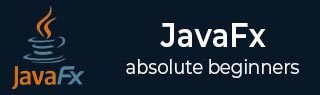
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX Animations
- JavaFX - Animations
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - Subtraction Operation
As the name suggests, the subtraction operation will subtract the elements of a set from another set. Most people are generally confused between the intersection operation, the two operations completely differ with respect to their operations. While intersection operation retrieves the common elements between two sets, the subtraction operation finds the common elements between two sets and removes them from the first set. If there are elements in second set that are not present in the first set, they are ignored.
Like other operations, the subtraction operation is also adopted in computer programming. It is available as difference operator in few programming languages; but in JavaFX, this operation can be used on 2D shapes.
Subtraction Operation in JavaFX
In JavaFX, the subtraction operation works with the area covered by two or more 2D shapes. It eliminates the area of the second shape from the area of the first shape. If the areas of these two shapes are fully exclusive, the area of first shape is retained as the result. Technically, this operation takes two or more shapes as an input. Then, it returns the area of the first shape excluding the area overlapped by the second one as shown below.
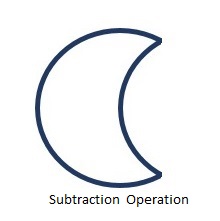
You can perform the Subtraction Operation on the shapes using the method named subtract(). Since this is a static method, you should call it using the class name (Shape or its subclasses) as shown below.
Shape shape = Shape.subtract(circle1, circle2);
Following is an example of the Subtraction Operation. In here, we are drawing two circles and performing a subtraction operation on them.
Save this code in a file with name SubtractionExample.java.
Example
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.paint.Color; import javafx.stage.Stage; import javafx.scene.shape.Circle; import javafx.scene.shape.Shape; public class SubtractionExample extends Application { @Override public void start(Stage stage) { //Drawing Circle1 Circle circle1 = new Circle(); //Setting the position of the circle circle1.setCenterX(250.0f); circle1.setCenterY(135.0f); //Setting the radius of the circle circle1.setRadius(100.0f); //Setting the color of the circle circle1.setFill(Color.DARKSLATEBLUE); //Drawing Circle2 Circle circle2 = new Circle(); //Setting the position of the circle circle2.setCenterX(350.0f); circle2.setCenterY(135.0f); //Setting the radius of the circle circle2.setRadius(100.0f); //Setting the color of the circle circle2.setFill(Color.BLUE); //Performing subtraction operation on the circle Shape shape = Shape.subtract(circle1, circle2); //Setting the fill color to the result shape.setFill(Color.DARKSLATEBLUE); //Creating a Group object Group root = new Group(shape); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Subtraction Example"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
Compile and execute the saved java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls SubtractionExample.java java --module-path %PATH_TO_FX% --add-modules javafx.controls SubtractionExample
Output
On executing, the above program generates a JavaFX window displaying the following output −
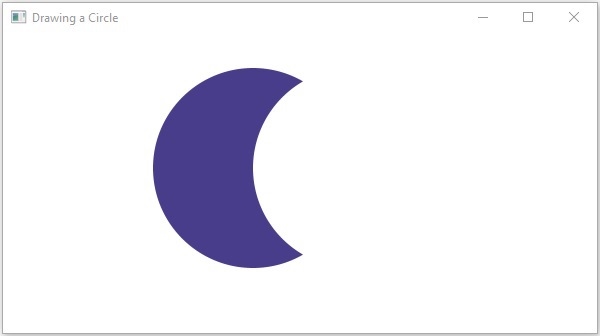
Example
Now, let us try to perform subtraction operation on two ellipses where we will subtract the area of second ellipse from the first ellipse. Save this file under the name EllipseSubtractionOperation.java.
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.paint.Color; import javafx.stage.Stage; import javafx.scene.shape.Ellipse; import javafx.scene.shape.Shape; public class EllipseSubtractionOperation extends Application { @Override public void start(Stage stage) { Ellipse ellipse1 = new Ellipse(); ellipse1.setCenterX(250.0f); ellipse1.setCenterY(100.0f); ellipse1.setRadiusX(150.0f); ellipse1.setRadiusY(75.0f); ellipse1.setFill(Color.BLUE); Ellipse ellipse2 = new Ellipse(); ellipse2.setCenterX(350.0f); ellipse2.setCenterY(100.0f); ellipse2.setRadiusX(150.0f); ellipse2.setRadiusY(75.0f); ellipse2.setFill(Color.RED); Shape shape = Shape.subtract(ellipse1, ellipse2); //Setting the fill color to the result shape.setFill(Color.DARKSLATEBLUE); //Creating a Group object Group root = new Group(shape); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Subtraction Example"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
Compile and execute the saved java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls EllipseSubtractionOperation.java java --module-path %PATH_TO_FX% --add-modules javafx.controls EllipseSubtractionOperation
Output
On executing, the above program generates a JavaFX window displaying the following output −
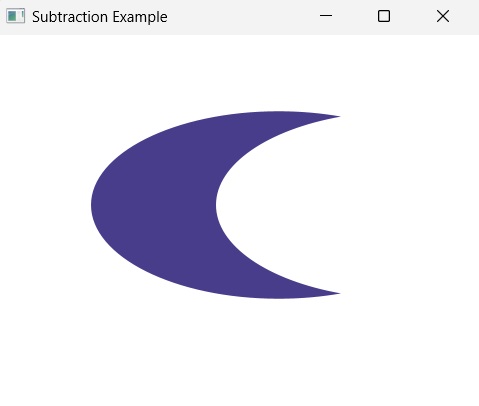