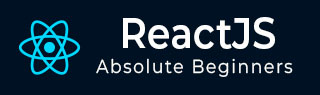
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - UIEvent Handler
Building websites and apps that are easy to use and can change as we interact with them is a big deal in web development. Imagine when we click on a button, scroll down a webpage, or type something on a keyboard - these actions are what we call user interface events. They are like the building blocks that let us do things on a website. So we will look at the UIEvent handlers in this tutorial.
First, let's define a UIEvent. It is a term used to describe simple user interface events on a web page like clicking a link, scrolling down a page, or moving our mouse. UIEvent is a member of an event type family tree that includes MouseEvent, TouchEvent, FocusEvent, and others. Each of them shows a distinct activity that a user can do on a website.’
Syntax
<div onScroll={e => console.log('I am in onScroll method')} />
Parameters
e − It is a React event object.
UIEvent Properties
Sr.No | Properties & Description |
---|---|
1 | UIEvent.detail A number that varies based on the event type, providing specific details about the event. |
2 | UIEvent.sourceCapabilities Provides information about the physical device responsible for generating a touch event. |
3 | UIEvent.view Returns a reference to the browser window or frame that generated the event. |
Examples
Example
We can use the onScroll event on a div element in a React component to determine when the user scrolls inside that div. When a scroll event happens, the event handler becomes active, helping us to do some action. Here is the code for our App.js file −
We have imported React and created the App functional component. We defined the onScroll function, which is the onScroll event handler. When the div is scrolled in this example, it logs 'I am onScroll' to the console.
We insert a div element within the return statement with the onScroll property set to the onScroll method. We also add additional CSS styles to the div to make it scrollable.
import React from "react"; function App() { const onScroll = (e) => { console.log("I am onScroll"); }; return ( <div onScroll={onScroll} style={{ height: "200px", // Add fixed height to create scrollable div overflow: "scroll" // Add scrollbars }} > <div style={{ height: "600px" }}> Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum. </div> </div> ); } export default App;
Output
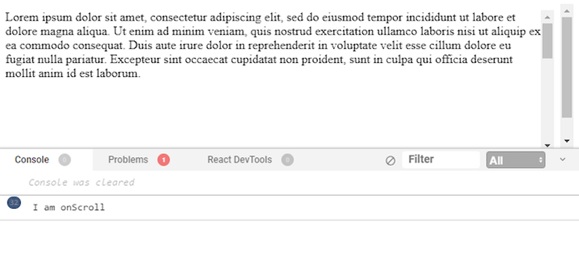
When we start our React application and scroll within the div, the console will display 'I am onScroll' each time the scroll event happens. This is a simple example of how to respond to user scroll actions in a React component with the onScroll event handler.
Example − Scroll Tracker App
This app tracks and displays the current vertical scroll position of the page as we scroll down. It uses the onScroll event to update the scroll position and displays it on the page. The code is given below −
import React, { useState } from 'react'; function App() { const [scrollPosition, setScrollPosition] = useState(0); const handleScroll = () => { setScrollPosition(window.scrollY); }; window.addEventListener('scroll', handleScroll); return ( <div> <h1>Scroll Tracker</h1> <p>Scroll Position: {scrollPosition}</p> <div style={{ height: '100vh', border: '1px solid #ccc' }}> Scroll down to see the position. </div> </div> ); } export default App;
Output
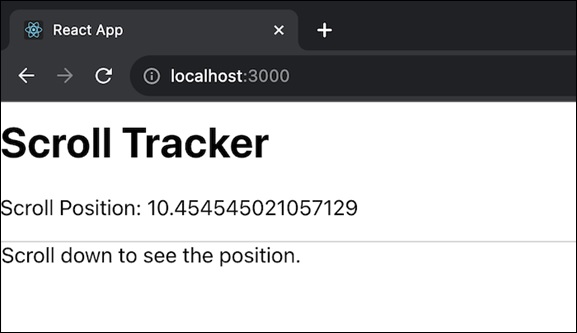
Example − Scroll to Top Button
In this app we will have a "Scroll to Top" button that becomes visible as we scroll down the page. When we click the button smoothly scrolls the page to the top. It utilizes the onScroll event to determine when to show the button, and a scrollTo function is triggered when the button is clicked.
import React, { useState } from 'react'; function App() { const [showButton, setShowButton] = useState(false); const handleScroll = () => { const scrollY = window.scrollY; setShowButton(scrollY > 200); // Show button when scrolled down }; const scrollToTop = () => { window.scrollTo({ top: 0, behavior: 'smooth', }); }; window.addEventListener('scroll', handleScroll); return ( <div> <h1>Scroll to Top Button</h1> <div style={{ height: '150vh', border: '1px solid #ccc' }}> Scroll down to see the button. </div> {showButton && ( <button onClick={scrollToTop} style={{ position: 'fixed', bottom: '20px', right: '20px' }}> Scroll to Top </button> )} </div> ); } export default App;
Output
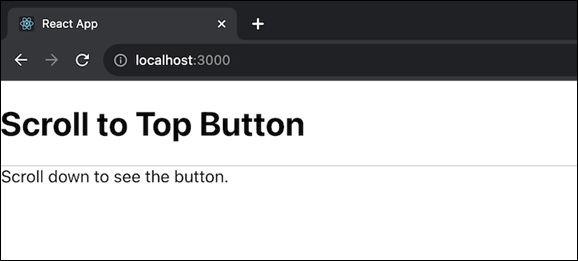
Summary
UIEvent handlers allow us to respond to web user activities. These properties describe interactions, like what was clicked or how far someone scrolled. Web developers can create a smooth and engaging user experience using handlers and these characteristics.
To Continue Learning Please Login