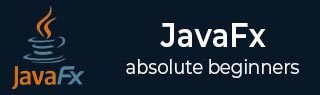
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX Animations
- JavaFX - Animations
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - Separator
A separator is a horizontal or vertical line that serves to divide UI elements of the JavaFX application. Note that it does not produce any action. However, it is possible to style it, apply visual effects to it, or even animate it to provide a better user experience. In the below figure, we can see a vertical and a horizontal separator −
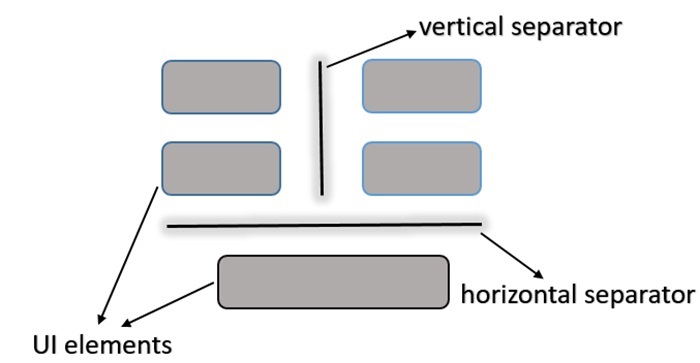
Separator in JavaFX
In JavaFX, the separator is represented by a class named Separator. This class belongs to the package javafx.scene.control. By instantiating this class, we can create a separator in JavaFX. This class has the following constructors −
Separator() − It creates a new horizontal separator.
Separator(Orientation orientation) − It creates a separator with the specified orientation. The orientation could be either vertical or horizontal.
Creating a Separator in JavaFX
We need to follow the steps given below to create a Separator in JavaFX.
Step 1: Create nodes that are going to be separated
Since separators are used to separate UI nodes in JavaFX. Hence, it is required to specify the UI elements that are going to be separated. For instance, we are creating Buttons using the below code −
// Creating Buttons Button btn1 = new Button("Button 1"); Button btn2 = new Button("Button 2"); Button btn3 = new Button("Button 3"); Button btn4 = new Button("Button 4");
Step 2: Instantiate the Separator class
In JavaFX, the separators are created by instantiating the class named Separators which belongs to a package javafx.scene.control. Instantiate this class inside the start() method as shown below −
// creating a separator Separator sep1 = new Separator();
Step 3: Set the desired Orientation
By default, the separator is horizontal, but, we can change its orientation by using the setOrientation() method and passing an Orientation enum value (HORIZONTAL or VERTICAL) as an argument. The code shown below will set the orientation to vertical −
sep1.setOrientation(Orientation.VERTICAL);
Also, the Separator occupies the full horizontal or vertical space allocated to it. Hence, it is necessary to set its maximum width or height as per the requirement by using the setMaxWidth() or setMaxHeight methods. To specify the horizontal or vertical alignment of the separator line within the allocated space, use the setHalignment or setValignment methods and pass an HPos or VPos enum value as an argument.
Step 4: Launch the Application
Once the Separator is created, follow the given steps below to launch the application properly −
Firstly, instantiate the class named Scene by passing the ChoiceBox object as a parameter value to its constructor. We can also pass dimensions of the application screen as optional parameters to this constructor.
Then, set the title to the stage using the setTitle() method of the Stage class.
Now, a Scene object is added to the stage using the setScene() method of the class named Stage.
Display the contents of the scene using the method named show().
Lastly, the application is launched with the help of the launch() method.
Example
The following JavaFX program demonstrates how to create a separator between buttons in JavaFX application. Save this code in a file with the name JavafxSeparator.java.
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.control.Separator; import javafx.scene.layout.GridPane; import javafx.geometry.Orientation; import javafx.stage.Stage; import javafx.geometry.Pos; public class JavafxSeparator extends Application { @Override public void start(Stage stage) { // creating grid to contain buttons GridPane newgrid = new GridPane(); // set horizontal and vertical gap newgrid.setHgap(10); newgrid.setVgap(10); newgrid.setAlignment(Pos.CENTER); // Creating Buttons Button btn1 = new Button("Button 1"); Button btn2 = new Button("Button 2"); Button btn3 = new Button("Button 3"); Button btn4 = new Button("Button 4"); // adding buttons to the grid newgrid.add(btn1, 0, 0); newgrid.add(btn2, 2, 0); newgrid.add(btn3, 0, 1); newgrid.add(btn4, 2, 1); // creating a horizontal and a vertical separator Separator sep1 = new Separator(); Separator sep2 = new Separator(); sep2.setOrientation(Orientation.VERTICAL); // Setting background color to the separators sep1.setStyle("-fx-background-color: #D2691E;"); sep2.setStyle("-fx-background-color: #D2691E;"); // adding separators to the grid newgrid.add(sep1, 0, 2, 2, 1); newgrid.add(sep2, 1, 0, 1, 2); // creating scene and stage Scene scene = new Scene(newgrid, 400, 300); stage.setTitle("Separator in JavaFX"); stage.setScene(scene); stage.show(); } public static void main(String[] args) { launch(args); } }
To compile and execute the saved Java file from the command prompt, use the following commands −
javac --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxSeparator.java java --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxSeparator
Output
When we execute the above code, it will generate the following output.
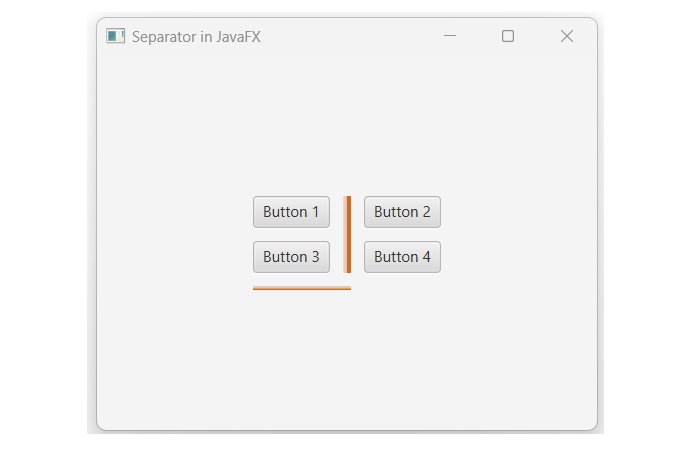
Creating a Separator in JavaFX using its parameterized constructor
As previously discussed, there are two ways to create a separator in JavaFX: one utilizes the default constructor of the Separator class, while the other uses its parameterized constructor. In the next example, we are going to use the parameterized constructor of the Separator class to create a separator in JavaFX. Save this code in a file with the name VerticalSeparator.java.
Example
import javafx.application.Application; import javafx.geometry.Insets; import javafx.scene.Scene; import javafx.scene.control.Separator; import javafx.scene.control.TextField; import javafx.scene.layout.HBox; import javafx.stage.Stage; import javafx.geometry.Orientation; public class VerticalSeparator extends Application { @Override public void start(Stage stage) { // creating three text fields TextField textField1 = new TextField(); TextField textField2 = new TextField(); TextField textField3 = new TextField(); // creating vertical separators Separator separator1 = new Separator(Orientation.VERTICAL); Separator separator2 = new Separator(Orientation.VERTICAL); // creating HBox HBox hbox = new HBox(); hbox.setPadding(new Insets(15, 12, 15, 12)); hbox.setSpacing(10); hbox.getChildren().addAll(textField1, separator1, textField2, separator2, textField3); // setting the scene and stage Scene scene = new Scene(hbox, 500, 300); stage.setScene(scene); stage.show(); } public static void main(String[] args) { launch(args); } }
Compile and execute the saved Java file from the command prompt by using the following commands −
javac --module-path %PATH_TO_FX% --add-modules javafx.controls VerticalSeparator.java java --module-path %PATH_TO_FX% --add-modules javafx.controls VerticalSeparator
Output
On executing the above code, it will generate the following output.
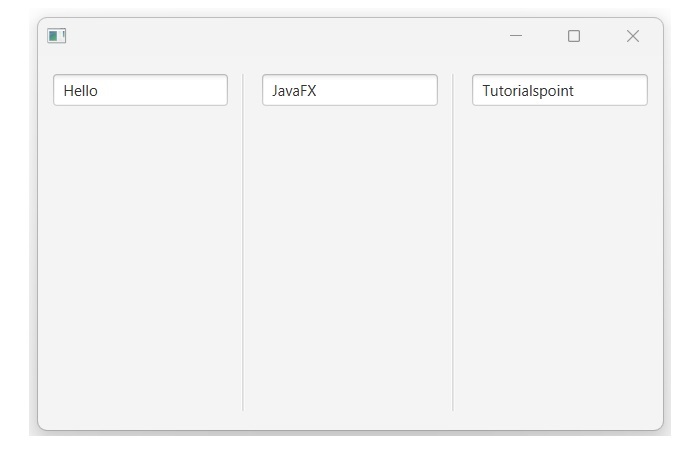