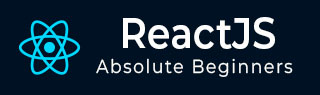
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - use server directive
Directives are somewhat like detailed instructions. They are required for working with React Server Components or developing something that uses them, such as a library.
There are two types of directives present in React: use client and use server.
'use client defines which parts of the code are executed on the client side, which is similar to how our web browser works.
'use server' defines server-side processes that can be accessed by client-side code, which is executed in our web browser. These directives make sure everything operates well in React Server Components.
So in this tutorial, we will go over the term "use server."
What is the ‘use server’?
'use server' is a particular command in React that we use while developing web applications. It helps us when creating functions that run on the server and can be invoked from the client. This can be very helpful for tasks like keeping data or making updates to our website.
Syntax
To make an async function callable by the client, add 'use server' at the top of its body. These are referred to as Server Actions.
async function MyApp(data) { 'use server'; // ... }
Examples
Example 1
Let us say we want to create a button that increments the number of likes on a specific post. When a user clicks the button, we need to update the like count on the server and then show the user the new count.
To do this, we create a function called 'incrementLike' and add the word 'use server' at the top. This notifies React that it is a function that can be called from the client side. So we will create a file named actions.js and use the code below −
actions.js
'use server'; let likeCount = 0; export default async function incrementLike() { likeCount++; return likeCount; }
In this code 'likeCount' starts at 0. When someone hits the like button, the 'incrementLike' function is called, and the 'likeCount' is increased by one. The updated count is then returned.
Now on the client side, when the like button is clicked, it will call the 'incrementLike' function. Here is the code for it −
import incrementLike from './actions'; import { useState, useTransition } from 'react'; export default function App() { const [isPending, startTransition] = useTransition(); const [likeCount, setLikeCount] = useState(0); const onClick = () => { startTransition(async () => { const currentCount = await incrementLike(); setLikeCount(currentCount); }); }; return ( <> <p>Total Likes: {likeCount}</p> <button onClick={onClick} disabled={isPending}>Like</button> </> ); }
Output
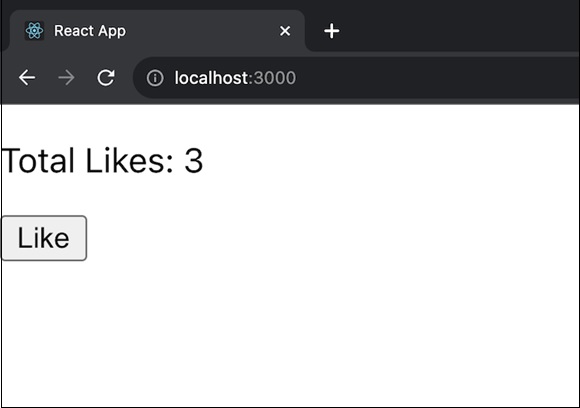
When someone clicks the like button, the function 'incrementLike' is called, and the server's like count is updated. The user is then presented the updated count.
Example 2
Here is another example of an app using the 'use-server' method. In this example the app contains a button for retrieving data from a server. The page will then display the retrieved data or an error message if there is a problem while fetching the data. So let’s see the code below −
App.js
'use server'; import { useState } from 'react'; import './App.css'; export default function App() { const [message, setMessage] = useState(''); const fetchData = async () => { try { // A server request const response = await fetch('https://api.github.com/users/john123/repos/12'); const data = await response.json(); setMessage(`Data from server: ${JSON.stringify(data)}`); } catch (error) { setMessage('Error fetching data from the server.'); } }; return ( <div className='App'> <p>{message}</p> <button onClick={fetchData}>Fetch Data</button> </div> ); }
Output
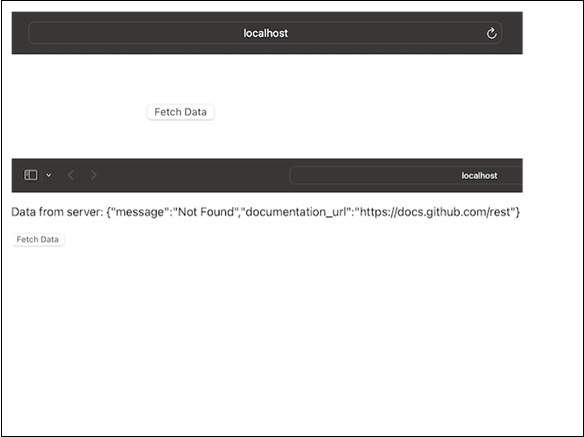
So we can see in the above output image when we click on the Fetch Data button the fetched data will be shown above the button. Remember we can modify the code to meet the individual needs and to replace the placeholder URL with the actual endpoint from which you want to retrieve data.
Example 3
Here is another app which makes use of the 'use-server' method, this time we will handle asynchronous operations. The app will include a button to do an asynchronous operation (with a delay). It will show a loading message while the operation is in progress. And then it will display the fetched data after success, and show an error message if there is an error. So the code for the same is as follows −
asyncApp.js
'use server'; import { useState } from 'react'; export default function AsyncApp() { const [isLoading, setIsLoading] = useState(false); const [data, setData] = useState(null); const [error, setError] = useState(null); const fetchData = async () => { try { setIsLoading(true); // server request delay await new Promise(resolve => setTimeout(resolve, 1500)); // Successful response const response = { message: 'Data fetched successfully!' }; setData(response); } catch (err) { // An error during the request setError('Error fetching data from the server.'); } finally { setIsLoading(false); } }; return ( <div> <h2>Async App</h2> {isLoading && <p>Loading...</p>} {data && <p>{data.message}</p>} {error && <p style={{ color: 'red' }}>{error}</p>} <button onClick={fetchData} disabled={isLoading}> Fetch Data </button> </div> ); }
Output
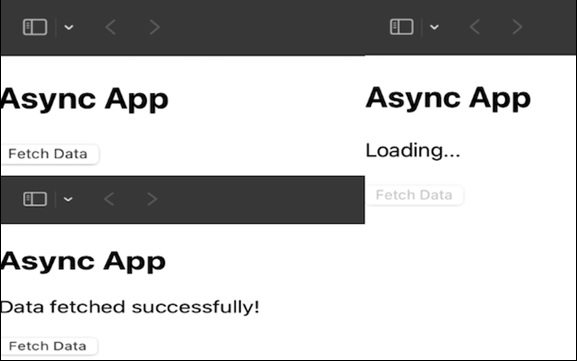
In the above output image we can see the asynchronous application. So when we click the Fetch Data button it will show the Loading message and then it will show the message that Data fetched successfully.
Limitations
If we want to use a 'Server Action' in our client-side code, we must include the term 'use server' at the top of the file where that action is defined.
'use server' can only be used with asynchronous methods, which can require some time to complete.
Always be careful with the information we provide to 'Server Actions'. Assume we are mailing a package; ensure it is secure and trustworthy. Check and secure the information we send.
Summary
The 'use server' is a magical spell that allows us to create functions that can make changes on the server from our website and keeps things in sync. It is useful for things like updating counts and preserving data.
To Continue Learning Please Login