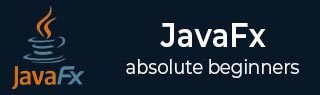
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Environment
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Path Objects
- JavaFX - Path Objects
- JavaFX - LineTo Path Object
- JavaFX - HLineTo Path Object
- JavaFX - VLineTo Path Object
- JavaFX - QuadCurveTo Path Object
- JavaFX - CubicCurveTo Path Object
- JavaFX - ArcTo Path Object
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX - Linear Gradient Pattern
- JavaFX - Radial Gradient Pattern
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX - Color Adjust Effect
- JavaFX - Color input Effect
- JavaFX - Image Input Effect
- JavaFX - Blend Effect
- JavaFX - Bloom Effect
- JavaFX - Glow Effect
- JavaFX - Box Blur Effect
- JavaFX - GaussianBlur Effect
- JavaFX - MotionBlur Effect
- JavaFX - Reflection Effect
- JavaFX - SepiaTone Effect
- JavaFX - Shadow Effect
- JavaFX - DropShadow Effect
- JavaFX - InnerShadow Effect
- JavaFX - Lighting Effect
- JavaFX - Light.Distant Effect
- JavaFX - Light.Spot Effect
- JavaFX - Point.Spot Effect
- JavaFX - DisplacementMap
- JavaFX - PerspectiveTransform
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX - Rotation Transformation
- JavaFX - Scaling Transformation
- JavaFX - Translation Transformation
- JavaFX - Shearing Transformation
- JavaFX Animations
- JavaFX - Animations
- JavaFX - Rotate Transition
- JavaFX - Scale Transition
- JavaFX - Translate Transition
- JavaFX - Fade Transition
- JavaFX - Fill Transition
- JavaFX - Stroke Transition
- JavaFX - Sequential Transition
- JavaFX - Parallel Transition
- JavaFX - Pause Transition
- JavaFX - Path Transition
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - Material Property
Until now, when we addressed the properties of a JavaFX shape object, it always only included the color of shape fill and type of shape strokes. However, JavaFX also allows you to assign the type of material for a 3D shape.
Material of a 3D object is nothing but the type of texture used to create a 3D object. For example, a box in real world can be made of different materials; like a cardboard, a metal, wood, or just any other solid. All of these form the same shape but with a different material. Similarly, JavaFX allows you to choose the type of material used to create a 3D object.
Material Property
The Material property is of the type Material and it is used to choose the surface of the material of a 3D shape. You can set the material of a 3D shape using the method setMaterial() as follows −
cylinder.setMaterial(material);
As mentioned above for this method, you need to pass an object of the type Material. The PhongMaterial class of the package javafx.scene.paint is a sub class of this class and provides 7 properties that represent a Phong shaded material. You can apply all these type of materials to the surface of a 3D shape using the setter methods of these properties.
Following are the type of materials that are available in JavaFX −
bumpMap − This represents a normal map stored as a RGB Image.
diffuseMap − This represents a diffuse map.
selfIlluminationMap − This represents a self-illumination map of this PhongMaterial.
specularMap − This represents a specular map of this PhongMaterial.
diffuseColor − This represents a diffuse color of this PhongMaterial.
specularColor − This represents a specular color of this PhongMaterial.
specularPower − This represents a specular power of this PhongMaterial.
By default, the material of a 3-Dimensional shape is a PhongMaterial with a diffuse color of light gray.
Example
Following is an example which displays various materials on the cylinder. Save this code in a file with the name CylinderMaterials.java.
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.PerspectiveCamera; import javafx.scene.Scene; import javafx.scene.image.Image; import javafx.scene.paint.Color; import javafx.scene.paint.PhongMaterial; import javafx.scene.shape.Cylinder; import javafx.stage.Stage; public class CylinderMaterials extends Application { @Override public void start(Stage stage) { //Drawing Cylinder1 Cylinder cylinder1 = new Cylinder(); //Setting the properties of the Cylinder cylinder1.setHeight(130.0f); cylinder1.setRadius(30.0f); //Setting the position of the Cylinder cylinder1.setTranslateX(100); cylinder1.setTranslateY(75); //Preparing the phong material of type bump map PhongMaterial material1 = new PhongMaterial(); material1.setBumpMap(new Image ("http://www.tutorialspoint.com/images/tplogo.gif")); //Setting the bump map material to Cylinder1 cylinder1.setMaterial(material1); //Drawing Cylinder2 Cylinder cylinder2 = new Cylinder(); //Setting the properties of the Cylinder cylinder2.setHeight(130.0f); cylinder2.setRadius(30.0f); //Setting the position of the Cylinder cylinder2.setTranslateX(200); cylinder2.setTranslateY(75); //Preparing the phong material of type diffuse map PhongMaterial material2 = new PhongMaterial(); material2.setDiffuseMap(new Image ("http://www.tutorialspoint.com/images/tp-logo.gif")); //Setting the diffuse map material to Cylinder2 cylinder2.setMaterial(material2); //Drawing Cylinder3 Cylinder cylinder3 = new Cylinder(); //Setting the properties of the Cylinder cylinder3.setHeight(130.0f); cylinder3.setRadius(30.0f); //Setting the position of the Cylinder cylinder3.setTranslateX(300); cylinder3.setTranslateY(75); //Preparing the phong material of type Self Illumination Map PhongMaterial material3 = new PhongMaterial(); material3.setSelfIlluminationMap(new Image ("http://www.tutorialspoint.com/images/tp-logo.gif")); //Setting the Self Illumination Map material to Cylinder3 cylinder3.setMaterial(material3); //Drawing Cylinder4 Cylinder cylinder4 = new Cylinder(); //Setting the properties of the Cylinder cylinder4.setHeight(130.0f); cylinder4.setRadius(30.0f); //Setting the position of the Cylinder cylinder4.setTranslateX(400); cylinder4.setTranslateY(75); //Preparing the phong material of type Specular Map PhongMaterial material4 = new PhongMaterial(); material4.setSpecularMap(new Image ("http://www.tutorialspoint.com/images/tp-logo.gif")); //Setting the Specular Map material to Cylinder4 cylinder4.setMaterial(material4); //Drawing Cylinder5 Cylinder cylinder5 = new Cylinder(); //Setting the properties of the Cylinder cylinder5.setHeight(130.0f); cylinder5.setRadius(30.0f); //Setting the position of the Cylinder cylinder5.setTranslateX(100); cylinder5.setTranslateY(300); //Preparing the phong material of type diffuse color PhongMaterial material5 = new PhongMaterial(); material5.setDiffuseColor(Color.BLANCHEDALMOND); //Setting the diffuse color material to Cylinder5 cylinder5.setMaterial(material5); //Drawing Cylinder6 Cylinder cylinder6 = new Cylinder(); //Setting the properties of the Cylinder cylinder6.setHeight(130.0f); cylinder6.setRadius(30.0f); //Setting the position of the Cylinder cylinder6.setTranslateX(200); cylinder6.setTranslateY(300); //Preparing the phong material of type specular color PhongMaterial material6 = new PhongMaterial(); //setting the specular color map to the material material6.setSpecularColor(Color.BLANCHEDALMOND); //Setting the specular color material to Cylinder6 cylinder6.setMaterial(material6); //Drawing Cylinder7 Cylinder cylinder7 = new Cylinder(); //Setting the properties of the Cylinder cylinder7.setHeight(130.0f); cylinder7.setRadius(30.0f); //Setting the position of the Cylinder cylinder7.setTranslateX(300); cylinder7.setTranslateY(300); //Preparing the phong material of type Specular Power PhongMaterial material7 = new PhongMaterial(); material7.setSpecularPower(0.1); //Setting the Specular Power material to the Cylinder cylinder7.setMaterial(material7); //Creating a Group object Group root = new Group(cylinder1 ,cylinder2, cylinder3, cylinder4, cylinder5, cylinder6, cylinder7); //Creating a scene object Scene scene = new Scene(root, 600, 400); //Setting camera PerspectiveCamera camera = new PerspectiveCamera(false); camera.setTranslateX(0); camera.setTranslateY(0); camera.setTranslateZ(-10); scene.setCamera(camera); //Setting title to the Stage stage.setTitle("Drawing a cylinder"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
Compile and execute the saved java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls CylinderMaterials.java java --module-path %PATH_TO_FX% --add-modules javafx.controls CylinderMaterials
Output
On executing, the above program generates a JavaFX window displaying 7 cylinders with Materials, Bump Map, Diffuse Map, Self-Illumination Map, Specular Map, Diffuse Color, Specular Color, (BLANCHEDALMOND) Specular Power, respectively, as shown in the following screenshot −
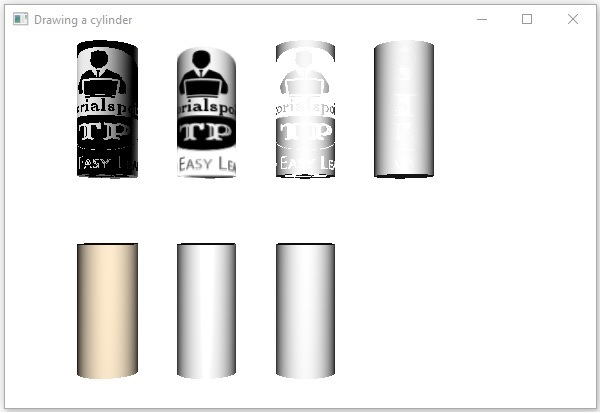