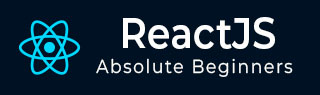
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - KeyboardEvent Handler
Creating dynamic and interactive user interfaces is important in the field of web development. Responding to keyboard events is one process to improve user experience. So we will look at how to handle keyboard events in a React application in this tutorial.
KeyboardEvent Handler Function
To react to keyboard events like key presses, we can use event handler methods in React. These event handler functions take an event object as a parameter, which contains information about the keyboard event. Let's look at the syntax of this event object −
<input onKeyDown={e => console.log('on Key Down')} onKeyUp={e => console.log('on Key Up')} />
Parameters
e − It is a React event object. And we can use this event object with some properties provided below.
KeyboardEvent properties
Sr.No | Properties & Description |
---|---|
1 | altKey Indicates whether or not the "Alt" key is pressed. |
2 | charCode Represents the key's Unicode character code. |
3 | code The actual key on the keyboard (for example, 'KeyA' for the 'A' key). |
4 | ctrlKey Indicates whether or not the "Ctrl" key is pressed. |
5 | key The label on the key (for example, 'A' for the 'A' key). |
6 | keyCode The key code is represented by this value. |
7 | metaKey Indicates the presence of the "Meta" key (on Mac, the 'Command' key). |
8 | location Specifies the location of the key on the keyboard. |
9 | repeat Indicates if the key event is a result of a key being held down. |
10 | shiftKey Indicates whether or not the "Shift" key is pressed. |
11 | which It returns a number for showing a system and implementation dependent numeric code. This is just like the keyCode. |
12 | data Contains additional information about the event. |
13 | view Provides information about the view (window) that generated the event. |
Events of KeyboardEvent handler
So there are some events available in the KeyboardEvent type.
onKeyDown − This event shows that a key has been pressed.
onKeyUp − This event shows that a key has been released.
Examples
Example − Log pressed keys
In this application we are going to create a simple React application in which we will be having a label called User Name and an input field to type the input text. So when the user enters the text in the input field then we will be able to see the common keyboard events in the console.
import React from 'react'; export default function App () { return ( <label> User Name: <input name="userName" onKeyDown={e => console.log('onKeyDown:', e.key, e.code)} onKeyUp={e => console.log('onKeyUp:', e.key, e.code)} /> </label> ); }
Output
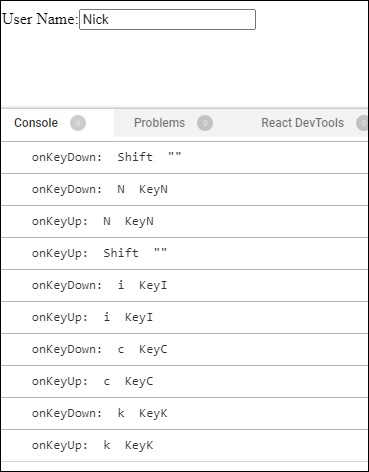
In the above example code, we have created an input field and added event handlers to the input field. So when any key is pressed ('onKeyDown'), we log the key and code related to that key. Just like this when the key is released ('onKeyUp'), we log the key and code.
Example − Key Press Detector
This app will detect and display the key pressed by the user. It will have a component called KeyPressDetector that uses the useState hook to keep track of the currently pressed key. Then we will use the useEffect hook to add and remove a global event listener for the 'keydown' event. Whenever a key is pressed, the handleKeyPress function is invoked, updating the state with the pressed key.
import React, { useState, useEffect } from 'react'; const KeyPressDetector = () => { const [pressedKey, setPressedKey] = useState(null); const handleKeyPress = (event) => { setPressedKey(event.key); }; useEffect(() => { window.addEventListener('keydown', handleKeyPress); return () => { window.removeEventListener('keydown', handleKeyPress); }; }, []); return ( <div> <p>Press any key:</p> <p>Pressed Key: {pressedKey}</p> </div> ); }; export default KeyPressDetector;
Output
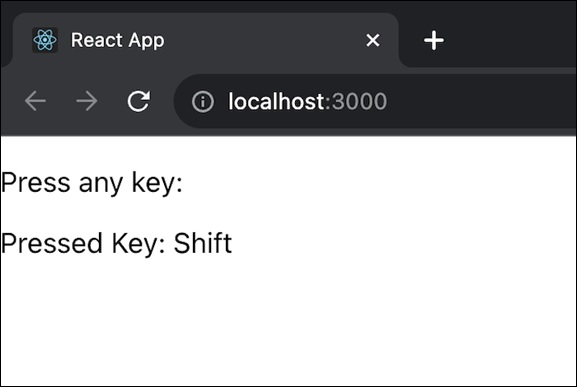
So we can see that the rendered component displays a message showing the user to press any key and shows the currently pressed key.
Example − Background Color Changer
In this app, we will create a BackgroundColorChanger component that changes the background color of a div when the user presses the 'Enter' key. It uses the useState hook to manage the background color state and the useEffect hook to add and remove the 'keydown' event listener. When the 'Enter' key is pressed, the handleKeyPress function generates a random color and updates the background color state.
import React, { useState, useEffect } from 'react'; const BackgroundColorChanger = () => { const [backgroundColor, setBackgroundColor] = useState('#ffffff'); const handleKeyPress = (event) => { if (event.key === 'Enter') { const randomColor = `#${Math.floor(Math.random() * 16777215).toString(16)}`; setBackgroundColor(randomColor); } }; useEffect(() => { window.addEventListener('keydown', handleKeyPress); return () => { window.removeEventListener('keydown', handleKeyPress); }; }, [backgroundColor]); return ( <div style={{ backgroundColor, padding: '20px' }}> <p>Press Enter to change background color</p> </div> ); }; export default BackgroundColorChanger;
Output
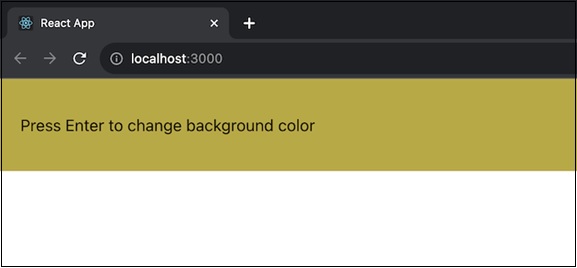
In the above app the rendered component displays a message showing the user to press 'Enter' to observe the color change. With each enter key press, the color will change.
Summary
Handling keyboard events in React can enhance the interactivity and user experience of our web applications. By using these event handler functions and the event object's properties, we can respond to key presses and create responsive interfaces.
To Continue Learning Please Login