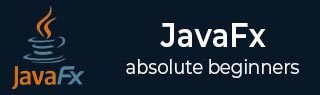
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX Animations
- JavaFX - Animations
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - Event Filters
Event filters enable you to handle an event during the event capturing phase of event processing. Event Capturing phase in event handling is a phase where an event travels through all the nodes in a dispatch chain. A node in this dispatch chain can either have one or more filters, or no filters at all, to handle an event.
Event Filters process the events, like mouse events, scroll events, keyboard events, etc. during this Event Capturing phase. However, these Event Filters need to be registered with the node in order to provide the event handling logic to the event generated on the node.
If a node does not contain an event filter, the event is directly passed to the target node. Otherwise, a single filter can be used for more than one node and event types. To simply summarize, Event filters are used to enable the parent node to provide common processing for its child nodes; and also to intercept an event and prevent child nodes from acting on the event.
Adding and Removing Event Filter
To add an event filter to a node, you need to register this filter using the method addEventFilter() of the Node class.
//Creating the mouse event handler EventHandler<MouseEvent> eventHandler = new EventHandler<MouseEvent>() { @Override public void handle(MouseEvent e) { System.out.println("Hello World"); circle.setFill(Color.DARKSLATEBLUE); } }; //Adding event Filter Circle.addEventFilter(MouseEvent.MOUSE_CLICKED, eventHandler);
In the same way, you can remove a filter using the method removeEventFilter() as shown below −
circle.removeEventFilter(MouseEvent.MOUSE_CLICKED, eventHandler);
Example
Following is an example demonstrating the event handling in JavaFX using the event filters. Save this code in a file with name EventFiltersExample.java.
import javafx.application.Application; import static javafx.application.Application.launch; import javafx.event.EventHandler; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.input.MouseEvent; import javafx.scene.paint.Color; import javafx.scene.shape.Circle; import javafx.scene.text.Font; import javafx.scene.text.FontWeight; import javafx.scene.text.Text; import javafx.stage.Stage; public class EventFiltersExample extends Application { @Override public void start(Stage stage) { //Drawing a Circle Circle circle = new Circle(); //Setting the position of the circle circle.setCenterX(300.0f); circle.setCenterY(135.0f); //Setting the radius of the circle circle.setRadius(25.0f); //Setting the color of the circle circle.setFill(Color.BROWN); //Setting the stroke width of the circle circle.setStrokeWidth(20); //Setting the text Text text = new Text("Click on the circle to change its color"); //Setting the font of the text text.setFont(Font.font(null, FontWeight.BOLD, 15)); //Setting the color of the text text.setFill(Color.CRIMSON); //setting the position of the text text.setX(150); text.setY(50); //Creating the mouse event handler EventHandler<MouseEvent> eventHandler = new EventHandler<MouseEvent>() { @Override public void handle(MouseEvent e) { System.out.println("Hello World"); circle.setFill(Color.DARKSLATEBLUE); } }; //Registering the event filter circle.addEventFilter(MouseEvent.MOUSE_CLICKED, eventHandler); //Creating a Group object Group root = new Group(circle, text); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting the fill color to the scene scene.setFill(Color.LAVENDER); //Setting title to the Stage stage.setTitle("Event Filters Example"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
Compile and execute the saved java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls EventFiltersExample.java java --module-path %PATH_TO_FX% --add-modules javafx.controls EventFiltersExample
Output
On executing, the above program generates a JavaFX window as shown below.
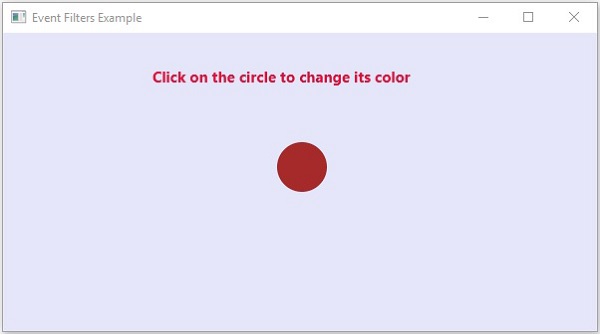
Example
We have seen how event filters processing a mouse event. Now, let us try to register event filters on other events like Keyboard events. Save this code in a file with name EventFilterKeyboard.java.
import javafx.application.Application; import javafx.event.EventHandler; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.scene.control.TextField; import javafx.scene.input.KeyEvent; import javafx.scene.paint.Color; import javafx.stage.Stage; public class EventFilterKeyboard extends Application{ @Override public void start(Stage primaryStage) throws Exception { //Adding Labels and TextFileds to the scene Label label1 = new Label("Insert Key"); Label label2 = new Label("Event"); label1.setTranslateX(100); label1.setTranslateY(100); label2.setTranslateX(100); label2.setTranslateY(150); TextField text1 = new TextField(); TextField text2 = new TextField(); text1.setTranslateX(250); text1.setTranslateY(100); text2.setTranslateX(250); text2.setTranslateY(150); //Creating EventHandler Object EventHandler<KeyEvent> filter = new EventHandler<KeyEvent>() { @Override public void handle(KeyEvent event) { text2.setText("Event : "+event.getEventType()); text1.setText(event.getText()); event.consume(); } }; //Registering Event Filter for the event generated on text field text1.addEventFilter(KeyEvent.ANY, filter); //Setting Group and Scene Group root = new Group(); root.getChildren().addAll(label1,label2,text1,text2); Scene scene = new Scene(root, 500, 300); primaryStage.setScene(scene); primaryStage.setTitle("Adding Event Filter"); primaryStage.show(); } public static void main(String[] args) { launch(args); } }
Compile and execute the saved java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls EventFilterKeyboard.java java --module-path %PATH_TO_FX% --add-modules javafx.controls EventFilterKeyboard
Output
On executing, the above program generates a JavaFX window as shown below.
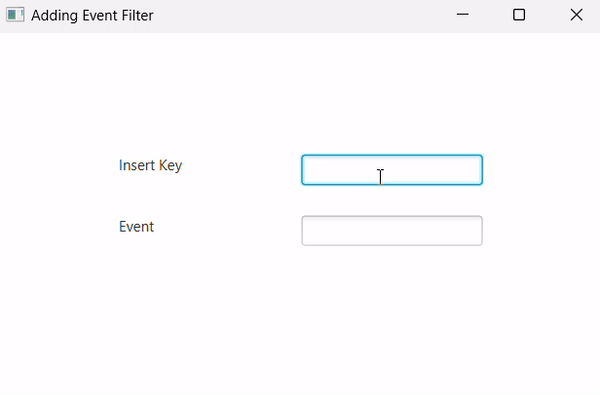