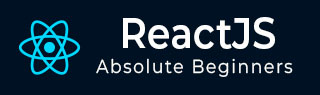
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - Reference API
React JS is a JavaScript library for building user interfaces. React allows developers to create interactive UI components efficiently. So this reference attempts to document every built-in function, hooks, components, and other essential functionalities provided by React JS. In this tutorial we will use the React@18 version.
In the React JS, functions are organized into two main sections based on their functionalities and usage. Here are the main functions in React JS
Built-in Hooks
In this we have included all the built-in hooks which can be used with different React features in your components.
S.No | Function & Description |
---|---|
1 | use() Lets us read the value of a resource. |
2 | useDebugValue() Add a label to a custom Hook. |
3 | useDeferredValue() Lets us defer updating a portion of the User Interface. |
4 | useId() Use for generating unique IDs. |
5 | useImperativeHandle() Helps us connect with a child component. |
6 | useInsertionEffect() Allows us for inserting elements into the DOM. |
7 | useLayoutEffect() Used to perform the layout measurements. |
8 | useSyncExternalStore() Lets us subscribe to an external store. |
9 | useTransition() Help us to update the state without blocking the UI. |
Built-in Component
In this we have documented components you can use in your code and other components as well.
S.No | Function & Description |
---|---|
1 | <Suspense> Display a fallback until its children have finished loading. |
Built-in React APIs
There are some built-in APIs React provides which are useful for defining components.
S.No | Function & Description |
---|---|
1 | cache() Cache the result of a data fetch. |
2 | createContext() It creates a context that components can provide. |
3 | lazy() Allows us to delay loading a component's code. |
4 | startTransition() Update the state without blocking the UI. |
Directives
These are the instructions for bundlers compatible with React Server Components.
S.No | Function & Description |
---|---|
1 | use client Used to mark what code runs on the client. |
2 | use server It is used to mark server-side functions. |
Built-in React DOM Hooks
There are some built-in React DOM hooks which run in the browser DOM environment. These Hooks are not best fit for non browser environments like Android, iOS, and Windows applications.
S.No | Function & Description |
---|---|
1 | useFormState() Update state as per the result of a form action. |
2 | useFormStatus() Gives status information of the last form submission. |
Event Handling Functions
In this section we have included some event handling functions that supports all built−in HTML and SVG components.
S.No | Function & Description |
---|---|
1 | event object Acts as a link between our code and the browser events. |
2 | AnimationEvent handler An event handler type for the CSS animation events. |
3 | ClipboardEvent handler It is an event handler type for the Clipboard API events. |
4 | CompositionEvent handler It is an event handler type for the input method editor events. |
5 | DragEvent handler Event handler type for the HTML Drag and Drop API events. |
6 | FocusEvent handler An event handler type for the focus events. |
7 | InputEvent handler An event handler type for the onBeforeInput event. |
8 | KeyboardEvent handler Handles events for keyboard events. |
9 | MouseEvent handler An event handler type for mouse events. |
10 | PointerEvent handler It is an event handler type for pointer events. |
11 | TouchEvent handler Event handler type for touch events. |
12 | TransitionEvent handler An event handler type for the CSS transition events. |
13 | UIEvent handler Event handler type for generic User Interface events. |
14 | WheelEvent handler An event handler type for the onWheel event. |
React DOM APIs
The react-dom package contains methods that are only supported for the web applications. In this section we have included APIs, Client APIs and server APIs.
S.No | Function & Description |
---|---|
1 | createPortal() Get the current Axes instance. |
2 | flushSync() Create a new Axes instance. |
3 | findDOMNode() Close the current figure. |
4 | createRoot() Clear the current figure. |
5 | hydrateRoot() Check if a figure with a given figure number exists. |
6 | renderToReadableStream() Create a new figure. |
7 | renderToString() Get the current figure. |
8 | renderToStaticMarkup() Get the labels of all figures. |
9 | cloneElement() Set the current Axes instance to a given axes. |
10 | isValidElement() Add contour labels to a contour plot. |
11 | PureComponent It is an enhanced version of the regular Component. |
Other Class components
These components are the base class for the React components defined as JavaScript classes. Class components are still supported by React so we have included them in the below section.
S.No | Function & Description |
---|---|
1 | componentDidCatch() Used to call when some child component throws an error. |
2 | componentDidUpdate() Used to call immediately after component has been re−rendered. |
3 | componentWillUnmount() Call it before your component is removed. |
4 | forceUpdate() Forces a component to re-render. |
5 | getChildContext() Specify the values for the legacy context. |
6 | getSnapshotBeforeUpdate() Enables your component to capture some information. |
7 | static contextType Lets us specify which legacy context is consumed. |
8 | static defaultProps Used to set the default props for the class. |
9 | static propTypes To declare the types of the props accepted by your component. |
10 | static getDerivedStateFromError() Call it when a child component throws an error during rendering. |
11 | static getDerivedStateFromProps() Used to call it right before calling render. |
12 | UNSAFE_componentWillMount() It is a lifecycle method that runs during server rendering. |
13 | UNSAFE_componentWillReceiveProps() Used to call when the component receives new props. |
14 | UNSAFE_componentWillUpdate() Call it before rendering with the new props or state. |
15 | createElement() Create a React element for an alternative to writing JSX. |
16 | createRef() Creates a ref object to contain arbitrary value. |
To Continue Learning Please Login