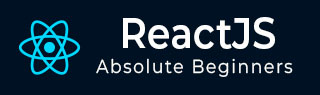
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - createRef() Function
createRef() is a React tool for managing and interacting with items in our application. It is often used in class components, but we will also discuss its equivalent, useRef(), which is commonly used in function components.
It is similar to a container that can hold an array of items. It is used in React for creating a reference object within class components. This reference object has a property called current that can be adjusted to various values.
Syntax
class MyClass extends Component { classRef = createRef(); // ... }
In this example, classRef is a reference to something, and we can use it to interact with that something in our component.
Parameters
createRef() does not accept any arguments.
Return Value
When we call it, it returns an object with the current property set to null by default. This attribute can later be changed to refer to different elements in our component.
Examples
Example 1
import React, { createRef, Component } from 'react'; import './App.css'; class App extends Component { inputRef = createRef(); componentDidMount() { this.inputRef.current.focus(); } render() { return <div className='App'><input ref={this.inputRef} /> </div> } } export default App;
Output

When we start the program, the typing cursor is immediately placed inside a box, ready for us to start typing. This is useful in cases where we want users to have a smooth and focused input experience, like online forms or search bars.
Example 2
In this app we have set a timer to tick every second. It can be used to display time that has passed or to start events at regular intervals. The timer begins when the app loads and ends when the app is closed.
import React, { createRef, Component } from 'react'; import './App.css'; class TimerComponent extends Component { timerRef = createRef(); componentDidMount() { this.timerRef.current = setInterval(() => { console.log('Timer tick'); }, 1000); } componentWillUnmount() { clearInterval(this.timerRef.current); } render() { return <div>Timer Component</div>; } } export default TimerComponent;
Output
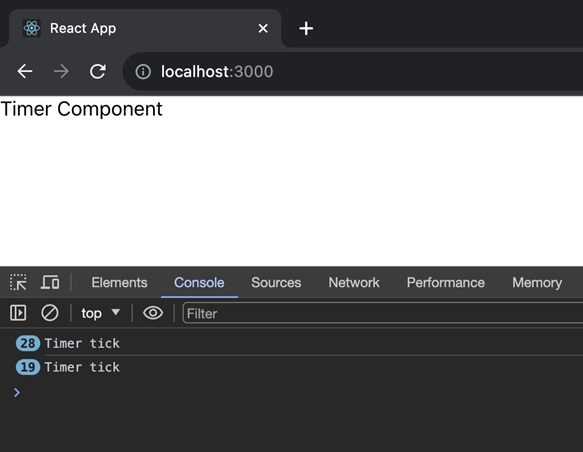
This application is all about managing time-sensitive tasks. When we launch the app, a timer begins ticking every second. It can be handy in situations when we need to keep time or trigger certain events at regular intervals. When we exit the app, the timer stops.
Example 3
When we click a button in this app, the color of a box changes to light blue. It shows how to use React to easily interact with and change the appearance of items on our website. This is useful for creating interactive and dynamic user interfaces.
import React, { createRef, Component } from 'react'; class App extends Component { divRef = createRef(); handleClick = () => { this.divRef.current.style.backgroundColor = 'lightblue'; }; render() { return <div ref={this.divRef} onClick={this.handleClick}><h1>Click me!</h1></div>; } } export default App;
Output

After running the App, which allows us to quickly interact with webpage elements. When we click a button, the color of a box changes to light blue. This example shows how React can let us easily adjust and control the look of items on our webpage.
Limitations
It is worth noting that every time we call createRef(), we get a new object. This means that if we compare two refs, even if they were created via createRef(), they are distinct objects. This is great for class components, but in function components, we can choose to useRef(), which always returns the same object.
Summary
createRef() is a useful function in React, particularly for class components. It allows us to easily reference and interact with elements. In function components, we might need to consider using useRef() for the same reference object.
To Continue Learning Please Login