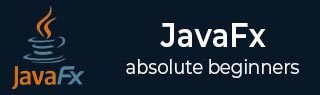
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX Animations
- JavaFX - Animations
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - Layout Panes VBox
If we use VBox as the layout in our application, all the nodes are set in a single vertical column.
The class named VBox of the package javafx.scene.layout represents the VBox pane. This class contains five properties, which are −
alignment − This property represents the alignment of the nodes inside the bounds of the VBox. You can set value to this property by using the setter method setAlignment().
fillHeight − This property is of Boolean type and on setting this to be true; the resizable nodes in the VBox are resized to the height of the VBox. You can set value to this property using the setter method setFillHeight().
spacing − This property is of double type and it represents the space between the children of the VBox. You can set value to this property using the setter method setSpacing().
In addition to these, this class also provides the following methods −
setVgrow() − Sets the vertical grow priority for the child when contained by a VBox. This method accepts a node and a priority value.
setMargin() − Using this method, you can set margins to the VBox. This method accepts a node and an object of the Insets class (A set of inside offsets for the 4 sides of a rectangular area)
Example
The following program is an example of the VBox layout. In this, we are inserting a text field and two buttons, play and stop. This is done with a spacing of 10 and each having margins with dimensions – (10, 10, 10, 10).
Save this code in a file with the name VBoxExample.java.
import javafx.application.Application; import javafx.collections.ObservableList; import javafx.geometry.Insets; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.control.TextField; import javafx.stage.Stage; import javafx.scene.layout.VBox; public class VBoxExample extends Application { @Override public void start(Stage stage) { //creating a text field TextField textField = new TextField(); //Creating the play button Button playButton = new Button("Play"); //Creating the stop button Button stopButton = new Button("stop"); //Instantiating the VBox class VBox vBox = new VBox(); //Setting the space between the nodes of a VBox pane vBox.setSpacing(10); //Setting the margin to the nodes vBox.setMargin(textField, new Insets(20, 20, 20, 20)); vBox.setMargin(playButton, new Insets(20, 20, 20, 20)); vBox.setMargin(stopButton, new Insets(20, 20, 20, 20)); //retrieving the observable list of the VBox ObservableList list = vBox.getChildren(); //Adding all the nodes to the observable list list.addAll(textField, playButton, stopButton); //Creating a scene object Scene scene = new Scene(vBox); //Setting title to the Stage stage.setTitle("Vbox Example"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
Compile and execute the saved java file from the command prompt using the following commands.
javac VBoxExample.java java VBoxExample.java
On executing, the above program generates a JavaFX window as shown below.
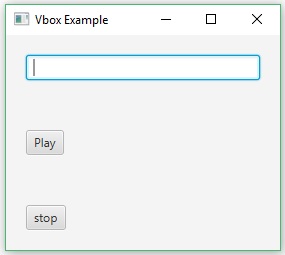