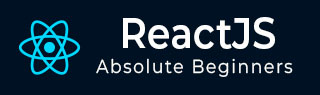
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - cache()
In React 18, there is a new feature called "cache function." This function remembers what occurs when specific inputs are passed to it. So, if we use the same function with the same arguments when working on something in React, it will not repeat the job. Instead, it will quickly return the remembered result, saving users time and resources. It is similar to our computer remembering stuff in order to perform more efficiently.
Syntax
const cachedFunc = cache(func);
Parameters
func − func is a function that can do a task with some information, and it can work with any kind of information we give it.
Return Value
When we use this cached version of the function with specific inputs, it checks if it already has a stored result for those inputs. If it does, it gives us that result without running the function again. But if it doesn't have a stored result, it runs the original function with those inputs, saves the result in its memory, and then gives us that result. So, the original function only runs when there's no saved result in the cache.
Examples
Example
Here is an example of how we can use the cache function in React 18. In this example, we will create a simple cache for calculating the square of a number.
import { cache } from 'react'; // Calculate the square of a number and cache the result export const square = cache(async (num) => { console.log(`Calculating the square of ${num}`); return num * num; }); const result1 = await square(5); // Calculate and cache the result const result2 = await square(5); // Return the memoized (cached) result console.log(result1); // Calculating the square of 5, 25 console.log(result2); // 25 (No recalculation, just returns the cached result) // Use the square function const result3 = await square(3); // This will calculate and cache the result. console.log(result3); // Output: Square of 3, 9
In this example, we created a square function that calculates and caches the square of a number. When we call it with the same input (for example, 5) during the same render pass, it returns the cached result, reducing the need for recalculation. When we call it again with a different input (for example, 3), it calculates and caches a new result.
Example − To avoid duplicate work, use cache
Here is another example that shows the use of the cache function in React −
The cache function is being used to save the results of a function named calculateProductStats. The cache-created getProductStats method is used to collect statistics for various items.
The ProductDetails component uses GetProductStats to retrieve data for a single product, while the SalesDetails component uses it to get data on several items in a report. This shows how we can apply memoization to various methods and components depending on our requirements.
import { cache } from 'react'; import calculateProductStats from 'lib/product'; const getProductStats = cache(calculateProductStats); function ProductDetails({product}) { const stats = getProductStats(product); // write code here } function SalesDetails({products}) { for (let product in products) { const stats = getProductStats(product); // write code here } // write code here }
If the same product object is presented in both ProductDetails and SalesReport, the two components can share work and only call calculateProductStats for that product once.
Assume ProductDetails is the first to be rendered. It will call getProductStats and see if a cached result exists. There will be a cache miss because this is the first time getProductStats is called with that product. getProductStats will then use that product to call calculateProductStats and save the result to cache.
When SalesReport produces its product list and comes to the same product object, it will call getProductStats and read the result from cache.
Example − Preload Data
Suppose we are creating a web page and need to get some data from a database to display on it. This data extraction can take some time. We can start fetching data in the background even before we need it using the new cache function.
Here's an example −
Let's say we have a getUser function that collects a user's data from a database. Even before we need the user's data, we start fetching it in the Page component by executing getUser(id). We can do other things in our component while that data retrieval is happening.
The user's info is then obtained by calling getUser again in the Profile component. If the Page component's initial call has already fetched and cached the user's data, the Profile component can use that cached data instead of performing another slow database access. This speeds up your page because it minimizes the wait time for fetching data.
import { cache } from 'react'; import fetchProductDetails from 'api/products'; const getProductDetails = cache(async (productId) => { return await fetchProductDetails(productId); }); function ProductInfo({ productId }) { // Fetch product details getProductDetails(productId); return ( <div> <h2>Product Information</h2> {getProductDetails(productId).description} </div> ); }
In this example, we have a ProductInfo component that starts receiving product details in the background with the cache function. This way, when we require the data, it is already in the cache, reducing the time it takes to display the product information on the page.
Limitations
When dealing with server requests in React, the memory storage for cached results from functions (the cache) for all memoized functions is cleaned each time a new server request is made.
Every time we use the cache function, a new, distinct function is created. So, if we call cache many times with the same original function, we receive separate memoized functions, and these memoized functions do not share the same memory storage for saved results.
Errors are also remembered by the memoized functions provided by cache. If the original function returns an error for any of the inputs, that error is preserved in memory. As a result, using the memoized function with the same inputs will result in the same error without re-running the function.
Importantly, the cache method is intended to be used only in a section of React known as "Server Components." It is not intended for use in other portions of our application.
Summary
The "cache function" is a new feature added in React 18 that allows developers to easily store and retrieve results of functions depending on stated inputs. This saves duplicate calculations and improves efficiency by returning cached results fast when the same inputs are encountered.
To Continue Learning Please Login