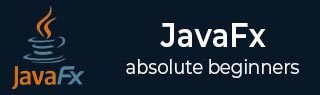
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX Animations
- JavaFX - Animations
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - Spinner
A Spinner is a UI control that allows the user to select a value from a predefined range or a ordered sequence. It can be either editable or non-editable. If it is editable, the user can type in a value otherwise not. It also provides up and down arrows so that a user can step through the values of sequence. The figure below illustrates a spinner −
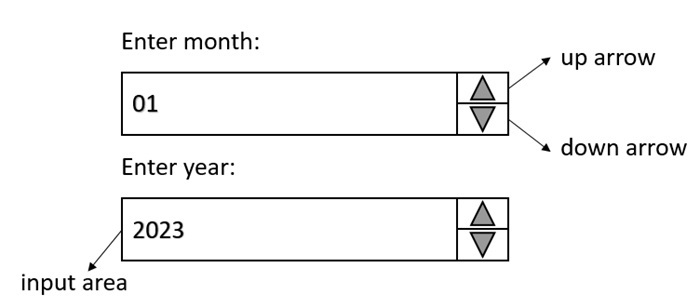
Creating a Spinner in JavaFX
In JavaFX, the spinner is created by instantiating a class named Spinner. This class belongs to the package javafx.scene.control. Some of the widely used constructors of the Spinner class are listed below −
Spinner() − It is used to create an empty spinner.
Spinner(double minVal, double maxVal, double initialVal) − It creates a new spinner with the specified minimum, maximum and initial values.
Spinner(double minVal, double maxVal, double initialVal, double valToStepBy) − It is used to construct a new spinner with the specified minimum, maximum and initial values along with the increment amount.
Example
The following JavaFX program demonstrates how to create a spinner in JavaFX application. Save this code in a file with the name JavafxSpinner.java.
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Spinner; import javafx.scene.layout.VBox; import javafx.stage.Stage; import javafx.scene.control.Label; import javafx.geometry.Pos; public class JavafxSpinner extends Application { @Override public void start(Stage stage) { // creating a label Label newlabel = new Label("Sample Spinner: "); // creating spinner and setting min, max, initial value Spinner newSpinner = new Spinner(0, 100, 25); // vbox to hold spinner VBox vbox = new VBox(newlabel, newSpinner); vbox.setAlignment(Pos.CENTER); // creating stage and scene Scene scene = new Scene(vbox, 400, 300); stage.setScene(scene); stage.setTitle("Spinner in JavaFX"); stage.show(); } public static void main(String[] args) { launch(args); } }
To compile and execute the saved Java file from the command prompt, use the following commands −
javac --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxSpinner.java java --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxSpinner
Output
When we execute the above code, it will generate the following output.
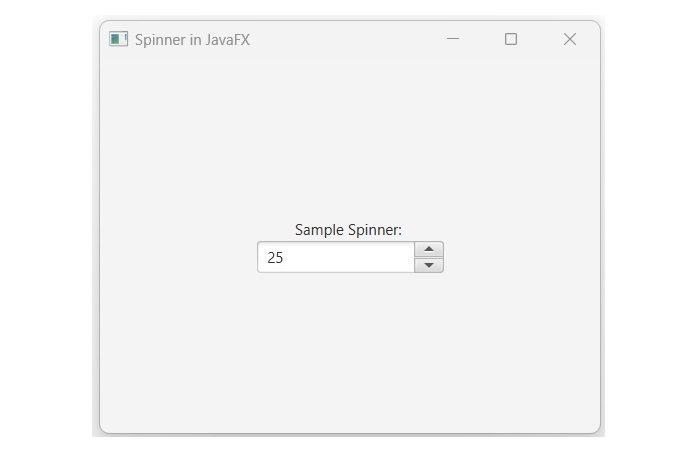
Setting the Size of Spinner
To set the size of a spinner, we can use setPrefSize() method. It is a built-in method which accepts heigth and width as a parameter.
Example
In the following example, we are going to create a Spinner of the specified size in JavaFX application. Save this code in a file with the name DemoSpinner.java.
import javafx.application.Application; import javafx.geometry.Insets; import javafx.scene.Scene; import javafx.scene.control.Spinner; import javafx.scene.layout.HBox; import javafx.scene.layout.VBox; import javafx.stage.Stage; import javafx.scene.control.Label; import javafx.geometry.Pos; public class DemoSpinner extends Application { @Override public void start(Stage stage) { // creating labels for spinner Label newlabel = new Label("Enter Date of Birth: "); Label setYear = new Label("Year: "); Label setMonth = new Label("Month: "); Label setDay = new Label("Day: "); // creating spinners and setting sizes Spinner year = new Spinner(1998, 2020, 2000); year.setPrefSize(65, 25); Spinner month = new Spinner(1, 12, 1); month.setPrefSize(60, 25); Spinner day = new Spinner(1, 31, 1); day.setPrefSize(60, 25); // HBox to hold labels and spinners HBox box1 = new HBox(); box1.setPadding(new Insets(15, 12, 15, 12)); box1.setSpacing(10); box1.getChildren().addAll(setYear, year, setMonth, month, setDay, day); // VBox to hold HBox and Label VBox box2 = new VBox(); box2.setAlignment(Pos.CENTER); box2.setPadding(new Insets(15, 12, 15, 12)); box2.setSpacing(10); box2.getChildren().addAll(newlabel, box1); // creating scene and stage Scene scene = new Scene(box2, 400, 400); stage.setScene(scene); stage.setTitle("Spinner in JavaFX"); stage.show(); } public static void main(String[] args) { launch(args); } }
Compile and execute the saved Java file from the command prompt by using the following commands −
javac --module-path %PATH_TO_FX% --add-modules javafx.controls DemoSpinner.java java --module-path %PATH_TO_FX% --add-modules javafx.controls DemoSpinner
Output
On executing the above code, it will generate the following output.
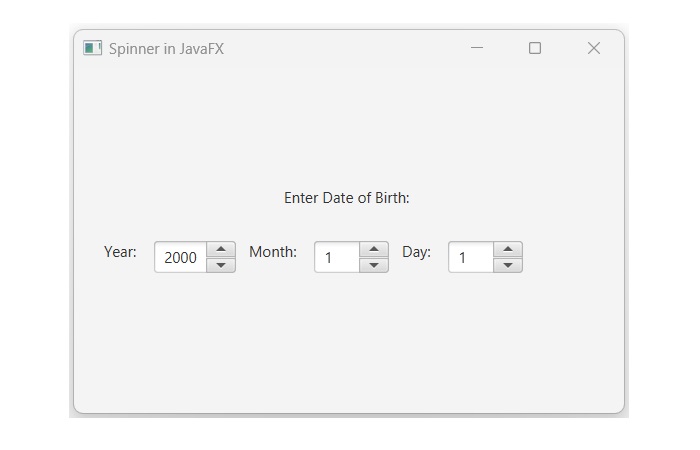