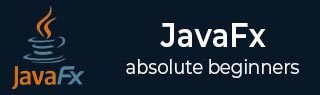
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX Animations
- JavaFX - Animations
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - ProgressIndicator
A progress indicator is a UI control that is used to indicate the progress of a task to the user. It is often used with the Task API to notify users about the progress of background tasks and how much time will be required to complete the user action. In this tutorial, we are going to learn how to create and use progress indicator in JavaFX. Before that, let's see how a general progress indicator looks like −
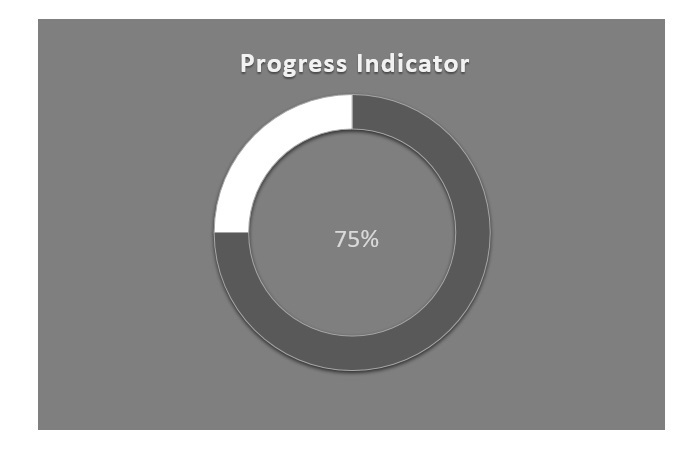
ProgressIndicator in JavaFX
In JavaFX, the progress indicator is represented by a class named ProgressIndicator. This class belongs to the package javafx.scene.control. By instantiating this class, we can create a progress indicator in JavaFX. Constructors of the ProgressIndicator class are listed below −
ProgressIndicator() − It constructs a new progress indicator without initial progress value.
ProgressIndicator(double progress) − It constructs a new progress indicator with a specified initial progress value.
Example
The following program demonstrates how to create a progress indicator in JavaFX. Save this code in a file with the name ProgressindicatorJavafx.java.
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.ProgressIndicator; import javafx.stage.Stage; public class ProgressindicatorJavafx extends Application { @Override public void start(Stage stage) { // Creating a progress indicator without an initial progress value ProgressIndicator progress = new ProgressIndicator(); // Create a scene and stage Scene scene = new Scene(progress, 400, 300); stage.setScene(scene); stage.setTitle("Progress Indicator in Javafx"); stage.show(); } public static void main(String[] args) { launch(args); } }
To compile and execute the saved Java file from the command prompt, use the following commands −
javac --module-path %PATH_TO_FX% --add-modules javafx.controls ProgressindicatorJavafx.java java --module-path %PATH_TO_FX% --add-modules javafx.controls ProgressindicatorJavafx
Output
When we execute the above code, it will generate the following output.
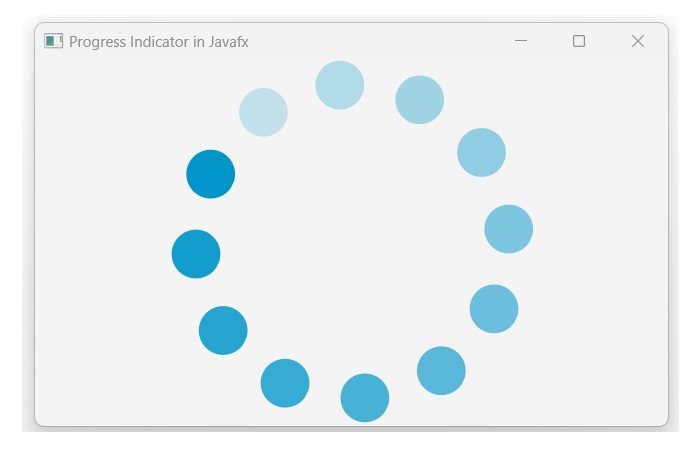
ProgressIndicator with an Initial Progress Value
We can set the initial progress value to the progress indicator by using the parameterized constructor of the ProgressIndicator class. The constructor accepts a progress value as an argument which is of double type ranging between 0.0 and 1.0. Here, 0.0 means no progress and 1.0 means complete. If the progress value is negative, the progress indicator is indeterminate, meaning that it does not show any specific progress amount.
Example
In the following example, we are creating a progress indicator initialized with a specific progress value. Additionally, we are also creating two buttons labelled 'Increase' and 'Decrease'. Clicking the 'Increase' button increments the progress value, while clicking 'Decrease' decrements it. Save this code in a file with the name Javafxprogress.java.
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.control.ProgressIndicator; import javafx.scene.layout.HBox; import javafx.stage.Stage; public class Javafxprogress extends Application { @Override public void start(Stage stage) { // Create a progress indicator with an initial progress of 0.5 ProgressIndicator progressIndctr = new ProgressIndicator(0.5); // Create a button that increases the progress by 0.1 Button buttonOne = new Button("Increase"); buttonOne.setOnAction(e -> { // Get the current progress value double progress = progressIndctr.getProgress(); // Increase the progress by 0.1 progress += 0.1; // Set the new progress value progressIndctr.setProgress(progress); }); // Create second button that decreases the progress by 0.1 Button buttonTwo = new Button("Decrease"); buttonTwo.setOnAction(e -> { // Get the current progress value double progress = progressIndctr.getProgress(); // Decrease the progress by 0.1 progress -= 0.1; // Set the new progress value progressIndctr.setProgress(progress); }); // Create an HBox to hold the progress indicator and the button HBox hbox = new HBox(10); hbox.getChildren().addAll(progressIndctr,buttonOne, buttonTwo); // Create a scene with the HBox and set it to the stage Scene scene = new Scene(hbox, 400, 300); stage.setScene(scene); stage.setTitle("Progress Indicator in JavaFX"); stage.show(); } public static void main(String[] args) { launch(args); } }
Compile and execute the saved Java file from the command prompt by using the following commands −
javac --module-path %PATH_TO_FX% --add-modules javafx.controls Javafxprogress.java java --module-path %PATH_TO_FX% --add-modules javafx.controls Javafxprogress
Output
On executing the above code, it will generate the following output.
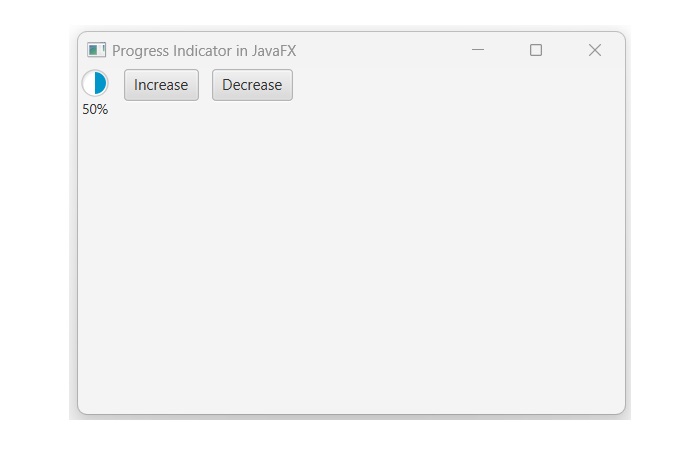