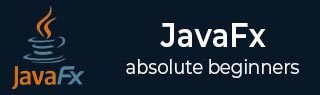
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX Animations
- JavaFX - Animations
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - Alert
An alert refers to a pop-up window or dialog box that appears on the screen to inform users about an error or any event. The information of an alert is not limited to the error message only, it can be any message including a simple "hello". For instance, the figure below illustrates a notification regarding folder deletion −
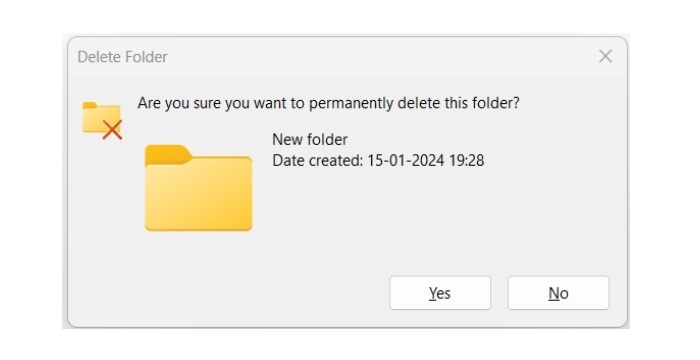
Alert in JavaFX
In JavaFX, an alert is represented by a class named Alert. This class belongs to the package javafx.scene.control. By instantiating this class, we can create an alert in JavaFX. Additionally, we need to pass an Alert.AlertType enumeration value to the constructor. This value determines the default properties of the dialog, such as the title, header, graphic, and buttons. Constructors of the Alert class are listed below −
Alert(Alert.AlertType typeOfalert) − It is used to construct an Alert with the sepcified alert type.
Alert(Alert.AlertType typeOfalert, String str, ButtonType buttons) − It constructs an Alert with the sepcified alert type, predefined text and button type.
How to create an Alert in JavaFX?
Follow the steps given below to create an alert in JavaFX.
Step 1: Instantiate the Alert class
To create an alert, instantiate the Alert class and pass the Alert.AlertType enumeration value to its constructor as a parameter value as shown in the below code −
// Creating an Alert Alert alert = new Alert(AlertType.INFORMATION);
Step 2: Set the Title for Alert Box
Use the setTitle() method to set a suitable title for the alert box as shown in the following code block −
// setting the title of alert box alert.setTitle("Alert Box");
Step 3: Set the Header text for Alert Box
The setHeaderText() method is used to set the header of the alert box. We are setting the Header text to "null" with the help of following code block −
// setting header text alert.setHeaderText(null);
Step 4: Set the Content text for Alert Box
To set the content text for an alert box, use the setContentText() method. It accepts a text of type String as shown in the below code −
// setting content text alert.setContentText("Showing an Alert in JavaFX!");
Step 5: Launch the Application
Once the alert is created and its properties are set, create a button that, when clicked, will display the alert. Next, define a layout pane such as VBox and HBox by passing the Button object to its constructor. Then, set the Scene and Stage. Lastly, launch the application with the help of the launch() method.
Example
The following JavaFX program demonstrates how to generate alert in a JavaFX application. Save this code in a file with the name ShowAlert.java.
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Alert; import javafx.scene.control.Alert.AlertType; import javafx.scene.control.Button; import javafx.scene.control.Label; import javafx.scene.layout.VBox; import javafx.stage.Stage; import javafx.geometry.Pos; public class ShowAlert extends Application { @Override public void start(Stage stage) { // Creating a Label Label label = new Label("On clicking the below button, it will display an alert...."); // Creating a Button Button button = new Button("Show Alert"); // Creating an Alert Alert alert = new Alert(AlertType.INFORMATION); alert.setTitle("Alert Box"); alert.setHeaderText(null); alert.setContentText("Showing an Alert in JavaFX!"); // Setting the Button's action button.setOnAction(e -> alert.showAndWait()); // Create a VBox to hold the Label and Button VBox vbox = new VBox(label, button); vbox.setAlignment(Pos.CENTER); // Create a Scene with the VBox as its root node Scene scene = new Scene(vbox, 400, 300); // Set the Title of the Stage stage.setTitle("Alert in JavaFX"); // Set the Scene of the Stage stage.setScene(scene); // Display the Stage stage.show(); } public static void main(String[] args) { launch(args); } }
To compile and execute the saved Java file from the command prompt, use the following commands −
javac --module-path %PATH_TO_FX% --add-modules javafx.controls ShowAlert.java java --module-path %PATH_TO_FX% --add-modules javafx.controls ShowAlert
Output
When we execute the above code, it will generate the following output.
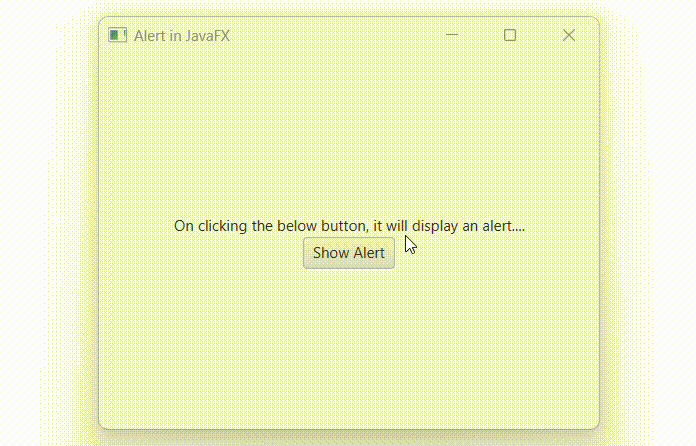
Types of Alert in JavaFX
The Alert class is a subclass of the Dialog class, and it provides support for a number of pre-built dialog (or alert) types namely confirmation, warning, information, error, and none. By changing the value of Alert.AlertType enumeration, we can use these different alert types.
Example
In the following example, we are going to demonstrates the types of Alert in JavaFX. Save this code in a file with the name JavafxAlert.java.
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Alert; import javafx.scene.control.Alert.AlertType; import javafx.scene.control.Button; import javafx.scene.control.Label; import javafx.scene.layout.VBox; import javafx.scene.layout.HBox; import javafx.stage.Stage; import javafx.geometry.Pos; import javafx.geometry.Insets; public class JavafxAlert extends Application { @Override public void start(Stage stage) { // Creating a Label Label label = new Label("Types of alert in JavaFX..."); // Creating Buttons Button cnfrmButtn = new Button("Confirm"); Button infrmButtn = new Button("Inform"); Button warngButtn = new Button("Warning"); Button errorButtn = new Button("Error"); // Creating information Alert and setting Button's action Alert infrmAlert = new Alert(AlertType.INFORMATION); infrmAlert.setContentText("It is an Information Alert!"); infrmButtn.setOnAction(e -> infrmAlert.showAndWait()); // Creating confirmation Alert and setting Button's action Alert cnfrmAlert = new Alert(AlertType.CONFIRMATION); cnfrmAlert.setContentText("It is a Confirmation Alert!"); cnfrmButtn.setOnAction(e -> cnfrmAlert.showAndWait()); // Creating warning Alert and setting Button's action Alert warngAlert = new Alert(AlertType.WARNING); warngAlert.setContentText("It is a Warning Alert!"); warngButtn.setOnAction(e -> warngAlert.showAndWait()); // Creating error Alert and setting Button's action Alert errorAlert = new Alert(AlertType.ERROR); errorAlert.setContentText("It is an Error Alert!"); errorButtn.setOnAction(e -> errorAlert.showAndWait()); // Create a HBox to hold the Buttons HBox box = new HBox(cnfrmButtn, infrmButtn, warngButtn, errorButtn); box.setAlignment(Pos.CENTER); box.setPadding(new Insets(15)); box.setSpacing(10); // Create a VBox to hold the Label and Button VBox vbox = new VBox(label, box); vbox.setAlignment(Pos.CENTER); // Create a Scene with the VBox as its root node Scene scene = new Scene(vbox, 400, 300); // Set the Title of the Stage stage.setTitle("Alert in JavaFX"); // Set the Scene of the Stage stage.setScene(scene); // Display the Stage stage.show(); } public static void main(String[] args) { launch(args); } }
Compile and execute the saved Java file from the command prompt using the following commands −
javac --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxAlert.java java --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxAlert
Output
On executing the above code, it will generate a window displaying four buttons. Each of the buttons is associated with different alerts.
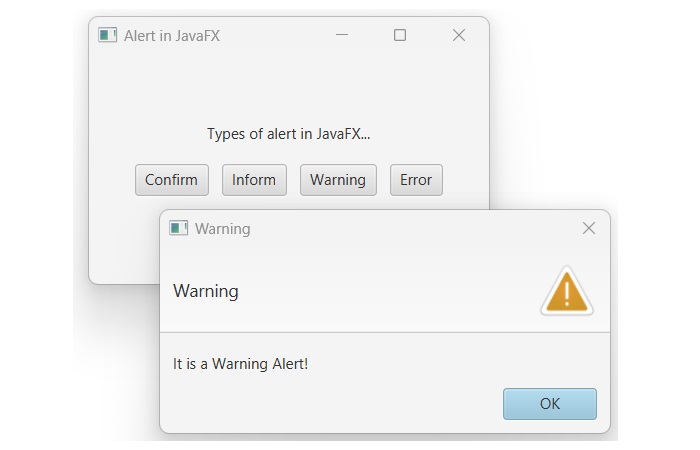