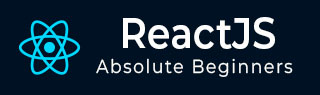
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - UNSAFE_componentWillMount() Method
The componentWillMount() function in React is like a preparation step just before a component appears on a web page. It happens before the component shows up. This function fetches data, but it is important to note that it does not provide any results until the component is displayed for the first time. Because fetching data can take some time, the component does not wait for this phase to complete before displaying itself.
In recent versions of React, it is not recommended using componentWillMount() anymore. They suggest using componentDidMount() instead. If we really want to use componentWillMount(), we can, but we have to call it UNSAFE_componentWillMount(). It is like a warning sign that React developers should avoid it.
Syntax
UNSAFE_componentWillMount() { //the action will come here that we want to execute }
Parameters
This method does not accept any parameters.
Return Value
This method should not return anything.
Examples
Example − Showing alert message
With the help of the UNSAFE_componentWillMount() function, here's an example of a React application that displays a warning before the component is loaded.
import React, { Component } from 'react'; class App extends Component { UNSAFE_componentWillMount() { alert("This message will appear before the component loads."); } render() { return ( <div> <h1>Welcome to My React App</h1> <p>This is a simple application using UNSAFE_componentWillMount().</p> </div> ); } } export default App;
Output
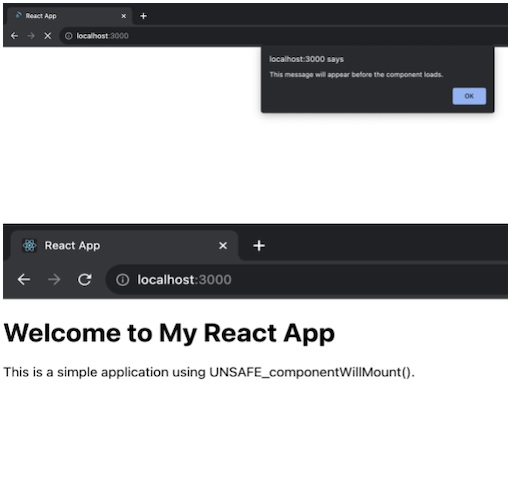
This is a simple example for applying UNSAFE_componentWillMount() to show a message before loading our React component on the web page. Keep in mind that in recent React versions, componentDidMount() is preferred over UNSAFE_componentWillMount() for similar functionality.
Example − Data Fetching App
In this example we will create a component to fetch data from the JSONPlaceholder API when it is about to mount. The fetched data will then be stored in the state of the component and the component renders the data once it is available. So the code for this app is as follows −
import React, { Component } from 'react'; import './App.css' class DataFetchingApp extends Component { constructor() { super(); this.state = { data: null, }; } UNSAFE_componentWillMount() { // fetching data from an API fetch('https://jsonplaceholder.typicode.com/todos/1') .then((response) => response.json()) .then((data) => { this.setState({ data }); }) .catch((error) => console.error('Error fetching data:', error)); } render() { const { data } = this.state; return ( <div className='App'> <h1>Data Fetching App</h1> {data ? ( <div> <h2>Data from API:</h2> <p>Title: {data.title}</p> <p>User ID: {data.userId}</p> <p>Completed: {data.completed ? 'Yes' : 'No'}</p> </div> ) : ( <p>Loading data...</p> )} </div> ); } } export default DataFetchingApp;
App.css
.App { align-items: center; justify-content: center; margin-left: 500px; margin-top: 50px; } h1 { color: red; } p { margin: 8px 0; color: green; }
Output
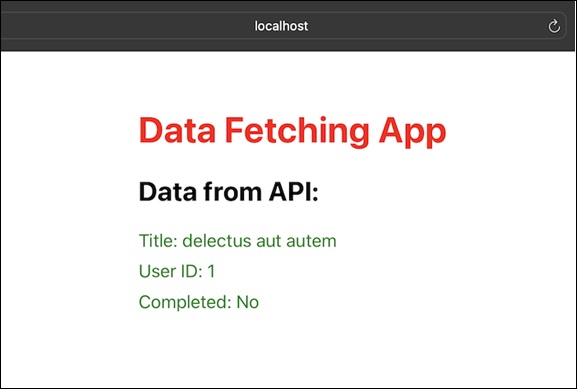
In this React app we have used UNSAFE_componentWillMount() to fetch data from an API before the component mounts. So we have used an API to fetch the data.
Example − Countdown Timer App
Now we have another simple React app that uses UNSAFE_componentWillMount() to set up a countdown timer. In this application we will have a timer which will count down the time of 10 seconds and after 10 seconds it will show an alert message on the screen that the time has been reached to zero. So the code for this app is as follows −
import React, { Component } from 'react'; import './App.css'; class CountdownTimerApp extends Component { constructor() { super(); this.state = { seconds: 10, }; } UNSAFE_componentWillMount() { this.startTimer(); } startTimer() { this.timerInterval = setInterval(() => { this.setState((prevState) => ({ seconds: prevState.seconds - 1, }), () => { if (this.state.seconds === 0) { clearInterval(this.timerInterval); alert('Countdown timer reached zero!'); } }); }, 1000); } render() { return ( <div className='App'> <h1>Countdown Timer App</h1> <p>Seconds remaining: {this.state.seconds}</p> </div> ); } } export default CountdownTimerApp;
Output
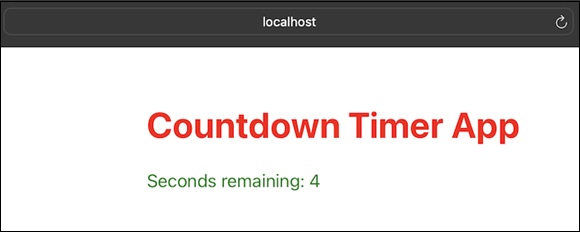
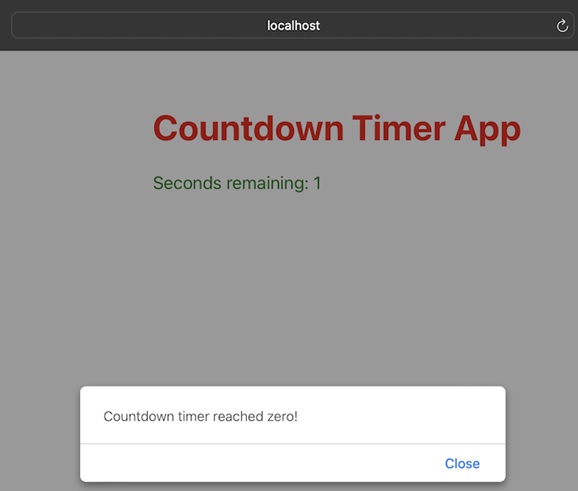
In this example the component begins a countdown timer in the UNSAFE_componentWillMount() lifecycle method. Every second, the timer decrements the seconds state. When the countdown reaches to zero, the interval is cleared and an alert is displayed.
Limitations
If the component uses getDerivedStateFromProps or getSnapshotBeforeUpdate, it does not call this method.
UNSAFE_componentWillMount does not guarantee that the component will be mounted in some current React situations, like Suspense.
It is named "unsafe" because, under certain situations, React can trash an in-progress component tree and reconstruct it, rendering UNSAFE_componentWillMount unreliable.
If we need to conduct activities that rely on component mounting use componentDidMount instead.
UNSAFE_componentWillMount is the only lifecycle method that is executed while the server is rendering.
It's comparable to the constructor for most practical uses, thus it is better to use the constructor for equivalent logic.
Summary
UNSAFE_componentWillMount() is an older React lifecycle function used for pre-component-loading tasks, but in modern React programming, it is recommended to follow current best practices and use componentDidMount() for these tasks.
To Continue Learning Please Login