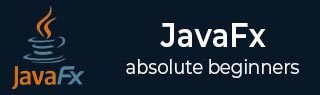
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Environment
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Path Objects
- JavaFX - Path Objects
- JavaFX - LineTo Path Object
- JavaFX - HLineTo Path Object
- JavaFX - VLineTo Path Object
- JavaFX - QuadCurveTo Path Object
- JavaFX - CubicCurveTo Path Object
- JavaFX - ArcTo Path Object
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX - Linear Gradient Pattern
- JavaFX - Radial Gradient Pattern
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX - Color Adjust Effect
- JavaFX - Color input Effect
- JavaFX - Image Input Effect
- JavaFX - Blend Effect
- JavaFX - Bloom Effect
- JavaFX - Glow Effect
- JavaFX - Box Blur Effect
- JavaFX - GaussianBlur Effect
- JavaFX - MotionBlur Effect
- JavaFX - Reflection Effect
- JavaFX - SepiaTone Effect
- JavaFX - Shadow Effect
- JavaFX - DropShadow Effect
- JavaFX - InnerShadow Effect
- JavaFX - Lighting Effect
- JavaFX - Light.Distant Effect
- JavaFX - Light.Spot Effect
- JavaFX - Point.Spot Effect
- JavaFX - DisplacementMap
- JavaFX - PerspectiveTransform
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX - Rotation Transformation
- JavaFX - Scaling Transformation
- JavaFX - Translation Transformation
- JavaFX - Shearing Transformation
- JavaFX Animations
- JavaFX - Animations
- JavaFX - Rotate Transition
- JavaFX - Scale Transition
- JavaFX - Translate Transition
- JavaFX - Fade Transition
- JavaFX - Fill Transition
- JavaFX - Stroke Transition
- JavaFX - Sequential Transition
- JavaFX - Parallel Transition
- JavaFX - Pause Transition
- JavaFX - Path Transition
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - TextField
The text field is a graphical user interface component used to accept user input in the form of text. It is the most easiest way of interaction between system and user. We can find a textfield inside a form or dialog window as shown in the below figure −
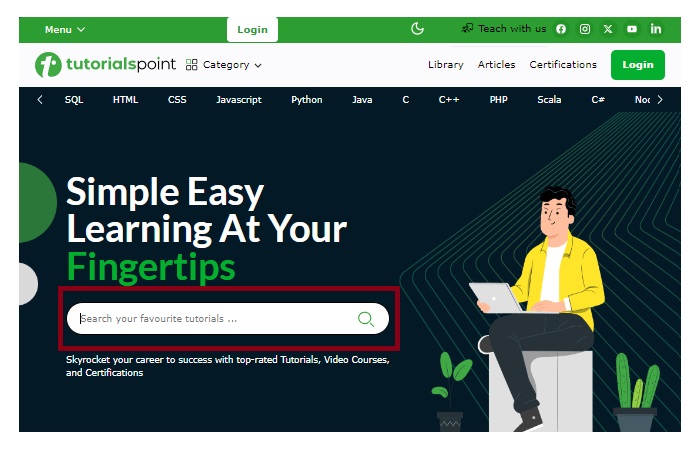
TextField in JavaFX
In JavaFX, the TextField class represents the text field which is a part of the package named javafx.scene.control. Using this we can accept input from the user and read it to our application. This class inherits the TextInputControl which is the base class of all the text controls class. To create a text field, instantiate the TextField class using any of the below constructors −
TextField() − This constructor will create an empty textfield.
TextField(String str) − It is the parameterized constructor which constructs a textfield with the specified text.
Note that in the latest versions of JavaFX, the TextField class accepts only a single line.
Example
In the following JavaFX program, we will create a hyperlink as a text. Save this code in a file with the name JavafxTextfield.java.
import javafx.application.Application; import javafx.geometry.Insets; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.scene.control.TextField; import javafx.scene.layout.HBox; import javafx.scene.paint.Color; import javafx.stage.Stage; public class JavafxTextfield extends Application { public void start(Stage stage) { //Creating nodes TextField textField1 = new TextField("Enter your name"); TextField textField2 = new TextField("Enter your e-mail"); //Creating labels Label label1 = new Label("Name: "); Label label2 = new Label("Email: "); //Adding labels for nodes HBox box = new HBox(5); box.setPadding(new Insets(25, 5 , 5, 50)); box.getChildren().addAll(label1, textField1, label2, textField2); //Setting the stage Scene scene = new Scene(box, 595, 150, Color.BEIGE); stage.setTitle("Text Field Example"); stage.setScene(scene); stage.show(); } public static void main(String args[]){ launch(args); } }
To compile and execute the saved Java file from the command prompt, use the following commands −
javac --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxTextfield.java java --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxTextfield
Output
When we execute, the above program will generate the following output.
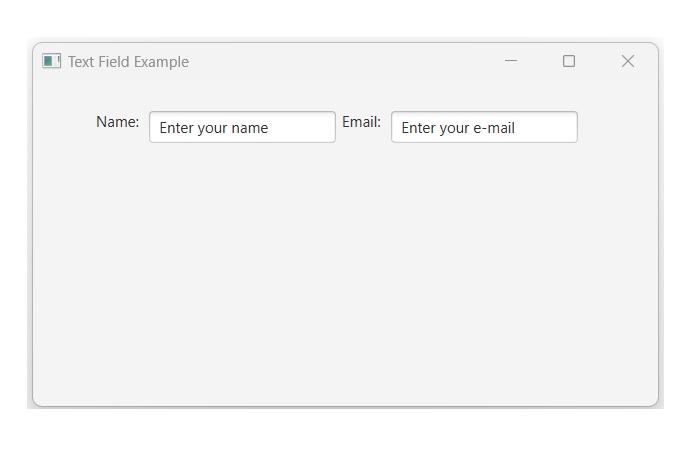