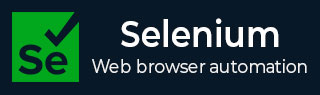
- Selenium Tutorial
- Selenium - Home
- Selenium - Overview
- Selenium - Components
- Selenium - Automation Testing
- Selenium - Environment Setup
- Selenium - Remote Control
- Selenium IDE Tutorial
- Selenium - IDE Introduction
- Selenium - Features
- Selenium - Limitations
- Selenium - Installation
- Selenium - Creating Tests
- Selenium - Creating Script
- Selenium - Store Variables
- Selenium - Alerts & Popups
- Selenium - Selenese Commands
- Selenium - Actions Commands
- Selenium - Accessors Commands
- Selenium - Assertions Commands
- Selenium - Assert/Verify Methods
- Selenium - Locating Strategies
- Selenium - Script Debugging
- Selenium - Verification Points
- Selenium - Pattern Matching
- Selenium - JSON Data File
- Selenium - Browser Execution
- Selenium - User Extensions
- Selenium - Code Export
- Selenium - JavaScript Functions
- Selenium - Plugins
- Selenium WebDriver Tutorial
- Selenium - Introduction
- Selenium WebDriver vs RC
- Selenium - Installation
- Selenium - First Test Script
- Selenium - Driver Sessions
- Selenium - Browser Options
- Selenium - Chrome Options
- Selenium - Edge Options
- Selenium - Firefox Options
- Selenium - Safari Options
- Selenium Web Elements
- Selenium - File Upload
- Selenium - Locator Strategies
- Selenium - Relative Locators
- Selenium - Finders
- Selenium - Find All Links
- Selenium - User Interactions
- Selenium - WebElement Commands
- Selenium - Browser Interactions
- Selenium - Browser Commands
- Selenium - Browser Navigation
- Selenium - Alerts & Popups
- Selenium - Handling Forms
- Selenium - Windows and Tabs
- Selenium - Handling Links
- Selenium - Input Boxes
- Selenium - Radio Button
- Selenium - Checkboxes
- Selenium - Dropdown Box
- Selenium - Handling IFrames
- Selenium - Handling Cookies
- Selenium - Date Time Picker
- Selenium - Dynamic Web Tables
- Selenium - Actions Class
- Selenium - Action Class
- Selenium - Keyboard Events
- Selenium - Key Up/Down
- Selenium - Copy and Paste
- Selenium - Handle Special Keys
- Selenium - Mouse Events
- Selenium - Drag and Drop
- Selenium - Scroll Operations
- Selenium - Waiting Strategies
- Selenium - Explicit/Implicit Wait
- Selenium - Support Features
- Selenium - Multi Select
- Selenium - Wait Support
- Selenium - Select Support
- Selenium - Color Support
- Selenium - Errors & Logging
- Selenium - Logging
- Selenium - Exception Handling
- Selenium - Miscellaneous
- Selenium - Handling Ajax Calls
- Selenium - JSON Data File
- Selenium - CSV Data File
- Selenium - Excel Data File
- Selenium - Cross Browser Testing
- Selenium - Multi Browser Testing
- Selenium - Multi Windows Testing
- Selenium - JavaScript Executor
- Selenium - Headless Execution
- Selenium - Capture Screenshots
- Selenium - Capture Videos
- Selenium - Page Object Model
- Selenium - Page Factory
- Selenium - Record & Playback
- Selenium - Frameworks
- Selenium - Browsing Context
- Selenium Grid Tutorial
- Selenium - Overview
- Selenium - Architecture
- Selenium - Components
- Selenium - Configuration
- Selenium - Create Test Script
- Selenium - Test Execution
- Selenium - Endpoints
- Selenium - Customizing a Node
- Selenium Reporting Tools
- Selenium - Reporting Tools
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & other Technologies
- Selenium - Java Tutorial
- Selenium - Python Tutorial
- Selenium - C# Tutorial
- Selenium - Javascript Tutorial
- Selenium - Kotlin Tutorial
- Selenium - Ruby Tutorial
- Selenium - Maven & Jenkins
- Selenium - Database Testing
- Selenium - LogExpert Logging
- Selenium - Log4j Logging
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash Testing
- Selenium - Apache Ant
- Selenium - Github
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium Miscellaneous Concepts
- Selenium - Automation Frameworks
- Selenium - Keyword Driven Framework
- Selenium - Data Driven Framework
- Selenium - Hybrid Driven Framework
- Selenium - SSL Certificate Error
- Selenium - Alternatives
- Selenium Useful Resources
- Selenium - Quick Guide
- Selenium - Useful Resources
- Selenium - Automation Practice
- Selenium - Discussion
Selenium WebDriver - WebElement Commands
Selenium Webdriver can be used to extract multiple information about a particular element on a web page. For example, we can validate if an element is available/unavailable, enabled/disabled or selected/unselected on a web page.
To verify if an element is available on a web page, we can take the help of the isDisplayed() method. If the element is available, isDisplayed() would return true, else false.
To verify if an element is selected on a web page, we can take the help of the isSelected() method. If the element is selected, isSelected() would return true, else false.
To verify if an element is enabled on a web page, we can take the help of the isEnabled() method. If the element is enabled, isEnabled() would return true, else false.
Let us now discuss the identification of a radio button on a web page shown in the below image. First, we would need to right click on the webpage, and then click on the Inspect button in the Chrome browser. Then, the corresponding HTML code for the whole page would be visible. For investigating a single element on a page, we would need to click on the left upward arrow, available to the top of the visible HTML code as highlighted below.
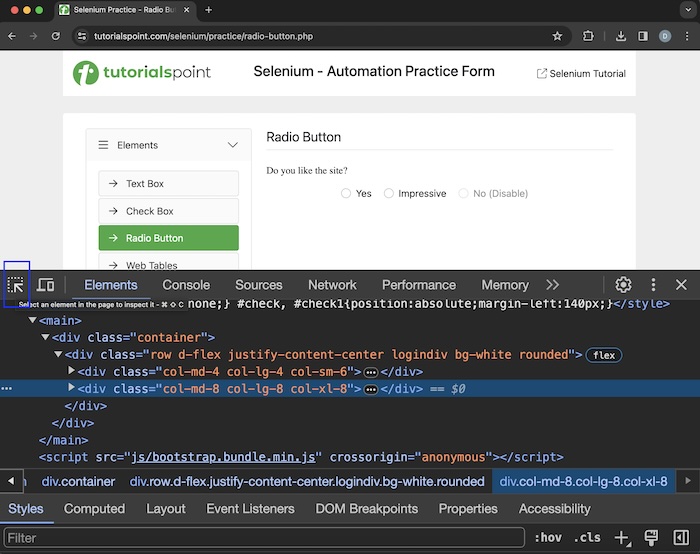
Once we had clicked and pointed the arrow to the radio button(highlighted in the below image), its HTML code was visible, both reflecting the input tagname(enclosed in <>) and value of type attribute as radio.
<input class="form-check-input" type="radio" name="tab" value="igottwo" onclick="show2();">
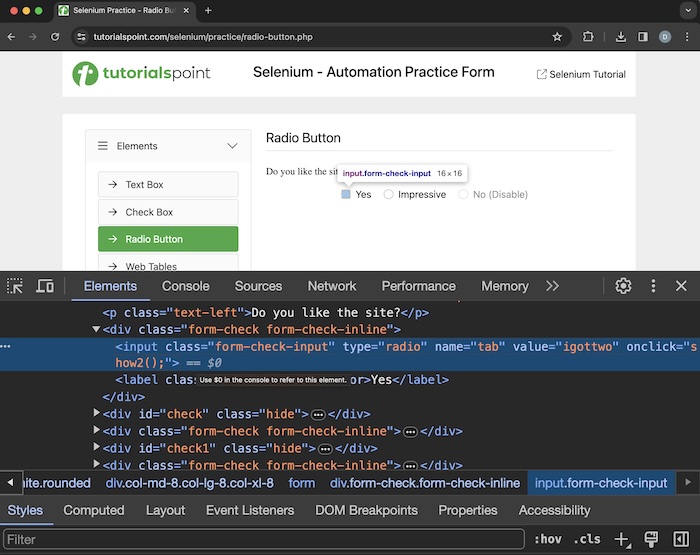
Let us take an example of the above page, where we would click one of the radio buttons with the help of the click() method. Then we would verify if the radio button is available, enabled and selected using the above discussed methods.
Syntax
Webdriver driver = new ChromeDriver(); WebElement radioBtn = driver.findElement(By.xpath("value of xpath")); radioBtn.click(); boolean result = radioBtn.isSelected(); boolean result1 = radioBtn.isDisplayed(); boolean result2 = radioBtn.isEnabled();
Example
Code Implementation on ValidatingRadioButtons.java class file.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class ValidatingRadioButtons { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will click radio button driver.get("https://www.tutorialspoint.com/selenium/practice/radio-button.php"); // identify radio button then click WebElement radiobtn = driver.findElement (By.xpath("/html/body/main/div/div/div[2]/form/div[1]/input")); radiobtn.click(); // verify if radio button is selected boolean result = radiobtn.isSelected(); System.out.println("Checking if a radio button is selected: " + result); // verify if radio button is displayed boolean result1 = radiobtn.isDisplayed(); System.out.println("Checking if a radio button is displayed: " + result1); // verify if radio button is enabled boolean result2 = radiobtn.isEnabled(); System.out.println("Checking if a radio button is enabled: " + result2); // identify another radio button WebElement radiobtn1 = driver.findElement (By.xpath("/html/body/main/div/div/div[2]/form/div[5]/input")); // verify if radio button is disabled boolean result3 = radiobtn1.isEnabled(); System.out.println("Checking if the other radio button is disabled: " + result3); // verify if radio button is unselected boolean result4 = radiobtn1.isEnabled(); System.out.println("Checking if the other radio button is unselected: " + result4); // Closing browser driver.quit(); } }
Output
Checking if a radio button is selected: true Checking if a radio button is displayed: true Checking if a radio button is enabled: true Checking if the other radio button is disabled: false Checking if the other radio button is unselected: false Process finished with exit code 0
In the above example, we had first clicked on a radio button, and then verified if the radio button was available, selected, and enabled in the console with the message - Checking if a radio button is selected: true, Checking if a radio button is displayed: true, Checking if a radio button is enabled: true. Then we had validated for another radio button if it is disabled and unselected with the message in the console - Checking if the other radio button is disabled: false, and Checking if the other radio button is unselected: false.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
In HTML terminology, every radio button is identified by the tagname called input. We can get the tag name of an element with the help of the getTagName() method.
Syntax
Webdriver driver = new ChromeDriver(); WebElement radioBtn = driver.findElement(By.xpath("value of xpath”)); String text = radioBtn.getTagName();
Example
Code Implementation on RadioButtonTagNames.java class file.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class RadioButtonTagNames { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will click radio button driver.get("https://www.tutorialspoint.com/selenium/practice/radio-button.php"); // identify radio button then click WebElement radiobtn = driver.findElement (By.xpath("/html/body/main/div/div/div[2]/form/div[1]/input")); String text = radiobtn.getTagName(); System.out.println("Get the radio button tagname: " + text); // Closing browser driver.quit(); } }
Output
Get the radio button tagname: input Process finished with exit code 0
In the above example, we had obtained the tagname of the radio button in the console with the message - Get the radio button tagname: input.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Let us take another example in the below image, where we would get the height, width, x, and y coordinates of the text Selenium - Automation Practice Form on the page. To get the x, and y coordinates of an element, we would use the getRect() method.
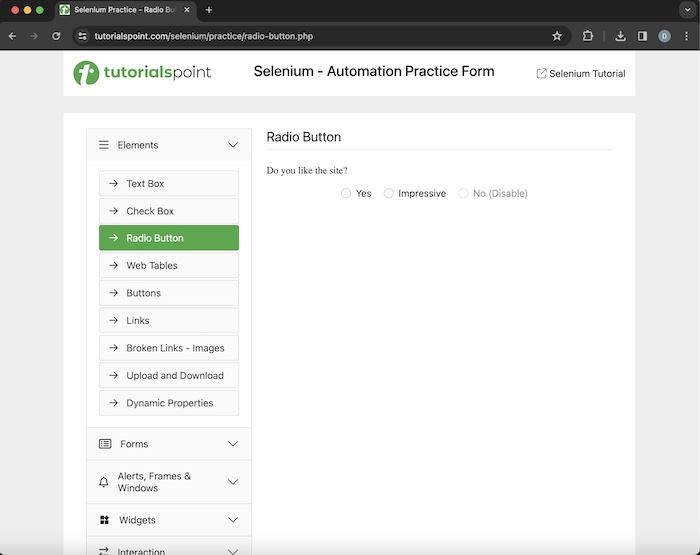
Syntax
Webdriver driver = new ChromeDriver(); WebElement e = driver.findElement(By.xpath("value of xpath”)); Rectangle res = e.getRect(); System.out.println("Getting height: " + res.getHeight()); System.out.println("Getting width: " + res.getWidth()); System.out.println("Getting x-coordinates: " + res.getX()); System.out.println("Getting y-coordinates: " + res.getY());
Example
Code Implementation on ElementPositions.java class file.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.Rectangle; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class ElementPositions { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage driver.get("https://www.tutorialspoint.com/selenium/practice/radio-button.php"); // Identify the element with xpath locator WebElement e = driver.findElement(By.xpath("/html/body/div/header/div[2]/h1")); Rectangle res = e.getRect(); // Getting height, width, x, and y coordinates of element System.out.println("Getting height: " + res.getHeight()); System.out.println("Getting width: " + res.getWidth()); System.out.println("Getting x-cordinates: " + res.getX()); System.out.println("Getting y-cordinates: " + res.getY()); // Closing browser driver.quit(); } }
Output
Getting height: 29 Getting width: 395 Getting x-cordinates: 453 Getting y-cordinates: 18 Process finished with exit code 0
In the above example, we had obtained the height, width, x and y coordinates of the text in the console with the message - Getting height: 29, Getting width: 395, Getting x-cordinates: 453, and Getting y-cordinates: 18.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Let us take another example in the below image, where we would background color the highlighted element Login (depicted in Styles section). We would take the help of the getCssValue() method and pass background-color as a parameter to that method.
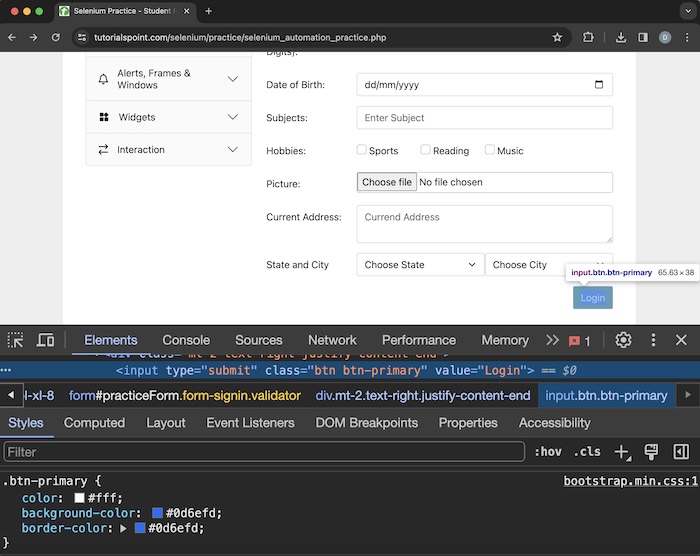
Syntax
Webdriver driver = new ChromeDriver(); WebElement e = driver.findElement(By.xpath("value of xpath”)); String c = e.getCssValue("background-color");
Example
Code Implementation on ElementsCSSProperty.java class file.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class ElementsCSSProperty { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Identify the element with xpath locator WebElement e = driver.findElement(By.xpath("//*[@id='practiceForm']/div[11]/input")); // Getting background color of the element System.out.println ("Getting background color of element: " + e.getCssValue("background-color")); // Closing browser driver.quit(); } }
Output
Getting background color of element: rgba(13, 110, 253, 1) Process finished with exit code 0
In the above example, we had obtained the background of an element on the page in rgb format in the console with the message - Getting background color of element: rgba(13, 110, 253, 1).
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Let us take an example of the below page, where we would first enter the text Selenium in the input box with the help of the sendKeys() method. Then we would fetch the runtime value of the element associated with DOM using the getAttribute() method pass value as a parameter to that method.
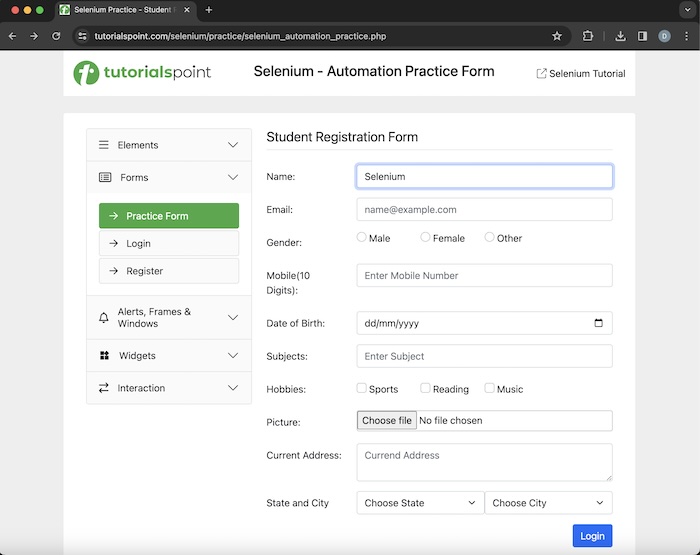
Syntax
Webdriver driver = new ChromeDriver(); WebElement e = driver.findElement(By.xpath("value of xpath”)); String c = e.getAttribute("value");
Example
Code Implementation on HandlingInputBox.java class file.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class HandlingInputsBox { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Identify the element with xpath locator WebElement e = driver.findElement(By.xpath("//*[@id='name']")); // enter text in input box e.sendKeys("Selenium"); // Get the value entered String text = e.getAttribute("value"); System.out.println("Entered text is: " + text); // clear the text entered e.clear(); // Get no text after clearing text String text1 = e.getAttribute("value"); System.out.println("Get text after clearing: " + text1); // Closing browser driver.quit(); } }
Output
Entered text is: Selenium Get text after clearing: Process finished with exit code 0
In the above example, we had first entered the text Selenium in the input box, and also retrieved the value entered in the console with the message- Entered text is: Selenium. Then cleared the value entered and there was no value in the input box after clearing up the text. Hence, we had also received the message in the console: Get text after clearing:.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Let us take an example of the below page, where we would obtain the text of the highlighted element - Selenium - Automation Practice Form with the help of the getText() method in Selenium Webdriver.
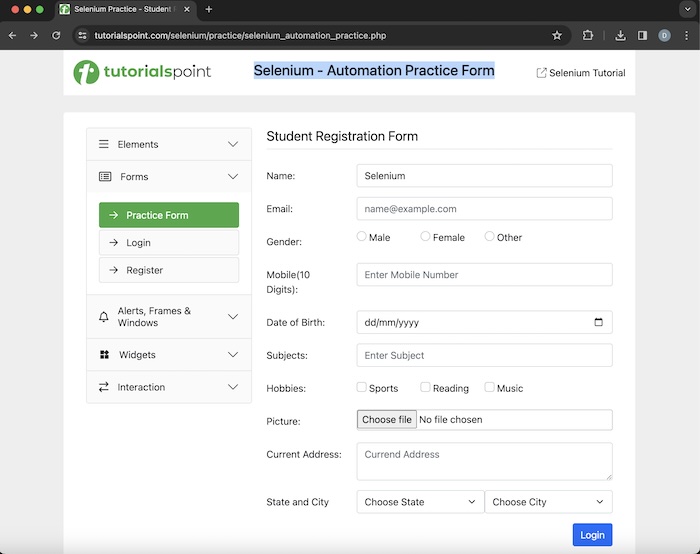
Syntax
Webdriver driver = new ChromeDriver(); WebElement e = driver.findElement(By.xpath("value of xpath”)); String c = e.getText();
Example
Code Implementation on ElementsText.java class file.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class ElementsText { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Identify the element with xpath locator WebElement e = driver.findElement(By.xpath("/html/body/div/header/div[2]/h1")); // Getting text of the element System.out.println("Getting element text: " + e.getText()); // Closing browser driver.quit(); } }
Output
Getting element text: Selenium - Automation Practice Form Process finished with exit code 0
In the above example, we obtained the text of an element in the console with the message - Getting element text: Selenium - Automation Practice Form.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Thus, in this tutorial, we had discussed different web element commands used in the Selenium Webdriver.