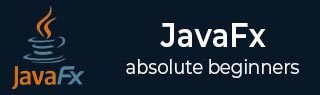
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX Animations
- JavaFX - Animations
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - Hyperlink
Hyperlinks are UI components that allow users to navigate to a web page or perform an action when clicked. They are similar to buttons but have different appearances and behavior. For example, we can find hyperlinks of any web page inside the address bar located at the top of display window as shown in the below figure −
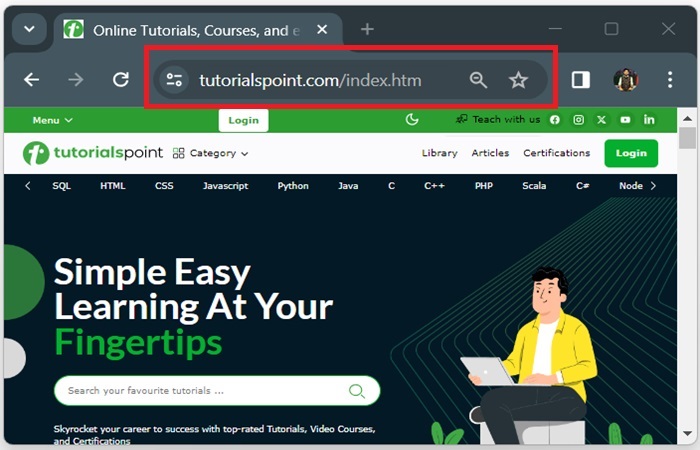
Creating Hyperlink in JavaFX
In JavaFX, the hyperlink is represented by a class named Hyperlink. This class belongs to the package javafx.scene.control. By instantiating this class, we can create a hyperlink in JavaFX. Constructors of the Hyperlink class are listed below −
Hyperlink() − It is the default constructor that constructs a hyperlink without label text.
Hyperlink(String str) − It creates a new hyperlink with the specified label text.
Hyperlink(String str, Node icons) − It creates a new hyperlink with the specified text and graphics labels.
In JavaFX, the hyperlinks are created in two forms namely text and image. While creating a hyperlink, our first step would be instantiating the Hyperlink class by using any of the above mentioned constructors. Then, specify the action that should be performed when the user clicks the link. To do so, we need to add an EventHandler to its setOnAction() method. Furthermore, this EventHandler will call the specified method which helps in navigation.
By changing the implementation of setOnAction() method, we can create hyperlinks for both local resources as well as those resources that are available on a remote server.
Example
In the following JavaFX program, we will create a hyperlink as a text. Save this code in a file with the name HyperlinkExample.java.
import javafx.application.Application; import javafx.event.ActionEvent; import javafx.event.EventHandler; import javafx.scene.Scene; import javafx.scene.control.Hyperlink; import javafx.scene.control.Label; import javafx.scene.layout.VBox; import javafx.stage.Stage; import javafx.geometry.Pos; public class HyperlinkExample extends Application { @Override public void start(Stage stage) { // Creating a Label Label labelText = new Label("On clicking the below text, it will redirect us to tutorialspoint"); // Create a hyperlink with text Hyperlink textLink = new Hyperlink("Visit TutorialsPoint"); // Set the action of the hyperlink textLink.setOnAction(new EventHandler() { @Override public void handle(ActionEvent event) { // Open the web page in the default browser getHostServices().showDocument("https://www.tutorialspoint.com/index.htm"); } }); // Create a scene with the hyperlink VBox root = new VBox(labelText, textLink); root.setAlignment(Pos.CENTER); Scene scene = new Scene(root, 400, 300); // Set the title and scene of the stage stage.setTitle("Hyperlink in JavaFX"); stage.setScene(scene); stage.show(); } public static void main(String[] args) { launch(args); } }
To compile and execute the saved Java file from the command prompt, use the following commands −
javac --module-path %PATH_TO_FX% --add-modules javafx.controls HyperlinkExample.java java --module-path %PATH_TO_FX% --add-modules javafx.controls HyperlinkExample
Output
When we execute, the above program will generate the following output.
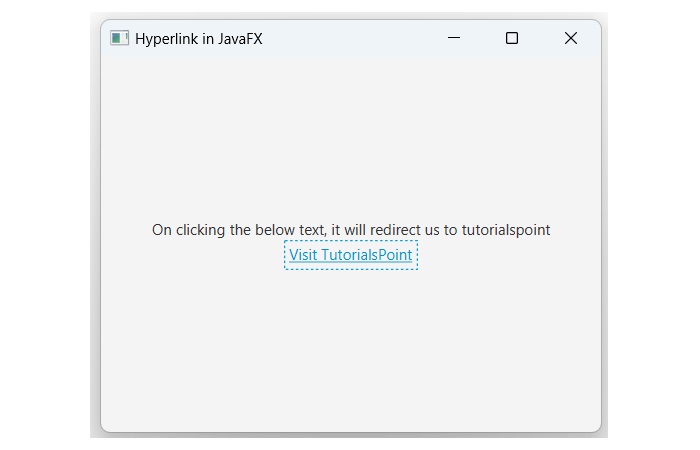
Creating Hyperlink as an Image
To create hyperlink with an image, instantiate the ImageView class and pass its obejct as a parameter value to the constructor of Hyperlink class as shown in the next example. Save this code in a file with the name HyperlinkImage.java.
import javafx.application.Application; import javafx.event.ActionEvent; import javafx.event.EventHandler; import javafx.scene.Scene; import javafx.scene.control.Hyperlink; import javafx.scene.control.Label; import javafx.scene.control.ContentDisplay; import javafx.scene.layout.VBox; import javafx.stage.Stage; import javafx.geometry.Pos; import javafx.scene.image.Image; import javafx.scene.image.ImageView; public class HyperlinkImage extends Application { @Override public void start(Stage stage) { // Creating a Label Label labelText = new Label("On clicking the below image, it will redirect us to tutorialspoint"); // Create a hyperlink with image Image image = new Image("tutorials_point.jpg"); ImageView imageV = new ImageView(image); imageV.setFitWidth(150); imageV.setFitHeight(150); Hyperlink imageLink = new Hyperlink("visit: ", imageV); // Set the content display position of the image imageLink.setContentDisplay(ContentDisplay.RIGHT); // Set the action of the hyperlink imageLink.setOnAction(new EventHandler() { @Override public void handle(ActionEvent event) { // Open the web page in the default browser getHostServices().showDocument("https://www.tutorialspoint.com/index.htm"); } }); // Create a scene with the hyperlink VBox root = new VBox(labelText, imageLink); root.setAlignment(Pos.CENTER); Scene scene = new Scene(root, 400, 300); // Set the title and scene of the stage stage.setTitle("Hyperlink in JavaFX"); stage.setScene(scene); stage.show(); } public static void main(String[] args) { launch(args); } }
Compile and execute the saved Java file from the command prompt using the following commands −
javac --module-path %PATH_TO_FX% --add-modules javafx.controls HyperlinkImage.java java --module-path %PATH_TO_FX% --add-modules javafx.controls HyperlinkImage
Output
On executing the above code, it will generate the following output.
