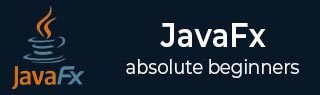
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX Animations
- JavaFX - Animations
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - DatePicker
The Date picker is a control that allows the user to select a date from a graphical calendar. It can be customized to display different date formats, restrict the range of selectable dates, and handle various events related to date selection. The figure below shows a typical datepicker −
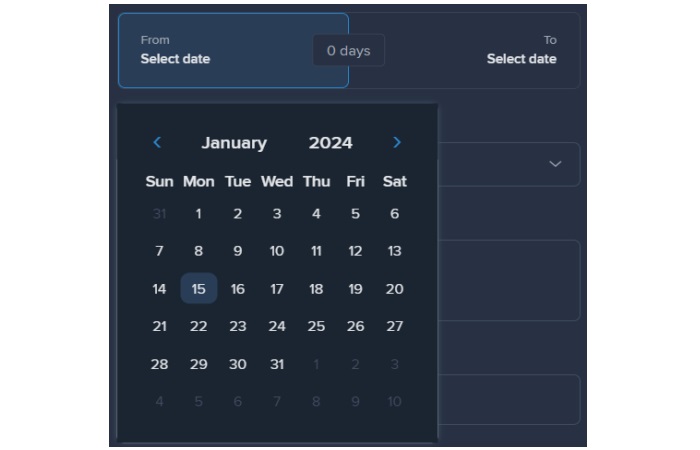
Creating DatePicker in JavaFX
In JavaFX, the the date picker is represented by a class named DatePicker. This class belongs to the package javafx.scene.control. By instantiating this class, we can create a date picker in JavaFX. Constructors of the DatePicker class are listed below −
DatePicker() − It is the default constructor that constructs a date picker without any pre-defined date.
DatePicker(LocalDate date) − It creates a new date picker with the specified date.
We can use any of the above mentioned constructors to embed a DatePicker within JavaFX application. The most freuently used is the default constructor of the DatePicker class which does not accept any arguments. Once we instantiate this class, our next step would be setting the initial date value. Following that, instantiate any layout pane to hold the DatePicker. Lastly, set the scene and stage to display the date picker on the screen.
Example
The following JavaFX program demonstrates how to create a datepicker in a JavaFX application. Save this code in a file with the name DatepickerDemo.java.
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.DatePicker; import javafx.scene.layout.VBox; import javafx.stage.Stage; import java.time.LocalDate; import javafx.scene.control.Label; import javafx.scene.paint.Color; import javafx.geometry.Pos; public class DatepickerDemo extends Application { @Override public void start(Stage stage) { // Creating a Label Label label = new Label("Please Enter preferred Date: "); // Create a DatePicker and set its initial value DatePicker datePicker = new DatePicker(); datePicker.setValue(LocalDate.of(2020, 1, 1)); // Create a Label to display the selection Label selectLabel = new Label(); selectLabel.setTextFill(Color.RED); // Add a listener to the value property of the DatePicker datePicker.valueProperty().addListener((observable, oldValue, newValue) -> { // Print the selected date selectLabel.setText("You selected: " + newValue); }); // Creating a VBox to hold all controls VBox root = new VBox(); root.setAlignment(Pos.CENTER); root.setSpacing(10); root.getChildren().addAll(label, datePicker, selectLabel); // Create a Scene and set it to the Stage Scene scene = new Scene(root, 400, 300); stage.setTitle("DatePicker in JavaFX"); stage.setScene(scene); stage.show(); } public static void main(String[] args) { launch(args); } }
To compile and execute the saved Java file from the command prompt, use the following commands −
javac --module-path %PATH_TO_FX% --add-modules javafx.controls DatepickerDemo.java java --module-path %PATH_TO_FX% --add-modules javafx.controls DatepickerDemo
Output
When we execute the above code, it will generate the following output.
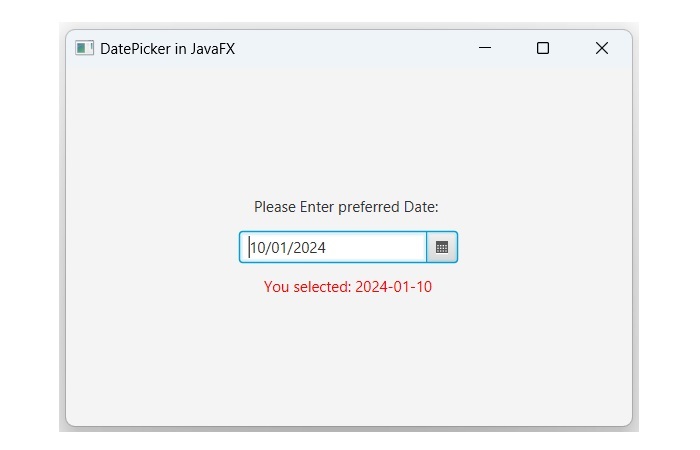
Showing week numbers in the Date Picker
By default, the DatePicker does not show week numbers in the calendar. However, if there is a need to display week numbers along with the date, then we can set the setShowWeekNumbers() method to true as shown in the next example. Save this code in a file with the name DatepickerDemo.java.
Example
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.DatePicker; import javafx.scene.layout.VBox; import javafx.stage.Stage; import java.time.LocalDate; import javafx.scene.control.Label; import javafx.scene.paint.Color; import javafx.geometry.Pos; public class DatepickerDemo extends Application { @Override public void start(Stage stage) { // Creating a Label Label label = new Label("Please Enter preferred Date: "); // Create a DatePicker and set its initial value DatePicker datePicker = new DatePicker(); datePicker.setValue(LocalDate.of(2024, 1, 1)); datePicker.setShowWeekNumbers(true); // Create a Label to display the selection Label selectLabel = new Label(); selectLabel.setTextFill(Color.RED); // Add a listener to the value property of the DatePicker datePicker.valueProperty().addListener((observable, oldValue, newValue) -> { // Print the selected date selectLabel.setText("You selected: " + newValue); }); // Creating a VBox to hold all controls VBox root = new VBox(); root.setAlignment( Pos.BASELINE_CENTER); root.setSpacing(10); root.getChildren().addAll(label, datePicker, selectLabel); // Create a Scene and set it to the Stage Scene scene = new Scene(root, 400, 300); stage.setTitle("DatePicker in JavaFX"); stage.setScene(scene); stage.show(); } public static void main(String[] args) { launch(args); } }
Compile and execute the saved Java file from the command prompt using the following commands −
javac --module-path %PATH_TO_FX% --add-modules javafx.controls DatepickerDemo.java java --module-path %PATH_TO_FX% --add-modules javafx.controls DatepickerDemo
Output
On executing, the above program generates a JavaFX window displaying a DatePicker with the week numbers as shown below −
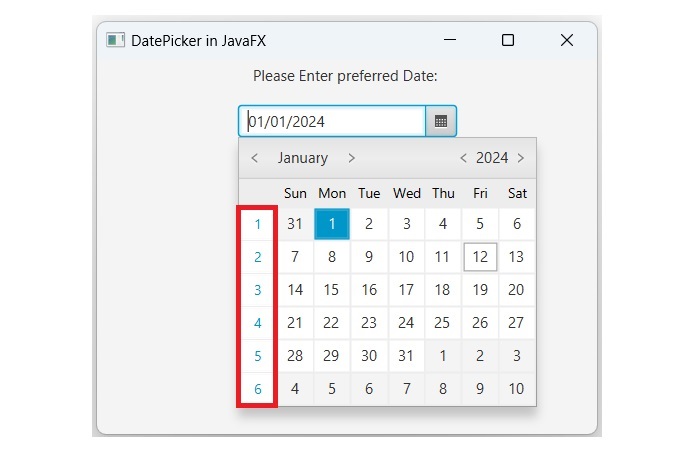