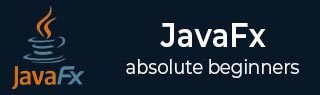
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Environment
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Path Objects
- JavaFX - Path Objects
- JavaFX - LineTo Path Object
- JavaFX - HLineTo Path Object
- JavaFX - VLineTo Path Object
- JavaFX - QuadCurveTo Path Object
- JavaFX - CubicCurveTo Path Object
- JavaFX - ArcTo Path Object
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX - Linear Gradient Pattern
- JavaFX - Radial Gradient Pattern
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX - Color Adjust Effect
- JavaFX - Color input Effect
- JavaFX - Image Input Effect
- JavaFX - Blend Effect
- JavaFX - Bloom Effect
- JavaFX - Glow Effect
- JavaFX - Box Blur Effect
- JavaFX - GaussianBlur Effect
- JavaFX - MotionBlur Effect
- JavaFX - Reflection Effect
- JavaFX - SepiaTone Effect
- JavaFX - Shadow Effect
- JavaFX - DropShadow Effect
- JavaFX - InnerShadow Effect
- JavaFX - Lighting Effect
- JavaFX - Light.Distant Effect
- JavaFX - Light.Spot Effect
- JavaFX - Point.Spot Effect
- JavaFX - DisplacementMap
- JavaFX - PerspectiveTransform
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX - Rotation Transformation
- JavaFX - Scaling Transformation
- JavaFX - Translation Transformation
- JavaFX - Shearing Transformation
- JavaFX Animations
- JavaFX - Animations
- JavaFX - Rotate Transition
- JavaFX - Scale Transition
- JavaFX - Translate Transition
- JavaFX - Fade Transition
- JavaFX - Fill Transition
- JavaFX - Stroke Transition
- JavaFX - Sequential Transition
- JavaFX - Parallel Transition
- JavaFX - Pause Transition
- JavaFX - Path Transition
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - Translation Transformation
A translation transformation simply moves an object to a different position on the same plane. You can translate a point in a 2D plane by adding translation coordinate (tx, ty) to the original coordinate (X, Y); this will get you the new coordinate (X’, Y’) where the point is relocated to.

Translation Transformation in JavaFX
In JavaFX, using the Translation transformation, a node can be shifted from one position to another position in the same application. This transformation is applied on a JavaFX node with the help of Translate class in javafx.scene.transform package. This class represents an Affine object that relocates the coordinates within the JavaFX application.
Example 1
Following is the program which demonstrates translation in JavaFX. Here, we are creating 2 circles (nodes) at the same location with the same dimensions, but with different colors (Brown and Cadetblue). We are also applying translation on the circle with a cadetblue color.
Save this code in a file with the name TranslationExample.java.
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.paint.Color; import javafx.scene.shape.Circle; import javafx.scene.transform.Translate; import javafx.stage.Stage; public class TranslationExample extends Application { @Override public void start(Stage stage) { //Drawing Circle1 Circle circle = new Circle(); //Setting the position of the circle circle.setCenterX(150.0f); circle.setCenterY(135.0f); //Setting the radius of the circle circle.setRadius(100.0f); //Setting the color of the circle circle.setFill(Color.BROWN); //Setting the stroke width of the circle circle.setStrokeWidth(20); //Drawing Circle2 Circle circle2 = new Circle(); //Setting the position of the circle circle2.setCenterX(150.0f); circle2.setCenterY(135.0f); //Setting the radius of the circle circle2.setRadius(100.0f); //Setting the color of the circle circle2.setFill(Color.CADETBLUE); //Setting the stroke width of the circle circle2.setStrokeWidth(20); //Creating the translation transformation Translate translate = new Translate(); //Setting the X,Y,Z coordinates to apply the translation translate.setX(300); translate.setY(50); translate.setZ(100); //Adding transformation to circle2 circle2.getTransforms().addAll(translate); //Creating a Group object Group root = new Group(circle,circle2); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Translation transformation example"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
Compile and execute the saved java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls TranslationExample.java java --module-path %PATH_TO_FX% --add-modules javafx.controls TranslationExample
Output
On executing, the above program generates a JavaFX window as shown below.
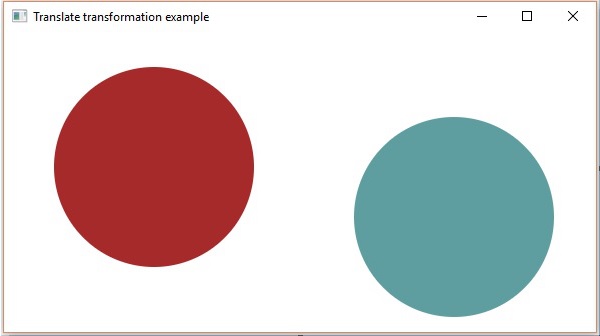
Example 2
In the following example, we are demonstrating translation on 3D shapes. Save this code in a file with the name TranslationExample3D.java.
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.paint.Color; import javafx.scene.shape.Cylinder; import javafx.scene.transform.Translate; import javafx.stage.Stage; public class TranslationExample3D extends Application { @Override public void start(Stage stage) { Cylinder cy1 = new Cylinder(50, 100); Cylinder cy2 = new Cylinder(50, 100); //Creating the translation transformation Translate translate = new Translate(); //Setting the X,Y,Z coordinates to apply the translation translate.setX(200); translate.setY(150); translate.setZ(100); //Adding transformation to circle2 cy2.getTransforms().addAll(translate); //Creating a Group object Group root = new Group(cy1,cy2); //Creating a scene object Scene scene = new Scene(root, 400, 300); //Setting title to the Stage stage.setTitle("Translation transformation example"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
Compile and execute the saved java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls TranslationExample3D.java java --module-path %PATH_TO_FX% --add-modules javafx.controls TranslationExample3D
Output
On executing, the above program generates a JavaFX window as shown below.

As we can see, with the original position of the cylinder we are not able to clearly see the full image like we do in the translated image. Therefore, translation transformation becomes necessary in cases like these.
To Continue Learning Please Login