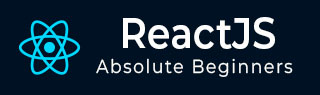
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - CompositionEvent Handler
CompositionEvent is a web development concept that mainly refers to user input and text submission on a webpage. It primarily comes up to the event that occurs when a person user indirectly enters text. Basically, consider the events that occur when someone types on a keyboard, but with a particular focus on languages and input methods that may need more complex handling.
So we can say that the CompositionEvent is a type of event that will be triggered when a user is typing something on a webpage. It is dealing with languages that might have accents or other complex characters. It is just like a behind-the-scenes event that helps web developers manage the text input process effectively.
Syntax
<input onCompositionStart={e => console.log('run on Composition Start')} onCompositionUpdate={e => console.log('run on Composition Update')} onCompositionEnd={e => console.log('run on Composition End')} />
Parameters
The data property in the CompositionEvent interface is a special message that informs us something different depending on when it is used −
The data property in the CompositionEvent interface is a special message that informs us something different depending on when it is used −
e − It is a simple React event object.
compositionStart − It tells us the text that was selected before we started entering something new for a "compositionStart" event. Even if we modify our selection, this message will still inform us what we chose when we started.
compositionStart − It tells us the text as we type it for a "compositionUpdate" event. As when we adjust the text it changes.
compositionEnd − A "compositionend" event shows the text that has been added to the document, or "committed" to the editor. This is what remains after we have finished writing and confirmed the text.
How is it useful?
CompositionEvents are useful for web developers because they offer information on the text input process, which is especially useful when dealing with complicated characters or other input methods. It enables developers to create a more flexible and user-friendly text input experience.
Examples
Example − Special Character App
Let's see a basic example of how we can use CompositionEvent in a React JS application. Let's say we want to manage the input of special characters.
import React, { useState } from "react"; function App() { const [textInput, setTextInput] = useState(""); const handleCompositionStart = (e) => { console.log("Composition Start"); }; const handleCompositionUpdate = (e) => { // Check if it is a CompositionEvent if (e instanceof CompositionEvent) { // Update the state setTextInput(e.data); } }; const handleCompositionEnd = (e) => { console.log("Composition End"); }; const handleInput = (e) => { // This event handles regular text input setTextInput(e.target.value); }; return ( <div> <input type="text" onCompositionStart={handleCompositionStart} onCompositionUpdate={handleCompositionUpdate} onCompositionEnd={handleCompositionEnd} onInput={handleInput} /> <p>Text Input: {textInput}</p> </div> ); } export default App;
Output
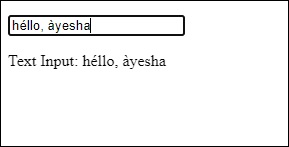
In the above code, the handleCompositionStart method is called when the user begins creating text, the handleCompositionEnd function is invoked when the user stops writing text and the handleCompositionUpdate method will call when the user updates the text.
These functions are linked to the onCompositionStart, onCompositionUpdate and onCompositionEnd events, respectively.
Example − Emoji Picker App
This app will be an input field with an emoji picker that uses composition events to allow users to input emojis easily. This code defines a EmojiPicker component that fetches emoji data. It uses the useEffect hook to fetch emoji data from an API (e.g., GitHub emoji API) or loads a static list. The code is given below for this app −
App.js
import React, { useState } from 'react'; import EmojiPicker from './EmojiPicker'; function App() { const [text, setText] = useState(''); const [showEmojiPicker, setShowEmojiPicker] = useState(false); const handleChange = (event) => { setText(event.target.value); }; const handleEmojiPick = (emoji) => { setText(text + emoji); setShowEmojiPicker(false); }; const handleCompositionStart = () => { // Hide emoji picker if composition starts setShowEmojiPicker(false); }; const toggleEmojiPicker = () => { setShowEmojiPicker(!showEmojiPicker); }; return ( <div> <input value={text} onChange={handleChange} onCompositionStart={handleCompositionStart} /> <button onClick={toggleEmojiPicker}>Toggle Emoji Picker</button> {showEmojiPicker && <EmojiPicker onEmojiPick={handleEmojiPick} />} </div> ); } export default App;
EmojiPicker.js
import React, { useState, useEffect } from 'react'; const EmojiPicker = ({ onEmojiPick }) => { const [emojiList, setEmojiList] = useState([]); useEffect(() => { // Fetch emoji data from an API fetch('https://api.github.com/emojis') .then((response) => response.json()) .then((data) => setEmojiList(Object.keys(data))); }, []); const handleEmojiClick = (emoji) => { onEmojiPick(emoji); }; return ( <div className="emoji-picker"> {emojiList.map((emoji) => ( <button key={emoji} onClick={() => handleEmojiClick(emoji)}> {emoji} </button> ))} </div> ); }; export default EmojiPicker;
Output
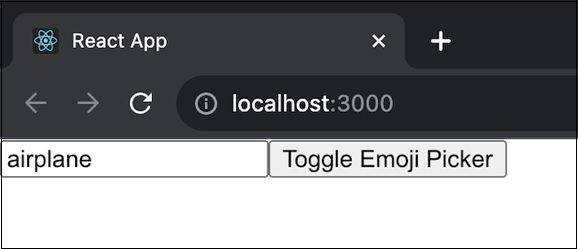
The app maps over the emojiList and renders each emoji as a button. Clicking on an emoji calls the handleEmojiClick function, which passes the selected emoji back to the parent component via the onEmojiPick prop.
Example − Counting Words App
This app demonstrates a simple way to use composition events to count words in real-time. In this app users can type in a text box. The onCompositionUpdate event is triggered as users compose text. The app counts the number of words in the composed text and displays the count.
import React, { useState } from 'react'; function App() { const [currentText, setCurrentText] = useState(''); const [wordCount, setWordCount] = useState(0); const handleChange = (event) => { setCurrentText(event.target.value); }; const handleCompositionStart = () => { console.log('Composition Start'); }; const handleCompositionUpdate = (event) => { if (event instanceof CompositionEvent) { setCurrentText(event.target.value); } }; const handleCompositionEnd = () => { const words = currentText.split(/\s+/); setWordCount(words.filter((word) => word !== '').length); console.log('Composition End'); }; return ( <div> <h2>Word Counter</h2> <textarea value={currentText} onChange={handleChange} onCompositionStart={handleCompositionStart} onCompositionUpdate={handleCompositionUpdate} onCompositionEnd={handleCompositionEnd} /> <p>Words: {wordCount}</p> </div> ); } export default App;
Output
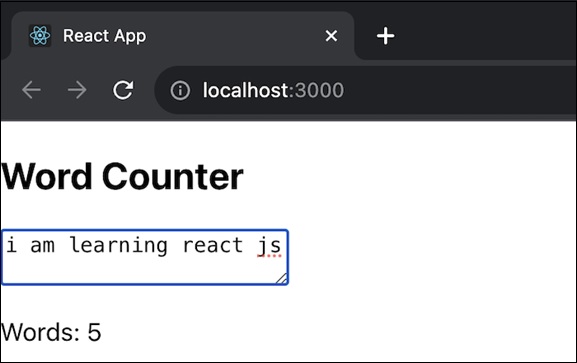
This is a basic example, but it showcases the potential of composition events for creating interactive and responsive applications.
Summary
The CompositionEvent is an important web development concept related to user text input on a webpage, particularly when dealing with languages and input methods requiring complex handling, such as those with accents or special characters. It is triggered during the typing process and helps web developers manage text input effectively.
To Continue Learning Please Login