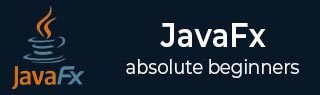
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX Animations
- JavaFX - Animations
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - TabPane
A TabPane serves as a container for one or more Tab objects. The term tab refers to a section at the top of display area that represents another web page or content. The content of a web page becomes visible only when its corresponding tab is clicked. We can see three tabs are open in the below figure −
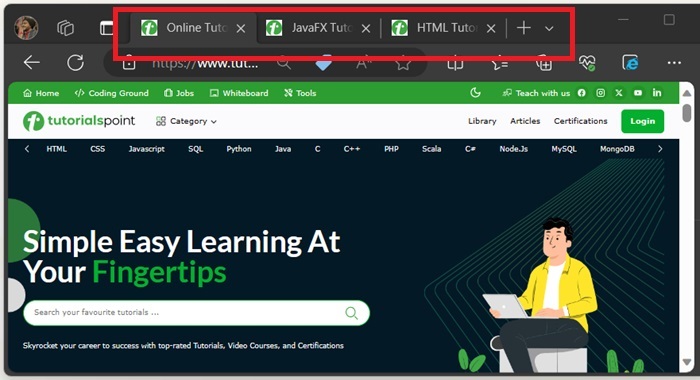
TabPane in JavaFX
In JavaFX, the class named TabPane represents a tabpane. To use the feature of tabpane, we need to create an instance of the TabPane class and add the tabs we want to display inside it. These tabs can contain any JavaFX nodes as the content of a tab, such as labels, buttons, images, text fields, videos and so on. We can use any of the below constructors to create a tabpane −
TabPane() − It is used to create an empty tabpane.
TabPane(Tab tabs) − It is the parameterized constructor of TabPane class which will create a new tabpane with the specified set of tabs.
Steps to create a TabPane in JavaFX
To create a TabPane in JavaFX, follow the steps given below.
Step 1: Create desired number of Tabs
As discussed earlier, a tabpane contains one or more Tabs. Therefore, our first step would be creating tabs to display within the tabpane. Here, we are going to create three tabs namely Label, Image and Video. In JavaFX, the tabs are created by instantiating the class named Tab which belongs to a package javafx.scene.control. A Tab object has a text property that sets the title of the tab. Create the tabs using the following code −
// Creating a Label tab Tab tab1 = new Tab("Label"); // Creating an Image tab Tab tab2 = new Tab("Image"); // Creating a Video tab Tab tab3 = new Tab("Video");
Step 2: Set the content of Tabs
The Tab object has a content property that sets the node to be displayed in the tab body. For this action, we use the setContent() method. It is used along with the Tab object and accepts Node object as an argument to its constructor as shown in the below code −
// setting the Label tab tab1.setContent(new Label("This is the first tab")); // setting the Image tab tab2.setContent(imageView); // setting the Video tab tab3.setContent(vbox);
Step 3: Instantiate the TabPane class
To create the tabpane, instantiate the TabPane class of package javafx.scene.control without passing any parameter value to its constructor and add all the tabs to the tabpane using the getTabs() method.
// Creating a TabPane TabPane tabPane = new TabPane(); // Adding all the tabs to the TabPane tabPane.getTabs().addAll(tab1, tab2, tab3);
Step 4: Launching Application
After creating the TabPane and adding the tabs to it, follow the given steps below to launch the application properly −
Firstly, instantiate the class named Scene by passing the TabPane object as a parameter value to its constructor. Also, pass the dimensions of the application screen as optional parameters to this constructor.
Then, set the title to the stage using the setTitle() method of the Stage class.
Now, a Scene object is added to the stage using the setScene() method of the class named Stage.
Display the contents of the scene using the method named show().
Lastly, the application is launched with the help of the launch() method.
Example
In the following example, we are going to create a TabPane in JavaFX application. Save this code in a file with the name JavafxtabsDemo.java.
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.scene.control.Tab; import javafx.scene.control.TabPane; import javafx.scene.image.Image; import javafx.scene.image.ImageView; import javafx.scene.media.Media; import javafx.scene.media.MediaPlayer; import javafx.scene.media.MediaView; import javafx.stage.Stage; import java.io.File; import javafx.scene.layout.VBox; import javafx.scene.layout.HBox; import javafx.scene.control.Button; import javafx.geometry.Pos; public class JavafxtabsDemo extends Application { @Override public void start(Stage stage) { // Creating a Label tab Tab tab1 = new Tab("Label"); tab1.setContent(new Label("This is the first tab")); // Creating an Image tab Tab tab2 = new Tab("Image"); Image image = new Image("tutorials_point.jpg"); ImageView imageView = new ImageView(image); tab2.setContent(imageView); // Creating a Video tab Tab tab3 = new Tab("Video"); // Passing the video file to the File object File videofile = new File("sampleTP.mp4"); // creating a Media object from the File Object Media videomedia = new Media(videofile.toURI().toString()); // creating a MediaPlayer object from the Media Object MediaPlayer mdplayer = new MediaPlayer(videomedia); // creating a MediaView object from the MediaPlayer Object MediaView viewmedia = new MediaView(mdplayer); //setting the fit height and width of the media view viewmedia.setFitHeight(350); viewmedia.setFitWidth(350); // creating video controls using the buttons Button pause = new Button("Pause"); Button resume = new Button("Resume"); // creating an HBox HBox box = new HBox(20); box.setAlignment(Pos.CENTER); box.getChildren().addAll(pause, resume); // function to handle play and pause buttons pause.setOnAction(act -> mdplayer.pause()); resume.setOnAction(act -> mdplayer.play()); // creating the root VBox vbox = new VBox(20); vbox.setAlignment(Pos.CENTER); vbox.getChildren().addAll(viewmedia, box); tab3.setContent(vbox); // Creating a TabPane TabPane tabPane = new TabPane(); // Adding all the tabs to the TabPane tabPane.getTabs().addAll(tab1, tab2, tab3); // Create a Scene with the TabPane as its root Scene scene = new Scene(tabPane, 400, 400); // Set the Title on the Stage stage.setTitle("TabPane in JavaFX"); // Set the Scene on the Stage stage.setScene(scene); stage.show(); } public static void main(String[] args) { launch(args); } }
To compile and execute the saved Java file from the command prompt, use the following commands −
javac --module-path %PATH_TO_FX% --add-modules javafx.controls,javafx.media JavafxtabsDemo.java java --module-path %PATH_TO_FX% --add-modules javafx.controls,javafx.media JavafxtabsDemo
Output
When we execute the above code, it will generate a TabPane with three tabs namely Label, Image and Video.
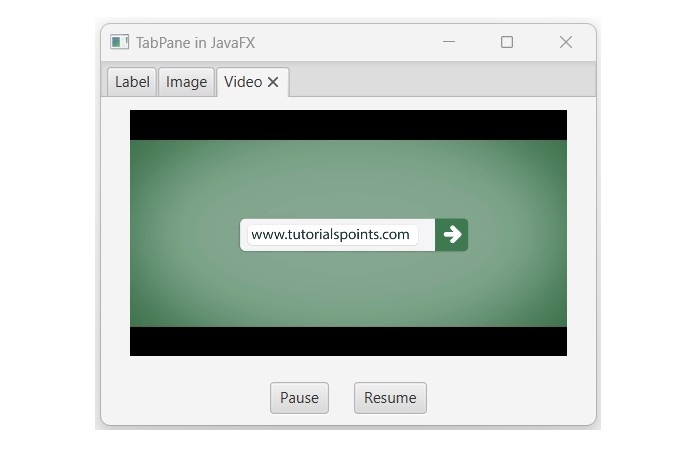