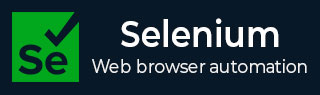
- Selenium Tutorial
- Selenium - Home
- Selenium - Overview
- Selenium - Components
- Selenium - Automation Testing
- Selenium - Environment Setup
- Selenium - Remote Control
- Selenium IDE Tutorial
- Selenium - IDE Introduction
- Selenium - Features
- Selenium - Limitations
- Selenium - Installation
- Selenium - Creating Tests
- Selenium - Creating Script
- Selenium - Store Variables
- Selenium - Alerts & Popups
- Selenium - Selenese Commands
- Selenium - Actions Commands
- Selenium - Accessors Commands
- Selenium - Assertions Commands
- Selenium - Assert/Verify Methods
- Selenium - Locating Strategies
- Selenium - Script Debugging
- Selenium - Verification Points
- Selenium - Pattern Matching
- Selenium - JSON Data File
- Selenium - Browser Execution
- Selenium - User Extensions
- Selenium - Code Export
- Selenium - JavaScript Functions
- Selenium - Plugins
- Selenium WebDriver Tutorial
- Selenium - Introduction
- Selenium WebDriver vs RC
- Selenium - Installation
- Selenium - First Test Script
- Selenium - Driver Sessions
- Selenium - Browser Options
- Selenium - Chrome Options
- Selenium - Edge Options
- Selenium - Firefox Options
- Selenium - Safari Options
- Selenium Web Elements
- Selenium - File Upload
- Selenium - Locator Strategies
- Selenium - Relative Locators
- Selenium - Finders
- Selenium - Find All Links
- Selenium - User Interactions
- Selenium - WebElement Commands
- Selenium - Browser Interactions
- Selenium - Browser Commands
- Selenium - Browser Navigation
- Selenium - Alerts & Popups
- Selenium - Handling Forms
- Selenium - Windows and Tabs
- Selenium - Handling Links
- Selenium - Input Boxes
- Selenium - Radio Button
- Selenium - Checkboxes
- Selenium - Dropdown Box
- Selenium - Handling IFrames
- Selenium - Handling Cookies
- Selenium - Date Time Picker
- Selenium - Dynamic Web Tables
- Selenium - Actions Class
- Selenium - Action Class
- Selenium - Keyboard Events
- Selenium - Key Up/Down
- Selenium - Copy and Paste
- Selenium - Handle Special Keys
- Selenium - Mouse Events
- Selenium - Drag and Drop
- Selenium - Scroll Operations
- Selenium - Waiting Strategies
- Selenium - Explicit/Implicit Wait
- Selenium - Support Features
- Selenium - Multi Select
- Selenium - Wait Support
- Selenium - Select Support
- Selenium - Color Support
- Selenium - Errors & Logging
- Selenium - Logging
- Selenium - Exception Handling
- Selenium - Miscellaneous
- Selenium - Handling Ajax Calls
- Selenium - JSON Data File
- Selenium - CSV Data File
- Selenium - Excel Data File
- Selenium - Cross Browser Testing
- Selenium - Multi Browser Testing
- Selenium - Multi Windows Testing
- Selenium - JavaScript Executor
- Selenium - Headless Execution
- Selenium - Capture Screenshots
- Selenium - Capture Videos
- Selenium - Page Object Model
- Selenium - Page Factory
- Selenium - Record & Playback
- Selenium - Frameworks
- Selenium - Browsing Context
- Selenium Grid Tutorial
- Selenium - Overview
- Selenium - Architecture
- Selenium - Components
- Selenium - Configuration
- Selenium - Create Test Script
- Selenium - Test Execution
- Selenium - Endpoints
- Selenium - Customizing a Node
- Selenium Reporting Tools
- Selenium - Reporting Tools
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & other Technologies
- Selenium - Java Tutorial
- Selenium - Python Tutorial
- Selenium - C# Tutorial
- Selenium - Javascript Tutorial
- Selenium - Kotlin Tutorial
- Selenium - Ruby Tutorial
- Selenium - Maven & Jenkins
- Selenium - Database Testing
- Selenium - LogExpert Logging
- Selenium - Log4j Logging
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash Testing
- Selenium - Apache Ant
- Selenium - Github
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium Miscellaneous Concepts
- Selenium - Automation Frameworks
- Selenium - Keyword Driven Framework
- Selenium - Data Driven Framework
- Selenium - Hybrid Driven Framework
- Selenium - SSL Certificate Error
- Selenium - Alternatives
- Selenium Useful Resources
- Selenium - Quick Guide
- Selenium - Useful Resources
- Selenium - Automation Practice
- Selenium - Discussion
Selenium - User Interactions
Selenium WebDriver can be used for various user interactions like clicking a link or a button, entering or clearing text from an input box, and selecting or deselecting options in a dropdown.
Let us discuss some of the most common commands that can be performed on an element.
click()
This method is used to perform a click even at the middle of an element. In case, middle of an element is hidden, an exception would be thrown.
Let us now discuss the identification of a link - No Content on a webpage shown in the below image. First, we would need to right click on the webpage, and then click on the Inspect button in the Chrome browser. Then, the corresponding HTML code for the whole page would be visible. For investigating a single element on a page, we would need to click on the left upward arrow, available to the top of the visible HTML code as highlighted below.
Once, we had clicked and pointed the arrow to the No Content hyperlink, its HTML code was visible.
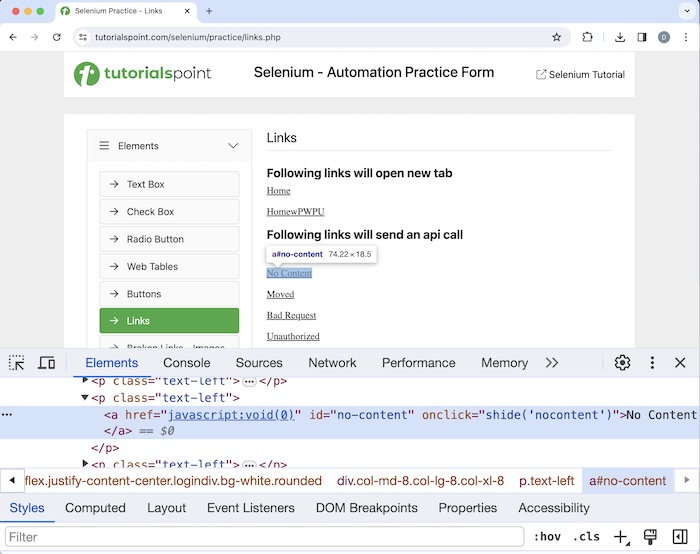
<a href="javascript:void(0)" id="no-content" onclick="shide('nocontent')">No Content</a>
We can identify a link with the help of the link text locator. With this, the first element with the matching value of the link text should be returned.
Let us take an example, where we would first click the No Content link, after which we would get the text Link has responded with status 204 and status text on a web page.
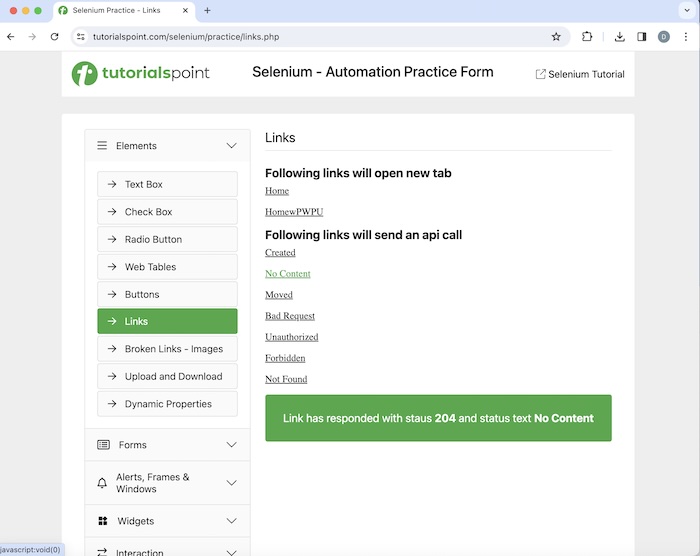
Syntax
Webdriver driver = new ChromeDriver(); driver.findElement(By.linkText("value of link text”));
Example
Code Implementation on HandlingLnks.java class file.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class HandlingLnks { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify link driver.get("https://www.tutorialspoint.com/selenium/practice/links.php"); // Identify link with link text driver.findElement(By.linkText("No Content")).click(); // identify text WebElement l = driver.findElement (By.xpath("/html/body/main/div/div/div[2]/div[2]")); // Get text after clicking the link System.out.println("Text is: " + l.getText()); // Closing browser driver.quit(); } }
Output
Text is: Link has responded with status 204 and status text No Content Process finished with exit code 0
In the above example, the text obtained after clicking on the link No Content was Text is: Link has responded with status 204 and status text No Content.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
sendKeys()
This method is used to enter some text in the edit box or any editable field. In HTML terminology, every input box is identified by the tagname called input. Also, an input box should have the type attribute having the value as text.
If an element is not editable, and sendKeys() method is applied on it, an exception should be thrown.
clear()
This method is used to clear text from an edit box or any editable field. If an element is not editable, and a clear() method is applied on it, an exception should be thrown.
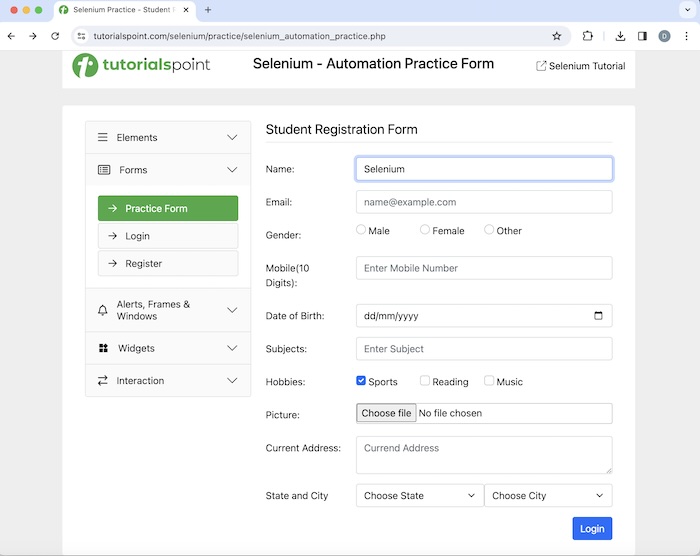
Let us take an example of the above page, where we would first enter some text in the input box with the help of the sendKeys() method. The value to enter is passed as an argument to the method. Then, we would wipe out the text entered with the clear() method.
Syntax
Webdriver driver = new ChromeDriver(); driver.findElement(By.xpath("value of xpath”)).sendKeys("value entered"); driver.findElement(By.xpath("value of xpath”)).clear();
Example
Code Implementation on HandlingInputBox.java class file.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class HandlingInptBox { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify edit box and enter driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Identify the input box with xpath locator WebElement e = driver.findElement(By.xpath("//*[@id='name']")); // enter text in input box e.sendKeys("Selenium"); // Get the value entered String text = e.getAttribute("value"); System.out.println("Entered text is: " + text); // clear the text entered e.clear(); // Get no text after clearing text String text1 = e.getAttribute("value"); System.out.println("Get text after clearing: " + text1); // Closing browser driver.quit(); } }
Output
Entered text is: Selenium Get text after clearing: Process finished with exit code 0
In the above example, we had first entered the text Selenium in the input box, and also retrieved the value entered in the console with the message- Entered text is: Selenium. Then cleared the value entered and there was no value in the input box after clearing up the text. Hence, we had also received the message in the console: Get text after clearing:.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Select class
There are multiple methods on Select class that help us to interact with dropdown. In HTML terminology, every dropdown is identified by the tagname called select. Also, each of its options are identified with the tagname called the option. There can be two types of dropdown on a web page - single select (allows selection one option) and multiple select (allows selection more than one option). In addition to it, a multiple select dropdown should have an attribute multiple.

<select class="form-select" id="inputGroupSelect03" aria-label="Example select with button addon"> <option selected="">Pick one title</option> <option value="1">Dr.</option> <option value="2">Mr.</option> <option value="3">Mrs.</option> <option value="3">Ms.</option> <option value="3">Proof.</option> <option value="3">Other</option> </select>
Please note one of the options Pick one title has the attribute selected, meaning this is selected by default, even before any option is selected.
Let us discuss some of the methods available in Selenium which would help us to access dropdowns on a web page.
getOptions() − returns the list of all options in the dropdown.
getFirstSelectedOption() − returns the selected option in the dropdown. If there are multi options selected, only the first item shall be returned.
isMultiple() − returns a boolean value, yields a true value if the dropdown allows selection of multiple items.
selectByIndex() − The index of the option to be selected by the dropdown is passed as a parameter. The index starts from 0.
selectByValue() − The value attribute of the option to be selected by the dropdown is passed as a parameter. The options in the dropdown should have the value attribute so that this method can be used.
selectByVisibleText() − The visible text of the option to be selected by the dropdown is passed as a parameter.
deselectByVisibleText() − The visible text of the option to be deselected by the dropdown is passed as a parameter. It is only applicable to multi-select dropdowns.
deselectByValue() − The value attribute of the option to be deselected by the dropdown is passed as a parameter. The options in the dropdown should have the value attribute so that this method can be used. It is only applicable to multi-select dropdowns.
deselectByIndex() − The index of the option to be deselected by the dropdown is passed as a parameter. The index starts from 0. It is only applicable to multi-select dropdowns.
deselectAll() − unselects all selected options from the dropdown.
getAllSelectedOptions() − returns all the selected options in the dropdown. If there are multi options selected, 0 or list of multiple selected options shall be returned. For a single select dropdown, the list of the 1 selected option shall be returned.
If a dropdown list has an attribute disabled for an option, that option would not be selected.
Let us take another example of the below page, where we would access the multi-select dropdown beside the text Multi Select drop down, select the values Electronics & Computers and Sports & Outdoors, and perform some validations with the methods discussed above.

<select id="demo-multiple-select" multiple=""> <option value="1">Books</option> <option value="2">Movies, Music & Games</option> <option value="3">Electronics & Computers</option> <option value="4">Home, Garden & Tools</option> <option value="5">Health & Beauty</option> <option value="6">Toys, Kids & Baby</option> <option value="7">Clothing & Jewelry</option> <option value="8">Sports & Outdoors</option> </select>
Syntax
WebDriver driver = new ChromeDriver(); // identify multiple dropdown WebElement dropdown = driver.findElement (By.xpath("<value of xpath>")); // object of Select class Select select = new Select(dropdown); // gets options of dropdown in list List<WebElement> options = select.getOptions(); for (WebElement opt : options){ System.out.println("Options are: " + opt.getText()); } // return true if multi-select dropdown Boolean b = select.isMultiple(); System.out.println("Boolean value for multiple dropdown: "+ b); // select item by value select.selectByValue("3"); // select item by index select.selectByIndex(7); // get all selected options of dropdown in list List<WebElement> selectedOptions = select.getAllSelectedOptions(); for (WebElement opt : selectedOptions){ System.out.println("Selected Options are: " + opt.getText()); } // get first selected option in dropdown WebElement f = select.getFirstSelectedOption(); System.out.println("First selected option is: "+ f.getText()); // deselect option by index select.deselectByIndex(7); // deselect all selected items select.deselectAll(); // get all selected options of dropdown after deselected List<WebElement> delectedOptions = select.getAllSelectedOptions(); System.out.println("No. options selected: " + delectedOptions.size());
Example
Code Implementation on SelectMultipleDropdowns.java class file.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.Select; import java.util.List; import java.util.concurrent.TimeUnit; public class SelectMultipleDropdowns { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will get dropdown driver.get("https://www.tutorialspoint.com/selenium/practice/select-menu.php"); // identify multiple dropdown WebElement dropdown = driver.findElement (By.xpath("//*[@id='demo-multiple-select']")); // object of Select class Select select = new Select(dropdown); // gets options of dropdown in list List<WebElement> options = select.getOptions(); for (WebElement opt : options){ System.out.println("Options are: " + opt.getText()); } // return true if multi-select dropdown Boolean b = select.isMultiple(); System.out.println("Boolean value for multiple dropdown: "+ b); // select item by value select.selectByValue("3"); // select item by index select.selectByIndex(7); // get all selected options of dropdown in list List<WebElement> selectedOptions = select.getAllSelectedOptions(); for (WebElement opt : selectedOptions){ System.out.println("Selected Options are: " + opt.getText()); } // get first selected option in dropdown WebElement f = select.getFirstSelectedOption(); System.out.println("First selected option is: "+ f.getText()); // deselect option by index select.deselectByIndex(7); // deselect all selected items select.deselectAll(); // get all selected options of dropdown after deselected List<WebElement> delectedOptions = select.getAllSelectedOptions(); System.out.println("No. options selected: " + delectedOptions.size()); // Closing browser driver.quit(); } }
Output
Options are: Books Options are: Movies, Music & Games Options are: Electronics & Computers Options are: Home, Garden & Tools Options are: Health & Beauty Options are: Toys, Kids & Baby Options are: Clothing & Jewelry Options are: Sports & Outdoors Boolean value for multiple dropdown: true Selected Options are: Electronics & Computers Selected Options are: Sports & Outdoors First selected option is: Electronics & Computers No. options selected: 0 Process finished with exit code 0
In the above example, we had obtained all the options of the dropdown with the message in the console - Options are: Books, Options are: Movies, Music & Games, Options are: Home, Garden & Tools, Options are: Health & Beauty, Options are: Toys, Kids & Baby, Options are: Clothing & Jewelry, Options are: Sports & Outdoors. We had also validated that the dropdown had multiple selection options with the message in the console - Boolean value for checking is: true.
We had retrieved the selected options in the dropdown with the message in the console - Selected Options are: Electronics & Computers, Selected Options are: Sports & Outdoors. We had also obtained the first selected option with the message in the console - First selected option is: Electronics & Computers. Finally, we deselected all the selected options in the dropdown, and hence obtained the message in the console - No. options selected: 0.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Selenium webdriver can be used to do scrolling actions on a web page with the help of the JavaScriptExecutor(an interface). Selenium Webdriver can execute the JavaScript commands using the JavaScriptExecutor.
Let us now discuss how to perform a scrolling till the visibility of an element on a web page with the help of scrollIntoView method.
Syntax
WebDriver driver = new ChromeDriver(); // identify element the element WebElement l = driver.findElement(By.xpath("value of xpath locator")); // JavascriptExecutor to scrolling to view of an element JavascriptExecutor javascriptExecutor = (JavascriptExecutor) driver; javascriptExecutor.executeScript("arguments[0].scrollIntoView();", l);
Example
Code Implementation on ScrollIntoView.java class file.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.JavascriptExecutor; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class ScrollVw { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Identify element to view WebElement e = driver.findElement(By.xpath("//*[@id='headingFive']/button")); // JavascriptExecutor to scrolling to view of an element JavascriptExecutor javascriptExecutor = (JavascriptExecutor) driver; javascriptExecutor.executeScript("arguments[0].scrollIntoView();", e); e.click(); // Closing browser driver.quit(); } }
In this tutorial, we had discussed various user interactions that can be performed using Selenium Webdriver.