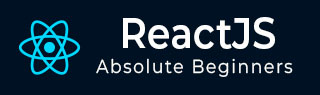
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - Event Object
In React, when we construct event handlers for things like button clicks, we get a special object known as a React event object (or synthetic event). This object acts as a link between our code and the browser events. It reduces gaps between web browsers and provides some important information.
How to use it?
Let's break this down using a simple example −
Let us say we have a button in our React app that we want to perform something when it is clicked. So we will define an onClick handler function as follows −
Sample
<button onClick={e => { console.log(e); // The event object }} />
In this code, ‘e’ is our React event object and it provides information about the button click.
Discover what event objects can do !
Let’s have a glance over some of the standard Event attributes implemented by React event objects −
Sr.No | Properties & Description |
---|---|
1 | e.bubbles This tells us if the event "bubbles" through the elements on the web page. For example, if we have a button inside a div, the click event may bubble up from the button to the div. It is a boolean (true or false). |
2 | e.cancelable It shows that we can stop the default behavior of the event. For example, we can prevent a form from submitting when a button is clicked. It is also a boolean type. |
3 | e.currentTarget It points to the element where our event handler is attached in our React component. It is a reference to the button in this case. |
4 | e.defaultPrevented Tells us if someone has used preventDefault() to stop the default action of the event. A boolean. |
5 | e.eventPhase It's a number that tells us which phase the event is in (like capturing, target, or bubbling). |
6 | e.isTrusted Shows whether the event was initiated by a user. If true, it was a genuine user action; if false, it might have been triggered by code. |
7 | e.target This points to the element where the event actually occurred. It could be the button. |
8 | e.timeStamp It gives you the time when the event happened. |
9 | e.nativeEvent We can access the underlying browser event using it. |
Example − Using event Objects
Let’s create a simple application to show the working of event object properties. So in this app we are creating a component called EventHandlingExample.js and then we will use this component in the App.js component to see its working.
EventHandlingExample.js
import React from 'react'; class EventHandlingExample extends React.Component { handleClick = (e) => { // Logging event properties console.log('Bubbles:', e.bubbles); console.log('Cancelable:', e.cancelable); console.log('Current Target:', e.currentTarget); console.log('Default Prevented:', e.defaultPrevented); console.log('Event Phase:', e.eventPhase); console.log('Is Trusted:', e.isTrusted); console.log('Target:', e.target); console.log('Time Stamp:', e.timeStamp); // Prevent the default behavior e.preventDefault(); } render() { return ( <div> <button onClick={this.handleClick}>Click Me</button> </div> ); } } export default EventHandlingExample;
App.js
import React from 'react'; import './App.css'; import EventHandlingExample from './EventHandlingExample'; function App() { return ( <div className="App"> <h1>React Event Object Example</h1> <EventHandlingExample /> </div> ); } export default App;
Output
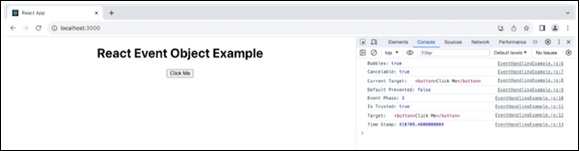
In the above example code, When we click the "Click Me" button, the event properties will be logged in the console. The e.preventDefault() line shows how to prevent the button from performing its default action.
Standard event Methods
Let’s have a glance over some of the standard Event methods which are implemented by React event objects −
Sr.No | Methods & Description |
---|---|
1 | e.preventDefault() This method can prevent the default behavior of the event. For example, you can use it to stop a form from submitting. |
2 | e.stopPropagation() This stops the event from traveling further up the React component tree. It can be helpful to prevent other event handlers from running. |
3 | e.isDefaultPrevented() To see if preventDefault() was called. Returns boolean value. |
4 | e.isPropagationStopped() To see if stopPropagation() was called. Returns boolean value. |
Example − Using event Methods
Let's create a basic React app that uses event object methods to prevent default behavior and stop event propagation when a button is clicked. Make sure the project includes React and ReactDOM. Make a new React component called EventMethodsExample that shows the use of event object methods −
EventMethodsExample.js
import React from 'react'; class EventMethodsExample extends React.Component { handleClick = (e) => { // Prevent the default behavior of the button e.preventDefault(); // Stop the event from propagating e.stopPropagation(); } render() { return ( <div> <button onClick={this.handleClick}>Click Me</button> <p>Clicking the button will prevent the default behavior and stop event propagation.</p> </div> ); } } export default EventMethodsExample;
App.js
import React from 'react'; import './App.css'; import EventMethodsExample from './EventMethodsExample'; function App() { return ( <div className="App"> <h1>React Event Object Methods Example</h1> <EventMethodsExample /> </div> ); } export default App;
Output
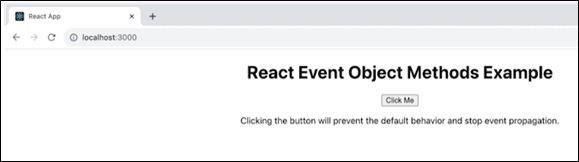
When we click the "Click Me" button after running our React application, it prevents the default behavior like form submission and prevents the event from propagating further up the React component tree.
This example shows how to use the event object's preventDefault() and stopPropagation() methods to manage event behavior.
Example − Using both event object and methods
Let us create one more example app to show the use of React event objects. In this example, we will create a simple React application that responds to a keypress event.
KeyPressExample.js
import React from 'react'; class KeyPressExample extends React.Component { handleKeyPress = (e) => { // Log the key that was pressed console.log('Pressed Key:', e.key); } render() { return ( <div> <h2>React Key Press Event Example</h2> <input type="text" placeholder="Press a key" onKeyPress={this.handleKeyPress} /> </div> ); } } export default KeyPressExample;
App.js
import React from 'react'; import './App.css'; import EventHandlingExample from './EventHandlingExample'; import EventMethodsExample from './EventMethodsExample'; import KeyPressExample from './KeyPressExample'; // Import the new component function App() { return ( <div> <h1>React Event Examples</h1> <EventHandlingExample /> <EventMethodsExample /> <KeyPressExample /> {/* Include the new component */} </div> ); } export default App;
Output
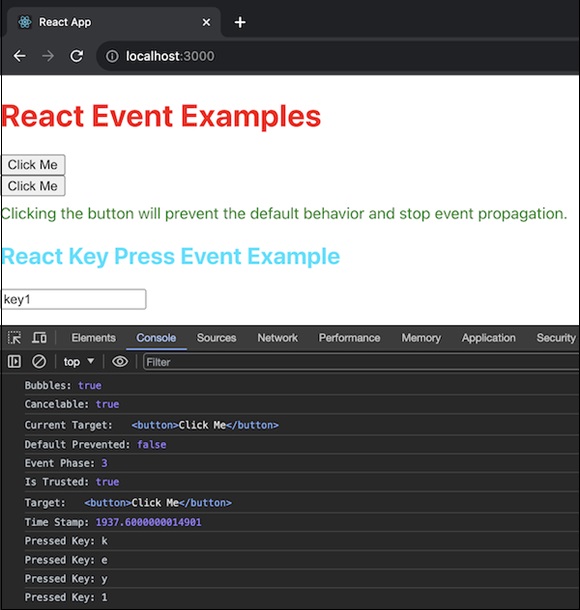
Conclusion
In simple terms, React event objects help us in handling user interactions in a reliable and effective manner, making sure our app functions properly over several web browsers and settings.
To Continue Learning Please Login