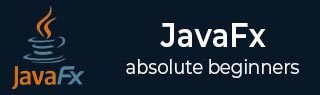
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX Animations
- JavaFX - Animations
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - RadioButton
A button is a component, which performs an action like submit and login when pressed. It is usually labeled with a text or an image specifying the respective action. A radio button is a type of button, which is circular in shape. It has two states, selected and deselected. The figure below shows a set of radio buttons −
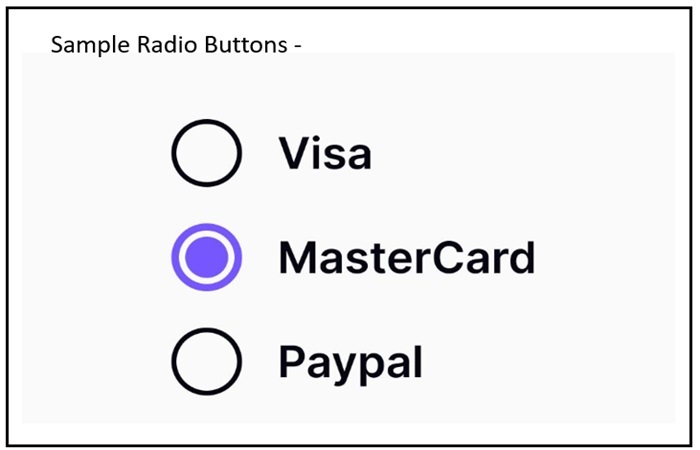
RadioButton in JavaFX
In JavaFX, the RadioButton class represents a radio button which is a part of the package named javafx.scene.control. It is the subclass of the ToggleButton class. Action is generated whenever a radio button is pressed or released. Generally, radio buttons are grouped using toggle groups, where you can only select one of them. We can set a radio button to a group using the setToggleGroup() method.
To create a radio button, use the following constructors −
RadioButton() − This constructor will create radio button without any label.
RadioButton(String str) − It is the parameterized constructor which constructs a radio button with the specified label text.
Example
Following is the JavaFX program that will create a RadioButton. Save this code in a file with the name JavafxRadiobttn.java.
import javafx.application.Application; import javafx.geometry.Insets; import javafx.scene.Scene; import javafx.scene.control.RadioButton; import javafx.scene.control.ToggleGroup; import javafx.scene.layout.VBox; import javafx.scene.paint.Color; import javafx.stage.Stage; public class JavafxRadiobttn extends Application { @Override public void start(Stage stage) { //Creating toggle buttons RadioButton button1 = new RadioButton("Java"); RadioButton button2 = new RadioButton("Python"); RadioButton button3 = new RadioButton("C++"); //Toggle button group ToggleGroup group = new ToggleGroup(); button1.setToggleGroup(group); button2.setToggleGroup(group); button3.setToggleGroup(group); //Adding the toggle button to the pane VBox box = new VBox(5); box.setFillWidth(false); box.setPadding(new Insets(5, 5, 5, 50)); box.getChildren().addAll(button1, button2, button3); //Setting the stage Scene scene = new Scene(box, 400, 300, Color.BEIGE); stage.setTitle("Toggled Button Example"); stage.setScene(scene); stage.show(); } public static void main(String args[]){ launch(args); } }
Compile and execute the saved Java file from the command prompt using the following commands −
javac --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxRadiobttn.java java --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxRadiobttn
Output
On executing, the above program will generate the following output −
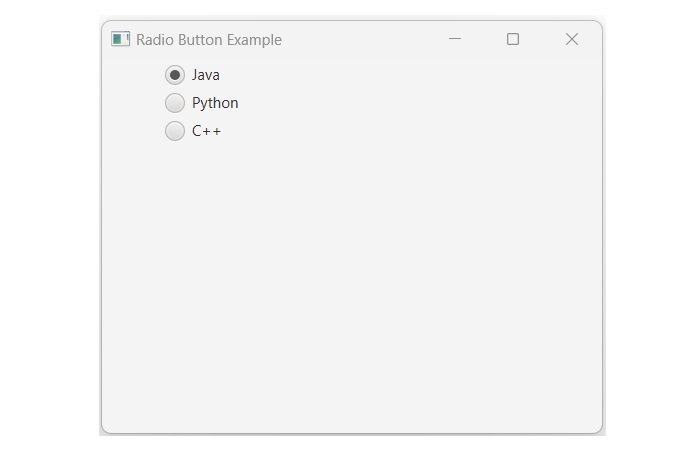