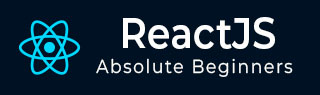
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - UNSAFE_componentWillReceiveProps() Method
UNSAFE_componentWillReceiveProps is a React function. It is used to show that a component is about to get new props or context. Remember that if the component uses getDerivedStateFromProps or getSnapshotBeforeUpdate, UNSAFE_componentWillReceiveProps will not be called. Furthermore, it does not guarantee that the component will receive the new props, which is especially important with recent React features like Suspense.
Syntax
UNSAFE_componentWillReceiveProps(nextProps, nextContext)
Parameters
nextProps − These are the new props received by the component from its parent component. We can see what has changed by comparing nextProps to this.props.
nextContext − The component will need to get a new context from the closest provider. To find out what is different, compare nextContext to this.context. This is only available if static contextType or static contextTypes is given.
Return Value
The method does not return anything. It is just a function that React calls when props or context are about to change.
Examples
Example − Message App
Here is an example of how to make use of UNSAFE_componentWillReceiveProps in a small React app. In this example, we will create a component that shows a message and updates it as new props receive.
import React, { Component } from 'react'; class MessageComponent extends Component { constructor(props) { super(props); this.state = { message: props.initialMessage, }; } // This is the UNSAFE_componentWillReceiveProps function UNSAFE_componentWillReceiveProps(nextProps) { if (nextProps.newMessage !== this.props.newMessage) { // Check if the new message is different this.setState({ message: nextProps.newMessage }); } } render() { return <div>{this.state.message}</div>; } } class App extends Component { constructor() { super(); this.state = { message: 'Hello, Tutorialspoint!', newMessage: 'Welcome to the React App!', }; } updateMessage = () => { this.setState({ newMessage: 'Message is Updated!' }); }; render() { return ( <div> <MessageComponent initialMessage={this.state.message} newMessage={this.state.newMessage} /> <button onClick={this.updateMessage}>Update Message</button> </div> ); } } export default App;
Output
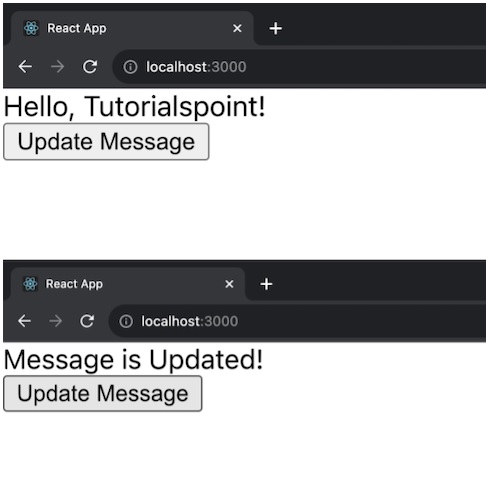
App and MessageComponent are the two components in the above example. When new props are received, MessageComponent updates its message with UNSAFE_componentWillReceiveProps. The App component has a button that changes the message.
Example − Counter App
Let us create another simple React app and apply CSS to the apps for styling. This app will be a basic ToDo List which shows the usage of UNSAFE_componentWillReceiveProps() function. So below is the code mentioned for this app −
TodoList.js
import React, { Component } from 'react'; import './TodoList.css'; class TodoList extends Component { constructor(props) { super(props); this.state = { todos: [], newTodo: '', }; } UNSAFE_componentWillReceiveProps(nextProps) { // Update todos when receiving new props if (nextProps.todos !== this.props.todos) { this.setState({ todos: nextProps.todos }); } } handleInputChange = (event) => { this.setState({ newTodo: event.target.value }); }; handleAddTodo = () => { if (this.state.newTodo) { const updatedTodos = [...this.state.todos, this.state.newTodo]; this.setState({ todos: updatedTodos, newTodo: '' }); } }; render() { return ( <div className="todo-container App"> <h2>ToDo List</h2> <ul> {this.state.todos.map((todo, index) => ( <li key={index}>{todo}</li> ))} </ul> <div className="input-container"> <input type="text" value={this.state.newTodo} onChange={this.handleInputChange} placeholder="Add a new todo" /> <button onClick={this.handleAddTodo}>Add</button> </div> </div> ); } } export default TodoList;
TodoList.css
.todo-container { max-width: 400px; margin: 20px auto; padding: 20px; border: 1px solid #ccc; border-radius: 8px; box-shadow: 0 0 10px rgba(0, 0, 0, 0.1); } ul { list-style: none; padding: 0; } li { margin-bottom: 8px; } .input-container { margin-top: 16px; display: flex; } input { flex-grow: 1; padding: 8px; margin-right: 8px; } button { padding: 8px 16px; background-color: #4caf50; color: #fff; border: none; border-radius: 4px; cursor: pointer; } button:hover { background-color: #45a049; }
Output
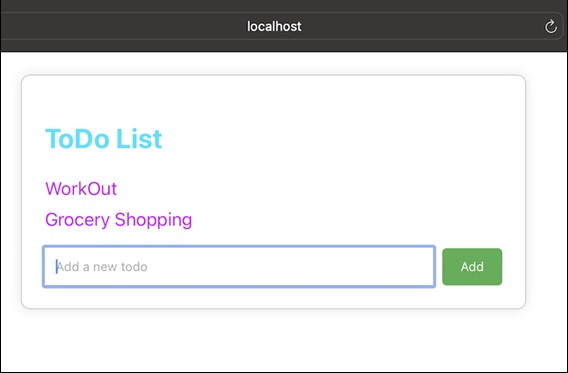
Example − Profile App
Below is an example of another React app using UNSAFE_componentWillReceiveProps() along with CSS for styling. This app is a simple "User Profile" component that displays the user's name and age.
UserProfile.js
import React, { Component } from 'react'; import './UserProfile.css'; class UserProfile extends Component { constructor(props) { super(props); this.state = { name: 'John', age: 20, }; } UNSAFE_componentWillReceiveProps(nextProps) { // Update state when receiving new props if (nextProps.user !== this.props.user) { this.setState({ name: nextProps.user.name, age: nextProps.user.age, }); } } render() { return ( <div className="user-profile-container"> <p className="user-name">Name: {this.state.name}</p> <p className="user-age">Age: {this.state.age}</p> </div> ); } } export default UserProfile;
UserProfile.css
.user-profile-container { max-width: 300px; margin: 20px; padding: 16px; border: 1px solid #ddd; border-radius: 4px; box-shadow: 0 0 8px rgba(0, 0, 0, 0.1); } .user-name, .user-age { font-size: 18px; color: #333; margin: 8px 0; }
Output
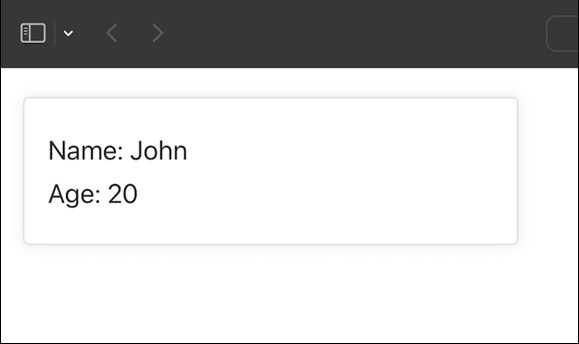
Please keep in mind that in modern React, we normally use other lifecycle methods or hooks to give functionality similar to UNSAFE_componentWillReceiveProps.
Summary
UNSAFE_componentWillReceiveProps is an old React lifecycle function that is called when a component is going to receive new props from its parent. It is used to respond to prop changes by comparing the new props to the existing ones. When dealing with props changes in new React code, it is best to use the alternatives for more secure and consistent behavior.
To Continue Learning Please Login