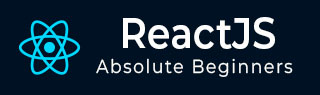
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - Carousel
React provides carousel component through third party UI component library. React community provides a large collection of UI / UX components and it is tough to choose the right library for our requirement. Bootstrap UI library is one of the popular choice for the developer and it is extensively used. React Bootstrap (https://react-bootstrap.github.io/) has ported almost all the bootstrap UI components to the React library and it has best support for Carousel component as well.
Let us learn how to use Carousel component from react-bootstrap library in this chapter.
What is Carousel?
Carousel is basically a slideshow cycling through a series of content with rich animation support. It accepts a series of images as its main content. It also accepts title content for each slides. It has buttons / indicators to navigate from current content to the next / previous content. The time duration to pause and show a content can be configured as per requirement.
Carousel component
Carousel component allows the developer to create simple carousel with bootstrap design in a web application. Carousel component accepts two components,
Carousel.Item
Carousel component accepts multiple Carousel.Item items. Each Carousel.Items is a slide and can accepts a image. A sample code is as follows −
<Carousel> <Carousel.Item> <img className="d-block w-100" src="react_bootstrap_carousel_slide1.png" alt="First slide" /> </Carousel.Item> <Carousel.Item> <img className="d-block w-100" src="react_bootstrap_carousel_slide2.png" alt="Second slide" /> </Carousel.Item> </Carousel>
Carousel.Caption
Carousel.Caption is a special component to show a short description about the slide and it should be included inside the Carousel.Item component. A sample code is as follows −
<Carousel.Item> <img className="d-block w-100" src="react_bootstrap_carousel_slide1.png" alt="First slide" /> <Carousel.Caption> <h3>React Bootstrap</h3> <p>React component library providing bootstrap components</p> </Carousel.Caption> </Carousel.Item>
Carousel component accepts a small set of props to configure the functionality and they are follows,
controls (boolean)
Enables / Disables the controls such as previous / next buttons
keyboard (boolean)
Enables keyboard controls
touch (boolean)
Enables / Disable touch controls
indicators (boolean)
Enables / Disables the indicators at the bottom of the carousel
nextIcon (React node)
Option to customize custom next icons
nextLabel (string)
Option to customize next label
prevIcon (React Node)
Option to customize custom previous icons
prevLabel (string)
Option to customize previous label
interval (number)
Time duration to pause and play between two slide
activeIndex (number)
Represent the index number of the slide to be shown
slide (boolean)
Enable / disable auto slide feature
variant (dark)
Enables the different variant of the carousel design. dark option changes the carousel theme from light to dark
bsPrefix (string)
Prefix used to customize the underlying CSS classes
onSelect (function)
Enables to attach a function to handle onSelect event
onSlide (function)
Enables to attach a function to handle onSlide event
Carousel.Item component accepts a few props to configure the functionality and they are follows,
interval (number)
Time duration to pause for the individual slide
bsPrefix (string)
Prefix used to customize the underlying CSS classes
Applying Carousel component
First of all, create a new react application and start it using below command.
create-react-app myapp cd myapp npm start
Next, install the bootstrap and react-bootstrap library using below command,
npm install --save bootstrap react-bootstrap
Next, create a simple carousel component, SimpleCarousel (src/Components/SimpleCarousel.js) and render a carousel as shown below −
import { Carousel } from 'react-bootstrap'; function SimpleCarousel() { return ( <Carousel fade indicators={false} controls={false}> <Carousel.Item> <img className="d-block w-100" src="react_bootstrap_carousel_slide1.png" alt="First slide" /> <Carousel.Caption> <h3>React Bootstrap</h3> <p>React component library providing bootstrap components</p> </Carousel.Caption> </Carousel.Item> <Carousel.Item> <img className="d-block w-100" src="react_bootstrap_carousel_slide2.png" alt="Second slide" /> <Carousel.Caption> <h3>Button</h3> <p>React Bootstrap button component</p> </Carousel.Caption> </Carousel.Item> <Carousel.Item> <img className="d-block w-100" src="react_bootstrap_carousel_slide3.png" alt="Third slide" /> <Carousel.Caption> <h3>Carousel</h3> <p>React bootstrap Carousel component</p> </Carousel.Caption> </Carousel.Item> </Carousel> ); } export default SimpleCarousel;
Here,
Imported Carousel component and added a single Carousel component.
Used fade props in Carousel component to change the animation type
Used indicators props in Carousel component to remove indicators
Used controls props in Carousel component to remove controls
Added three Carousel.Item items and used three images.
Added Carousel.Caption in each Carousel.Item component and set the captions for each slide.
Next, open App.css (src/App.css) and remove all the styles.
// remove all default styles
Next, open App component (src/App.js), import the bootstrap css and update the content with our new carousel component as shown below −
import './App.css' import "bootstrap/dist/css/bootstrap.min.css"; import SimpleCarousel from './Components/SimpleCarousel' function App() { return ( <div className="container"> <div style={{ padding: "10px" }}> <div style={{ width: "400px", height: "400px", backgroundColor: "skyblue" }}> <SimpleCarousel /> </div> </div> </div> ); } export default App;
Here,
Imported the bootstrap classes using import statement
Rendered our new SimpleCarousel component.
Included the App.css style
Finally, open the application in the browser and check the final result. Carousel component will be rendered as shown below −
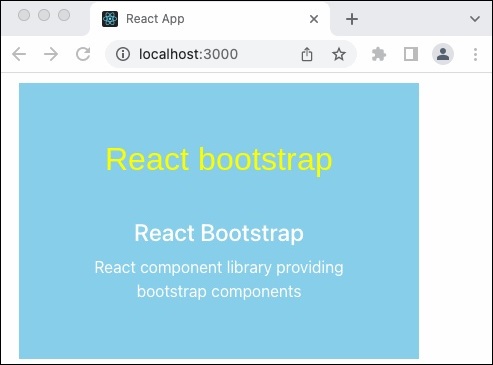
Add controls and indicators
Let us update our component to include controls to navigate next and previous slide and also indicator to identify the current slide position.
First of all, open our carousel application and update the SimpleCarousel component as shown below −
import { Carousel } from 'react-bootstrap'; function SimpleCarousel() { return ( <Carousel fade indicators={true} controls={true}> <Carousel.Item> // ...
Here,
Used indicators props to enable indicators
Used controls props to enable controls
Next, open the application in the browser and check the final result. Carousel component will be rendered with controls and indicators as shown below −
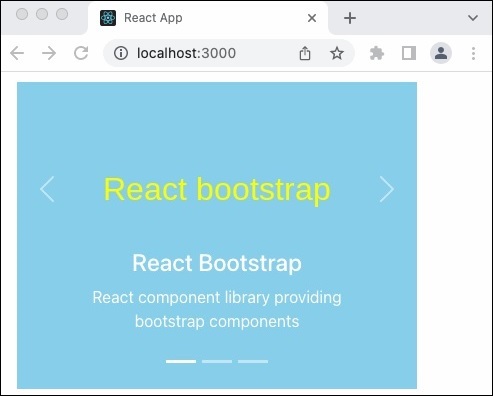
Summary
React-bootstrap Carousel component provides all necessary options to create a clean and simple carousel component.