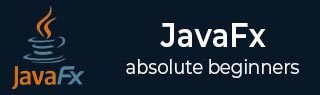
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Environment
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Path Objects
- JavaFX - Path Objects
- JavaFX - LineTo Path Object
- JavaFX - HLineTo Path Object
- JavaFX - VLineTo Path Object
- JavaFX - QuadCurveTo Path Object
- JavaFX - CubicCurveTo Path Object
- JavaFX - ArcTo Path Object
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX - Linear Gradient Pattern
- JavaFX - Radial Gradient Pattern
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX - Color Adjust Effect
- JavaFX - Color input Effect
- JavaFX - Image Input Effect
- JavaFX - Blend Effect
- JavaFX - Bloom Effect
- JavaFX - Glow Effect
- JavaFX - Box Blur Effect
- JavaFX - GaussianBlur Effect
- JavaFX - MotionBlur Effect
- JavaFX - Reflection Effect
- JavaFX - SepiaTone Effect
- JavaFX - Shadow Effect
- JavaFX - DropShadow Effect
- JavaFX - InnerShadow Effect
- JavaFX - Lighting Effect
- JavaFX - Light.Distant Effect
- JavaFX - Light.Spot Effect
- JavaFX - Point.Spot Effect
- JavaFX - DisplacementMap
- JavaFX - PerspectiveTransform
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX - Rotation Transformation
- JavaFX - Scaling Transformation
- JavaFX - Translation Transformation
- JavaFX - Shearing Transformation
- JavaFX Animations
- JavaFX - Animations
- JavaFX - Rotate Transition
- JavaFX - Scale Transition
- JavaFX - Translate Transition
- JavaFX - Fade Transition
- JavaFX - Fill Transition
- JavaFX - Stroke Transition
- JavaFX - Sequential Transition
- JavaFX - Parallel Transition
- JavaFX - Pause Transition
- JavaFX - Path Transition
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - Stroke Type Property
Two dimensional(2D) shapes, in geometry, are usually referred to as structures that has only two dimensions of measure, commonly length and breadth, and lie on an XY plane. These structures can either be open or closed figures.
Open figures include curves like Cubic curve, Quadrilateral curve, etc. whereas closed figures include all types of polygons, circles etc.
In JavaFX, these 2D shapes can be drawn on an application by importing the javafx.scene.shape package. However, to improve the quality of a shape, there are various properties and operations that can be performed on it.
In this chapter, we will learn about Stroke Type property in detail.
Stroke Type Property
The Stroke Type property of 2D shapes is used to specify the type of boundary line a 2D shape must have. In JavaFX, this property is set using the StrokeType class. You can set the type of the stroke using the method setStrokeType() as follows −
Path.setStrokeType(StrokeType.stroke_type);
The stroke_type of a shape can be −
Inside − The boundary line will be drawn inside the edge (outline) of the shape (StrokeType.INSIDE).
Outside − The boundary line will be drawn outside the edge (outline) of the shape (StrokeType.OUTSIDE).
Centered − The boundary line will be drawn in such a way that the edge (outline) of the shape passes exactly thorough the center of the line (StrokeType.CENTERED).
By default, the stroke type of a shape is centered. Following is the diagram of a triangle with different Stroke Types −
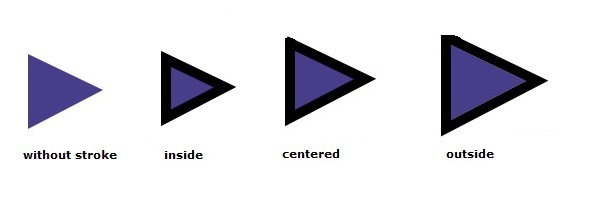
Example
Let us see an example demonstrating the usage of StrokeType property on a 2D shape. Save this file with the name StrokeTypeExample.java.
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.shape.Polygon; import javafx.scene.shape.StrokeType; import javafx.scene.paint.Color; import javafx.stage.Stage; public class StrokeTypeExample extends Application { @Override public void start(Stage stage) { //Creating a Triangle Polygon triangle = new Polygon(); //Adding coordinates to the polygon triangle.getPoints().addAll(new Double[]{ 100.0, 50.0, 170.0, 150.0, 100.0, 250.0, }); triangle.setFill(Color.BLUE); triangle.setStroke(Color.BLACK); triangle.setStrokeWidth(7.0); triangle.setStrokeType(StrokeType.CENTERED); //Creating a Group object Group root = new Group(triangle); //Creating a scene object Scene scene = new Scene(root, 300, 300); //Setting title to the Stage stage.setTitle("Drawing a Triangle"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
Compile and execute the saved java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls StrokeTypeExample.java java --module-path %PATH_TO_FX% --add-modules javafx.controls StrokeTypeExample
Output
On executing, the above program generates a JavaFX window displaying a triangle with centered stroke type as shown below.
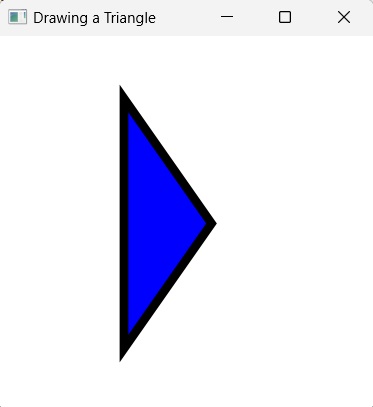
Example
Let us see an example demonstrating the usage of INSIDE StrokeType property on a 2D shape. We are using a larger width here in order to properly show the difference between INSIDE and CENTERED stroke types from previous example. Save this file with the name StrokeTypeEllipse.java.
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.shape.Ellipse; import javafx.scene.shape.StrokeType; import javafx.scene.paint.Color; import javafx.stage.Stage; public class StrokeTypeEllipse extends Application { @Override public void start(Stage stage) { //Drawing an ellipse Ellipse ellipse = new Ellipse(); //Setting the properties of the ellipse ellipse.setCenterX(150.0f); ellipse.setCenterY(100.0f); ellipse.setRadiusX(100.0f); ellipse.setRadiusY(50.0f); ellipse.setFill(Color.ORANGE); ellipse.setStroke(Color.BLACK); ellipse.setStrokeWidth(20.0); ellipse.setStrokeType(StrokeType.INSIDE); //Creating a Group object Group root = new Group(ellipse); //Creating a scene object Scene scene = new Scene(root, 300, 300); //Setting title to the Stage stage.setTitle("Drawing a Ellipse"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
Compile and execute the saved java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls StrokeTypeEllipse.java java --module-path %PATH_TO_FX% --add-modules javafx.controls StrokeTypeEllipse
Output
On executing, the above program generates a JavaFX window displaying a triangle with centered stroke type as shown below.
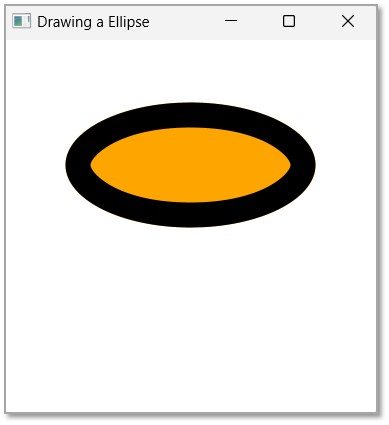