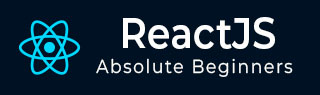
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - useDebugValue Hook
The useDebugValue hook is a React tool that displays custom labels and values for custom hooks in the React DevTools. This is very handy for debugging and providing more information about our own hooks.
Syntax
useDebugValue(value, formatFn);
Parameters
value − This is the value that we want to display. It can be any JavaScript value, like a text, integer, or object, or it can also be a function.
formatFn − This is a function that takes a value as an argument and returns a string representing the label or description of the item. We can also add a custom label to the value.
Return Value
The hook ‘useDebugValue’ returns nothing.
Reference
To display a readable debug value we can call ‘useDebugValue’ at the top level of our custom Hook −
import { useDebugValue, useState } from 'react'; function useCounter() { const [count, setCount] = useState(0); // Display the count and a status message useDebugValue(count, (count) => `Count: ${count}, Status: ${count === 0 ? 'Empty' : 'Not Empty'}`); const increment = () => setCount(count + 1); const decrement = () => setCount(count - 1); return { count, increment, decrement }; }
Examples
We can use this hook in two different ways. First we can use it by adding a label to a custom Hook and secondly we can use it by deferring formatting of a debug value. So we will see both the options one by one.
Example − Adding a label to a custom Hook
To display a readable debug value we will call useDebugValue at the top level of our custom Hook.
import { useDebugValue } from 'react'; function signInStatus() { // ... useDebugValue(isSignIn ? 'SignIn' : 'Signout'); }
In this example we will show a custom label using the useDebugValue hook as per the authentication status of the user.
useAuthStatus.js
import { useDebugValue } from "react"; export function useAuthStatus() { const isAuthenticated = checkAuthentication(); // Use the useDebugValue hook useDebugValue(isAuthenticated ? "Authenticated" : "Not Authenticated"); return isAuthenticated; } function checkAuthentication() { // Checking authentication status of the user return Math.random() < 0.5; // Authentication status will be False // return Math.random() > 0.5; // Authentication status will be True }
App.js
import { useAuthStatus } from "./useAuthStatus.js"; function AuthStatusComponent() { const isAuthenticated = useAuthStatus(); return ( <div> <h1>{isAuthenticated ? "Authenticated" : "Not Authenticated"}</h1> </div> ); } export default function App() { return <AuthStatusComponent />; }
Output
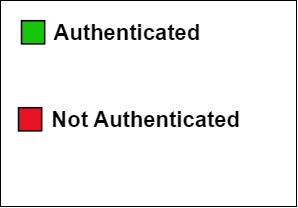
Example − Deferring debug value formatting
As a second parameter to useDebugValue hook, we can also specify a formatting function.
The useDateValue custom hook in the below example combines the useDebugValue hook with the formatDateString formatting method. This removes the need to use toDateString() on the Date variable for each render. This technique makes the code more efficient. The formatDateString method is only called when the component is examined because of the useDebugValue hook.
useDateValue.js
import { useDebugValue } from 'react'; export function useDateValue(date) { // Define a formatting function that formats the Date value const formatDateString = (date) => date.toDateString(); // Use the useDebugValue hook with the formatting function useDebugValue(date, formatDateString); return date; }
App.js
import { useDateValue } from './useDateValue.js'; function DateComponent() { const currentDate = useDateValue(new Date()); return ( <div> <h1>Current Date: {currentDate.toDateString()}</h1> </div> ); } export default function App() { return <DateComponent />; }
Output
Current Date: Wed Oct 11 2023
Example − Counter App
So we can add useDebugValue to display the count in a more informative way when debugging. The useDebugValue hook is useful for providing additional information about the value of a custom hook or component during debugging.
import React, { useState, useDebugValue } from 'react'; function App() { const [count, setCount] = useState(0); const increment = () => { setCount(count + 1); }; const decrement = () => { setCount(count - 1); }; // Display the count value in a more informative way for debugging useDebugValue(`Count: ${count}`); return ( <div> <h1>Counter App</h1> <p>Count: {count}</p> <button onClick={increment}>Increment</button> <button onClick={decrement}>Decrement</button> </div> ); } export default App;
Output
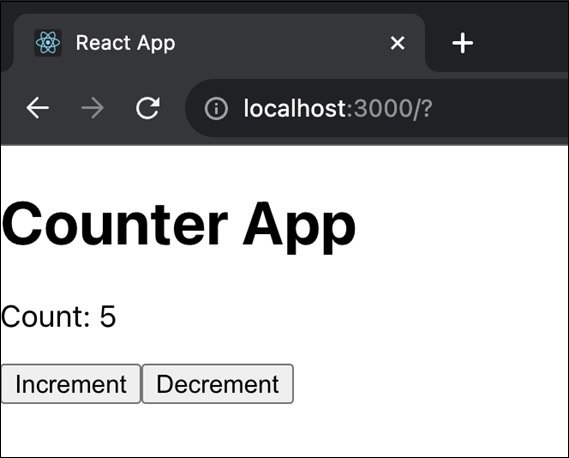
Note
The useDebugValue hook is a useful tool for helping developers understand and debug their own code. However, it is important to use it carefully and to be aware of its limitations. It is a tool in our tools that we should use carefully to avoid problems and make our code simpler to deal with.
To Continue Learning Please Login