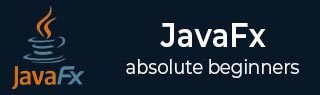
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX Animations
- JavaFX - Animations
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - Transformations
Transformation means changing some graphics into something else by applying rules. We can have various types of transformations such as Translation, Scaling Up or Down, Rotation, Shearing, etc.
Using JavaFX, you can apply transformations on nodes such as rotation, scaling and translation. All these transformations are represented by various classes and these belong to the package javafx.scene.transform.
S.No | Transformation & Description |
---|---|
1 | Rotation
In rotation, we rotate the object at a particular angle θ (theta) from its origin. |
2 | Scaling
To change the size of an object, scaling transformation is used. |
3 | Translation
Moves an object to a different position on the screen. |
4 | Shearing
A transformation that slants the shape of an object is called the Shear Transformation. |
Multiple Transformations
You can also apply multiple transformations on nodes in JavaFX. The following program is an example which performs Rotation, Scaling and Translation transformations on a rectangle simultaneously.
Save this code in a file with the name −
MultipleTransformationsExample.java.
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.paint.Color; import javafx.scene.shape.Rectangle; import javafx.scene.transform.Rotate; import javafx.scene.transform.Scale; import javafx.scene.transform.Translate; import javafx.stage.Stage; public class MultipleTransformationsExample extends Application { @Override public void start(Stage stage) { //Drawing a Rectangle Rectangle rectangle = new Rectangle(50, 50, 100, 75); //Setting the color of the rectangle rectangle.setFill(Color.BURLYWOOD); //Setting the stroke color of the rectangle rectangle.setStroke(Color.BLACK); //creating the rotation transformation Rotate rotate = new Rotate(); //Setting the angle for the rotation rotate.setAngle(20); //Setting pivot points for the rotation rotate.setPivotX(150); rotate.setPivotY(225); //Creating the scale transformation Scale scale = new Scale(); //Setting the dimensions for the transformation scale.setX(1.5); scale.setY(1.5); //Setting the pivot point for the transformation scale.setPivotX(300); scale.setPivotY(135); //Creating the translation transformation Translate translate = new Translate(); //Setting the X,Y,Z coordinates to apply the translation translate.setX(250); translate.setY(0); translate.setZ(0); //Adding all the transformations to the rectangle rectangle.getTransforms().addAll(rotate, scale, translate); //Creating a Group object Group root = new Group(rectangle); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Multiple transformations"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
Compile and execute the saved java file from the command prompt using the following commands.
javac MultipleTransformationsExample.java java MultipleTransformationsExample
On executing, the above program generates a JavaFX window as shown below.
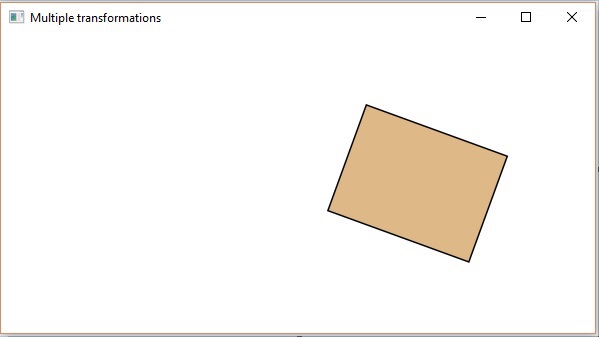
Transformations on 3D Objects
You can also apply transformations on 3D objects. Following is an example which rotates and translates a 3-Dimensional box.
Save this code in a file with the name RotationExample3D.java.
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.shape.Box; import javafx.scene.transform.Rotate; import javafx.scene.transform.Translate; import javafx.stage.Stage; public class RotationExample3D extends Application { @Override public void start(Stage stage) { //Drawing a Box Box box = new Box(); //Setting the properties of the Box box.setWidth(150.0); box.setHeight(150.0); box.setDepth(150.0); //Creating the translation transformation Translate translate = new Translate(); translate.setX(400); translate.setY(150); translate.setZ(25); Rotate rxBox = new Rotate(0, 0, 0, 0, Rotate.X_AXIS); Rotate ryBox = new Rotate(0, 0, 0, 0, Rotate.Y_AXIS); Rotate rzBox = new Rotate(0, 0, 0, 0, Rotate.Z_AXIS); rxBox.setAngle(30); ryBox.setAngle(50); rzBox.setAngle(30); box.getTransforms().addAll(translate,rxBox, ryBox, rzBox); //Creating a Group object Group root = new Group(box); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Drawing a cylinder"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
Compile and execute the saved java file from the command prompt using the following commands.
javac RotationExample3D.java java RotationExample3D
On executing, the above program generates a JavaFX window as shown below.
