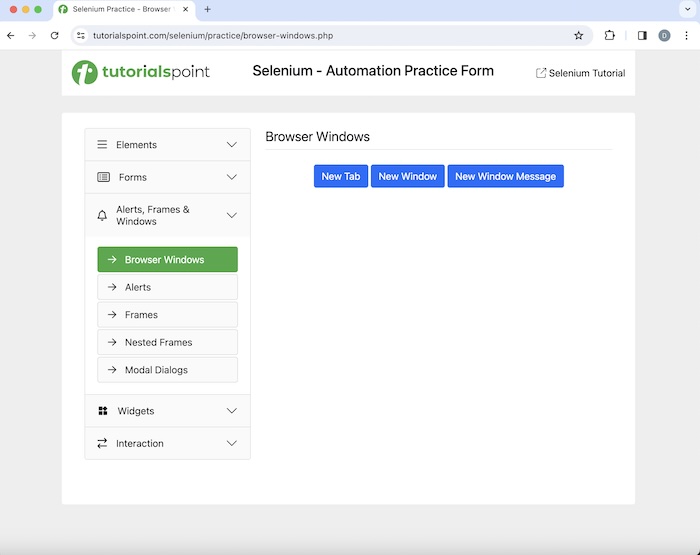
Example
Code Implementation on Windows.java class file.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.Set; import java.util.concurrent.TimeUnit; public class Windows { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 12 secs driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS); // Opening the webpage where we will open a new window driver.get("https://www.tutorialspoint.com/selenium/practice/browser-windows.php"); // click link and navigate to next window WebElement b = driver.findElement (By.xpath("/html/body/main/div/div/div[2]/button[3]")); b.click(); // Get the window handle of the original window String oW = driver.getWindowHandle(); // get all opened windows handle ids Set<String> windows = driver.getWindowHandles(); // Iterating through all window handles for (String w : windows) { if(!oW.equalsIgnoreCase(w)) { // switching to child window driver.switchTo().window(w); // accessing element in new window WebElement e = driver.findElement (By.xpath("/html/body/main/div/div/h1")); System.out.println("Text in new window is: " + e.getText()); driver.close(); break; } } // switching to parent window driver.switchTo().window(oW); // accessing element in parent window WebElement e1 = driver.findElement (By.xpath("/html/body/main/div/div/div[2]/h1")); System.out.println("Text in parent window is: " + e1.getText()); // quitting the browser session driver.quit(); } }
Output
Text in new window is: New Window Message Text in parent window is: Browser Windows Process finished with exit code 0
In the above example, we captured the text on the new window and received the message in the console - Text in new window is: New Window Message. Then we closed the child window and switched back to the parent window. Lastly, obtain the text on the parent window in the console - Text in parent window is: Browser Windows.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Thus there is a difference between close() and quit() methods. While the close() method only closes the browser window in focus, the quit() method closes everything associated with the driver session(browser and all background driver processes).
Selenium 4 gives the option to open a new tab or window. To open a new window, we would take the help of the method −
driver.switchTo().newWindow(WindowType.WINDOW)
Let us take another example where we would first open a window and launch an application having the text - Check Box.
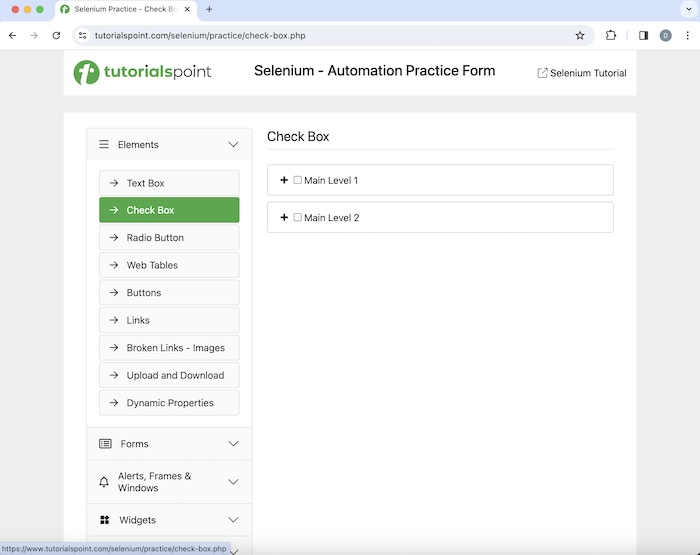
Then we would open a new window and launch another application having the text - Radio Button.

Example
Code Implementation on NewWindow.java class file.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.WindowType; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class NewWindow { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 12 secs driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS); // Opening the webpage in window 1 driver.get("https://www.tutorialspoint.com/selenium/practice/check-box.php"); // get text in window 1 WebElement e = driver.findElement (By.xpath("/html/body/main/div/div/div[2]/h1")); System.out.println("Text is: " + e.getText()); // Initiate the Webdriver WebDriver newDriver = driver.switchTo().newWindow(WindowType.WINDOW); // Opening the webpage in new window driver.get("https://www.tutorialspoint.com/selenium/practice/radio-button.php"); // get text in window 2 WebElement e1 = driver.findElement (By.xpath("/html/body/main/div/div/div[2]/form/h1")); System.out.println("Text in new window is: " + e1.getText()); // quitting the browser session driver.quit(); } }
Output
Text is: Check Box Text in new window is: Radio Button Process finished with exit code 0
In the above example, we captured the text in the first window and received the message in the console - Text is: Check Box. Then opened another window and an application there. Finally, we obtained the text in the new window in the console - Text in new window is: Radio Button.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Selenium 4 gives the option to open a new tab or window. To open a new tab, we would take the help of the method −
driver.switchTo().newWindow(WindowType.TAB)
Let us take another example where we would first open a window and launch an application having the text - Check Box.
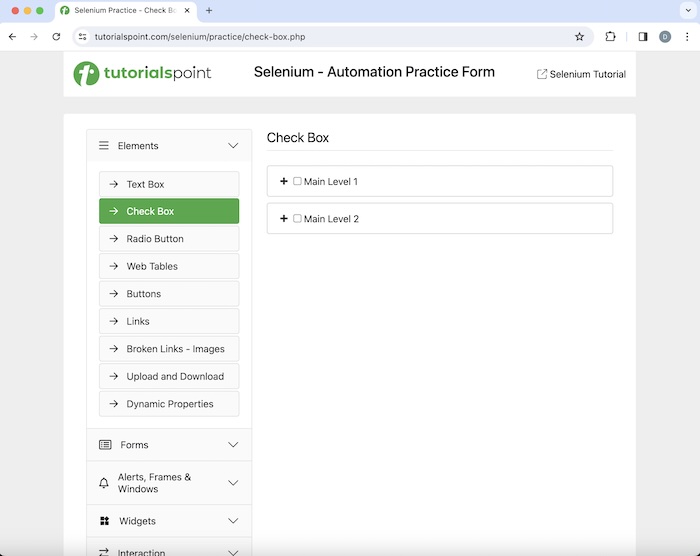
Then we would open a new tab and launch another application having the text - Radio Button.
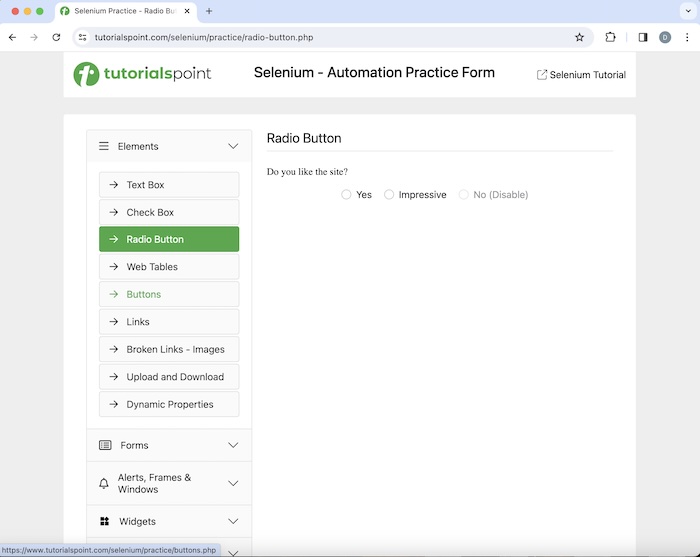
Example
Code Implementation on NewTabs.java class file.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.WindowType; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class NewTabs { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 12 secs driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS); // Opening the webpage in window 1 driver.get("https://www.tutorialspoint.com/selenium/practice/check-box.php"); // get text in window 1 WebElement e = driver.findElement (By.xpath("/html/body/main/div/div/div[2]/h1")); System.out.println("Text is: " + e.getText()); // Initiate the Webdriver WebDriver newDriver =driver.switchTo().newWindow(WindowType.TAB); // Opening the webpage in new tab driver.get("https://www.tutorialspoint.com/selenium/practice/radio-button.php"); // get text in other tab WebElement e1 = driver.findElement (By.xpath("/html/body/main/div/div/div[2]/form/h1")); System.out.println("Text in other tab is: " + e1.getText()); // quitting the browser session driver.quit(); } }
Output
Text is: Check Box Text in other tab is: Radio Button Process finished with exit code 0
In the above example, we captured the text in the first window and received the message in the console - Text is: Check Box. Then opened another tab and an application there. Finally, we obtained the text in the new tab in the console - Text in other tab is: Radio Button.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Thus, in this tutorial, we had discussed how to handle windows and tabs using the Selenium Webdriver.