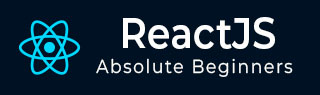
- ReactJS Tutorial
- ReactJS - Home
- ReactJS - Introduction
- ReactJS - Installation
- ReactJS - Features
- ReactJS - Advantages & Disadvantages
- ReactJS - Architecture
- ReactJS - Creating a React Application
- ReactJS - JSX
- ReactJS - Components
- ReactJS - Nested Components
- ReactJS - Using Newly Created Components
- ReactJS - Component Collection
- ReactJS - Styling
- ReactJS - Properties (props)
- ReactJS - Creating Components using Properties
- ReactJS - props Validation
- ReactJS - Constructor
- ReactJS - Component Life Cycle
- ReactJS - Event management
- ReactJS - Creating an Event−Aware Component
- ReactJS - Introduce Events in Expense Manager APP
- ReactJS - State Management
- ReactJS - State Management API
- ReactJS - Stateless Component
- ReactJS - State Management Using React Hooks
- ReactJS - Component Life Cycle Using React Hooks
- ReactJS - Layout Component
- ReactJS - Pagination
- ReactJS - Material UI
- ReactJS - Http client programming
- ReactJS - Form Programming
- ReactJS - Controlled Component
- ReactJS - Uncontrolled Component
- ReactJS - Formik
- ReactJS - Conditional Rendering
- ReactJS - Lists
- ReactJS - Keys
- ReactJS - Routing
- ReactJS - Redux
- ReactJS - Animation
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - Table
- ReactJS - Managing State Using Flux
- ReactJS - Testing
- ReactJS - CLI Commands
- ReactJS - Building and Deployment
- ReactJS - Example
- Hooks
- ReactJS - Introduction to Hooks
- ReactJS - Using useState
- ReactJS - Using useEffect
- ReactJS - Using useContext
- ReactJS - Using useRef
- ReactJS - Using useReducer
- ReactJS - Using useCallback
- ReactJS - Using useMemo
- ReactJS - Custom Hooks
- ReactJS Advanced
- ReactJS - Accessibility
- ReactJS - Code Splitting
- ReactJS - Context
- ReactJS - Error Boundaries
- ReactJS - Forwarding Refs
- ReactJS - Fragments
- ReactJS - Higher Order Components
- ReactJS - Integrating With Other Libraries
- ReactJS - Optimizing Performance
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - React Without ES6 ECMAScript
- ReactJS - React Without JSX
- ReactJS - Reconciliation
- ReactJS - Refs and the DOM
- ReactJS - Render Props
- ReactJS - Static Type Checking
- ReactJS - Strict Mode
- ReactJS - Web Components
- Additional Concepts
- ReactJS - Date Picker
- ReactJS - Helmet
- ReactJS - Inline Style
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - Carousel
- ReactJS - Icons
- ReactJS - Form Components
- ReactJS - Reference API
- ReactJS Useful Resources
- ReactJS - Quick Guide
- ReactJS - Useful Resources
- ReactJS - Discussion
ReactJS - createRoot() Method
The createRoot is an essential part of React, which is an important web development framework. It allows us to quickly integrate our React code into a web page. It is like the core of our React project, where all of our web features are kept. We can also change how it works to improve our web app. So we will see the importance of createRoot.
So createRoot allows us to set up a special place in a web page where we can show our React content.
When we use createRoot, it gives us a new container element where our React components can be mounted. This is useful in situations where we want to have independent sections of our app that don't interfere with each other.
Syntax
Here is an example of how we can put it to use −
Use this to make a special spot on our web page for our React code.
const root = createRoot(domNode, optionalObject)
Use this to actually display what we have created with React.
root.render(reactNode)
Use this to actually display what we have created with React.
root.render(reactNode)
We can use this if we want to take our React code away from the web page.
root.unmount()
Parameters
domNode − This is a part of a web page, like a box, where React will put our code. We can use it to do things like showing what we have made with React.
optional options − If we want, we can use this to customize how our React code works in that box..
reactNode − It is like the thing we want to show using React.
Return Value
The object returned by createRoot has two methods: render and unmount. The root.render and root.unmount returns undefined.
Examples
So we can use createRoot in three different ways −
By rendering an app fully built with React − If our whole web application is made using React, we can use "createRoot" to show the entire thing on the web.
Example 1
App.js
import { useState } from 'react'; function CountFunc() { const [counter, setCounter] = useState(0); return ( <button onClick={() => setCounter(counter + 1)}> This button is clicked {counter} times </button> ); } export default function App() { return ( <> <h1>Hello, Tutorialspoint!</h1> <CountFunc /> </> ); }
index.js
import { createRoot } from 'react-dom/client'; import App from './App.js'; import './styles.css'; const root = createRoot(document.getElementById('root')); root.render(<App />);
Output
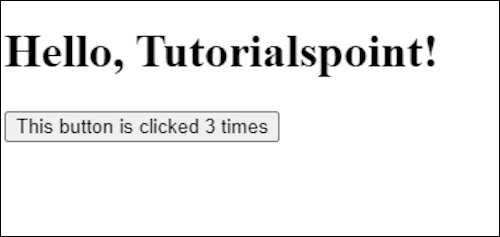
Rendering a page created partially with React− Sometimes, we might only want to use React for a part of our web page. In that case, we can use "createRoot" to display just that part.
Example 2
App.js
import React, { useState } from "react"; import "./App.css"; function App() { const [todos, setTodos] = useState([ { id: 1, text: "Buy cloths" }, { id: 2, text: "Finish work" }, { id: 3, text: "Go for a run" } ]); return ( <div className="App"> <h1>ToDo List</h1> <ul> {todos.map((todo) => ( <li key={todo.id}>{todo.text}</li> ))} </ul> </div> ); } export default App;
index.js
import React from "react"; import ReactDOM from "react-dom"; import App from "./App"; const rootDomNode = document.getElementById("root"); const root = ReactDOM.createRoot(rootDomNode); root.render(<App />);
Output
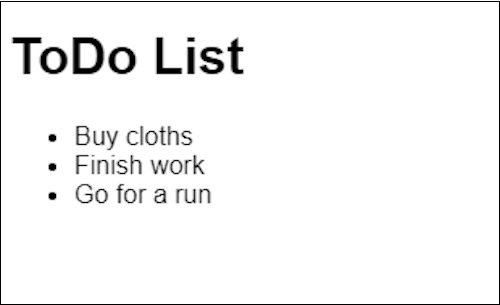
This is a simple React app that displays a list of todo tasks. We can modify or customize this program to meet our requirements, adding functionality like adding and deleting items, dates, and so on.
Updating a root component − If we want to make changes to what is already displayed on our web page using React, we can use createRoot to do this without changing the other parts of the page.
Example 3
App.js
export default function App({counter}) { return ( <> <h1>Hello, Tutorialspoint! {counter}</h1> <input placeholder="Type something here" /> </> ); }
index.js
import { createRoot } from 'react-dom/client'; import './styles.css'; import App from './App.js'; const root = createRoot(document.getElementById('root')); let i = 0; setInterval(() => { root.render(<App counter={i} />); i++; }, 1000);
Output

We can call render more than once on the same root.
Summary
The createRoot function in React JS is a way to create a new rendering area for our components. It allows us to have independent sections in our app by creating a fresh root ReactDOM render tree. This can be helpful for keeping different parts of our application organized and isolated. In simple terms, it provides a clean slate to render components in a separate container.
To Continue Learning Please Login