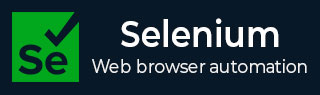
- Selenium Tutorial
- Selenium - Home
- Selenium - Overview
- Selenium - Components
- Selenium - Automation Testing
- Selenium - Environment Setup
- Selenium - Remote Control
- Selenium IDE Tutorial
- Selenium - IDE Introduction
- Selenium - Features
- Selenium - Limitations
- Selenium - Installation
- Selenium - Creating Tests
- Selenium - Creating Script
- Selenium - Store Variables
- Selenium - Alerts & Popups
- Selenium - Selenese Commands
- Selenium - Actions Commands
- Selenium - Accessors Commands
- Selenium - Assertions Commands
- Selenium - Assert/Verify Methods
- Selenium - Locating Strategies
- Selenium - Script Debugging
- Selenium - Verification Points
- Selenium - Pattern Matching
- Selenium - JSON Data File
- Selenium - Browser Execution
- Selenium - User Extensions
- Selenium - Code Export
- Selenium - JavaScript Functions
- Selenium - Plugins
- Selenium WebDriver Tutorial
- Selenium - Introduction
- Selenium WebDriver vs RC
- Selenium - Installation
- Selenium - First Test Script
- Selenium - Driver Sessions
- Selenium - Browser Options
- Selenium - Chrome Options
- Selenium - Edge Options
- Selenium - Firefox Options
- Selenium - Safari Options
- Selenium Web Elements
- Selenium - File Upload
- Selenium - Locator Strategies
- Selenium - Relative Locators
- Selenium - Finders
- Selenium - Find All Links
- Selenium - User Interactions
- Selenium - WebElement Commands
- Selenium - Browser Interactions
- Selenium - Browser Commands
- Selenium - Browser Navigation
- Selenium - Alerts & Popups
- Selenium - Handling Forms
- Selenium - Windows and Tabs
- Selenium - Handling Links
- Selenium - Input Boxes
- Selenium - Radio Button
- Selenium - Checkboxes
- Selenium - Dropdown Box
- Selenium - Handling IFrames
- Selenium - Handling Cookies
- Selenium - Date Time Picker
- Selenium - Dynamic Web Tables
- Selenium - Actions Class
- Selenium - Action Class
- Selenium - Keyboard Events
- Selenium - Key Up/Down
- Selenium - Copy and Paste
- Selenium - Handle Special Keys
- Selenium - Mouse Events
- Selenium - Drag and Drop
- Selenium - Scroll Operations
- Selenium - Waiting Strategies
- Selenium - Explicit/Implicit Wait
- Selenium - Support Features
- Selenium - Multi Select
- Selenium - Wait Support
- Selenium - Select Support
- Selenium - Color Support
- Selenium - Errors & Logging
- Selenium - Logging
- Selenium - Exception Handling
- Selenium - Miscellaneous
- Selenium - Handling Ajax Calls
- Selenium - JSON Data File
- Selenium - CSV Data File
- Selenium - Excel Data File
- Selenium - Cross Browser Testing
- Selenium - Multi Browser Testing
- Selenium - Multi Windows Testing
- Selenium - JavaScript Executor
- Selenium - Headless Execution
- Selenium - Capture Screenshots
- Selenium - Capture Videos
- Selenium - Page Object Model
- Selenium - Page Factory
- Selenium - Record & Playback
- Selenium - Frameworks
- Selenium - Browsing Context
- Selenium Grid Tutorial
- Selenium - Overview
- Selenium - Architecture
- Selenium - Components
- Selenium - Configuration
- Selenium - Create Test Script
- Selenium - Test Execution
- Selenium - Endpoints
- Selenium - Customizing a Node
- Selenium Reporting Tools
- Selenium - Reporting Tools
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & other Technologies
- Selenium - Java Tutorial
- Selenium - Python Tutorial
- Selenium - C# Tutorial
- Selenium - Javascript Tutorial
- Selenium - Kotlin Tutorial
- Selenium - Ruby  Tutorial
- Selenium - Maven & Jenkins
- Selenium - Database Testing
- Selenium - LogExpert Logging
- Selenium - Log4j Logging
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash Testing
- Selenium - Apache Ant
- Selenium - Github
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium Miscellaneous Concepts
- Selenium - Automation Frameworks
- Selenium - Keyword Driven Framework
- Selenium - Data Driven Framework
- Selenium - Hybrid Driven Framework
- Selenium - SSL Certificate Error
- Selenium - Alternatives
- Selenium Useful Resources
- Selenium - Quick Guide
- Selenium - Useful Resources
- Selenium - Automation Practice
- Selenium - Discussion
Selenium WebDriver - Exception Handling
Exception Handling can be performed using the Selenium Webdriver. While developing our tests, we should ensure that the scripts can continue their execution even if there is an error. An exception is similar to an error encountered while our tests are triggered for execution.
If an exception occurs due to an element not found or if the expected result doesn't match with actuals or for any other reasons, our code should generate an exception and end the test in a logical way rather than terminating the script abruptly. Exceptions are of two types −
Checked Exceptions − These exceptions can be taken care of while developing the code prior compilation of it.
Unchecked Exceptions − These exceptions can be detected only at runtime and are more difficult to handle than the checked exceptions.
The method of identifying and solving the unchecked exceptions is known as exception handling. Some of the exceptions available in Selenium are −
ElementNotVisibleException − This exception is generated when an element is available in DOM, but it is invisible. Hence no actions can be done on it.
ElementNotInteractableException − This exception is generated when an element is available in DOM. However, when performing action on it, another element is getting affected.
ElementClickInterceptedException − This exception is generated when an element click command could not be achieved. This is because the element which is receiving the event is hiding the element that requested the click operation.
ElementNotSelectableException − This exception is generated when an element which is unselectable is made an attempt to select
InsecureCertificateException − This exception is generated when a navigation is responsible to hit a certificate warning. This resulted in creating an expired and incorrect certificate of TLS.
ErrorInResponseException − This exception is generated due to an error on the server side.
ImeActivationFailedException − This exception is generated due to a failed activation of the IME engine.
ImeNotAvailableException − This exception is generated if IME support is unavailable.
InvalidElementStateException − This exception is generated if a command remains incomplete as the element state is not valid
InvalidArgumentException − This exception is generated if a command argument is not valid.
InvalidCoordinatesException − This exception is generated if the coordinates for operations are not valid.
InvalidCookieDomainException − This exception is generated for adding a cookie under a different domain and not in the present URL.
InvalidSwitchToTargetException − This exception is generated when the target window or frame to be switched is nonexistent.
InvalidSelectorException − This exception is generated if the selector to identify an element fails to get a WebElement.
MoveTargetOutOfBoundsException − This exception is generated when the target to the method ActionsChains move() is not valid.
InvalidSessionIdException − This exception is generated if the provided session id is either inactive or nonexistent and is not a part of the active sessions.
NoSuchFrameException − This exception is generated when the target frame to be switched is nonexistent.
NoAlertPresentException − This exception is generated when the target alert to be switched is nonexistent.
NoSuchCookieException − This exception is generated when there is no matching cookie amongst the cookies of the present browsing active content.
NoSuchAttributeException − This exception is generated when an element attribute is missing.
UnableToSetCookieException − This exception is generated when a driver is not able to set a cookie.
NoSuchWindowException − This exception is generated when the target window to be switched is nonexistent.
TimeoutException − This exception is generated when a command execution does not complete in a time range.
StaleElementReferenceException − This exception is generated when an element reference is currently stale.
UnexpectedTagNameException − This exception is generated when an assisting class did not find the proper web element.
UnexpectedAlertPresentException − This exception is generated when an unexpected alert has come up.
NoSuchElementException − This exception is a child class of NotFoundException class. It is generally thrown by the findElement() method, when an element cannot be identified in DOM.
SessionNotFoundException − This exception is thrown when the driver makes an attempt to do something on the web page while the browser session is terminated or closed.
WebDriverException − This exception is thrown when the driver makes an attempt to work straight away just after closure of the driver session.
Let us discuss some of the ways to handle exceptions −
Use try-catch block
The try indicates the beginning of the block and catch is placed just after the try block to resolve the exception.
Syntax
try { //Perform Action } catch(Exception e1) { //Catch block 1 }
Use try and multiple catch blocks
Some pieces of code can produce multiple exceptions. Each catch block is used to handle a single type of exception.
Syntax
try { //Perform Action } catch(Exception e1) { //Catch block 1 } catch(Exception e2) { //Catch block 2 }
Use throw and throws
The throw keyword is used to generate a customized exception. If an exception is thrown without handling it, we should have the throws keyword mentioned in the method signature.
Syntax
public static void errorHandling() throws Exception try { //Perform Action } catch(Exception e) { throw(e); }
Use try-catch-finally block
The try indicates the beginning of the block and catch is placed just after the try block to resolve the exception. The finally block of code executes regardless of whether the script had thrown an exception or NOT.
Syntax
try { //Perform Action } catch(Exception e1) { //Catch block 1 } finally { //The finally block always executes. }
printStackTrace() − This provides information about the exception, stack trace, and other critical information about the execution.
toString() − This describes the exception name and its purpose.
getMessage() − This describes the exception.
Let us take an example where an error got encountered in the program and execution was halted in between since there was no exception handling in the code.
Example
Code Implementation on ExceptionsEncountered.java class file.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class ExceptionsEncountered { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // launch an application and open a URL driver.get("https://www.tutorialspoint.com/selenium/practice/auto-complete.php"); // identify element with incorrect xpath value WebElement l = driver.findElement(By.xpath("//*[@id='tag']")); // enter text l.sendKeys("Selenium"); // Quitting browser driver.quit(); } }
Output
Exception in thread "main" org.openqa.selenium.NoSuchElementException: no such element: Unable to locate element: {"method":"xpath","selector":"//*[@id='tag']"} (Session info: chrome=121.0.6167.160) For documentation on this error, please visit: https://www.selenium.dev/documentation/webdriver/troubleshooting/errors#no-such-element-exception Process finished with exit code 1
In the above example, we have received the NoSuchElementException. As exception handling was not applied in the above implementation, the execution halted immediately after the error was encountered.
Finally, the message Process finished with exit code 1 was received, signifying unsuccessful execution of the code.
Let us see how to add the exception handling in the above test using the try-catch and finally block.
Example
Code Implementation on ExceptionHandlingEncountered.java class file.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.NoSuchElementException; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class ExceptionHandlingEncountered { public static void main(String[] args) { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // launch an application and open a URL driver.get("https://www.tutorialspoint.com/selenium/practice/auto-complete.php"); //try-catch finally block try { // identify element with incorrect xpath value WebElement l = driver.findElement(By.xpath("//*[@id='tag']")); // enter text l.sendKeys("Selenium"); } catch (NoSuchElementException e){ System.out.println("Catch block executed after try block"); } // finally block finally { System.out.println("finally block executed after try - catch block"); } // Quitting browser driver.quit(); } }
Output
Catch block executed after try block finally block executed after try - catch block Process finished with exit code 0
In this tutorial, we had discussed how to perform exception handling using Selenium Webdriver.