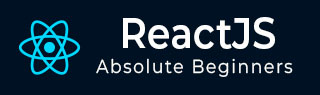
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - isValidElement() Function
As we know React is a JavaScript library for creating UIs. A key concept in React is the "React element." So we will look at how to use React's isValidElement method to figure out that a value is a React element.
What is the isValidElement?
The isValidElement method in React is used to determine whether or not a value is a React element. People may ask why we need to use isValidElement to determine whether or not an item is a React element. There can be times when we need to communicate with libraries or functions that take React components as inputs. In such cases, we have to ensure that the value we provide is a React element. This is when the isValidElement function comes in handy.
Syntax
const isElement = isValidElement(value)
Parameters
value − It is the value we want to check. The value can be of any type.
Return Value
The method returns true if the value is a React element else it returns false.
Using the isValidElement we can verify that something is a React element. The fundamental building blocks of a React application are React elements. They represent our user interface's structure and content.
Examples
Example − Simple app
We have to import isValidElement from the 'react' package in order to use it. Here's the way we can check whether a value is a React element −
import React, { isValidElement, createElement } from 'react'; // These are react elements console.log(isValidElement(<p />)); console.log(isValidElement(createElement('p'))); // These are not React elements console.log(isValidElement(25)); console.log(isValidElement('Hello')); console.log(isValidElement({ age: 42 }));
Output
true true false false false
Example − Render List of Items
Now we will create a small React app that shows the concept of React elements and the use of isValidElement. In this app, we will render a list of items.
import React, { isValidElement, createElement } from 'react'; function ItemList() { const items = [ <li key="item1">Item 1</li>, createElement('li', { key: 'item2' }, 'Item 2'), 'Item 3', // Not a React element ]; return ( <ul> {items.map((item, index) => ( isValidElement(item) ? item : <li key={`item${index}`}>{item}</li> ))} </ul> ); } function App() { return ( <div> <h1>React isValidElement function Demo</h1> <ItemList /> </div> ); } export default App;
Output
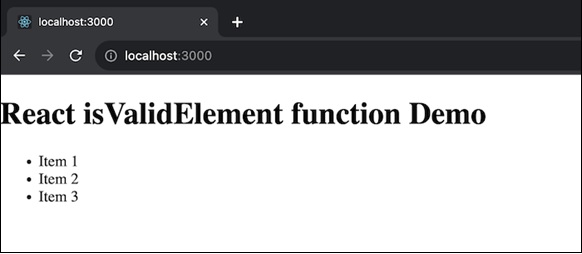
In the App component, the <ItemList /> component is rendered, which contains an unordered list. Inside this list, there are three list items: "Item 1"- A React element created using JSX. "Item 2" - A React element created using createElement. "Item 3" - Not a React element, it is just a plain string.
Example − User Input Form App
Let us create a form where users can input information. We will use isValidElement to check if the entered data is a valid React element. The code for this app is as follows −
import React, { useState, isValidElement } from 'react'; const App = () => { const [inputValue, setInputValue] = useState(''); const handleInputChange = (e) => { setInputValue(e.target.value); }; const handleSubmit = (e) => { e.preventDefault(); const isElement = isValidElement(<p>{inputValue}</p>); if (isElement) { alert(`You entered a valid React element: ${inputValue}`); } else { alert('Please enter a valid input.'); } }; return ( <div> <h1>User Input Form App</h1> <form onSubmit={handleSubmit}> <label> Enter Something: <input type="text" value={inputValue} onChange={handleInputChange} /> </label> <button type="submit">Submit</button> </form> </div> ); }; export default App;
Output
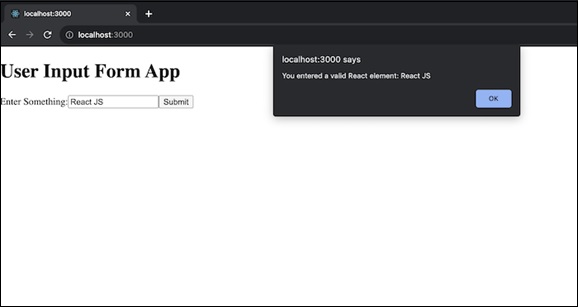
After running the app it displays a heading and an input field with a submit button. The user sees the message "User Input Form App" at the top. When the user types something into the input field. The user clicks the "Submit" button. The app checks if the entered value is a valid React element using isValidElement. If it is valid, an alert message appears, saying "You entered a valid React element: [inputValue]". If it is not valid, an alert message appears, saying "Please enter a valid input."
Summary
The isValidElement is a handy function to check whether or not a value is a React element. While it is rarely required, it is useful when it is necessary to confirm that our values are valid with React-based libraries or methods.
To Continue Learning Please Login