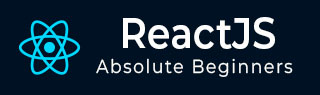
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - forceUpdate() Method
Components in React act as building blocks for a website or app. They can show multiple things and perform different actions. Usually, a component only changes or re-renders when its own state or the information it gets from outside (referred to as props) changes.
React components will re-render only if the component's state or the props given to it change, but if we need to re-render the component if any data changes, we use React's forceUpdate() method. Calling forceUpdate() will forcibly re-render the component, ignoring the shouldComponentUpdate() method and instead calling the render() method of the component.
So, forceUpdate() is a way to make a React component refresh itself, even if it doesn’t think it needs to.
Syntax
forceUpdate(callbackFunc)
Parameters
callbackFunc − It is an optional callback function If we define a callback, React will execute it after the update is committed.
Return Value
This function does not give anything in return.
Examples
Example 1
Let us create a basic React app that shows how to use the forceUpdate function. In this example, we will have a component that gets a random number from an external API and updates the UI when a button is clicked.
NumberDisplay.js
import React, { Component } from 'react'; class NumberDisplay extends Component { constructor() { super(); this.state = { randomNumber: null, }; } fetchData = async () => { // An API call to get a random number const response = await fetch('https://www.random.org/integers/?num=1&min=1&max=100&format=plain'); const data = await response.text(); this.setState({ randomNumber: parseInt(data) }); }; forceUpdateHandler = () => { // Use forceUpdate to manually update the component this.forceUpdate(); }; render() { return ( <div> <h1>Random Number: {this.state.randomNumber}</h1> <button onClick={this.fetchData}>Fetch New Number</button> <button onClick={this.forceUpdateHandler}>Force Update</button> </div> ); } } export default NumberDisplay;
App.js
import React from 'react'; import NumberDisplay from './NumberDisplay'; function App() { return ( <div> <h1>ForceUpdate Example App</h1> <NumberDisplay /> </div> ); } export default App;
Output
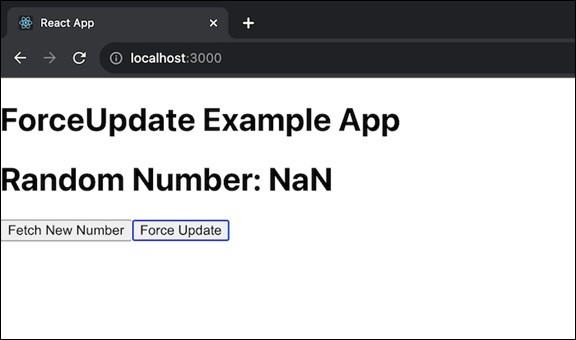
Example 2
Let us say we have a simple app that counts things. This Counter App has a number displayed on the screen, starting at 0. We can increase the count by clicking the "Increase Count" button. But, there is another button labeled "Force Update." When we click it, even if we didn't increase the count, the app will refresh and show the current count again. The forceUpdate() function is used here to make sure the display is always up to date.
import React from 'react'; class CounterApp extends React.Component { constructor() { super(); this.state = { count: 0 }; } increaseCount() { this.setState({ count: this.state.count + 1 }); } forceUpdateCounter() { this.forceUpdate(); } render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={() => this.increaseCount()}>Increase Count</button> <button onClick={() => this.forceUpdateCounter()}>Force Update</button> </div> ); } } export default CounterApp;
Output
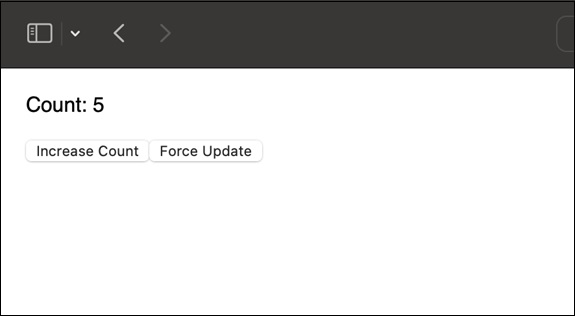
Example 3
Now let us create a Message App. This program displays a message on the screen, which is set to "Hello, world!" at first. By clicking the "Change Message" button, we can change the message. But there is a "Force Update" button. When we click this button, the app will refresh and display the current message. To achieve this refreshing behavior, the forceUpdate() function is needed.
import React from "react"; class MessageApp extends React.Component { constructor() { super(); this.state = { message: "Hello, world!" }; } changeMessage(newMessage) { this.setState({ message: newMessage }); } forceUpdateMessage() { this.forceUpdate(); } render() { return ( <div> <p>{this.state.message}</p> <button onClick={() => this.changeMessage("New Message")}> Change Message </button> <button onClick={() => this.forceUpdateMessage()}>Force Update</button> </div> ); } } export default MessageApp;
Output
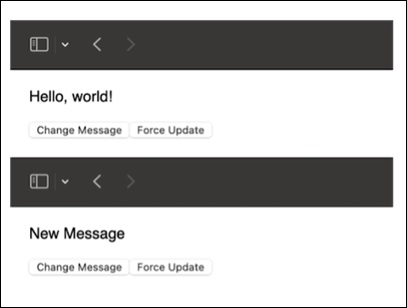
Limitations
React will refresh without checking shouldComponentUpdate if we use forceUpdate.
Summary
For React components, forceUpdate works similarly like a refresh button. While it is not something we are going to use on a regular basis, it can be useful when our component receives data from an external source. Remember that it is normally preferable to let React handle updates automatically, but forceUpdate is available if we require more control.
To Continue Learning Please Login