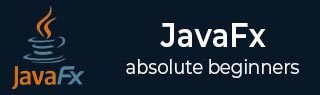
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX Animations
- JavaFX - Animations
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - Tooltip
The tooltip is a small pop-up window that displays some additional information when the user hovers over a node or a control. It is mainly used for explaining the functionality of a button, menu, or image or to provide some hints for a text field. In the below figure, we can see the tooltip explaining the menu's functionality −
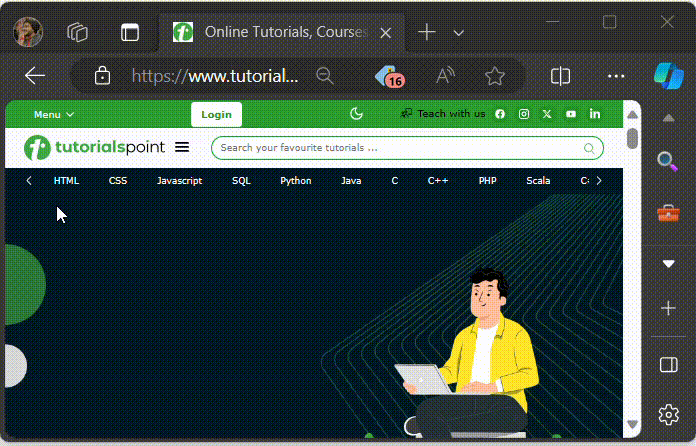
Tooltip in JavaFX
In JavaFX, the tooltip is represented by a class named Tooltip which is a part of javafx.scene.control package. Each UI component of this package comes with a built-in method named setTooltip() which is used for associating a tooltip. We can specify the tooltip text either by passing it to the setText() method or by using the constructor listed below −
Tooltip() − It is the default constructor that constructs a tooltip without any texts.
Tooltip(String str) − It constructs a new tooltip with the predefined text.
Steps to create a tooltip in JavaFX
To create a Tooltip in JavaFX, follow the steps given below.
Step 1: Create a node to associate with Tooltip
We know that a tooltip explains the features of specified node. This node can be any JavaFX component such as menu, image and text fields. Here, we are going to use an image as a node. To create an image in JavaFX, instantiate the ImageView class and pass the path of image as a parameter value to its constructor.
//Passing path of an image Image image = new Image(new FileInputStream("tutorials_point.jpg")); //Setting the image view ImageView imageView = new ImageView(image);
Step 2: Instantiate the Tooltip class
To create a tooltip in JavaFX, instantiate the Tooltip class and pass the tooltip text as a parameter value to its constructor using the below code −
//Creating tool tip for the given image Tooltip toolTipTxt = new Tooltip("This is the new logo of Tutorialspoint");
Step 3: Associate the tooltip to the node
To associate the Tooltip to the specified node, we use the install() mehtod which accepts Tooltip and ImageView objects as arguments.
//Setting the tool tip to the image Tooltip.install(imageView, toolTipTxt);
Step 4: Launching Application
After creating the Tooltip, follow the given steps below to launch the application properly −
Firstly, create a VBox that holds the nodes vertically.
Next, instantiate the class named Scene by passing the VBox object as a parameter value to its constructor along with the dimensions of the application screen.
Then, set the title of the stage using the setTitle() method of the Stage class.
Now, a Scene object is added to the stage using the setScene() method of the class named Stage.
Display the contents of the scene using the method named show().
Lastly, the application is launched with the help of the launch() method.
Example
In the following example, we are going to create a tooltip in JavaFX application. Save this code in a file with the name JavafxTooltip.java.
import javafx.application.Application; import javafx.geometry.Insets; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.scene.image.Image; import javafx.scene.image.ImageView; import javafx.scene.control.Tooltip; import javafx.scene.layout.VBox; import java.io.FileInputStream; import java.io.FileNotFoundException; import javafx.stage.Stage; public class JavafxTooltip extends Application { @Override public void start(Stage stage) throws FileNotFoundException { //Creating a label Label labeltext = new Label("Hover over Image to see the details...."); //Passing path of an image Image image = new Image(new FileInputStream("tutorials_point.jpg")); //Setting the image view ImageView imageView = new ImageView(image); //Setting the position of the image imageView.setX(50); imageView.setY(25); //setting the fit height and width of the image view imageView.setFitHeight(350); imageView.setFitWidth(350); //Setting the preserve ratio of the image view imageView.setPreserveRatio(true); //Creating tool tip for the given image Tooltip toolTipTxt = new Tooltip("This is the new logo of Tutorialspoint"); //Setting the tool tip to the image Tooltip.install(imageView, toolTipTxt); // to display the content vertically VBox box = new VBox(5); box.setPadding(new Insets(25, 5 , 5, 50)); box.getChildren().addAll(labeltext, imageView); //Setting the stage Scene scene = new Scene(box, 400, 350); stage.setTitle("Example of Tooltip in JavaFX"); stage.setScene(scene); stage.show(); } public static void main(String args[]){ launch(args); } }
To compile and execute the saved Java file from the command prompt, use the following commands −
javac --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxTooltip.java java --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxTooltip
Output
When we execute the above code, it will generate a Tooltip for an image as shown in the following output.
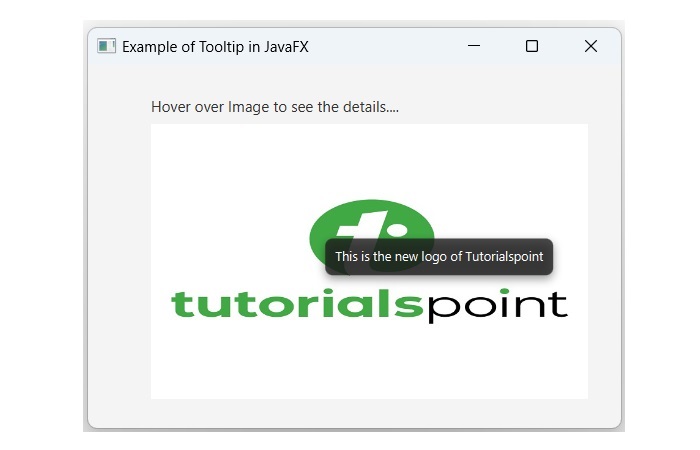
Adding icons to the Tooltip
Icons are small images that graphically illustrate a command or function of an application. To add icons to the JavaFX tooltip, we use the setGraphic() method which accepts the ImageView object and displays the icon on the left of tooltip text.
Example
Following is the JavaFX program that will create a Tooltip with icon. Save this code in a file with the name JavafxTooltip.java.
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.scene.image.Image; import javafx.scene.image.ImageView; import javafx.scene.control.Tooltip; import javafx.scene.layout.VBox; import java.io.FileInputStream; import java.io.FileNotFoundException; import javafx.stage.Stage; public class JavafxTooltip extends Application { public void start(Stage stage) throws FileNotFoundException { // Creating a label Label labeltext = new Label("Hover over this text to see the tooltip...."); // Instantiating Tooltip class Tooltip toolT = new Tooltip(); // Passing path of an image Image icon = new Image(new FileInputStream("faviconTP.png")); // adding the icon for tooltip toolT.setGraphic(new ImageView(icon)); // adding the text toolT.setText("This is the new logo of Tutorialspoint"); // setting the tooltip to the label labeltext.setTooltip(toolT); //Setting the stage Scene scene = new Scene(labeltext, 400, 300); stage.setTitle("Example of Tooltip in JavaFX"); stage.setScene(scene); stage.show(); } public static void main(String args[]){ launch(args); } }
Compile and execute the saved Java file from the command prompt using the following commands −
javac --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxTooltip.java java --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxTooltip
Output
On executing, the above program will generate the following output −
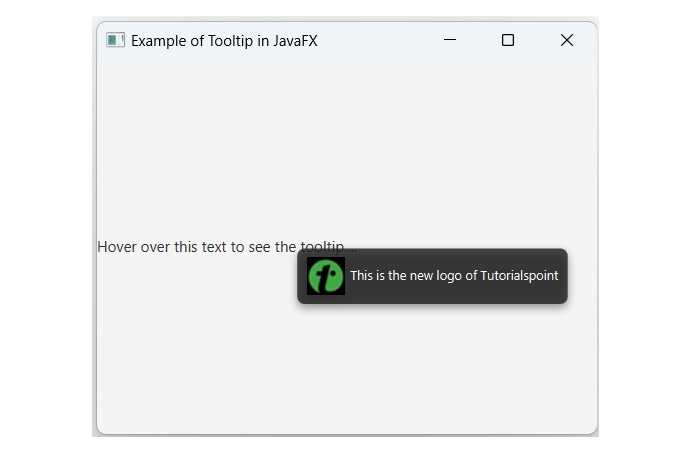