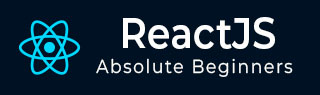
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - TransitionEvent Handler
The TransitionEvent handler method is similar to a special detective in a computer program that monitors changes in how things look (like the colors or sizes) on a web page.
Assume we have a button on a website that changes color easily as we click it. The TransitionEvent handler method identifies when a color change happens and can be used to perform an action when the change is complete.
In simple terms, the TransitionEvent handler method helps our website in reacting when visual effects.
Syntax
<div onTransitionEnd={e => console.log('onTransitionEnd')} />
Parameters
e − This is a React event object we can use with TransitionEvent properties.
Types of TransitionEvent
There are four major sorts of transition events to be aware of −
Sr.No | Types & Description |
---|---|
1 | transitioncancel This event happens when an animation or transition is stopped or canceled before it finishes. |
2 | transitionend This event occurs when an animation or transition has successfully completed. |
3 | transitionrun This event takes place when an animation or transition is prepared to start, but it may not have actually started moving yet. |
4 | transitionstart This event happens when an animation or transition begins to make changes. |
Properties of TransitionEvent
There are some transition event properties to be aware of −
Sr.No | Properties & Description |
---|---|
1 | elapsedTime This property tells us how much time has passed since the start of the transition or animation. |
2 | propertyName This property indicates the name of the CSS property that is changing during the transition or animation. |
3 | pseudoElement It helps identify if the animation is on an element or something added with CSS. |
Examples
Example − CSS Transition App
To show the concept of the TransitionEvent handler method, we can create a component that changes a CSS transition and uses the transitionend event to identify when the transition is complete. So we will create a button that changes its background color with a transition and displays a message when the transition ends. The code is as follows −
import React, { useState } from "react"; function App() { const [isTransition, setTransition] = useState(false); const [message, setMessage] = useState(""); const handleButtonClick = () => { setTransition(true); setMessage("Transition..."); // delay and then reset the transition state setTimeout(() => { setTransition(false); setMessage("Transition Complete"); }, 1000); }; return ( <div className="App"> <button className={`transition-button ${ isTransition ? "Transition" : "" }`} onClick={handleButtonClick} > Click me to start the transition </button> <div className="message">{message}</div> </div> ); } export default App;
Output
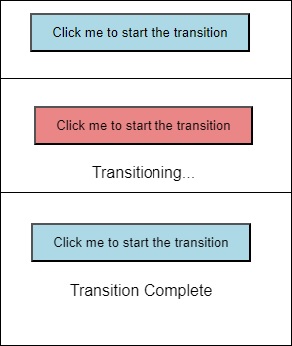
This example uses a basic transition in response to a button click, as well as the TransitionEvent concept. When we click the button, the transition starts and the message will inform us when it is finished.
Example − Simple Animation using TransitionEvent
This app shows the opacity of a div element on click and logs the transition duration. When we click the "Animate" button, the div's opacity will simply change from 1 (fully opaque) to 0 (completely transparent) over 1 second. The onTransitionEnd handler logs the time it took for the transition to complete.
import React, { useState } from "react"; function App() { const [isAnimating, setIsAnimating] = useState(false); const handleClick = () => { setIsAnimating(true); }; const handleTransitionEnd = (e) => { console.log(`Transition completed: ${e.elapsedTime} seconds`); setIsAnimating(false); }; return ( <div> <button onClick={handleClick}>Animate</button> <div style={{ opacity: isAnimating ? 0 : 1 }} transition="opacity 1s ease" onTransitionEnd={handleTransitionEnd} > Click me to animate! </div> </div> ); } export default App;
Output
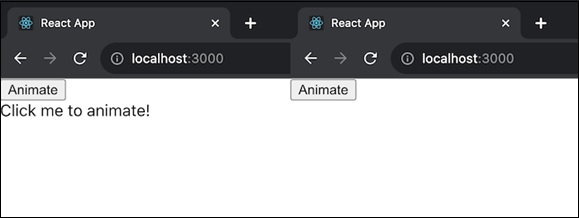
Example − Accordion with Expand and Collapse Animation
This app displays an accordion with content panels that expand and collapse on click. Clicking on a panel title expands or collapses its content smoothly over 0.5 seconds. The onTransitionEnd handler function logs the title of the panel that finished transitioning.
import React, { useState } from "react"; const panels = [ { title: "Panel 1", content: "This is the content of panel 1." }, { title: "Panel 2", content: "This is the content of panel 2." }, { title: "Panel 3", content: "This is the content of panel 3." }, ]; function App() { const [activePanelIndex, setActivePanelIndex] = useState(null); const handleClick = (index) => { setActivePanelIndex(index === activePanelIndex ? null : index); }; const handleTransitionEnd = (e) => { console.log( `Panel transitioned: ${e.target.parentNode.querySelector(".panel-title").textContent}` ); }; return ( <div> {panels.map((panel, index) => ( <div key={index} className="panel"> <h3 className="panel-title" onClick={() => handleClick(index)} > {panel.title} </h3> <div className="panel-content" style={{ maxHeight: activePanelIndex === index ? "500px" : "0" }} transition="max-height 0.5s ease" onTransitionEnd={handleTransitionEnd} > {panel.content} </div> </div> ))} </div> ); } export default App;
Output
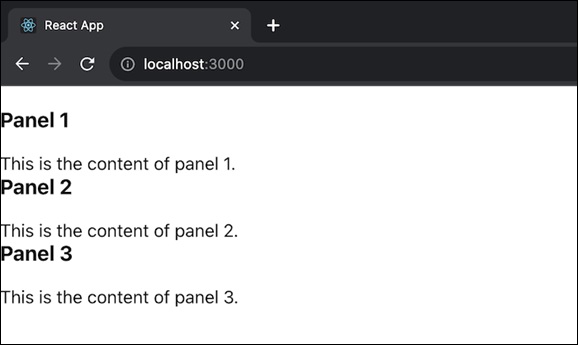
Summary
A TransitionEvent handler function is a web development tool for identifying and reacting to transitions or animations on a webpage. It is like an observer that can react to certain CSS transition events.
These methods allow developers to add custom actions or responses to these transition events, allowing developers to create interactive and dynamic web applications.
To Continue Learning Please Login